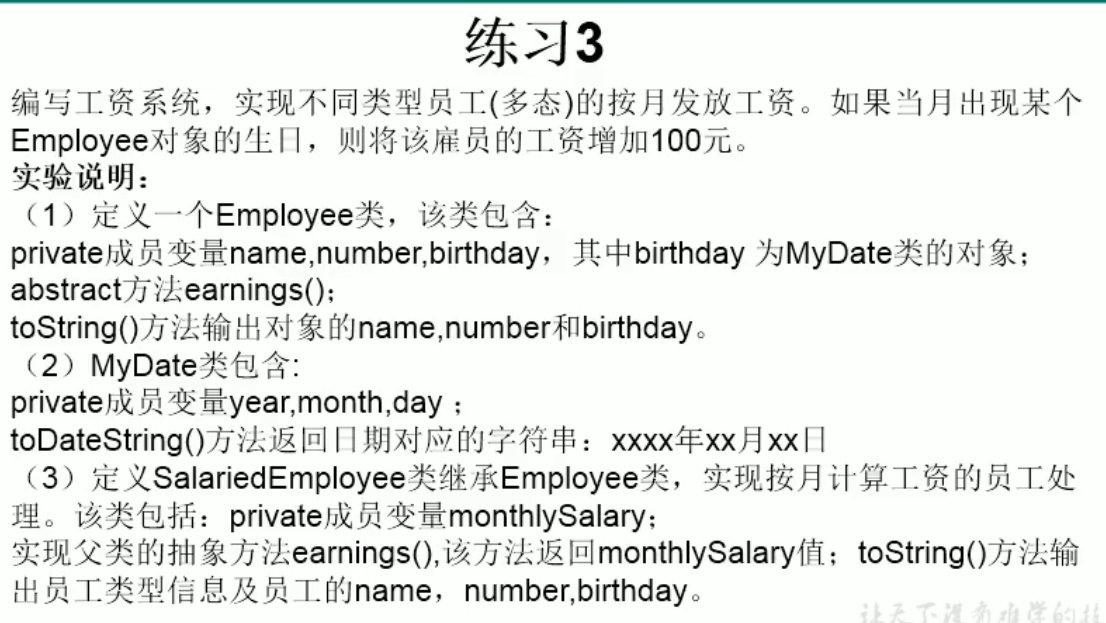
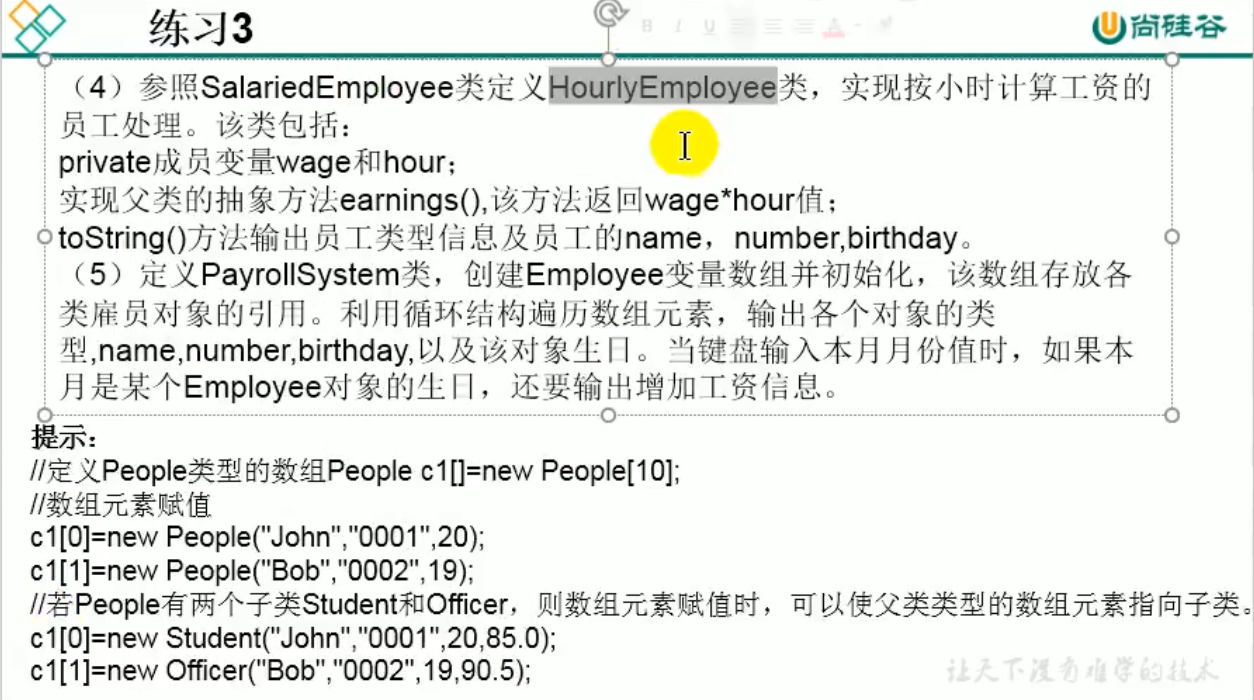
package com.atguigu.exercise2;
abstract public class Employee {
//属性
private String name;
private int number;
private MyDate birthday;
//构造器
public Employee(String name, int number, MyDate birthday) {
super();
this.name = name;
this.number = number;
this.birthday = birthday;
}
//方法
//抽象方法
public abstract int earnings();//收入
@Override
public String toString() {
return "name=" + name + ", number=" +
number + ", birthday=" + birthday.toDateString();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getNumber() {
return number;
}
public void setNumber(int number) {
this.number = number;
}
public MyDate getBirthday() {
return birthday;
}
public void setBirthday(MyDate birthday) {
this.birthday = birthday;
}
}
package com.atguigu.exercise2;
public class MyDate {
//属性
private int year;
private int month;
private int day;
//构造器
public MyDate(){
}
public MyDate(int year,int month,int day){
this.year = year;
this.month = month;
this.day = day;
}
//方法
public String toDateString() {
return year + "年" + month + "月" + day + "日";
}
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
public int getDay() {
return day;
}
public void setDay(int day) {
this.day = day;
}
}
package com.atguigu.exercise2;
public class SalariedEmployee extends Employee{
//属性
private int monthlySalary;//月工资
//构造器
public SalariedEmployee(String name, int number, MyDate birthday) {
super(name, number, birthday);
}
public SalariedEmployee(String name, int number, MyDate birthday,int monthlySalary) {
super(name, number, birthday);
this.monthlySalary = monthlySalary;
}
//方法
@Override
public int earnings() {
return monthlySalary;
}
@Override
