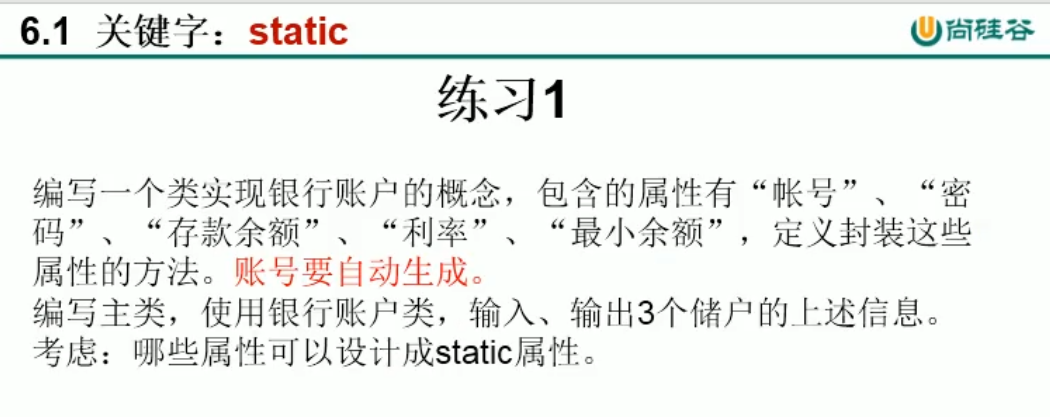
package com.atguigu.exercise2;
public class Account {
//属性
private int id;
private String pwd = "000000";//密码
private double balance;//余额
private static double interestRate;//利率
private static double minMoney = 1.0;//最小余额
private static int init = 1001;//用于自动生成id使用的
//构造器
public Account(){
id = init++;
}
public Account(String pwd,double balance){
id = init++;
this.pwd = pwd;
this.balance = balance;
}
//方法
public String getPwd() {
return pwd;
}
public void setPwd(String pwd) {
this.pwd = pwd;
}
public static double getInterestRate() {
return interestRate;
}
public static void setInterestRate(double interestRate) {
Account.interestRate = interestRate;
}
public static double getMinMoney() {
return minMoney;
}
public static void setMinMoney(double minMoney) {
Account.minMoney = minMoney;
}
public int getId() {
return id;
}
public double getBalance() {
return balance;
}
@Override
public String toString() {
return "Account [id=" + id + ", pwd=" + pwd + ", balance=" + balance + "]";
}
}
package com.atguigu.exercise2;
public class AccountTest {
public static void main(String[] args) {
Account acct1 = new Account();
Account acct2 = new Account("qwerty",2000);
System.out.println(acct1);
System.out.println(acct2);
}
}