1. 异常机制(重点)
1.1 基本概念
- java.lang.Throwable类是Java语言中错误和异常的超类
- 其中Exception类主要用于描述由于编程错误或偶然外在因素导致的轻微错误,通常可以编码解决,如:0作为除数等。
AirthmeticException-算数异常错误
ArrayIndexOutOFBoundsException-数组下标越界异常
NullPointerException-空指针异常错误
ClassCastException-类型转换错误
NumberFormatException-数据格式异常
1.2 异常的分类
- 运行时异常
- 非检异常 -在编译阶段不会被编译器检测出来
其他异常-输入输出异常和其他异常
- 检测性异常
运行时异常:
- AirthmeticException-算数异常错误
- ArrayIndexOutOFBoundsException-数组下标越界异常
- NullPointerException-空指针异常错误
- ClassCastException-类型转换错误
- NumberFormatException-数据格式异常 ```java package com.lagou.task16;
/**
- @author 西风月
- @date 2020/8/3
@description */ public class ExceptionTest { public static void main(String[] args) throws InterruptedException {
//1_非检测性异常
//System.out.println(5/0); //编译正常, 运行时会发生算数异常 ArithmeticException, 下面的代码不会执行
//2-检测性异常
Thread.sleep(1000); //编译报错, 不处理就无法运行到运行阶段 java.lang.InterruptedException
System.out.println("程序正常结束!");
} } ``` 注意: **
当程序执行过程中出现异常但没有手动处理时, 则有Java虚拟机采用默认方式处理异常, 而默认处理方式就是: 打印异常的名称、异常发生原因、异常发生的位置以及终止程序。**1.3 异常的避免
```bash package com.lagou.task16;
import java.io.IOException;
/**
- @author 西风月
- @date 2020/8/3
- @description
*/
public class ExceptionPreventTest {
public static void main(String[] args) {
int ia = 10; int ib = 0; if(0 != ib) { System.out.println(ia / ib); //算数异常 ArithmeticException }
int[] arr = new int[5];
int pos = 5;
if(pos >= 0 && pos < 5) {
System.out.println(arr[pos]); //数组下标越界异常->ArrayIndexOutOfBoundsException
}
String str = null;
if(null != str) {
System.out.println(str.length()); //空指针异常-NullPointerException
}
Exception ex = new Exception();
if(ex instanceof IOException) {
IOException ie = (IOException) ex; //类型转换异常-ClassCastException
}
String str1 = "123a";
if(str1.matches("\\d+")) {
System.out.println(Integer.parseInt(str1)); //数据格式异常-NumberFormatException
}
System.out.println("程序总算正常结束了!");
}
}
- 增加条件判断避免异常的缺点:
- 造成代码臃肿
- 可读性较差
<a name="ddvAE"></a>
## 1.4 异常的捕获
<a name="ffOel"></a>
## <br />
<a name="48ln8"></a>
## 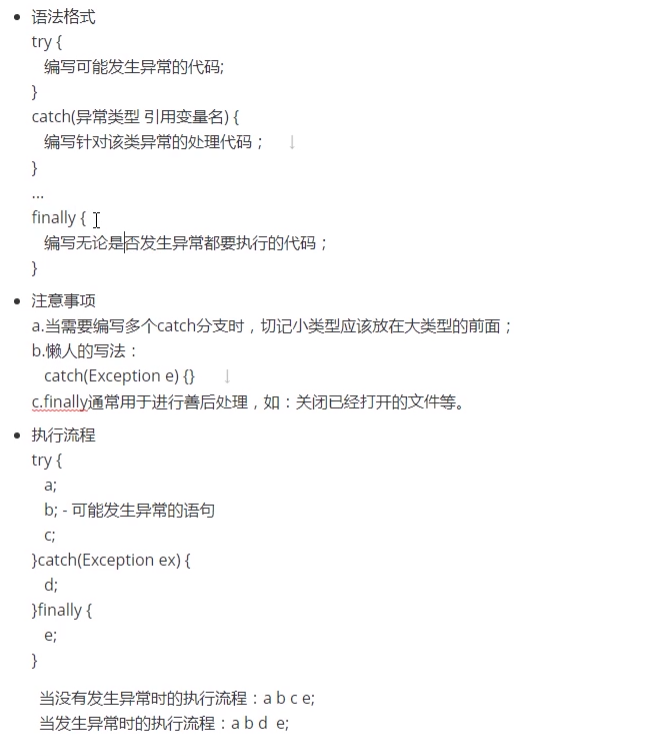
```java
package com.lagou.task16;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
/**
* @author 西风月
* @date 2020/8/3
* @description
*/
public class ExceptionCatchTest {
public static void main(String[] args) {
//创建一个FileInputStream类型的对象于d:/a.txt文件相关联, 打开文件
FileInputStream fis = null; //FileNotFoundException-编译报错:文件不存在 --> 检测性异常
try {
System.out.println("1");
//当程序执行过程中发生了异常后直奔catch分支进行处理
fis = new FileInputStream("e:/a.txt");
System.out.println("2");
} catch (FileNotFoundException e) {
System.out.println("3");
e.printStackTrace();
System.out.println("4");
}
// 关闭文件
try {
System.out.println("5");
fis.close();
System.out.println("6");
