Activity概述
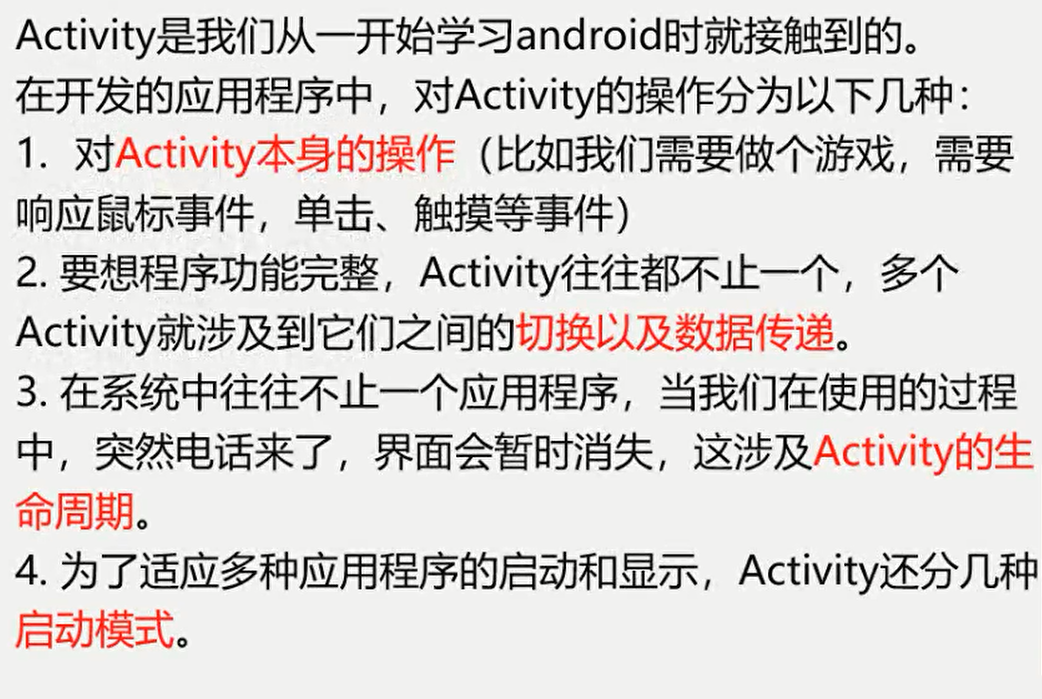
Activity的响应事件
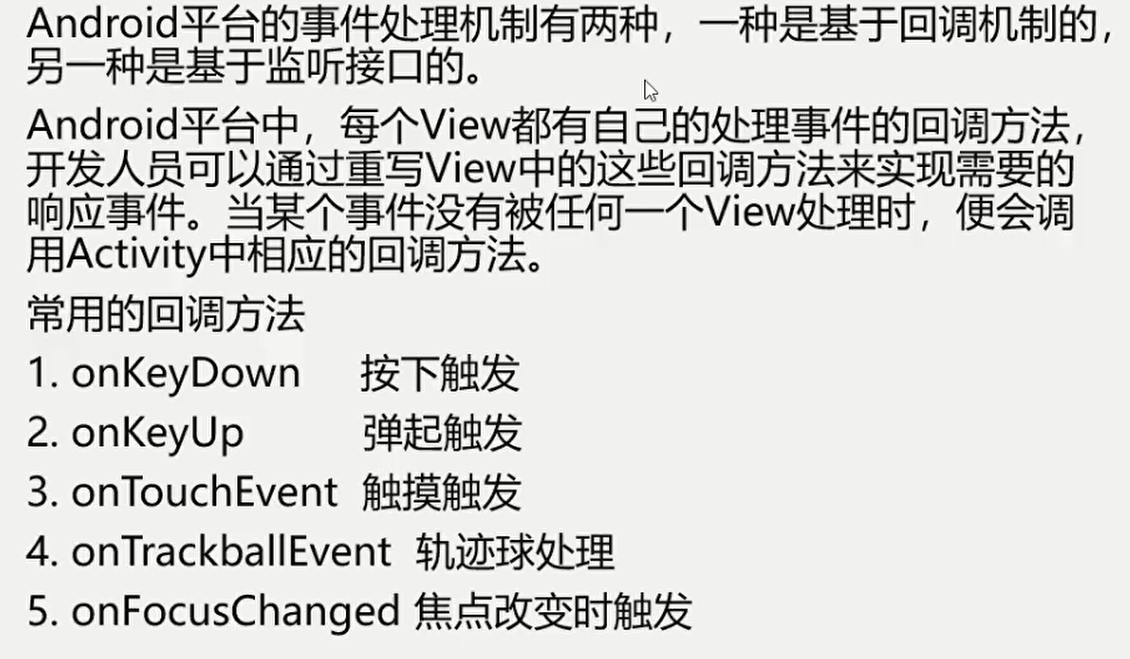
Activity之间的数据传递
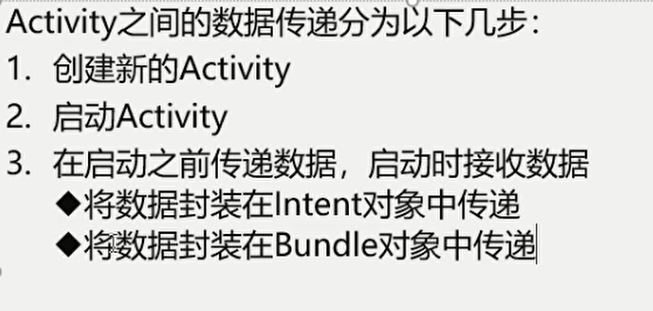
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<TextView
android:id="@+id/tv1"
android:textSize="20sp"
android:text="第一个activity"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btn1"
android:text="启动第二个activity"
android:onClick="btnClick1"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btn2"
android:text="启动第二个activity-传数据1"
android:onClick="btnClick2"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btn3"
android:text="启动第二个activity-传数据2"
android:onClick="btnClick3"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btn4"
android:text="启动第二个activity-传回数据1"
android:onClick="btnClick4"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
