https://blog.csdn.net/d18865556765/article/details/52156330?utm_medium=distribute.pc_relevant_download.none-task-blog-baidujs-2.nonecase&depth_1-utm_source=distribute.pc_relevant_download.none-task-blog-baidujs-2.nonecase
package myCalendar;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
import javax.swing.JComboBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JButton;
public class Lunar {
private int year;
private int month;
private int day;
private boolean leap;
final static String chineseNumber[] = {"一", "二", "三", "四", "五", "六", "七", "八", "九", "十", "十一", "十二"};
static SimpleDateFormat chineseDateFormat = new SimpleDateFormat("yyyy年MM月dd日");
final static long[] lunarInfo = new long[]
{0x04bd8, 0x04ae0, 0x0a570, 0x054d5, 0x0d260, 0x0d950, 0x16554, 0x056a0, 0x09ad0, 0x055d2,
0x04ae0, 0x0a5b6, 0x0a4d0, 0x0d250, 0x1d255, 0x0b540, 0x0d6a0, 0x0ada2, 0x095b0, 0x14977,
0x04970, 0x0a4b0, 0x0b4b5, 0x06a50, 0x06d40, 0x1ab54, 0x02b60, 0x09570, 0x052f2, 0x04970,
0x06566, 0x0d4a0, 0x0ea50, 0x06e95, 0x05ad0, 0x02b60, 0x186e3, 0x092e0, 0x1c8d7, 0x0c950,
0x0d4a0, 0x1d8a6, 0x0b550, 0x056a0, 0x1a5b4, 0x025d0, 0x092d0, 0x0d2b2, 0x0a950, 0x0b557,
0x06ca0, 0x0b550, 0x15355, 0x04da0, 0x0a5d0, 0x14573, 0x052d0, 0x0a9a8, 0x0e950, 0x06aa0,
0x0aea6, 0x0ab50, 0x04b60, 0x0aae4, 0x0a570, 0x05260, 0x0f263, 0x0d950, 0x05b57, 0x056a0,
0x096d0, 0x04dd5, 0x04ad0, 0x0a4d0, 0x0d4d4, 0x0d250, 0x0d558, 0x0b540, 0x0b5a0, 0x195a6,
0x095b0, 0x049b0, 0x0a974, 0x0a4b0, 0x0b27a, 0x06a50, 0x06d40, 0x0af46, 0x0ab60, 0x09570,
0x04af5, 0x04970, 0x064b0, 0x074a3, 0x0ea50, 0x06b58, 0x055c0, 0x0ab60, 0x096d5, 0x092e0,
0x0c960, 0x0d954, 0x0d4a0, 0x0da50, 0x07552, 0x056a0, 0x0abb7, 0x025d0, 0x092d0, 0x0cab5,
0x0a950, 0x0b4a0, 0x0baa4, 0x0ad50, 0x055d9, 0x04ba0, 0x0a5b0, 0x15176, 0x052b0, 0x0a930,
0x07954, 0x06aa0, 0x0ad50, 0x05b52, 0x04b60, 0x0a6e6, 0x0a4e0, 0x0d260, 0x0ea65, 0x0d530,
0x05aa0, 0x076a3, 0x096d0, 0x04bd7, 0x04ad0, 0x0a4d0, 0x1d0b6, 0x0d250, 0x0d520, 0x0dd45,
0x0b5a0, 0x056d0, 0x055b2, 0x049b0, 0x0a577, 0x0a4b0, 0x0aa50, 0x1b255, 0x06d20, 0x0ada0};
//====== 传回农历 y年的总天数
final private static int yearDays(int y) {
int i, sum = 348;
for (i = 0x8000; i > 0x8; i >>= 1) {
if ((lunarInfo[y - 1900] & i) != 0) sum += 1;
}
return (sum + leapDays(y));
}
//====== 传回农历 y年闰月的天数
final private static int leapDays(int y) {
if (leapMonth(y) != 0) {
if ((lunarInfo[y - 1900] & 0x10000) != 0)
return 30;
else
return 29;
} else
return 0;
}
//====== 传回农历 y年闰哪个月 1-12 , 没闰传回 0
final private static int leapMonth(int y) {
return (int) (lunarInfo[y - 1900] & 0xf);
}
//====== 传回农历 y年m月的总天数
final private static int monthDays(int y, int m) {
if ((lunarInfo[y - 1900] & (0x10000 >> m)) == 0)
return 29;
else
return 30;
}
//====== 传回农历 y年的生肖
final public String animalsYear() {
final String[] Animals = new String[]{"鼠", "牛", "虎", "兔", "龙", "蛇", "马", "羊", "猴", "鸡", "狗", "猪"};
return Animals[(year - 4) % 12];
}
//====== 传入 月日的offset 传回干支, 0=甲子
final private static String cyclicalm(int num) {