public String toString() {
return "SalariedEmployee[name=" + getName() + ", number=" +
super.getNumber() + ", birthday=" + getBirthday() + "]";
}
public int getMonthlySalary() {
return monthlySalary;
}
public void setMonthlySalary(int monthlySalary) {
this.monthlySalary = monthlySalary;
}
}
package com.atguigu.exercise2;
public class HourlyEmployee extends Employee{
//属性
private int wage;//每小时的工资
private int hour;//月工作的小时数
//构造器
public HourlyEmployee(String name,int number,MyDate birthday) {
super(name, number, birthday);
}
public HourlyEmployee(String name,int number,MyDate birthday,int wage,int hour) {
super(name, number, birthday);
this.hour = hour;
this.wage = wage;
}
public int getWage() {
return wage;
}
//方法
public void setWage(int wage) {
this.wage = wage;
}
public int getHour() {
return hour;
}
public void setHour(int hour) {
this.hour = hour;
}
@Override
public int earnings() {
return wage*hour;
}
@Override
public String toString() {
return "HourlyEmployee ["+super.toString()+"]";
}
}
package com.atguigu.exercise2;
import java.util.Scanner;
public class PayrollSystem {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入当月的月份:");
int month = scanner.nextInt();
Employee[] emps= new Employee[2];
emps[0] = new SalariedEmployee("马胜",1002,new MyDate(1992,3,12),6500);
emps[1] = new HourlyEmployee("小明",2001,new MyDate(1991,5,4),60,240);
for(int i = 0;i < emps.length;i++){
System.out.println(emps[i]);//遍历员工信息
//结算工资
double salary = emps[i].earnings();
if(month == emps[i].getBirthday().getMonth()){
salary = emps[i].earnings();//有生日时的月工资
System.out.println("生日快乐,奖励100元!");
System.out.println("月工资为:" + (salary + 100));//月工资
}else{
System.out.println("月工资为:" + salary );
}
}
}
}