第一章:Java基础语法
第一个小案例HelloWorld
/*
Java程序中最基本的组成单位是类。
类的定义格式:
public class 类名{
}
*/
public class HelloWorld{
/*
这是main方法
main是程序入口,代码执行是从main方法开始的
*/
public static void main(String[] args){
// 这里是输出语句
System.out.println("HelloWorld");
}
}
一、常量类型和数据类型
1. 关键字
关键字的字母都是小写
System.out.println(); 输出内容并换行 System.out.print(); 输出内容不换行
2. 常量
public class HelloWorld{
public static void main(String[] args){
//字符串常量
System.out.println("我是字符串");
System.out.println("----------");
//整数
System.out.println(666);
System.out.println("----------");
//小数
System.out.println(13.14);
System.out.println("----------");
//字符常量
System.out.println('A');
System.out.println("----------");
//布尔值
System.out.println(true);
System.out.println("----------");
//空值 null 空值不能直接输出
}
}
3. 数据类型
public class HelloWorld{
public static void main (String[] args){
int a = 666;
System.out.println(a);
a = 888;
System.out.println(a);
}
}
long类型的变量定义的时候,为了防止整数过大,后面要加L
long a = 10000000L;
float类型的变量定义的时候,为了防止不兼容,后边要加F
float a = 13.14F;
4. 类型转换
自动类型转换
强制类型转换
二、运算符
1. 算数运算符
public class HelloWorld{
public static void main (String[] args){
int a = 6;
int b = 4;
System.out.println(a + b);
System.out.println(a - b);
System.out.println(a * b);
System.out.println(a / b);
System.out.println(a % b);
//除法得到的是商 取余得到的是余数
//整数相除只能得到整数,要想得到小数,必须有浮点数参与
System.out.println(6.0 / 4);
}
}
2. 字符的“+”操作
3. 字符串的“+”操作
4. 赋值运算符
5. 自增自减运算符
6. 关系运算符
7. 逻辑运算符
8. 短路逻辑运算符
public class HelloWorld{
/*
三元运算符
格式:
关系表达式 ? 表达式1 : 表达式2;
例子:
a > b ? a : b;
执行流程:
首先计算关系表达式的结果
结果为true,表达式1的值就是最终结果
结果为false,表达式2的值就是最终结果
*/
public static void main(String[] atgs){
int a = 10;
int b = 20;
int max = a > b ? a : b;
System.out.println(max);
}
}
三、数据输入
1. Scanner使用的基本步骤
导包
import java.util.Scanner; 导包必须要出现在类定义的上边
创建对象
Scanner sc = new Scanner(System.in);
接收数据
int x = sc.nextInt();
import java.util.Scanner;
public class HelloWorld{
public static void main(String[] args){
//创建对象
Scanner sc = new Scanner(System.in);
//接收数据
int x = sc.nextInt();
//输出数据
System.out.println("x:"+x);
}
}
2. 三元运算符加数据输入拓展
import java.util.Scanner;
public class HelloWorld{
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入第一个数值:");
int a = sc.nextInt();
System.out.println("请输入第二个数值:");
int b = sc.nextInt();
System.out.println("请输入第三个数值:");
int c = sc.nextInt();
int max = a > b ? a : b;
max = b>c ? b :c;
System.out.println("max是:"+max);
}
}
四、判断语句
1. if语句1(单一if)
1.2 if语句2(加上else判断)
1.3 if语句3(多次判断)
2. 奇偶数判断
import java.util.Scanner;
public class HelloWorld{
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入一个整数:");
int a = sc.nextInt();
//这里对变量a取余,如果能被2整除是偶数,不能的话就是奇数
if (a%2 == 0){
System.out.println("该数是偶数");
}else{
System.out.println("该数是奇数");
}
System.out.println("语句结束了");
}
}
3. 简单案例
import java.util.Scanner;
public class HelloWorld{
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入考试分数(0-100):");
int a = sc.nextInt();
if (a>100 || a<0){
System.out.println("请输入(0-100)");
}else if(a >=95 && a <= 100){
System.out.println("奖励山地自行车一辆");
}else if(a>=90 && a<=94){
System.out.println("奖励游乐场玩一次");
}else if(a>=80 && a<=89){
System.out.println("奖励变形金刚玩具一个");
}else{
System.out.println("奖励胖揍一顿");
}
}
}
涉及到运算符和判断语句的运用
4. swich语句
import java.util.Scanner;
public class HelloWorld{
public static void main (String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入1-3");
int a = sc.nextInt();
switch (a){
case 1:
System.out.println("你输入的是星期一");
break;
case 2:
System.out.println("你输入的是星期二");
break;
case 3:
System.out.println("你输入的是星期三");
break;
default:
System.out.println("你输入的有误");
//default在最后的话 default里面的break可以省略
break;
}
}
}
4.1 case穿透案例
//一年12个月份,输入一个月份让其显示对应的季节
