/*
* EC(G)
* VO(G) / GO
* a「window.a」
*
* 变量提升:
* var a;
*/
console.log(a); //undefined
if (!('a' in window)) { //'a' in window===true
var a = 13;
}
console.log(a); //undefined
/!*
* EC(G)
* fn --> 0x000 {GO}
* 变量提升: function fn; 声明
*!/
console.log(fn); //undefined
// fn(); //Uncaught TypeError: fn is not a function
if ('fn' in window) {
/!*
* EC(BLOCK)
* fn --> 0x000 [[scope]]:EC(BLOCK)
* 作用域链:<EC(BLOCK),EC(G)>
* 变量提升:function fn(){...} 声明+定义
*!/
fn(); //“哈哈哈”
function fn() {
console.log('哈哈哈');
} //把之前对fn的操作,同步给全局一份
}
fn(); //“哈哈哈”
/!*
* EC(G)
* 变量提升:--
*!/
f = function () {return true;}; //window.f=function...
g = function () {return false;}; //window.g=function...
(function () {
/!*
* EC(AN)
* g
* 作用域链:<EC(AN),EC(G)>
* 形参赋值:--
* 变量提升:function g; 声明
*!/
// g() //报错:g is not a function
if ([] == ![]) {
/!*
* EC(BLOCK)
* g --> 0x000 [[scope]]:EC(BLOCK)
* 作用域链:<EC(BLOCK),EC(AN)>
* 变量提升:function g(){...} 声明+定义
*!/
f = function () {return false;} //修改了全局的f值
function g() {return true;} //EC(AN)中的g --> 0x000
}
})();
console.log(f()); //false
console.log(g()); //false
// []==![] => []==false => 0==0 => true
function sum(a) { //es6中没有变量提升,但是有一个自我检测的一个机制,在代码自上而下执行前,会先进行检测,
console.log(a); //经过了词法解析 所以报错
let a = 100;
console.log(a);
}
sum(200);
/*
* EC(G)
* obj --> 0x001 => {a:'包子',b:'馒头',name:'珠峰'}
* a = 'name'
* fn --> 0x000 [[scope]]:EC(G)
* 变量提升:var obj; var a; function fn(obj){...};
*/
var obj = {
a: '包子',
b: '面条'
};
var a = 'name';
function fn(obj) {
/*
* EC(FN)
* obj --> 0x001
* --> 0x002 => {1:1}
* 作用域链:<EC(FN),EC(G)>
* 形参赋值:obj=0x001
*/
console.log(obj); //=>{a:'包子',b:'面条'}
obj[a] = '珠峰'; //把变量a存储的值作为属性名去操作 obj['name']='珠峰'
obj.b = '馒头';
obj = {};
obj[1] = 1;
console.log(obj); //=>{1:1}
}
fn(obj); //fn(0x001)
console.log(obj); //=>{a:'包子',b:'馒头',name:'珠峰'}
var i = 5;
function fn(i) {
return function (n) {
console.log(n + (++i));
}
}
var f = fn(1);
f(2);//4
fn(3)(4);//8
fn(5)(6);//12
f(7);//10
console.log(i);//5
var i = 20;
function fn() {
i -= 2;
var i = 10;
return function (n) {
console.log((++i) - n);
}
}
var f = fn();
f(1);//console.log(11-1)=>10
f(2);//console.log(++11-2)=>12-2=>10
fn()(3);//console.log(++10-3)=>11-3=>8
fn()(4);//console.log(++10-4)=>11-4=>7
f(5);//console.log(++12-5)=>13-5=>8
console.log(i);//20
let x = 1;
function A(y){
let x = 2;
function B(z){
console.log(x+y+z);
}
return B;
}
let C = A(2);
C(3); //7 x=2 y=2 +z=3 2+2+3=7
//EC(G)
// VO(G)/Go
// fn======>x001
// x====>5
// f======>X002
// 代码提升
// fn
let x = 5;
function fn(x) {
return function(y) {
console.log(y + (++x));
}
}
let f = fn(6);
//EC(Fn)
// x===》6=》7
// return X002;//{function(y){console.log(y+(++x))}}
f(7); //14 执行 x002(7)
fn(8)(9);//18
//EC(Fn2)
// x===>8 9
// return x003;//{function(y){console.log(y+(++x))}}
// EC(AN) X003(9)
// Scope<EC(AN),EC(Fn2)>
// y=====>9
// consoloe.log(y+(++x)) 9+9=18
f(10);//15
//x002(10)=>
// EC(f2)
// Scope<EC(f2),EC(f1)>
// AO
// y====10 console.log(y+(++x); 10+8=18
console.log(x);//5
//=>如果去掉function fn(x)的x呢?
