package com.zsy.gulimall.product.entity;
import com.baomidou.mybatisplus.annotation.*;
import java.io.Serializable;
import java.util.List;
import com.fasterxml.jackson.annotation.JsonInclude;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import lombok.Data;
import lombok.EqualsAndHashCode;
/**
* <p>
* 商品三级分类
* </p>
*
* @author liulq
* @since 2022-03-19
*/
@Data
@TableName("pms_category")
@EqualsAndHashCode(callSuper = false)
@ApiModel(value="PmsCategory对象", description="商品三级分类")
public class PmsCategory implements Serializable {
private static final long serialVersionUID = 1L;
@ApiModelProperty(value = "分类id")
@TableId(value = "cat_id", type = IdType.AUTO)
private Long catId;
@ApiModelProperty(value = "分类名称")
private String name;
@ApiModelProperty(value = "父分类id")
private Long parentCid;
@ApiModelProperty(value = "层级")
private Integer catLevel;
@ApiModelProperty(value = "是否显示[0-不显示,1显示]")
@TableLogic(value = "1", delval = "0")
private Integer showStatus;
@ApiModelProperty(value = "排序")
private Integer sort;
@ApiModelProperty(value = "图标地址")
private String icon;
@ApiModelProperty(value = "计量单位")
private String productUnit;
@ApiModelProperty(value = "商品数量")
private Integer productCount;
@JsonInclude(JsonInclude.Include.NON_EMPTY)
@TableField(exist = false)
private List<PmsCategory> children;
}
实现
public List<CategoryEntity> listWithTree() {
//1、查出所有分类
List<CategoryEntity> entities = baseMapper.selectList(null);
//2、组装成父子的树形结构
//2.1)、找到所有的一级分类,给children设置子分类
return entities.stream()
// 过滤找出一级分类
.filter(categoryEntity -> categoryEntity.getParentCid() == 0)
// 处理,给一级菜单递归设置子菜单
.peek(menu -> menu.setChildren(getChildless(menu, entities)))
// 按sort属性排序
.sorted(Comparator.comparingInt(menu -> (menu.getSort() == null ? 0 : menu.getSort())))
.collect(Collectors.toList());
}
/**
* 递归查找所有菜单的子菜单
*/
private List<CategoryEntity> getChildless(CategoryEntity root, List<CategoryEntity> all) {
return all.stream()
.filter(categoryEntity -> categoryEntity.getParentCid().equals(root.getCatId()))
.peek(categoryEntity -> {
// 找到子菜单
categoryEntity.setChildren(getChildless(categoryEntity, all));
})
.sorted(Comparator.comparingInt(menu -> (menu.getSort() == null ? 0 : menu.getSort())))
.collect(Collectors.toList());
}
效果
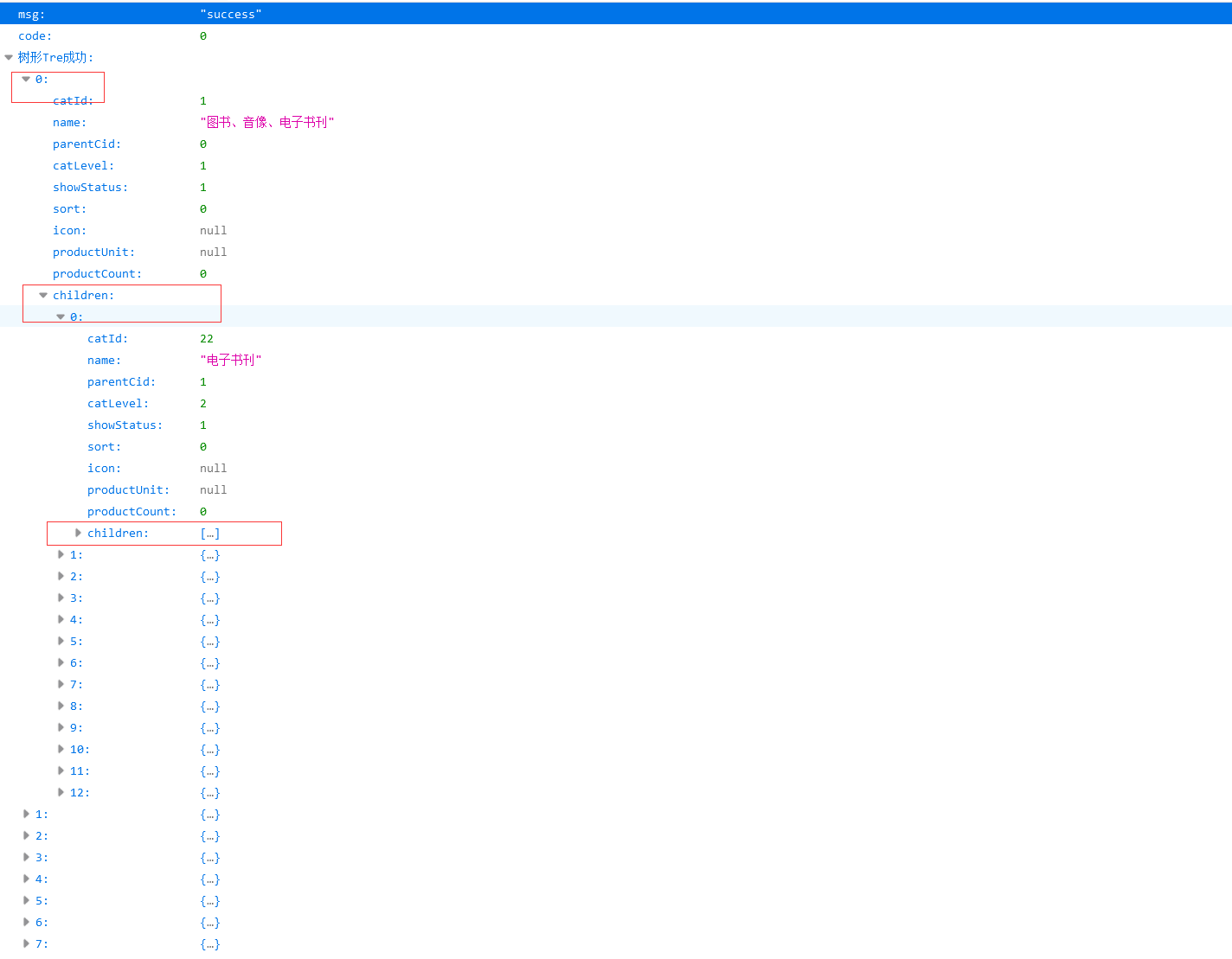