import java.util.Scanner;
public class HelloWorld{
public static void main (String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入1-12的月份");
int a = sc.nextInt();
switch (a){
case 1:
case 11:
case 12:
System.out.println("冬季");
break;
case 2:
case 3:
case 4:
System.out.println("春季");
break;
case 5:
case 6:
case 7:
System.out.println("夏季");
break;
case 8:
case 9:
case 10:
System.out.println("秋季");
break;
default:
System.out.println("你输入的有误");
}
}
}
五、循环结构
1. for循环
import java.util.Scanner;
public class HelloWorld{
public static void main (String[] args){
Scanner sc = new Scanner(System.in);
int a;
for (a=1;a<10;a++){
System.out.println(a);
}
}
}
1.2 求和
import java.util.Scanner;
public class HelloWorld{
public static void main (String[] args){
Scanner sc = new Scanner(System.in);
int c=0;
int a;
for (a=1;a<10;a++){
c += a;
System.out.println(c);
}
System.out.println(c);
}
}
1.3 求偶数和
import java.util.Scanner;
public class HelloWorld{
public static void main (String[] args){
Scanner sc = new Scanner(System.in);
int c=0;
int a;
for (a=1;a<10;a++){
if (a%2 == 0){
c += a;
System.out.println(c);
}
}
System.out.println(c);
}
}
1.4 进阶(对100-999的数字进行判断,个、十、百每位数字的立方和等于原数字,则条件成立输出结果)
import java.util.Scanner;
public class HelloWorld{
public static void main (String[] args){
Scanner sc = new Scanner(System.in);
for (int c=100; c<=999; c++){
int g = c%10;
int s = c/10%10;
int b = c/100;
if (g*g*g + s*s*s + b*b*b == c){
System.out.println("符合条件:"+c);
}
}
}
}
2. while循环
public class HelloWorld{
public static void main (String[] args){
int a = 1;
while (a<10){
System.out.println(a);
a++;
}
}
}
2.2 案例
public class HelloWorld{
public static void main (String[] args){
double a = 0.1;
int c = 0;
while (a<=8844430){
c++;
a *= 2;
}
System.out.println("一共需要折叠:"+c+"次,长度是:"+a);
}
}
3. do…while循环
public class HelloWorld{
public static void main (String[] args){
int c = 0;
do {
System.out.println(c);
c++;
}while (c<10);
}
}
4. 三种循环的区别和死循环
public class HelloWorld{
public static void main (String[] args){
//--------------------------------第一种区别
//for循环
for (int c=5; c<5; c++){
System.out.println(c);
}
System.out.println("以上for循环");
//while循环
int c = 5;
while (c<5){
System.out.println(c);
c++;
}
System.out.println("以上while循环");
//do...while循环
int b = 5;
do {
System.out.println(b);
b++;
}while (b<5);
System.out.println("以上do...while循环");
System.out.println("");
//--------------------------------第二种区别
//for循环
for (int g=1; g<5; g++){
System.out.println("我是变量g我只能在这里显示"+g);
}
System.out.println("以上for循环");
//while循环
int f = 1;
while (f<5){
System.out.println("我是变量f我可以全局显示"+f);
f++;
}
System.out.println("循环体外"+f);
System.out.println("以上while循环");
//--------------------------------死循环
for(;;){
System.out.println("for");
}
while(true){
System.out.println("while");
}
do{
System.out.pirntln("di...while");
}while(true);
}
}
5. 跳转控制语句
6.循环嵌套
//取出一天的小时和分钟
public class HelloWorld{
public static void main (String[] args){
for (int hour = 0; hour<24; hour++){
int minute = 0;
do{
System.out.println(hour+"时"+minute+"分");
minute++;
}while (minute<60);
}
}
}
六、Random随机数
案例:猜数字小游戏
//需求:随机生成1-100的数字当作谜底 让用户输入判断是否等于谜底 给出相应的提示 直到结果等于66停止
import java.util.Random;
import java.util.Scanner;
public class HelloWorld{
public static void main(String[] args){
Random r = new Random();
int a = r.nextInt(100)+1;
while(true){
Scanner sc = new Scanner(System.in);
System.out.println("请输入要猜的数字(1-100)");
int c = sc.nextInt();
if (c<a){
System.out.println("数字"+c+"猜小了");
}else if (c>a){
System.out.println("数字"+c+"猜大了");
}else{
System.out.println("数字"+c+"恭喜你猜对了");
break;
}
}
}
}
七、数组
1. 数组(array)是用与存储多个相同类型数据的存储模型
2. 数组的初始化.
