- 编程题 提示用户输入年月日信息,判断这一天是这一年中的第几天并打印。 ```java / 提示用户输入年月日信息,判断这一天是一年中的第几天 /
import java.util.Scanner;
public class PrintWhatDay {
//0-声明两个数组分别表示平年与闰年12个月的天数
static int[] arr1 = {31,28,31,30,31,30,31,31,30,31,30,31};
static int[] arr2 = {31,29,31,30,31,30,31,31,30,31,30,31};
public static void main(String[] args) {
//1. 提示用户输入年月日
System.out.println("请依次输入 年 月 日: ");
Scanner sc = new Scanner(System.in);
int year = sc.nextInt();
int month = sc.nextInt();
int day = sc.nextInt();
//2. 调用函数计算总天数
System.out.println(countDays(year, month, day));
}
private static int countDays(int year, int month, int day) { //注意:[静态方法]不能调用 [非 静 态 方 法]
//1. 判断当日year是平年还是闰年
//能被4整除,并且不能被100整除 || 能被400整除
int[] arr = arr1;
if( (year%4 == 0 && year%100 != 0) || (year%400 == 0) ) {
arr = arr2; //将arr指向arr2
}
//2. 计算总天数
int countdays = 0;
for(int i = 0; i < month-1; i++) {
countdays += arr[i];
}
countdays += day;
return countdays;
}
}
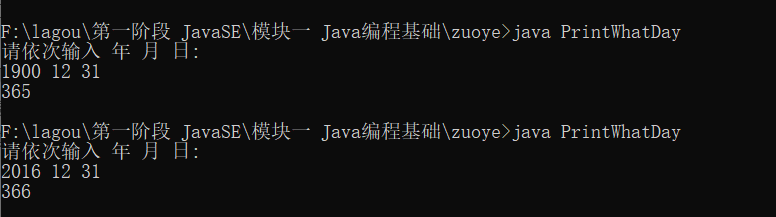
2. 编程题 编程找出 1000 以内的所有完数并打印出来。 所谓完数就是一个数恰好等于它的因子之和,如:6=1+2+3
```java
/*
编程找出 1000 以内的所有完数并打印出来。
所谓完数就是一个数恰好等于它的因子之和
如:6=1+2+3
*/
import java.lang.Math;
public class WanShu {
public static void main(String[] args) {
int sum = 0;
for(int i = 1; i < 1001; i++) {
for(int j = 2; j <= (int)(Math.sqrt(i)); j++) { //注 :使用sqrt降低算法时间复杂度
if(i%j == 0) {
sum += (j==i/j)?j:(j + i/j); //因子是成对出现的
}
}
if(++sum == i) {
System.out.println(i);
}
//System.out.println(i+":"+sum);
sum = 0; //每次循环sum需要重置
}
}
}
- 编程题 实现双色球抽奖游戏中奖号码的生成,中奖号码由 6 个红球号码和 1 个蓝球号码组成。 其中红球号码要求随机生成 6 个 1~33 之间不重复的随机号码。 其中蓝球号码要求随机生成 1 个 1~16 之间的随机号码。 ```java /* 实现双色球抽奖游戏中奖号码的生成 中奖号码由 6 个红球号码和 1 个蓝球号码组成。 其中红球号码要求随机生成 6 个 1~33 之间不重复的随机号码。 其中蓝球号码要求随机生成 1 个 1~16 之间的随机号码。
*/ import java.util.Random; import java.util.Arrays;
public class DoubleColorSphere { public static void main(String[] args) throws Exception { int[] blueSphere = new int[6]; Random ra = new Random();
/*2-循环摇号,碰到重复重新摇*/
for(int i = 0; i < blueSphere.length; i++) {
blueSphere[i] = ra.nextInt(33)+1;
if(i > 0 && checkNum(blueSphere, i, blueSphere[i])) {
System.out.println("号码"+blueSphere[i]+"重复,重新摇......");
Thread.sleep(1000); // 表示模拟睡眠1秒的效果
/*注意这里需要 -1 */
i--;
}
}
System.out.println("本期开奖号码为:" + Arrays.toString(blueSphere).replace(",", "")+" + "+(ra.nextInt(16)+1));
}
/*查询arr数组中的前len个元素是否存在var相等的变量*/
private static boolean checkNum(int[] arr, int len, int var) {
for(int i = 0; i < len; i++) {
if(arr[i] == var) {
//System.out.println(var+" "+ arr[i]);
return true;
}
}
return false;
}
}

4. 编程题 自定义数组扩容规则,当已存储元素数量达到总容量的 80%时,扩容 1.5 倍。 例如,总容量是 10,当输入第 8 个元素时,数组进行扩容,容量从 10 变 15。
```java
/*
自定义数组扩容规则 当已存储元素数量达到总容量的 80%时,扩容 1.5 倍。
例如,总容量是 10,当输入第 8 个元素时,数组进行扩容,容量从 10 变 15。
*/
import java.util.Random;
import java.util.Arrays;
public class ExpandArray {
public static void main(String[] args) throws Exception {
//1. 定义数组
int[] arra = new int[10];
int highNum = arra.length*8/10; //预警值
Random ra = new Random();
int i = 0;
//2. 用for循环赋值,当已存储元素数量达到总容量的80%,扩容处理
while(true) {
arra[i++] = ra.nextInt(10);
if(i >= highNum) {
System.out.println("扩容中......");
//System.out.println("before:"+Arrays.toString(arra));
Thread.sleep(1500); // 模拟睡眠1500ms的效果
int newLen = arra.length*15/10;
//int newLen = (arra.length + arra.length>>1);
System.out.println("扩容后的长度:"+newLen);
int[] newArr = new int[newLen];
System.arraycopy(arra, 0, newArr, 0, highNum);
arra = newArr;
//System.out.println("After:"+Arrays.toString(arra));
highNum = arra.length*8/10;
}
}
}
}
- 编程题 使用二维数组和循环实现五子棋游戏棋盘的绘制,具体如下:
/*
编程题 使用二维【整型】数组和循环实现五子棋游戏棋盘的绘制
*/
public class DrawPannel {
public static void main(String[] args) {
int[][] arr = new int[17][17];
/*1. 数组的初始化操作*/
for(int i = 0x0; i <= 0xf+1; i++) {
for(int j = 0x0; j <= 0xf+1; j++) {
if( (i==0 || j==0) && (i + j > 0)) {
arr[i][j] = i+j-1;
}
}
}
/*2. 打印数组元素*/
for(int i = 0x0; i <= 0xf+1; i++) {
for(int j = 0x0; j <= 0xf+1; j++) {
if( i+j == 0)
System.out.print(" ");
else if(i==0 || j==0) {
System.out.printf("%x ", arr[i][j]); //格式化输出
}
else {
System.out.print("+ ");
}
}
System.out.println();
}
}
}