final String[] Gan = new String[]{"甲", "乙", "丙", "丁", "戊", "己", "庚", "辛", "壬", "癸"};
final String[] Zhi = new String[]{"子", "丑", "寅", "卯", "辰", "巳", "午", "未", "申", "酉", "戌", "亥"};
return (Gan[num % 10] + Zhi[num % 12]);
}
//====== 传入 offset 传回干支, 0=甲子
final public String cyclical() {
int num = year - 1900 + 36;
return (cyclicalm(num));
}
public static String getChinaDayString(int day) {
String chineseTen[] = {"初", "十", "廿", "卅"};
int n = day % 10 == 0 ? 9 : day % 10 - 1;
if (day > 30)
return "";
if (day == 10)
return "初十";
else
return chineseTen[day / 10] + chineseNumber[n];
}
/** */
/**
* 传出y年m月d日对应的农历.
* yearCyl3:农历年与1864的相差数 ?
* monCyl4:从1900年1月31日以来,闰月数
* dayCyl5:与1900年1月31日相差的天数,再加40 ?
*
* @param
* @return
*/
public String getLunarDate(int year_log, int month_log, int day_log) {
//@SuppressWarnings("unused")
int yearCyl, monCyl, dayCyl;
int leapMonth = 0;
String nowadays;
Date baseDate = null;
Date nowaday = null;
try {
baseDate = chineseDateFormat.parse("1900年1月31日");
} catch (ParseException e) {
e.printStackTrace(); //To change body of catch statement use Options | File Templates.
}
nowadays = year_log + "年" + month_log + "月" + day_log + "日";
try {
nowaday = chineseDateFormat.parse(nowadays);
} catch (ParseException e) {
e.printStackTrace(); //To change body of catch statement use Options | File Templates.
}
//求出和1900年1月31日相差的天数
int offset = (int) ((nowaday.getTime() - baseDate.getTime()) / 86400000L);
dayCyl = offset + 40;
monCyl = 14;
//用offset减去每农历年的天数
// 计算当天是农历第几天
//i最终结果是农历的年份
//offset是当年的第几天
int iYear, daysOfYear = 0;
for (iYear = 1900; iYear < 10000 && offset > 0; iYear++) {
daysOfYear = yearDays(iYear);
offset -= daysOfYear;
monCyl += 12;
}
if (offset < 0) {
offset += daysOfYear;
iYear--;
monCyl -= 12;
}
//农历年份
year = iYear;
yearCyl = iYear - 1864;
leapMonth = leapMonth(iYear); //闰哪个月,1-12
leap = false;
//用当年的天数offset,逐个减去每月(农历)的天数,求出当天是本月的第几天
int iMonth, daysOfMonth = 0;
for (iMonth = 1; iMonth < 13 && offset > 0; iMonth++) {
//闰月
if (leapMonth > 0 && iMonth == (leapMonth + 1) && ! leap) {
-- iMonth;
leap = true;
daysOfMonth = leapDays(year);
} else
daysOfMonth = monthDays(year, iMonth);
offset -= daysOfMonth;
//解除闰月
if (leap && iMonth == (leapMonth + 1)) leap = false;
if (! leap) monCyl++;
}
//offset为0时,并且刚才计算的月份是闰月,要校正
if (offset == 0 && leapMonth > 0 && iMonth == leapMonth + 1) {
if (leap) {
leap = false;
} else {
leap = true;
-- iMonth;
-- monCyl;
}
}
//offset小于0时,也要校正
if (offset < 0) {
offset += daysOfMonth;
-- iMonth;
-- monCyl;
}
month = iMonth;
day = offset + 1;
if (((month) == 1) && day == 1) {
return "春节";
} else if (((month) == 1) && day == 15) {
return "元宵";
} else if (((month) == 5) && day == 5)
return "端午";
else if (((month) == 8) && day == 15)
return "中秋";
else if (day == 1)
return chineseNumber[month - 1] + "月";
else
return getChinaDayString(day);
}
public String toString() {
if (chineseNumber[month - 1] == "一" && getChinaDayString(day) == "初一")
return "农历" + year + "年";
