定义字符串
设置一个变量。 使用 单引号 或者 双引号 来定义字符串。
# 定义字符串
a = "helloworld"
b = '1000'
# 打印变量的类型 type()
print(type(a))
print(type(b))
执行,可以看到运行结果。
自己在编辑器中编写如下代码,执行,查看结果
# 定义字符串
a = "helloworld"
b = '1000'
# 打印变量的类型 type()
print(type(a))
print(type(b))
# 只要是在单引号 或者双引号中 都是字符串
c = "中文"
d = '中国'
e = '1234abc中'
print(c,type(c))
print(d,type(d))
print(e,type(e))
运行 可以看到结果
也可以使用 三个引号,定义多行的字符串。
# 定义多行字符串
content = """
发布主题
选择话题板块
输入内容
"""
print(content)
# 使用 三个单引号 来定义 可以进行换行。
c1 = '''发布主题
选择话题板块
输入内容'''
print(c1,type(c1))
字符串访问字符
🎈通过[数字]索引(下标)方式访问
h e l l o w o r l d
0 1 2 3 4 5 6 7 8 9
-10 -9 -8 -7 -6 -5 -4 -3 -2 -1
字符串中的每个字符都由下标(索引) 默认从0 开始, 0表示第一个字符。
比如
word = "helloworld"
# 第一
print(word[0])
# 第二
print(word[1])
# 最后一个 使用 -1 表示
print(word[-1])
# 倒数第二 使用 -2 表示
print(word[-2])
- 0 表示第一个
- 1 表示第二个
- -1 表示最后一个
- -2 表示倒数第二个
但是需要注意: 索引值不能超过字符串本身的长度。 比如helloworld 本身只有10位,如果超出范围,就会报错
# 超出范围,报错
print(word[10])
通过[m:n] 区间访问
通过索引的方式只能找到其中的1个字符。如果要访问多个字符。
word = "helloworld"
# 指定区间 (取头不取尾)包含第一个值 不包含第二个值。
print(word[0:3]) # hel # 0 表示第1个, 3表示第4个值。 包含第1个值,不包含第4个(取到第3个值)
#
print(word[1:3]) # el # 1表示第2个(包含) 3表示第4个(不包含) 取到第三个值
print(word[2:3]) # l # 2表示第3个(包含) 3表示第4个(不包含) 取到第三个值
# 也可以倒序
print(word[-3:-1]) # # -3表示倒数第3个(包含) -1表示倒数第1个(不包含) 取到倒数第二个值
# [:4] 第一个值不写,默认从第一个值开始取
print(word[:4]) # hell 前4位
# [-3:] 第二个值不写,取到最后一位
print(word[-3:]) # rld # 后3位
- [:n] 如果第一个值不写,默认就从第一个值开始取
- [m:] 如果第二个值不写,默认取到最后一位。
应用场景
比如给定字符串,获取 对应的版本号
请将python的版本号获取出来将(3.10.0 获取出来)。version = "Python 3.10.0"
version = "Python 3.10.0" print(version[7:]) # 3.10.0
通过[m:n:step] 取值
- m 表示 从 m位置开始取值(包含)
- n 表示 取到n位置(不包含)
- step 表示步长,默认值1
word = "hello world"
# 1 表示 从索引1开始(第2位,包含)
# 5 表示 到索引5结束(第6,不包含)
# 1 表示 从左向右 顺序依次取值
print(word[1:5:1]) # ello
# 2 表示 从左向右 顺序 中间隔1个值
print(word[1:5:2]) # el
# 前面2个值不写,取全部
# 2 从左向右 顺序 中间隔1个值
print(word[::2]) # hlowrd
# 默认 step值 正数,从左向右取值。 负数会反方向取值。
# 倒序打印 -1 表示反向
print(word[::-1]) # dlrow olleh
# -2 表示倒序 中间隔一个值
print(word[::-2]) # drwolh
