导入代码库
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
% matplotlib inline
import warnings
warnings.filterwarnings('ignore')
# 不发出警告
from bokeh.io import output_notebook
output_notebook()
# 导入notebook绘图模块
from bokeh.plotting import figure,show
from bokeh.models import ColumnDataSource
# 导入图表绘制、图标展示模块
# 导入ColumnDataSource模块
工具栏的位置
# 工具栏 tools
# 设置位置
p1 = figure(plot_width = 300, plot_height = 300,
toolbar_location = 'above') # 工具栏位置:"above","below","left","right"
p1.circle(np.random.randn(100),np.random.randn(100))
p2 = figure(plot_width=300, plot_height=300,
toolbar_location="below",
toolbar_sticky=False)
# 工具栏位置设置为"below"时,可增加toolbar_sticky参数使得toolsbar不被遮挡
p2.circle(np.random.randn(100),np.random.randn(100))
show(p1)
show(p2)
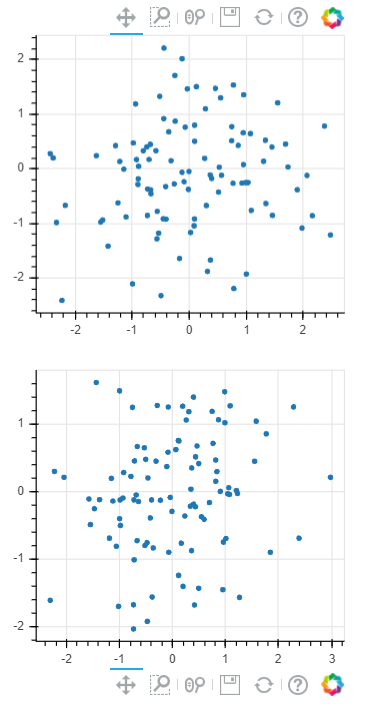
移动、放大缩小、存储、刷新
# 工具栏 tools
# 移动、放大缩小、存储、刷新
TOOLS = '''
pan, xpan, ypan,
box_zoom,
wheel_zoom, xwheel_zoom, ywheel_zoom,