} /*catch (Exception e) { //小类型的要放在大类型之前
e.printStackTrace();
}*/
/*catch (IOException e) { //在开发中更推荐使用上面这种写法, 可读性
System.out.println("7");
e.printStackTrace();
System.out.println("8");
} catch (NullPointerException e) {
e.printStackTrace();
}*/ catch (Exception e) { //多态
e.printStackTrace();
}
System.out.println("世界上最真情得相依就是你在try, 我在catch, 无论你发什么脾气我都默默承受并静静得处理,到那时再来期待我们得finally!");
//当程序没有发生异常得执行流程是: 1-2-5-6 世界上...
//当程序执行过程中发生异常又没有手动处理空指针的执行流程 1-3-4-5 空指针异常导致程序终止
//当程序执行过程中发生NullPointerException时得执行流程 1-3-4-5 世界上......
//手动处理异常和没有处理的区别: 代码是否可以继续向下执行
}
}
package com.lagou.task16;
/**
* @author 西风月
* @date 2020/8/8
* @description
*/
public class ExceptionFinallyTest {
public static int test() {
try {
int[] arr = new int[5];
System.out.println(arr[5]);
return 0;
} catch (ArrayIndexOutOfBoundsException e) {
e.printStackTrace();
return 1;
} finally {
return 2; //提前结束方法并返回数据
}
}
public static void main(String[] args) {
try {
int ia = 10;
int ib = 0;
//int ib = 1;
System.out.println(ia/ib);
System.out.println("No Exception!");
} catch (ArithmeticException e) {
e.printStackTrace();
String str1 = null;
//str1.length(); //会发生空指针异常
} finally {
System.out.println("无论是否发生异常都记得来执行我哦!"); //依然执行
}
System.out.println("Over! "); //不执行
System.out.println("===============");;
//笔试考点
int test = test();
System.out.println(test); //2
}
}
1.5 异常的抛出
异常的生成
- 孩子不能比爹更坏
1.6 自定义异常类
- 序列化版本号
- 于序列化操作有关系
package com.lagou.task16;
/**
* @author 西风月
* @date 2020/8/9
* @description
*/
public class AgeException extends Exception {
static final long serialVersionUID = -3387516993124228878L;
public AgeException() {
}
public AgeException(String message) {
super(message);
}
}
package com.lagou.task16;
/**
* @author 西风月
* @date 2020/8/9
* @description
*/
public class Person {
private String name;
private int age;
public Person() {
}
public Person(String name, int age) /*throws AgeException*/ {
setName(name);
setAge(age);
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) /*throws AgeException*/ {
if(age > 0 && age < 150) {
this.age = age;
} else {
//System.out.println("年龄不合理哦!!!");
try {
throw new AgeException("年龄不合理哦!!!");
} catch (AgeException e) {
e.printStackTrace();
}
}
}
}
package com.lagou.task16;
/**
* @author 西风月
* @date 2020/8/9
* @description
*/
public class PersonTest {
public static void main(String[] args) {
/*Person p1 = null;
try {
p1 = new Person("张飞", -30);
} catch (AgeException e) {
e.printStackTrace();
}
System.out.println("p1 = " + p1);*/
Person p1 = new Person("zhangfei", -30);
System.out.println("p1 = " + p1); //当向上抛出异常则p1为null(构造方法还未结束就被抛出异常),采用就地处理的方式则不然
}
}
2. File类(重点)
2.1 基本概念
2.2 常用的方法
package com.lagou.task16;