else if (getChinaDayString(day) == "初一")
return chineseNumber[month - 1] + "月";
else
return getChinaDayString(day);
//return year + "年" + (leap ? "闰" : "") + chineseNumber[month - 1] + "月" + getChinaDayString(day);
}
}
class Clock extends Canvas implements Runnable {
/**
*
*/
private static final long serialVersionUID = 3660124045489727166L;
MainFrame mf;
Thread t;
String time;
public Clock(MainFrame mf) {
this.mf = mf;
setSize(280, 40);
setBackground(Color.white);
t = new Thread(this); //实例化线程
t.start(); //调用线程
}
public void run() {
while (true) {
try {
Thread.sleep(1000); //休眠1秒钟
} catch (InterruptedException e) {
System.out.println("异常");
}
this.repaint(100);
}
}
public void paint(Graphics g) {
Font f = new Font("宋体", Font.BOLD, 16);
SimpleDateFormat SDF = new SimpleDateFormat("yyyy'年'MM'月'dd'日'HH:mm:ss");//格式化时间显示类型
Calendar now = Calendar.getInstance();
time = SDF.format(now.getTime()); //得到当前日期和时间
g.setFont(f);
g.setColor(Color.black);
g.drawString(time, 45, 25);
}
}
class MainFrame extends JFrame {
/**
*
*/
private static final long serialVersionUID = 1L;
JPanel panel = new JPanel(new BorderLayout());
JPanel panel1 = new JPanel();
JPanel panel2 = new JPanel(new GridLayout(7, 7));
JPanel panel3 = new JPanel();
JLabel[] label = new JLabel[49];
JLabel y_label = new JLabel("年份");
JLabel m_label = new JLabel("月份");
JComboBox com1 = new JComboBox();
JComboBox com2 = new JComboBox();
JButton but1 = new JButton("上个月");
JButton but2 = new JButton("下个月");
int re_year, re_month;
int x_size, y_size;
String year_num;
Calendar now = Calendar.getInstance(); // 实例化Calendar
MainFrame() {
super("万年历");
setSize(600, 700);
x_size = (int) (Toolkit.getDefaultToolkit().getScreenSize().getWidth());
y_size = (int) (Toolkit.getDefaultToolkit().getScreenSize().getHeight());
setLocation((x_size - 300) / 2, (y_size - 350) / 2);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
panel1.add(but1);
panel1.add(y_label);
panel1.add(com1);
panel1.add(m_label);
panel1.add(com2);
panel1.add(but2);
for (int i = 0; i < 49; i++) {
label[i] = new JLabel("", JLabel.CENTER);// 将显示的字符设置为居中
panel2.add(label[i]);
}
panel3.add(new Clock(this));
panel.add(panel1, BorderLayout.NORTH);
panel.add(panel2, BorderLayout.CENTER);
panel.add(panel3, BorderLayout.SOUTH);
panel.setBackground(Color.white);
panel1.setBackground(Color.white);
panel2.setBackground(Color.white);
panel3.setBackground(Color.gray);
Init();
but1.addActionListener(new AnAction());
but2.addActionListener(new AnAction());
com1.addActionListener(new ClockAction());
com2.addActionListener(new ClockAction());
setContentPane(panel);
setVisible(true);
setResizable(false);
}
class AnAction implements ActionListener {
public void actionPerformed(ActionEvent e) {
int c_year, c_month, c_week;
c_month = Integer.parseInt(com2.getSelectedItem().toString()) - 1; // 得到当前月份,并减1,计算机中的月为0-11
c_year = Integer.parseInt(com1.getSelectedItem().toString()) - 1; // 得到当前所选年份
if (e.getSource() == but1) {
