1.1 String类的概念
java.lang.String 无需import即可使用类
public final class String
extends Object
implements Serializable, Comparable<String>, CharSequence
private final byte[] value; //常量
比较接口,字符序列的接口
所有的字符串都可以用String类来表述
- 从jdk1.9开始,该类的底层不再使用char[],而使用byte[],从而节约了空间。
- 该类描述的字符串是个常量 不可以更改,因此可以被共享使用。
/**
- @author 西风月
- @date 2020/7/26
- @description
*/
public class StringPoolTest {
public static void main(String[] args) {
} }//验证一下常量池的概念 // 目前为止,只有String这个特殊类除了new的方式之外还可以直接字符串赋值(包装类除外) String str1 = "abc"; String str2 = "abc"; System.out.println(str1 == str2);
<a name="H5Dgz"></a>
# 1.3 常用的构造方法
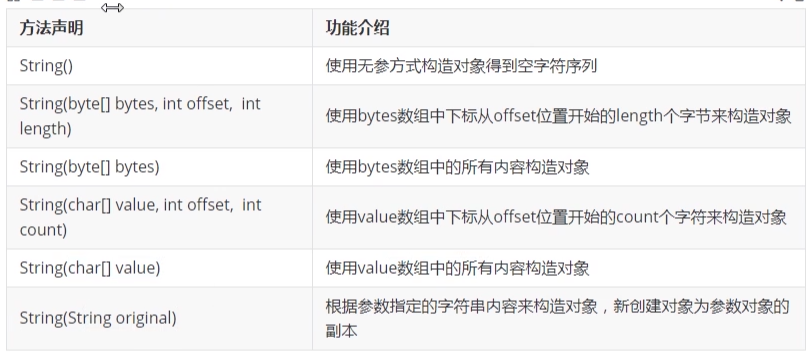
```java
package com.lagou.task12;
/**
* @author 西风月
* @date 2020/7/26
* @description
*/
public class StringConstructorTest {
public static void main(String[] args) {
String str1 = new String();
System.out.println("str1 = " + str1);
System.out.println("-----------------------------------------------");
//a == 'a'
byte[] arr = {97, 98, 99, 100, 101};
//构造字符串的思路:就是先将每个整数翻译成对应的字符,再将所有的字符串起来。
String str2 = new String(arr, 1, 3);
System.out.println("str2 = " + str2);
String str3 = new String(arr);
System.out.println("str3 = " + str3);
System.out.println("-----------------------------------------------");
char[] carr = {'h', 'e', 'l', 'l','0'};
String str4 = new String(carr, 0, 3);
System.out.println("str4 = " + str4);
String str5 = new String(carr);
System.out.println("str5 = " + str5);
System.out.println("-----------------------------------------------");
String str6 = new String("world");
System.out.println("str6 = " + str6);
}
}
String类的笔试考点
- 双引号与new方式初始化的区别
- 常量池 / 堆区
- 常量优化机制
package com.lagou.task12;
/**
* @author 西风月
* @date 2020/7/26
* @description
*/
public class StringExamTest {
public static void main(String[] args) {
//1. 请问下面代码会创建几个对象,分别存放在什么位置
//String str1 = "hello"; //常量池
//String str1 = new String("hello"); //两个对象, 一个在常量池中, 1个在堆区中
String str1 = "hello";
String str2 = "hello";
String str3 = new String("hello");
String str4 = new String("hello");
System.out.println(str1 == str2);
System.out.println(str1.equals(str2));
System.out.println(str3 == str4);
System.out.println(str3.equals(str4));
System.out.println(str2 == str4);
System.out.println(str2.equals(str4));
System.out.println("-------------------------------------------------------------");
//3. 常量的优化机制
String str5 = "abcd";
String str6 = "ab" + "cd"; //常量优化机制
System.out.println(str5 == str6); //ture
String str7 = "abcd";
String str8 = str7 + ""; //没有常量优化
System.out.println(str5 == str8);
}
}
1.4 常用的成员方法
package com.lagou.task12;
import java.util.Arrays;
/**
* @author 西风月
* @date 2020/7/26
* @description
*/
public class StringByteCharTest {
public static void main(String[] args) {
//1. 创建String对象并打印
String str1 = "world";
byte[] barr = str1.getBytes();
System.out.println(Arrays.toString(barr));
String str2 = new String(barr);
System.out.println("str2 = " + str2);
char[] carr = str1.toCharArray();
System.out.println(Arrays.toString(carr));
String str3 = new String(carr);
System.out.println("str3 = " + str3);
}
}
package com.lagou.task12;
/**
* @author 西风月
* @date 2020/7/26
* @description
*/
public class StringJudgeTest {
public static void main(String[] args) {
String str1 = "上海自来水来自海上";
int i = 0;
int j = str1.length() - 1;
while(i < j) {
if(str1.charAt(i++) == str1.charAt(j--)) continue;
System.out.println(str1 + "不是回文");
return;
}
System.out.println(str1 + "是回文");
}
}
package com.lagou.task12;
/**
* @author 西风月
* @date 2020/7/26
* @description
*/
public class StringCompareTest {
public static void main(String[] args) {
String str1 = "hello";
System.out.println("str1 = " + str1);
System.out.println(str1.compareTo("world"));
System.out.println(str1.compareTo("heihei"));
System.out.println(str1.compareTo("haha"));
System.out.println(str1.compareTo("hehe"));
System.out.println(str1.compareTo("helloworld")); //长度
System.out.println(str1.compareToIgnoreCase("HELLO"));
}
}
package com.lagou.task12;
/**
* @author 西风月
* @date 2020/7/26
* @description
*/