import java.io.File;
import java.io.FileFilter;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.time.LocalDateTime;
import java.util.Date;
/**
* @author 西风月
* @date 2020/8/11
* @description
*/
public class FileTest {
//自定义成员方法实现当前目录跟子目录中所有元素的打印
public static void show(File file, String pre) {
File[] filesArray = file.listFiles();
assert filesArray != null;
for (File tf : filesArray) {
//判断是否为文件,若是则直接打印目录名称
String name = tf.getName();
if(tf.isFile()) {
System.out.println(pre+name);
}
else if(tf.isDirectory()) {
System.out.println(pre + "[" + name + "]");
show(tf, pre+" ");
}
}
}
public static void main(String[] args) throws IOException {
//1. 构造File类型的对象与 F:/a.txt关联
File f1 = new File("F:/a.txt");
//2. 若文件存在则获取文件的相关特征并打印后删除文件
if(f1.exists()) {
System.out.println("文件的名称是:" + f1.getName());
System.out.println("文件的大小是:" + f1.length());
Date d1 = new Date(f1.lastModified());
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
System.out.println("文件的最后一次修改时间: " + sdf.format(d1));
//绝对路径 如c:/ d:/ /..
//相对路径 ../ ./
System.out.println("文件的绝对路径:" + f1.getAbsolutePath());
System.out.println(f1.delete() ? "文件删除成功!" : "文件删除失败!");
} else {
//3. 若文件不存在则创建新文件
System.out.println(f1.createNewFile() ? "文件创建成功!" : "文件创建失败!" );
}
//4. 实现目录的删除和创建
File f2 = new File("f:/捣乱/猜猜我是谁/你猜我猜不猜/死鬼");
if(f2.exists()) {
System.out.println("目录名称是:" + f2.getName());
System.out.println(f2.delete() ? "目录删除成功!" : "目录删除失败!");
} else {
System.out.println(f2.mkdir() ? "目录创建成功!" : "目录创建失败!"); //创建单层目录
//System.out.println(f2.mkdirs() ? "目录创建成功!" : "目录创建失败!"); //创建多级目录
}
System.out.println("----------------------------------");
//5. 实现将指定目录中的所有文件打印出来
File f3 = new File("F:/捣乱");
File[] filesArray = f3.listFiles();
for (File tf : filesArray) {
//判断是否为文件,若是则直接打印目录名称
String name = tf.getName();
if(tf.isFile()) System.out.println(name);
else if(tf.isDirectory()) System.out.println("[" + name + "]");
}
System.out.println("----------------------------------");
//6. 实现目录中所有内容获取的同时进行过滤
//匿名内部类的语法格式 接口/父类类型 引用变量名 = new 接口/父类类型() { 方法的重写 };
// FileFilter fileFilter = new FileFilter() {
// @Override
// public boolean accept(File pathname) {
// //若文件名是以.avi结尾的则返回 true, 否则返回 false就是表示丢弃
// return pathname.getName().endsWith(".avi");
// }
// };
//lambda表达式的使用
FileFilter fileFilter = (File pathname) -> {return pathname.getName().endsWith(".avi");};
File[] filesArray2 = f3.listFiles(fileFilter);
for(File tf : filesArray2) {
System.out.println(tf);
}
System.out.println("----------------------------------");
show(new File("f:/捣乱"), "");
}
}
3. 任务总结
- 异常机制(重点)
- 概念
- 分类
- 避免的异常的发生
- 对异常进行捕获处理
- 抛出异常,抛给调用者处理
- 自定义异常类
- File(类)
- 概念
- 文件的管理
- 目录的管理
- 目录的遍历