zoom_in, xzoom_in, yzoom_in,
zoom_out, xzoom_out, yzoom_out,
save,reset
'''
p = figure(plot_width=800, plot_height=400,toolbar_location="above",
tools = TOOLS)
# 添加toolbar
# 这里tools = '' 则不显示toolbar
p.circle(np.random.randn(500),np.random.randn(500))
show(p)
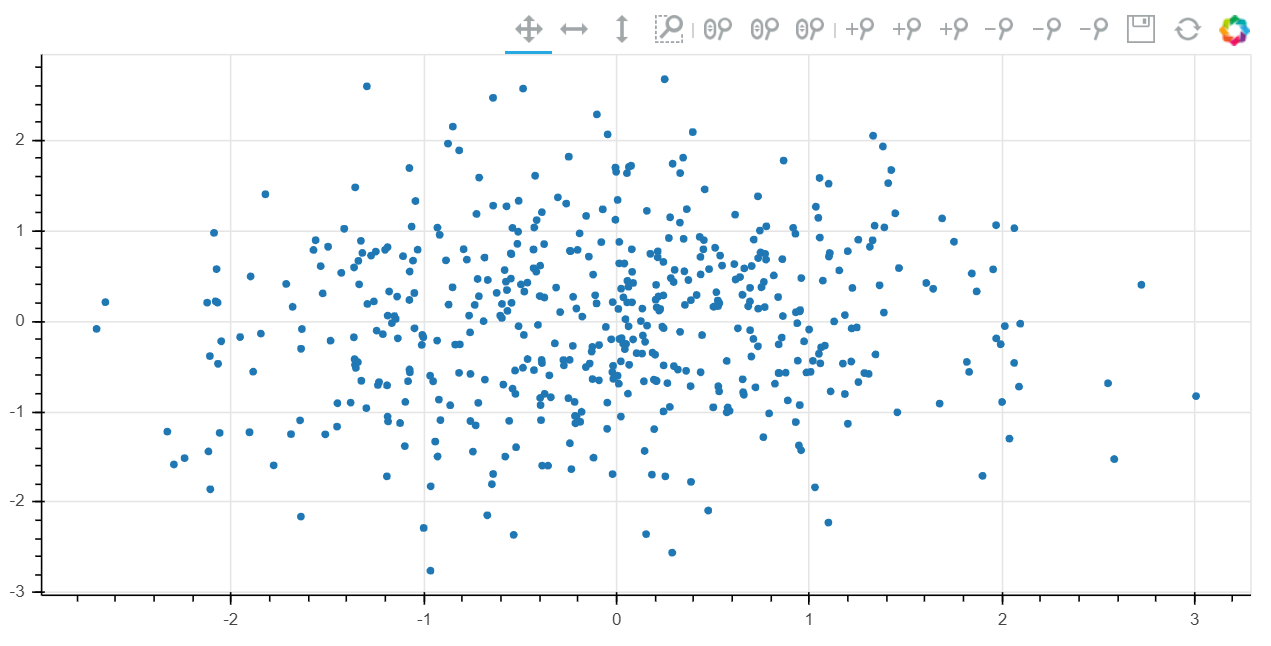
选择
# 工具栏 tools
# 选择
TOOLS = '''
box_select,lasso_select,
reset
'''
p = figure(plot_width=800, plot_height=400,toolbar_location="above",
tools = TOOLS)
# 添加toolbar
p.circle(np.random.randn(500),np.random.randn(500))
show(p)
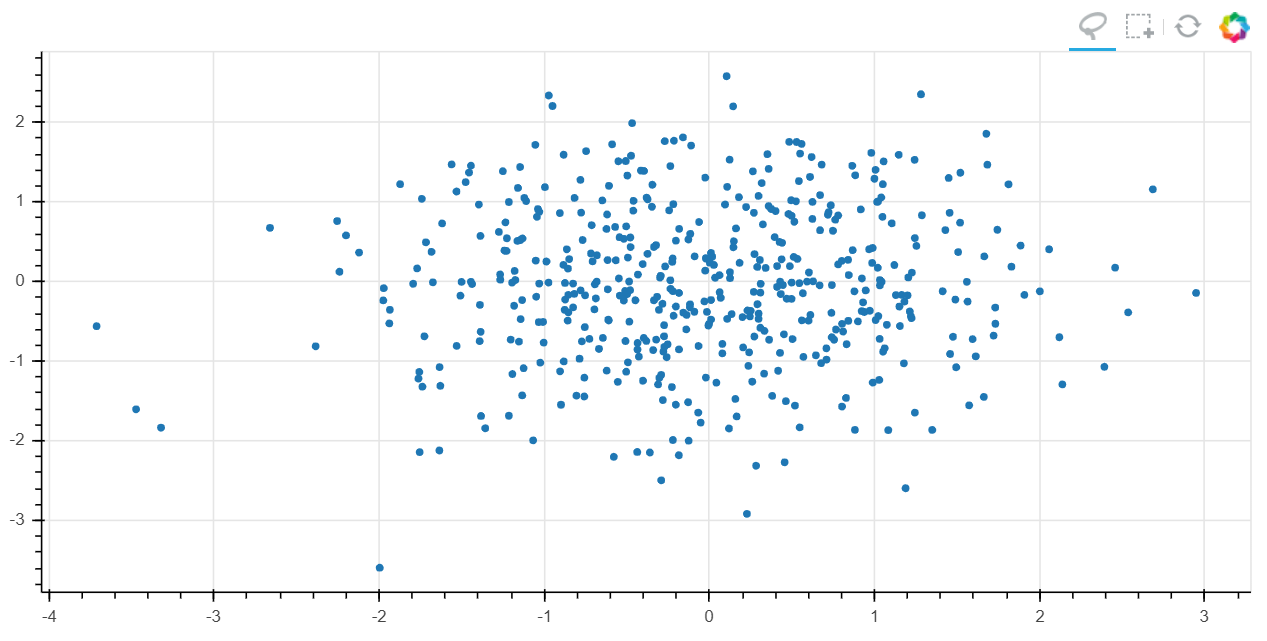
提示框、十字线
# 工具栏 tools
# 提示框、十字线
from bokeh.models import HoverTool
# 用于设置显示标签内容
df = pd.DataFrame({'A':np.random.randn(500)*100,
'B':np.random.randn(500)*100,
'type':np.random.choice(['pooh', 'rabbit', 'piglet', 'Christopher'],500),
'color':np.random.choice(['red', 'yellow', 'blue', 'green'],500)})
df.index.name = 'index'