activity_main2.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".Main2Activity">
<TextView
android:id="@+id/tv1"
android:textSize="20sp"
android:text="第二个activity"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<TextView
android:id="@+id/tv2"
android:textSize="20sp"
android:text="传递的数据:"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/btn1"
android:textSize="20sp"
android:text="返回"
android:onClick="btnRet"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
MainActivity.java
package com.bluelesson.activitydemo;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.util.Log;
import android.view.KeyEvent;
import android.view.MotionEvent;
import android.view.View;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@Override
public boolean onKeyUp(int keyCode, KeyEvent event) {
Log.d("15pb","onKeyUp");
return super.onKeyUp(keyCode, event);
}
@Override
public boolean onKeyDown(int keyCode, KeyEvent event) {
Log.d("15pb","onKeyDown");
return super.onKeyDown(keyCode, event);
}
@Override
public boolean onTouchEvent(MotionEvent event) {
Log.d("15pb","onTouchEvent");
return super.onTouchEvent(event);
}
public void btnClick1(View view) {
// 1. 创建Intent对象
Intent intent = new Intent();
// 2. 设置信息
intent.setClass(this,
Main2Activity.class
);
// 3. 启动activity
startActivity(intent);
}
public void btnClick2(View view) {
// 1. 创建Intent对象
Intent intent = new Intent();
// 2. 设置信息
// 2.1 设置类类型
intent.setClass(this,
Main2Activity.class
);
// 2.2 设置传递的数据
intent.putExtra("name","hello");
// 3. 启动activity
startActivity(intent);
}
public void btnClick3(View view) {
// 1. 创建Intent对象
