- 一、张量的操作
- 1.2
torch.stack()
- if dim=0, 会将原有维度往后移动一个维度,在维度0新建一个维度进行拼接
- 03. 张量索引
- 04. 张量变换
- 二、张量的数学运算
- 三、线性回归
- -- coding: utf-8 --
- @Time : 2020/5/1 16:32
- @Author : DarrenZhang
- @FileName: linear regression.py
- @Software: PyCharm
- https://www.yuque.com/darrenzhang">@Blog :https://www.yuque.com/darrenzhang
- @Brief : Linear Regression Model
- create training data
- build linear regression model
- beginning to iterate
- 张量的操作:拼接、切分、索引和变换
- 张量的数学运算
- 线性回归
一、张量的操作
01. 张量拼接
1.1 torch.cat()
功能:将张量按维度dim进行拼接
功能:会创建一个新的维度,在新创建的维度dim上进行拼接
print(“\nt_stack:{} shape:{}”.format(t_stack, t_stack.shape))
> NOTE:
> - `cat()` 方法不会扩张张量的维度
> - `stack()` 方法会扩展张量的维度
<a name="0CkdJ"></a>
## 02. 张量切分
<a name="GTk4a"></a>
### 2.1 `torch.chunk()`
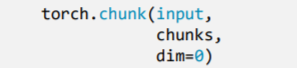
- 功能:将张量按维度 `dim` 进行平均切分
- 返回值:张量列表
- `input` : 要切分的张量
- `chunks` : 要切分的份数
- `dim` : 要切分的维度
> 注意事项:若不能整除,最后一份张量小于其他张量
```python
a = torch.ones((2, 7)) # 7
list_of_tensors = torch.chunk(a, dim=1, chunks=3) # 3
for idx, t in enumerate(list_of_tensors):
print("第{}个张量:{}, shape is {}".format(idx+1, t, t.shape))
- 结果:
2.2 torch.spilt()
- 功能:将张量按维度
dim
进行切分 - 返回值:张量列表
tensor
: 要切分的张量split_size_or_sections
: 为int时,表示每一份的长度;为list时,按list元素切分dim
: 要切分的维度t = torch.ones((2, 5)) list_of_tensors_01 = torch.split(t, 2, dim=1) # [2 , 1, 2] for idx, t in enumerate(list_of_tensors_01): print("第{}个张量:{}, shape is {}".format(idx + 1, t, t.shape))
t = torch.ones((2, 5)) list_of_tensors_02 = torch.split(t, [2, 1, 2], dim=1) # [2 , 1, 2] for idx, t in enumerate(list_of_tensors_02): print("第{}个张量:{}, shape is {}".format(idx+1, t, t.shape))
list_of_tensors_03 = torch.split(t, [2, 1, 1], dim=1) for idx, t in enumerate(list_of_tensors_03): print("第{}个张量:{}, shape is {}".format(idx, t, t.shape))
NOTE:
- 如果
split_size_or_sections
是 list 时,list中的元素之和一定要等于 dim 上的长度
- 如果
03. 张量索引
3.1 torch.index_select()
- 功能:在维度dim上,按index索引数据
返回值:依index索引数据拼接的张量
- input: 要索引的张量
- dim: 要索引的维度
- index : 要索引数据的序号
t = torch.randint(0, 9, size=(3, 3)) idx = torch.tensor([0, 2], dtype=torch.long) # float 类型会报错 t_select = torch.index_select(t, dim=0, index=idx) print("t:\n{}\nt_select:\n{}".format(t, t_select))
结果:
从一个3x3 的tensor中,dim=0,按行索引,索引值是[0, 2],只把第一行和第三行的值提取出来
3.2 torch.masked_select()
- 功能:按
mask
中的True
进行索引 返回值:一维张量
input
: 要索引的张量mask
: 与input
同形状的布尔类型张量t = torch.randint(0, 9, size=(3, 3)) mask = t.ge(5) # >=5 的返回True. ge is `>=` ; gt is `>` / other parameters:le lt t_select = torch.masked_select(t, mask) print("t:\n{}\nmask:\n{}\nt_select:\n{} ".format(t, mask, t_select))
结果:
04. 张量变换
4.1 torch.reshape()
- 功能:变换张量形状
input
: 要变换的张量shape
: 新张量的形状NOTE:
- 当张量在内存中是连续时,新张量与input共享数据内存
t = torch.randperm(8)