1. 动态初始化
package com.iteima;
public class Array {
public static void main(String[] args){
int[] arr = new int[3];
/*
左边:
int:定义数组元素类型是int类型
[]:说明定义的是一个数组
arr:定义的数组变量名
右边:
new:为数组申请新的内存空间
int:定义数组的元素类型是int
[]:这是一个数组
3:数组的长度,即数组的成员数
*/
}
}
2. 静态初始化
3. 数组的访问
package com.iteima;
public class Array {
public static void main(String[] args){
int[] arr = new int[3];
//输出数组名,改名字是内存申请创建的
System.out.println(arr);
//输出数组里的元素的值
System.out.println(arr[0]);
System.out.println(arr[1]);
System.out.println(arr[2]);
}
}
4. 内存分配
5. 数组操作常见的两个小问题
6. 数组的常见操作
1. 遍历(arr.length可以获取数组的长度)
package com.iteima;
public class Array{
public static void main(String[] args){
int[] arr = {1,2,3};
for(int a=0;a<arr.length;a++){
System.out.println(arr[a]);
}
}
}
2. 最大值和最小值
public class Array{
public static void main(String[] args){
int[] arr = {102,45,98,73,60};
int max = arr[0];
int min = arr[0];
for(int a=1; a<arr.length; a++){
if (arr[a]>max){
max = arr[a];
}else if (arr[a]<min){
min = arr[a];
}
}
System.out.println("最大值是:"+max);
System.out.println("最小值是:"+min);
}
}
七、方法
1. 什么是方法
2. 方法的定义和调用
- 案例一: ```java package com.iteima;
public class Array { public static void main(String[] args) { //方法的调用 isEvenNumber(); }
//方法的定义
public static void isEvenNumber() {
int number = 9;
if (number % 2 == 0) {
System.out.println("该数字是偶数" + number);
} else {
System.out.println("该数字是奇数" + number);
}
}
}
- 案例二:
```java
package com.iteima;
import java.util.Scanner;
public class Array {
public static void main(String[] args) {
getMax();
}
public static void getMax() {
Scanner sc = new Scanner(System.in);
System.out.println("请输入a的值:");
int a = sc.nextInt();
Scanner sc2 = new Scanner(System.in);
System.out.println("请输入b的值:");
int b = sc2.nextInt();
if (a > b) {
System.out.println(a);
} else {
System.out.println(b);
}
}
}
3. 带参数的方法的定义和调用
- 定义格式
- 调用格式
4. 形参和实参
- 案例 ```java package com.iteima;
public class Array { public static void main(String[] args) { getMax(10, 20); }
public static void getMax(int a, int b) {
if (a > b) {
System.out.println(a);
} else {
System.out.println(b);
}
}
}
<a name="HqVv0"></a>
#### 5. 带返回值的方法的定义和调用
- 定义
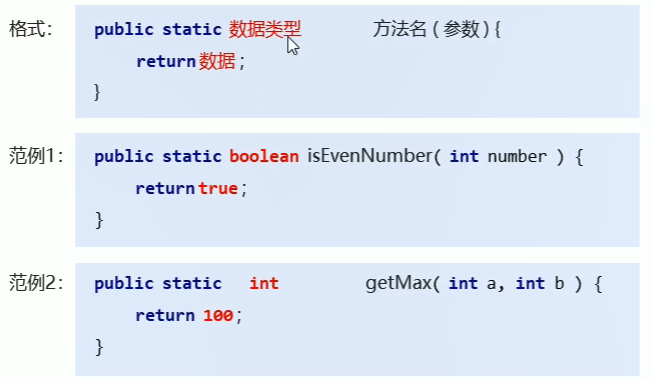
- 调用
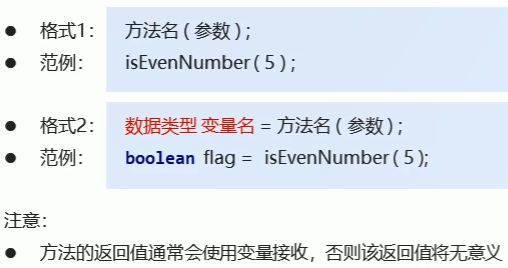
- 案例
```java
public class Array {
public static void main(String[] args) {
System.out.println(getBool(20));
}
public static boolean getBool(int a) {
if (a % 2 == 0) {
return (true);
} else {
return (false);
}
}
}
6. 方法的注意事项
7. 方法重载
public class Array {
public static void main(String[] args) {
System.out.println(compare(10, 20));
System.out.println(compare((byte)10,(byte)20));
System.out.println(compare((short)10,(short)20));
System.out.println(compare(10L, 20L));
}
public static boolean compare(int a, int b) {
System.out.println("int");
return (a == b);