if (c_month == 0) {
c_year = c_year - 1;
c_month = 11;
} else
c_month = c_month - 1;
}
if (e.getSource() == but2) {
if (c_month == 11) {
c_year = c_year + 1;
c_month = 0;
} else
c_month = c_month + 1;
}
com1.setSelectedIndex(c_year);
com2.setSelectedIndex(c_month);
c_year = Integer.parseInt(com1.getSelectedItem().toString()); // 得到当前所选年份
c_month = Integer.parseInt(com2.getSelectedItem().toString()) - 1; // 得到当前月份,并减1,计算机中的月为0-11
c_week = use(c_year, c_month); // 调用函数use,得到星期几
Resetday(c_week, c_year, c_month); // 调用函数Resetday
}
}
/* class NextAction implements ActionListener
{
public void actionPerformed(ActionEvent arg0)
{
int c_year, c_month,c_week;
c_month = Integer.parseInt(com2.getSelectedItem().toString()) - 1; // 得到当前月份,并减1,计算机中的月为0-11
c_year = Integer.parseInt(com1.getSelectedItem().toString()); // 得到当前所选年份
if(c_month==11)
{
c_year=c_year+1;
c_month=0;
}
c_week = use(c_year, c_month); // 调用函数use,得到星期几
Resetday(c_week, c_year, c_month); // 调用函数Resetday
}
}
*/
class ClockAction implements ActionListener {
public void actionPerformed(ActionEvent arg0) {
int c_year, c_month, c_week;
c_year = Integer.parseInt(com1.getSelectedItem().toString()); // 得到当前所选年份
c_month = Integer.parseInt(com2.getSelectedItem().toString()) - 1; // 得到当前月份,并减1,计算机中的月为0-11
c_week = use(c_year, c_month); // 调用函数use,得到星期几
Resetday(c_week, c_year, c_month); // 调用函数Resetday
}
}
public void Init() {
int year, month_num, first_day_num;
String log[] = {"日", "一", "二", "三", "四", "五", "六"};
for (int i = 0; i < 7; i++) {
label[i].setText(log[i]);
}
for (int i = 0; i < 49; i = i + 7) {
label[i].setForeground(Color.red); // 将星期日的日期设置为红色
}
for (int i = 6; i < 49; i = i + 7) {
label[i].setForeground(Color.blue);// 将星期六的日期设置为hong色
}
for (int i = 1; i < 10000; i++) {
com1.addItem("" + i);
}
for (int i = 1; i < 13; i++) {
com2.addItem("" + i);
}
month_num = (int) (now.get(Calendar.MONTH)); // 得到当前时间的月份
year = (int) (now.get(Calendar.YEAR)); // 得到当前时间的年份
com1.setSelectedIndex(year - 1); // 设置下拉列表显示为当前年???????????
com2.setSelectedIndex(month_num); // 设置下拉列表显示为当前月
first_day_num = use(year, month_num);
Resetday(first_day_num, year, month_num);
}
public int use(int reyear, int remonth) {
int week_num;
now.set(reyear, remonth, 1); // 设置时间为所要查询的年月的第一天
week_num = (int) (now.get(Calendar.DAY_OF_WEEK));// 得到第一天的星期
return week_num;
}
// @SuppressWarnings("deprecation")
public void Resetday(int week_log, int year_log, int month_log) {
String[][] riLI = new String[49][49];
String log[] = {"日", "一", "二", "三", "四", "五", "六"};
for (int i = 0; i < 7; i++) {
riLI[0][i] = (log[i]);
}
int month_day_score; // 存储月份的天数
int count;
Lunar lunar;
int month_day;
String[] LunarDate = new String[49];
month_day_score = 0;
count = 1;
for (int i = 1; i < 49; i++) {
for (int j = 0; j < 49; j = j + 7) {
if (i != j && i != j + 6)
label[i].setForeground(Color.black);
}
}
Date date = new Date(year_log, month_log + 1, 1); // now MONTH是从0开始的, 对于一月第几天来说,DAY_OF_MONTH第一天就是1. 对于一年第几个月来说,MONTH一月份是0,二月份是1...
