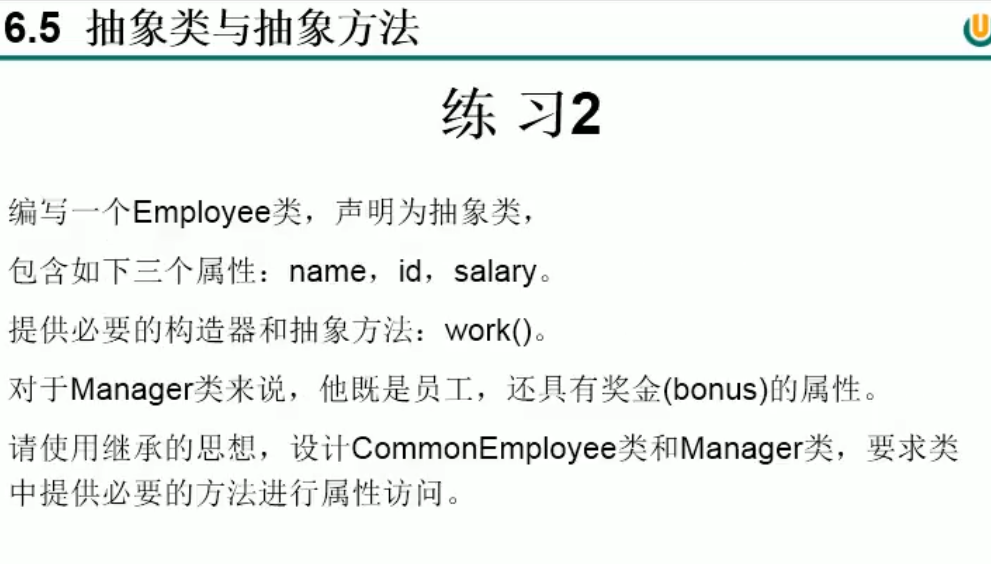
package com.atguigu.exercise1;
public abstract class Employee {//雇员
//属性
private String name;
private int id;
private int salary;//工资
//构造器
public Employee(){
}
public Employee(String name,int id,int salary){
this.name = name;
this.id = id;
this.salary = salary;
}
//方法
public abstract void work();
}
package com.atguigu.exercise1;
public class Manager extends Employee{//管理人员,经理
private int bonus;//奖金
//构造器
public Manager(){
}
public Manager(int bonus){
this.bonus = bonus;
}
public Manager(int bonus,String name,int id,int salary){
super();
this.bonus = bonus;
}
@Override
public void work() {
System.out.println("管理员工");
}
}
package com.atguigu.exercise1;
public class CommonEmployee extends Employee{//普通员工
@Override
public void work() {
System.out.println("员工在一线生产产品");
}
}
package com.atguigu.exercise1;
public class EmployeeTest {
public static void main(String[] args) {
//多态
Employee manager = new Manager(5000,"Tom",1001,50000);
manager.work();
CommonEmployee commonEmployee = new CommonEmployee();
commonEmployee.work();
}
}