t_reshape = torch.reshape(t, (-1, 2, 2)) # -1
print("t:{}\nt_reshape:\n{}".format(t, t_reshape))
t[0] = 1024
print("t:{}\nt_reshape:\n{}".format(t, t_reshape))
print("t.data 内存地址:{}".format(id(t.data)))
print("t_reshape.data 内存地址:{}".format(id(t_reshape.data)))
- 结果
4.2 torch.transpose()
、 torch.t()
torch.transpose()
的功能:交换张量的两个维度input
: 要变换的张量dim0
: 要交换的维度dim1
: 要交换的维度
torch.t()
的功能:2维张量转置。对矩阵而言,等价于torch.transpose(input, 0, 1)
t = torch.rand((2, 3, 4)) t_transpose = torch.transpose(t, dim0=1, dim1=2) # c*h*w h*w*c print("t shape:{}\nt_transpose shape: {}".format(t.shape, t_transpose.shape))
4.3
torch.squeeze()
、torch.unsqueeze()
torch.squeeze()
功能:压缩长度为1的维度(轴)- dim: 若为None,移除所有长度为1的轴; 若指定维度,当且仅当该轴长度为1时,可以被移除;
torch.unsqueeze()
功能:依据dim
扩展维度dim
: 扩展的维度t = torch.rand((1, 2, 3, 1)) t_sq = torch.squeeze(t) t_0 = torch.squeeze(t, dim=0) t_1 = torch.squeeze(t, dim=1) t_2 = torch.unsqueeze(t, dim=2) print(t.shape) print(t_sq.shape) print(t_0.shape) print(t_1.shape) print(t_2.shape)
结果
torch.Size([1, 2, 3, 1]) torch.Size([2, 3]) torch.Size([2, 3, 1]) torch.Size([1, 2, 3, 1]) torch.Size([1, 2, 1, 3, 1])
二、张量的数学运算
分为三大类:
- 功能:逐元素计算 input+alpha×other(在残差连接中有重要应用)
input
: 第一个张量alpha
: 乘项因子other
: 第二个张量
- 加法优化代码API
三、线性回归
线性回归是分析一个变量与另外一(多个)变量之间关系的方法。公式:y = wx + b
- 确定模型 : Model : y = wx + b
- 选择损失函数 : MSE(均方误差):
- 求解梯度并且更新参数 w, b
- w = w - lr * w.grad
- b = b - lr * w.grad
```python
-- coding: utf-8 --
@Time : 2020/5/1 16:32
@Author : DarrenZhang
@FileName: linear regression.py
@Software: PyCharm
@Blog :https://www.yuque.com/darrenzhang
@Brief : Linear Regression Model
import torch import matplotlib.pyplot as plt torch.manual_seed(100)
lr = 0.001 # learning rate
create training data
x = torch.randn(20, 1) 10 print(x.shape) print(x.data) y = 2x + (5 + torch.randn(20, 1)) # y = wx + b + noise
build linear regression model
w = torch.randn(1, requires_grad=True) b = torch.zeros(1, requires_grad=True)
beginning to iterate
for iteration in range(10000):
# forward
wx = torch.mul(w, x)
y_pred = torch.add(wx, b)
# compute MSE loss
loss = (0.5 * (y - y_pred) ** 2).mean()
# backward
loss.backward()
# update parameters
b.data.sub_(lr * b.grad) # b_update = b - lr * b.grad
w.data.sub_(lr * w.grad)
# clean grad to zero
w.grad.zero_()
b.grad.zero_()
# draw the process
if iteration % 100 == 0:
plt.scatter(x.data.numpy(), y.data.numpy())
plt.plot(x.data.numpy(), y_pred.data.numpy(), 'r-', lw=5)
plt.text(2, 20, 'Loss=%.4f' % loss.data.numpy(), fontdict={'size': 20, 'color': 'red'})
plt.xlim(1.5, 10)
plt.ylim(8, 28)
plt.title("Iteration: {}\nw: {} b: {}".format(iteration, w.data.numpy(), b.data.numpy()))
plt.pause(0.5)
if loss.data.numpy() < 1:
break
```