source = ColumnDataSource(df)
print(df.head())
# 创建数据 → 包含四个标签
hover = HoverTool(tooltips=[
("index", "$index"),
("(x,y)", "($x, $y)"),
("A", "@A"),
("B", "@B"),
("type", "@type"),
("color", "@color"),
])
# 设置标签显示内容
# $index:自动计算 → 数据index
# $x:自动计算 → 数据x值
# $y:自动计算 → 数据y值
# @A:显示ColumnDataSource中对应字段值
p1 = figure(plot_width=800, plot_height=400,toolbar_location="above",
tools=[hover,'box_select,reset,wheel_zoom,pan,crosshair']) # 注意这里书写方式
# 如果不设置标签,就只写hover,例如 tools='hover,box_select,reset,wheel_zoom,pan,crosshair'
p1.circle(x = 'A',y = 'B',source = source,size = 10,alpha = 0.3, color = 'color')
show(p1)
p2 = figure(plot_width=800, plot_height=400,toolbar_location="above",
tools=[hover,'box_select,reset,wheel_zoom,pan'])
p2.vbar(x = 'index', width=1, top='A',source = source)
show(p2)
print(hover)
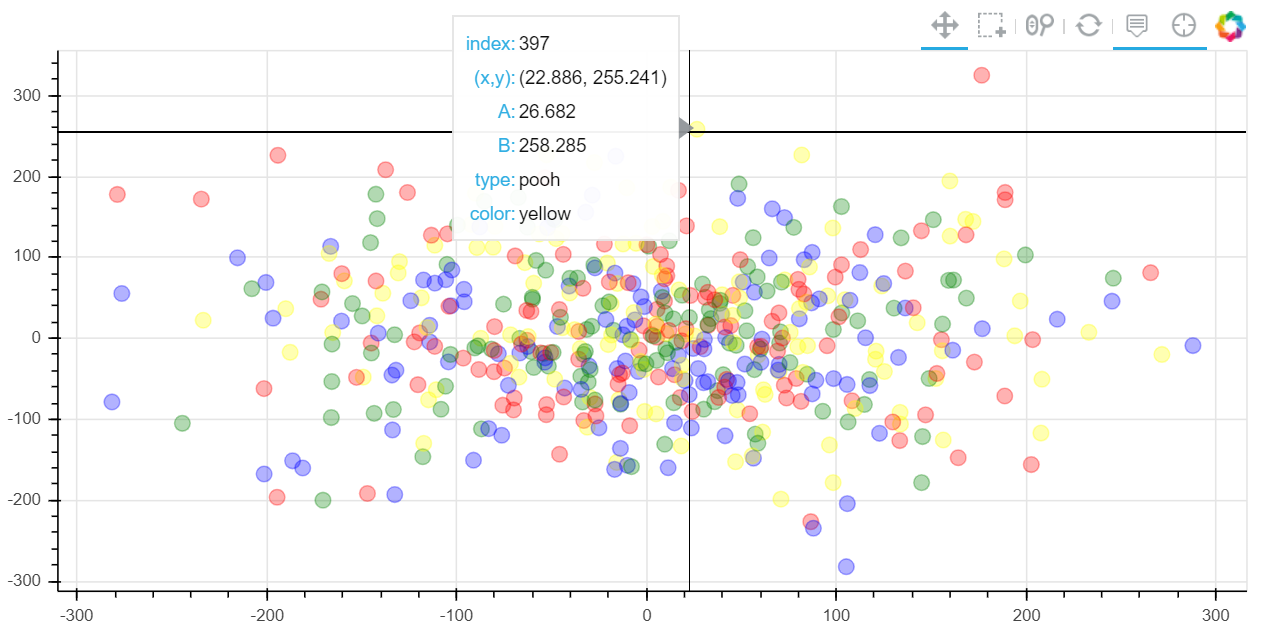
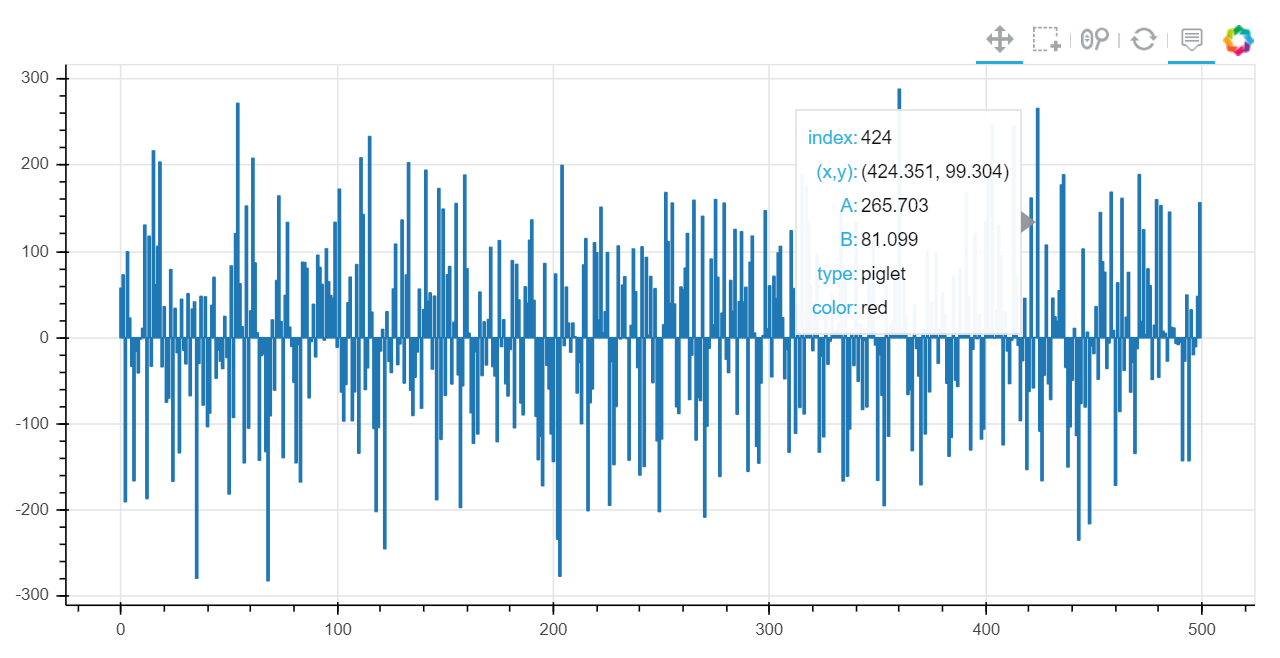