Calendar cal = Calendar.getInstance();
cal.setTime(date);
cal.add(Calendar.MONTH, - 1); // 前个月
month_day_score = cal.getActualMaximum(Calendar.DAY_OF_MONTH);// 最后一天
month_day = month_day_score;
for (int i = 7; i < 49; i++) { // 初始化标签
label[i].setText("");
}
week_log = week_log + 6; // 将星期数加6,使显示正确
month_day_score = month_day_score + week_log;
lunar = new Lunar();
for (int i = 0; i < month_day; i++) {
LunarDate[i] = lunar.getLunarDate(year_log, month_log + 1, i + 1);
}
for (int i = week_log; i < month_day_score; i++, count++) {
if (month_log == 9 && count == 1) {
label[i].setText(count + "国庆");
label[i].setForeground(Color.red);
riLI[0][i] = String.valueOf(count);
riLI[1][i] = "国庆";
} else if (month_log == 0 && count == 1) {
label[i].setText(count + "元旦");
label[i].setForeground(Color.red);
riLI[0][i] = String.valueOf(count);
riLI[1][i] = "元旦";
} else if (month_log == 11 && count == 24) {
label[i].setText(count + "平安夜");
label[i].setForeground(Color.red);
riLI[0][i] = String.valueOf(count);
riLI[1][i] = "平安夜";
} else if (month_log == 11 && count == 25) {
label[i].setText(count + "圣诞");
label[i].setForeground(Color.red);
riLI[0][i] = String.valueOf(count);
riLI[1][i] = "圣诞";
} else if (month_log == 1 && count == 14) {
label[i].setText(count + "情人节");
label[i].setForeground(Color.red);
riLI[0][i] = String.valueOf(count);
riLI[1][i] = "情人节";
} else if (month_log == 4 && count == 1) {
label[i].setText(count + "劳动节");
label[i].setForeground(Color.red);
riLI[0][i] = String.valueOf(count);
riLI[1][i] = "劳动节";
} else if (LunarDate[i - week_log].equals("春节") || LunarDate[i - week_log].equals("元宵") || LunarDate[i - week_log].equals("端午") || LunarDate[i - week_log].equals("中秋")) {
label[i].setText(count + LunarDate[i - week_log]);
label[i].setForeground(Color.red);
riLI[0][i] = String.valueOf(count);
riLI[1][i] = LunarDate[i - week_log];
} else {
label[i].setText(count + LunarDate[i - week_log]);
riLI[0][i] = String.valueOf(count);
riLI[1][i] = LunarDate[i - week_log];
}
}
System.out.println("=============================================");
System.out.println(year_log + "年" + (month_log + 1) + "月");//输出年月
for (int i = 0; i < 7; i++) {//输出星期
System.out.print(riLI[0][i] + "\t");
}
System.out.println();
for (int i = 1; i < 6; i++) {
for (int j = 7 * i; j < 7 * (i + 1); j++) {
if (null != riLI[0][j]) {//输出公历
System.out.print(riLI[0][j] + "\t");
} else {
System.out.print(" \t");
}
}
System.out.println();
for (int j = 7 * i; j < 7 * (i + 1); j++) {
if (null != riLI[1][j]) {//输出农历
System.out.print(riLI[1][j] + "\t");
} else {
System.out.print(" \t");
}
}
System.out.println();
}
}
public static void main(String[] args) {
JFrame.setDefaultLookAndFeelDecorated(true);
new MainFrame();
}
}
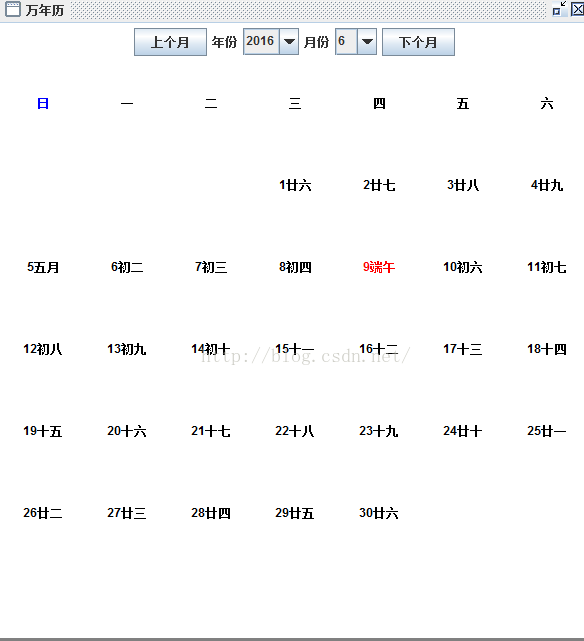