Intent intent = new Intent();
// 2. 设置信息
// 2.1 设置类类型
intent.setClass(this,
Main2Activity.class
);
// 2.2 设置传递的数据
Bundle bundle = new Bundle();
bundle.putString("name","hello15pb");
intent.putExtras(bundle);
// 3. 启动activity
startActivity(intent);
}
public void btnClick4(View view) {
// 1. 创建Intent对象
Intent intent = new Intent();
// 2. 设置信息
intent.setClass(this,
Main2Activity.class
);
// 3. 启动activity
// 传入请求码
startActivityForResult(intent,0x111);
}
@Override
protected void onActivityResult(int requestCode,
int resultCode,
Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if(resultCode == 0x111){
String name = data.getStringExtra("name");
Toast.makeText(this,
"传回的数据:"+name,
Toast.LENGTH_SHORT).show();
}
}
}
Main2Activity.java
package com.bluelesson.activitydemo;
import android.content.Intent;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.TextView;
public class Main2Activity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
// 接收数据
Intent intent = getIntent();
String name = intent.getStringExtra("name");
if(name == null){
Bundle bundle = intent.getExtras();
if(bundle != null){
name = bundle.getString("name");
}
}
// 显示数据
TextView textView = findViewById(R.id.tv2);
textView.setText("传递的数据:"+name);
}
public void btnRet(View view) {
// 返回时,可以设置数据
// setResult(0x111);
Intent intent = new Intent();
intent.putExtra("name","hi hello");
setResult(0x111,intent);
finish();//关闭activity
}
}
Activity的intent隐式启动
隐式启动
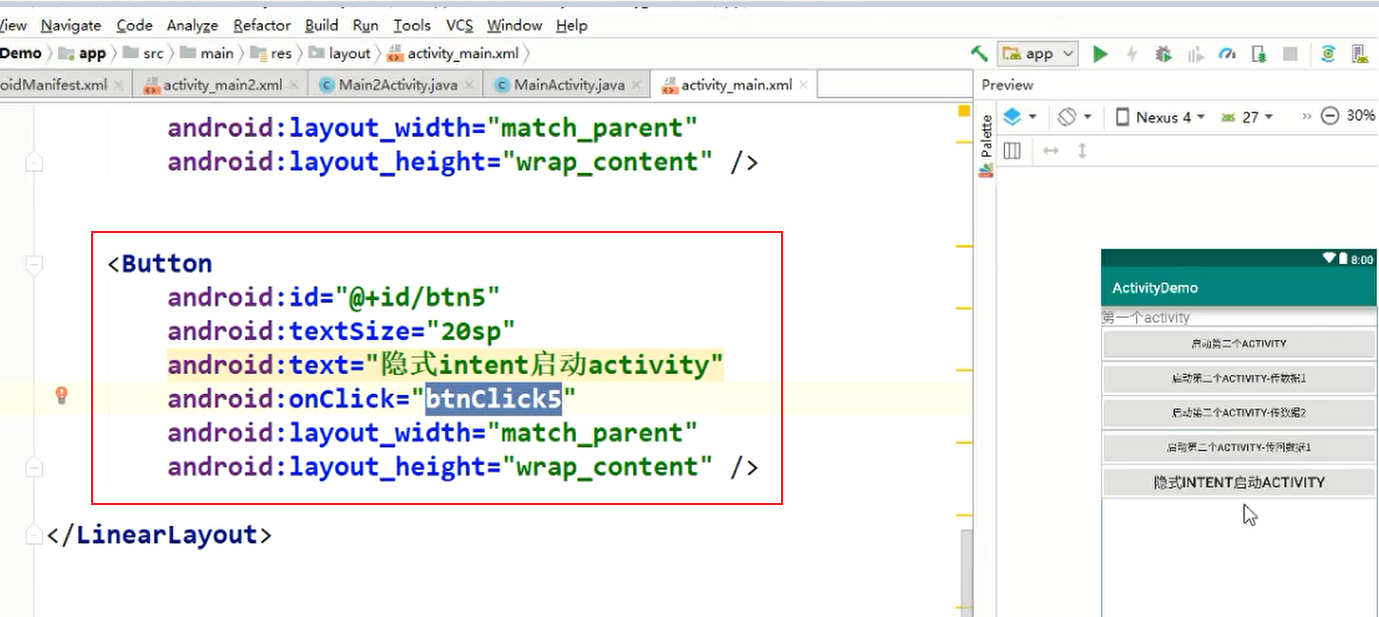
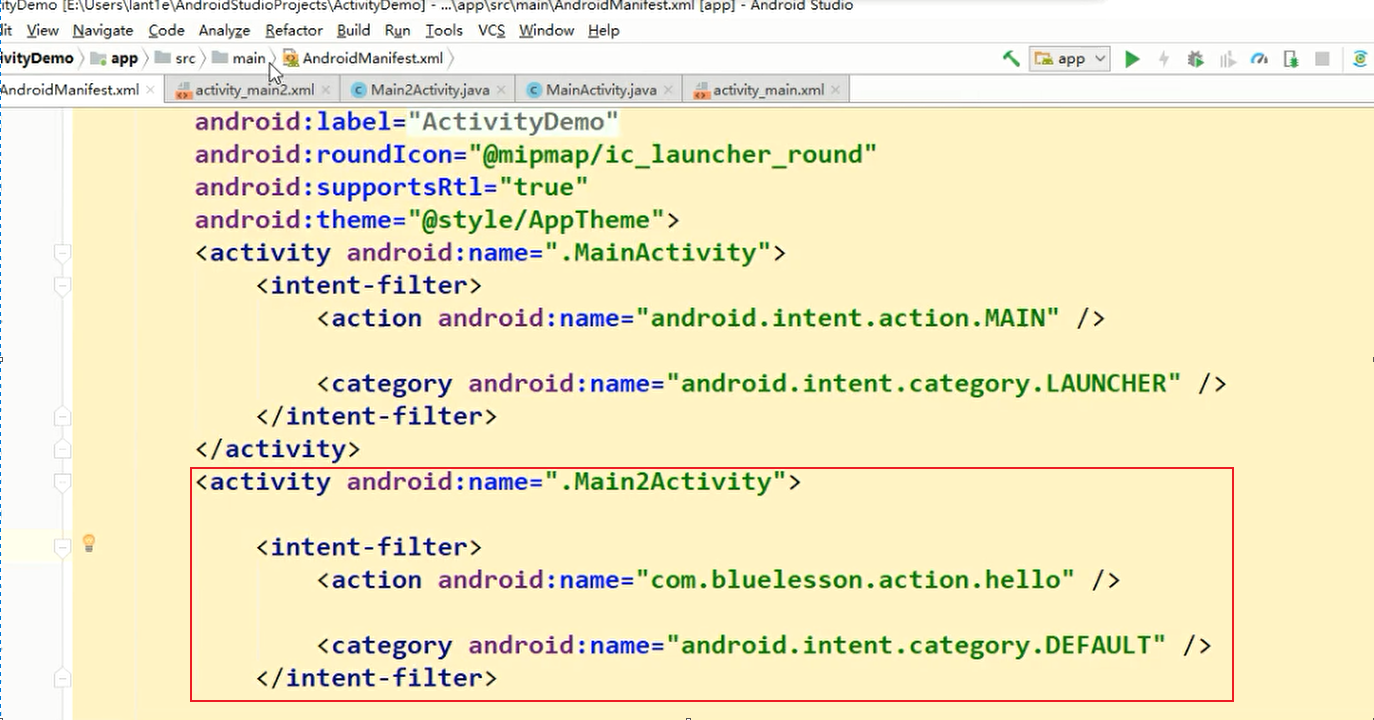
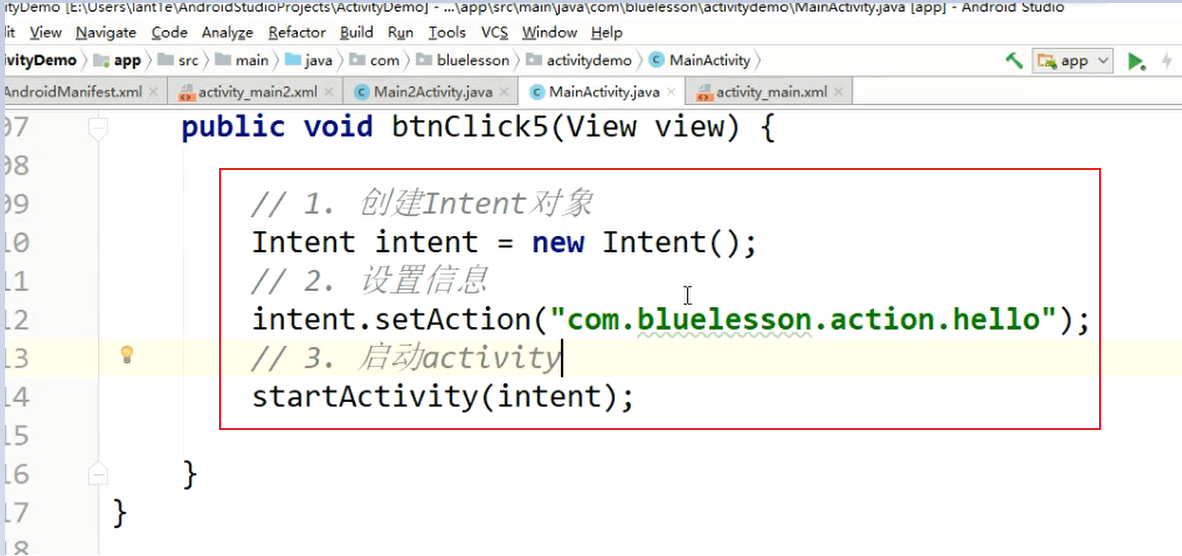
隐式启动设置data
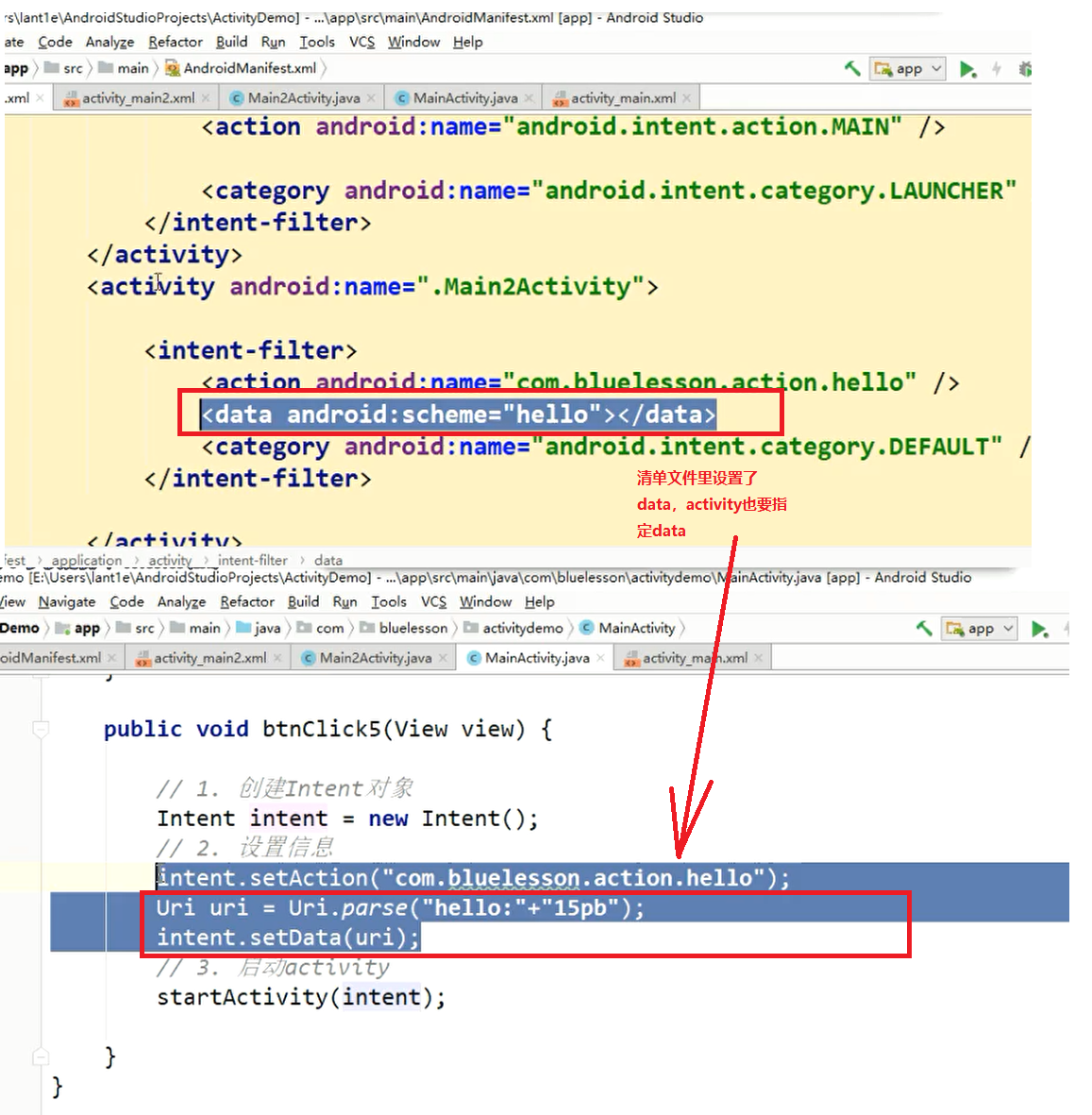