需求一:推盒子按照上下左右箭头 移动盒子
onkeydown 事件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.box {
position: relative;
left: 0px;
top: 0px;
width: 100px;
height: 100px;
background: lightcoral;
}
</style>
</head>
<body>
<div class="box"></div>
<script>
let box = document.querySelector('.box');
//keypress 有一些系统键监听不到
// key
let t = 0, l = 0;
window.onkeydown = function (e) {
// e.key e.code e.keyCode 表示按下的键盘信息
// e.key按的哪个键 e.code是英文键盘字符 e.keyCode是键盘数组
switch (e.keyCode) {
case 37:
// 左箭头
l -= 5;
box.style.left = l + 'px';
break;
case 38:
// 上箭头
t -= 5;
box.style.top = t + 'px';
break;
case 39:
l += 5;
box.style.left = l + 'px';
// 右箭头
break;
case 40:
// 下箭头
t += 5;
box.style.top = t + 'px';
break;
}
}
</script>
</body>
</html>
需求二 拖拽
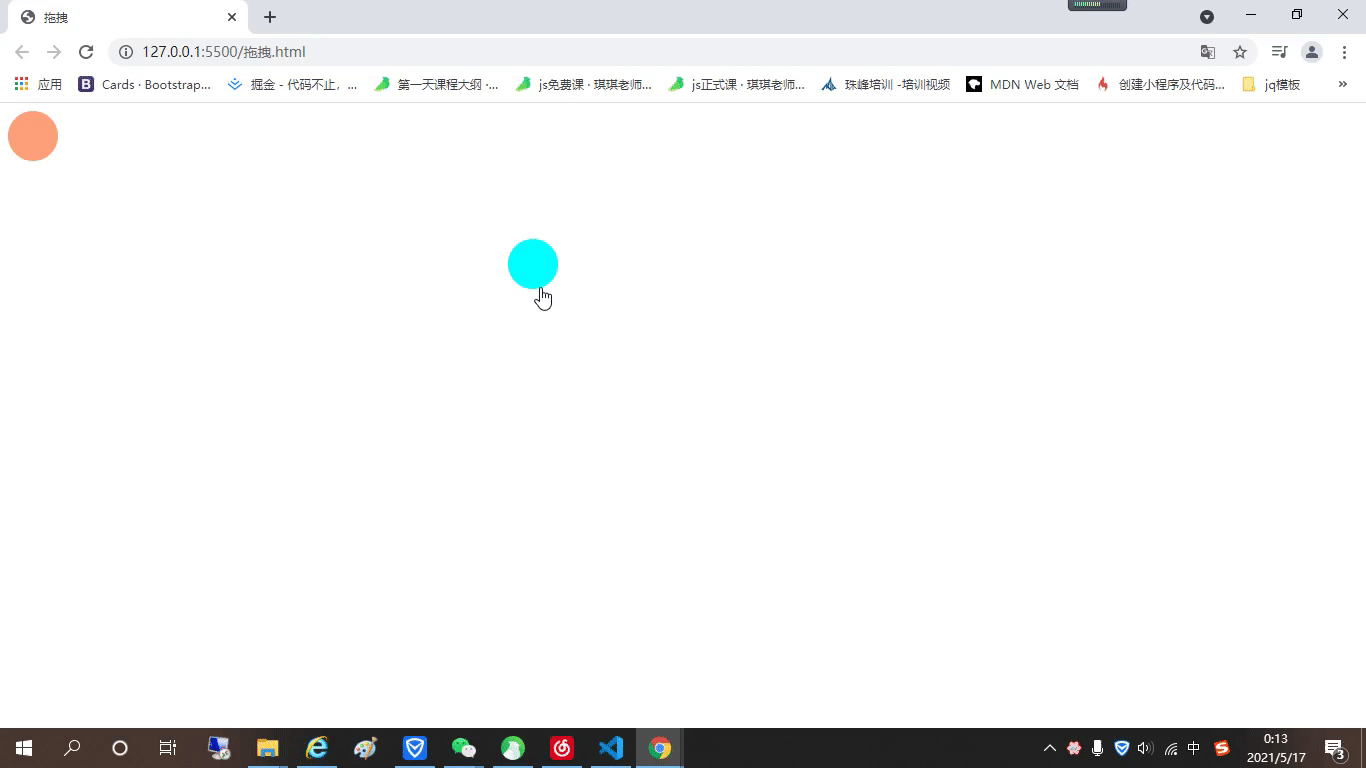
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>拖拽</title>
<style>
#box {
position: fixed;
width: 50px;
height: 50px;
background-color: lightsalmon;
border-radius: 50%;
cursor: pointer;
/* 用户禁止选中 */
-webkit-user-select: none;
-moz-user-select: none;
-o-user-select: none;
user-select: none;
}
#box2{
position: fixed;
width: 50px;
height: 50px;
background-color:aqua;
border-radius: 50%;
cursor: pointer;
-webkit-user-select: none;
-moz-user-select: none;
-o-user-select: none;
user-select: none;
}
/* 其实mouseup并没有失效,而是你拖动时,鼠标选中了其他的元素,其实的话,鼠标即使松开,浏览器内部还是认为用户在复制文字,鼠标还是按下的状态,所以不会触发mouseup事件。 */
</style>
</head>
<body>
<div id="box">
</div>
<div id="box2">
</div>
<script>
let box = document.querySelector('#box');
let box2= document.querySelector('#box2');
function start(e) {
// 计算最大宽度和高度
this.maxTop = document.documentElement.clientHeight - this.offsetHeight;
this.maxLeft = document.documentElement.clientWidth - this.offsetWidth;
//记录球的初始位置和鼠标按下的位置
let pos = this.getBoundingClientRect();
// 球的初始位置
this.posLeft = pos.left;
this.postTop = pos.top;
// 鼠标按下的位置
this.startX = e.pageX;
this.startY = e.pageY;
// 为了下面的move和end正常拿到球的this,强制改变this指向,把改变this指向后的函数体绑定给window。
// this从window改成了球
// this._move是存储改变this指向之后的函数体
// 把所有需用用到的属性和方法都放在this上,
this._move = move.bind(this);
window.addEventListener('mousemove', this._move);
this._end = end.bind(this);
window.addEventListener('mouseup', this._end);
};
function move(e) {
// 改变之后的this是鼠标按下的元素
// 计算鼠标移动的距离【鼠标移动后的位置-鼠标移动前的位置】+球的初始位置=球最后的位置
let top = e.pageY - this.startY + this.postTop;
let left = e.pageX - this.startX + this.posLeft;
// 让top/left分别和最大值最小值比较,划定界限
top = top > this.maxTop ? this.maxTop : (top < 0 ? 0 : top);
left = left > this.maxLeft ? this.maxLeft : (left < 0 ? 0 : left);
// top left 就是最后球的位置
this.style.top=top+'px';
this.style.left=left+'px';
};
function end() {
window.removeEventListener('mousemove', this._move);
window.removeEventListener('mouseup', this._end);
};
box.addEventListener('mousedown', start);
box2.addEventListener('mousedown',start);
</script>
</body>
</html>