let a=0,
b=0;
function A(a){
A=function(b){
alert(a+b++);
};
alert(a++);
}
A(1);//2
A(2);//4
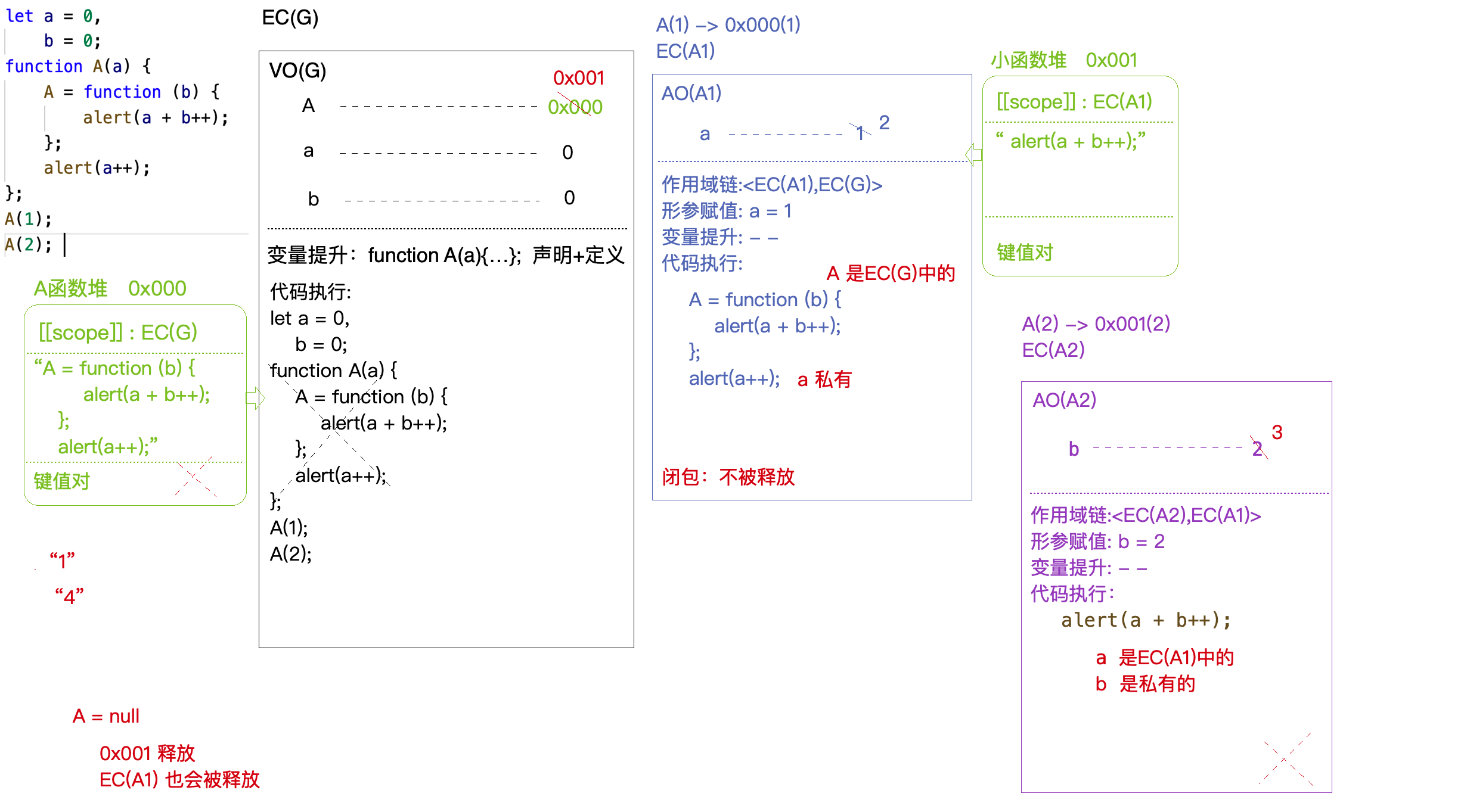