public class StringManyMethodTest {
public static void main(String[] args) {
String str1 = new String(" Let Me Give You Some Color To See See!");
System.out.println("str1 = " + str1);
System.out.println(str1.contains("some"));
System.out.println(str1.contains("Some"));
System.out.println(str1.toUpperCase());
System.out.println(str1.toLowerCase());
System.out.println(str1.trim());
System.out.println("str1 = " + str1);
System.out.println(str1.startsWith("Let"));
System.out.println(str1.trim().startsWith("Let"));
System.out.println(str1.endsWith("SEE!"));
System.out.println(str1.toUpperCase().endsWith("SEE!"));
System.out.println(str1.startsWith( "Let", 5));
}
}
package com.lagou.task12;
import java.util.Scanner;
public class StringEqualsTest {
public static void main(String[] args) {
int i = 0;
Scanner sc = new Scanner(System.in);
while(true) {
System.out.println("请输入用户名和密码信息:");
String name = sc.next();
String pwd = sc.next();
//if (name.equals("admin") && pwd.equals("123456")) {
if("admin".equals(name) && "123456".equals(pwd)) { //相比于上面方式,采用这种方式可以防止空指针的异常
System.out.println("登录成功!");
break;
}
if(i++ == 2) {
System.out.println("账户已冻结,请联系客服人员!");
break;
}
System.out.println("用户名或密码输入有误,请重试!您还有" + (3-i) + "次机会!");
}
}
}
package com.lagou.task12;
/**
* @author 西风月
* @date 2020/7/27
* @description
*/
public class StringIndexTest {
public static void main(String[] args) {
String str1 = new String("Good Good Study, Day Day Up!");
System.out.println(str1);
System.out.println("pos = " + str1.indexOf('g')); //查找不存在,返回-1
System.out.println("pos = " + str1.indexOf('G'));
System.out.println("pos = " + str1.indexOf('G', 1));
System.out.println("=============================================");
System.out.println(str1.indexOf("day"));
System.out.println(str1.indexOf("Day"));
System.out.println(str1.indexOf("Day", 17+3));
System.out.println("==============================================");
int pos = 0;
while((pos = str1.indexOf("Day", pos)) != -1) {
System.out.println(pos);
pos += "Day".length();
}
System.out.println("==============================================");
System.out.println(str1.lastIndexOf('G'));
System.out.println(str1.lastIndexOf('G', 4));
System.out.println("=============================================");
System.out.println(str1.lastIndexOf("Day"));
System.out.println(str1.lastIndexOf("Day", 20));
System.out.println(str1.lastIndexOf("Day", 10));
}
}
package com.lagou.task12;
import java.util.Scanner;
/**
* @author 西风月
* @date 2020/7/27
* @description
*/
public class SubStringTest {
public static void main(String[] args) {
String str1 = "Happy Wife, Happy Life!";
System.out.println("str1 = " + str1);
System.out.println(str1.substring(0,10));
System.out.println(str1.substring(11, str1.length()));
System.out.println("请输入一个字符串:");
Scanner sc = new Scanner(System.in);
String str3 = sc.nextLine();
System.out.println("请输入一个字符:");
String str4 = sc.next();
System.out.println(str2.substring(str3.indexOf(str4)+str4.length()));
}
}
1.5 正则表达式
package com.lagou.task12;
import java.util.Scanner;
/**
* @author 西风月
* @date 2020/7/27
* @description
*/
public class StringRegTest {
public static void main(String[] args) {
//String reg1 = "\\d{6}";
//String reg1 = "[0-9]{6}";
//String reg1 = "[1-9]\\d{4,14}";
//String reg1 = "1[34578]\\d{9}";
String reg1 = "(\\d{6})(\\d{4})(\\d{2})(\\d{2})(\\d{3})([0-9|X])";
Scanner sc = new Scanner(System.in);
System.out.println(sc.next().matches(reg1));
}
}
- 正则表达式举例: ```java package com.lagou.task12;
import java.util.Arrays;
/**
- @author 西风月
- @date 2020/7/27
@description */ public class StringRegMethodTest { public static void main(String[] args) {
String str1 = "1001,zhangfei,30"; System.out.println("str1=" + str1); System.out.println(Arrays.toString(str1.split(","))); System.out.println(str1.replace(',', '-')); String str6 = "1234abc5678defg990hik"; System.out.println(str6.replaceFirst("\\d+", "&")); System.out.println(str6.replaceAll("[a-z]+", "===="));
} }
```
总结
- String 类(重点)
- 概念
- 常量池
- 常用的构造方法
- 常用的成员方法
- 正则表达式的概念与使用、相关的方法等
- String 类(重点)