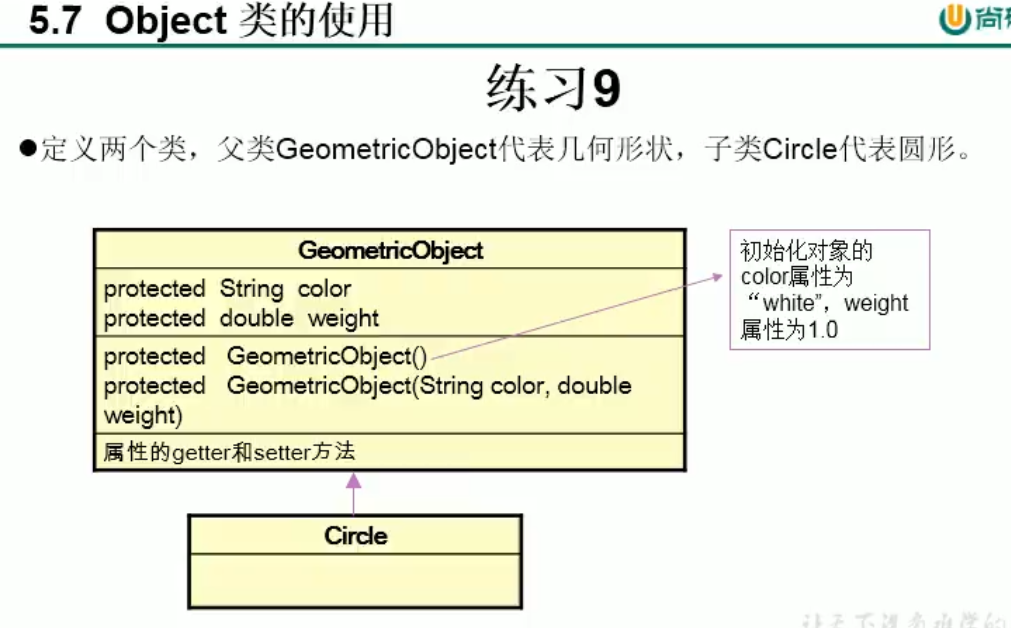
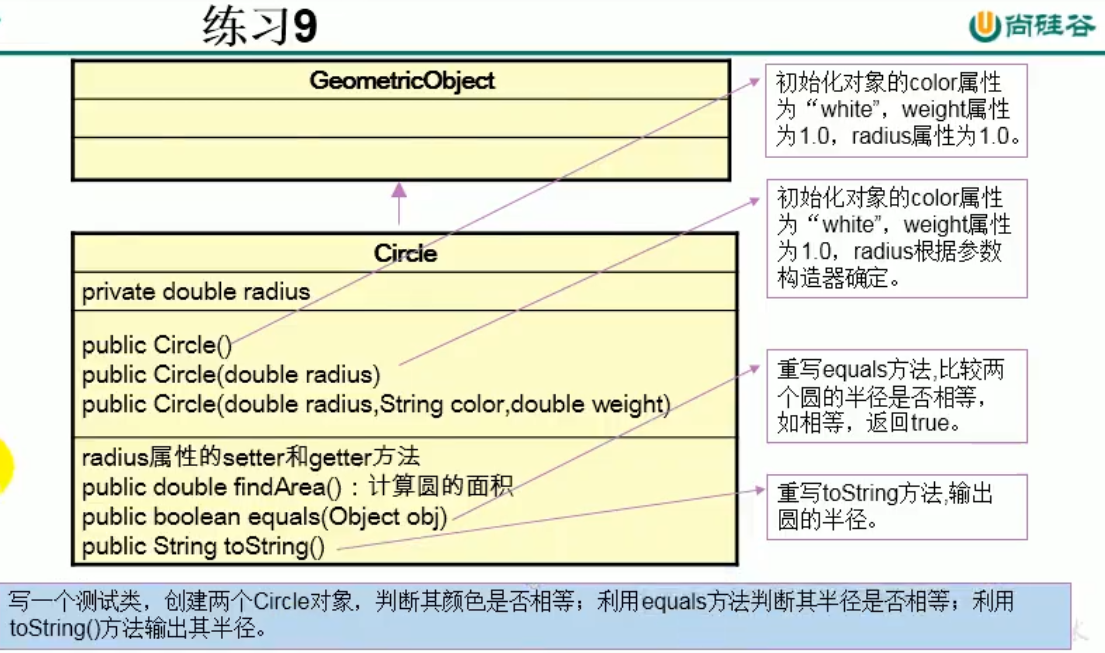
package com.atguigu.exercise5;
public class GeometricObject {//几何形状
//属性
protected String color;
protected double weight;
//构造器
protected GeometricObject(){
this.color = "white";
this.weight = 1.0;
}
protected GeometricObject(String color,double weight){
this.color = color;
this.weight = weight;
}
//方法
public String getColor(){
return color;
}
public void setColor(String color){
this.color = color;
}
public double getWeight() {
return weight;
}
public void setWeight(double weight) {
this.weight = weight;
}
}
package com.atguigu.exercise5;
public class Circle extends GeometricObject {// 圆形
// 属性
private double radius;
// 构造器
public Circle() {
super();
radius = 1.0;
// color = "white";
// weight = 1.0; // super()中已经包含了这两个属性
}
public Circle(double radius) {
super();
this.radius = radius;
}
public Circle(double radius, String color, double weight) {
super(color, weight);
this.radius = radius;
}
// 方法
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
// 计算圆的面积
public double findArea() {
return Math.PI * radius * radius;
}
//比较两个圆的半径是否相等,如果相等,返回true
@Override
public boolean equals(Object obj) {
if(this == obj){
return true;
}
if(obj instanceof Circle){
Circle c = (Circle)obj;
return this.radius == c.radius;
}
return false;
}
//输出圆的半径
@Override
public String toString() {
return "Circle [radius =" + radius + "]";
}
}
package com.atguigu.exercise5;
public class CircleTest {
public static void main(String[] args) {
Circle circle1 = new Circle(2.3);
Circle circle2 = new Circle(2.3, "white", 2.0);
//判断颜色是否相同
System.out.println("颜色是否相同:"+ circle1.getColor().equals(circle2.getColor()));
//判断半径是否相同
System.out.println("半径是否相同:"+ circle1.equals(circle2));
System.out.println(circle1);
System.out.println(circle2.toString());
}
}