这里取值都是有规律的,如果想要取无规律的值,只能一个一个写。
问: 如果我想无序,取其中的第一个字符,第三个字符,第八个字符这样的话应该怎么写
word[0]+word[2]+word[7]
使用的时候,比如 word[m:m:-1], 先看最后一个值。 -1
表示倒序
word[-6:-2:-1]
h e l l o w o r l d
0 1 2 3 4 5 6 7 8 9
-10 -9 -8 -7 -6 -5 -4 -3 -2 -1
对应的取值顺序
- -6 表示开始 向左取值
- 中间的-2 表示结束位置。 但是从 -6 开始取值向左 永远到不了 -2 这个位置
word = "hellowold"
print(word[-6:-1:-1])
字符串拼接
+ 拼接字符串
可以将两个或者多个字符串拼接为1 个字符串。
name = "xiaoming"
word = "hello"
# 将两个字符串拼接在一起
print(name+word)
运行结果
需要注意的是,如果 字符串拼接的时候 有数字,字符串不能直接跟数字进行拼接,需要先将数字转换为字符串,再进行拼接。
name = "xiaoming"
age = 20
直接拼接,会报错
name = "xiaoming"
age = 20
print(name+age)
* 数字n乘字符串 字符串重复n次
a = "hello"
print(a*10) # 字符串a重复10次
str() 转换为字符串
将数字转换为字符串。
name = "xiaoming"
word = "hello"
age = 20
# 将两个字符串拼接在一起
print(name+word)
print(name+str(age)) # str(age) 会先将 数字20 转换为字符串 "20"
🎈f 格式化输出
字符串中可以通过 f 格式化输出。比如上面的例子中 ,20 为数字,如果要跟字符串拼接,需要先将20转换为str 才可以。
在字符串前添加 f, 字符串中 {变量名的方式} 来获取对应的变量值。
name = "xiaoming"
word = "hello"
age = 20
# 将两个字符串拼接在一起
print(name+word)
print(name+str(age)) # str(age) 会先将 数字20 转换为字符串 "20"
print(f"我的姓名:{name},我的年龄:{age}")
执行结果
xiaominghello
xiaoming20
我的姓名:xiaoming,我的年龄:20
通过这种方式 数字也可以直接放里面。 后面的代码中 引用变量 我会使用这种方式。
转义字符
\t | tab 键缩进 |
---|---|
\n | 换行 |
在字符串中 添加对应的效果
names = "xiaoming\txiaowang\txiaohong\nzhangsan\tlisi"
print(names)
🎈r字符串
上面我们知道字符串中 \ 有特殊的含义。 但是在Windows的路径中。
file = "C:\Users\zengy\tables\name.csv"
本来 "C:\Users\zengy\tables\name.csv"
一个csv文件的路径。 但是里面有 \n \t 执行的时候,路径就会被转义。
所以执行的时候会报错。
解决Windows路径问题
有两种解决办法
使用
\\
file1 = "C:\\Users\\zengy\\tables\\name.csv" print(file1)
解决办法 字符串前添加 r, 字符串中的 \ 就失去了转义的作用。 ```python file2 = r”C:\Users\zengy\tables\name.csv” print(file2)
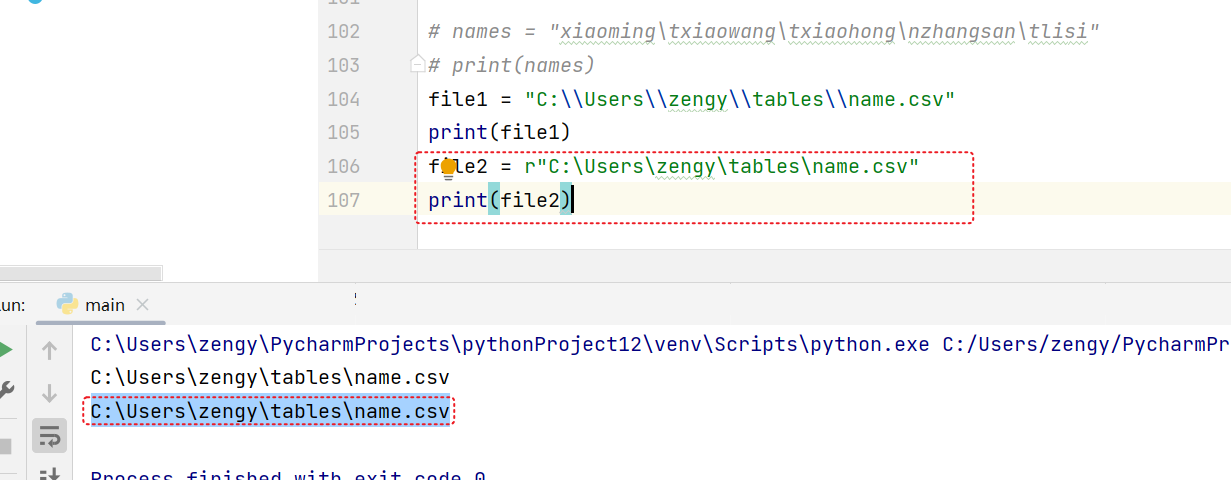
<a name="n1kvb"></a>
# 面试问题
Windows中一个文件的绝对路径用字符串来表示,怎么表示?<br />因为Windows系统的绝对文件路径中有 `\` 在python中有特殊的含义,所以要将特殊的含义去掉。<br />有两种解决办法:
1. 使用 双反斜杠的方式 `\\`
1. 字符串前添加 `r`
<a name="EyVt3"></a>
# 作业
```python
varsion = 'java version "1.8.0_331"'
获取java的版本 注意: 不要双引号。