}
public static boolean compare(long a, long b) {
System.out.println("long");
return a == b;
}
public static boolean compare(byte a, byte b) {
System.out.println("byte");
return a == b;
}
public static boolean compare(short a, short b) {
System.out.println("short");
return a == b;
}
}
8.方法的参数传递
基本类型的参数,形参值改变,不会影响实参的值
public class Array{
public static void main(String[] args){
int number=100;
System.out.println("调用前:"+number);
change(number);
System.out.println("调用后:"+number);
}
public static void change(int number){
number = 200;
}
}
引用类型的参数,形参值改变,会影响实参的值
public class Array{
public static void main(String[] args){
int[] arr={10,20,30};
System.out.println("调用前:"+arr[0]);
change(arr);
System.out.println("调用后:"+arr[0]);
}
public static void change(int[] arr){
arr[0] = 200;
}
}
案例:数组遍历使用方法调用来实现
//需求:设计一个方法用于数组遍历,要求遍历的结果是在一行上的。例如:[11, 22, 33, 44, 55]
public class Array {
public static void main(String[] args) {
int[] arr = {11, 22, 33, 44, 55};
printArray(arr);
}
/*
写方法首先需要明确的两件事
一:该方法是否具有返回值,如果不具备则默认void
二:该方法的调用参数是什么类型
*/
public static void printArray(int[] arr) {
System.out.print("[");
for (int a = 0; a < arr.length; a++) {
if (a == arr.length - 1) {
System.out.print(arr[a]);
} else {
System.out.print(arr[a] + ", ");
}
}
System.out.println("]");
}
}
案例:数组元素最大值的获取,使用方法调用来实现
public class Array {
public static void main(String[] args) {
int[] arr = {13, 52, 43, 99, 106};
printArray(arr);
}
/*
写方法首先需要明确的两件事
一:该方法是否具有返回值,如果不具备则默认void
二:该方法的调用参数是什么类型
*/
public static void printArray(int[] arr) {
int max = arr[0];
for (int a = 1; a < arr.length; a++) {
if (max < arr[a]) {
max = arr[a];
}
}
System.out.println("最大值是:" + max);
}
}
八、Debug调错
九、基础知识巩固练习
1.减肥计划 if 版本
//输入星期数,显示今天的减肥活动
/*
周一:跑步
周二:游泳
周三:慢走
周四:动感单车
周五:拳击
周六:爬山
周日:好好吃一顿
*/
import java.util.Scanner;
public class Array{
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入星期数(1-7):");
int week = sc.nextInt();
if (week == 1 ){
System.out.println("今天的活动是跑步");
}else if (week == 2){
System.out.println("今天的活动是游泳");
}else if (week == 3){
System.out.println("今天的活动是慢走");
}else if (week == 4){
System.out.println("今天的活动是动感单车");
}else if (week == 5){
System.out.println("今天的活动是拳击");
}else if (week == 6){
System.out.println("今天的活动是爬山");
}else if (week == 7){
System.out.println("今天的活动是好好吃一顿");
}else {
System.out.println("请输入正确的星期数(1-7)");
}
}
}
2. 减肥计划 switch 版本
//输入星期数,显示今天的减肥活动
/*
周一:跑步
周二:游泳
周三:慢走
周四:动感单车
周五:拳击
周六:爬山
周日:好好吃一顿
*/
import java.util.Scanner;
public class Array{
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入星期数(1-7):");
int week = sc.nextInt();
switch(week){
case 1:
System.out.println("今天的活动是跑步");
break;
case 2:
System.out.println("今天的活动是游泳");
break;
case 3:
System.out.println("今天的活动是慢走");
break;
case 4:
System.out.println("今天的活动是动感单车");
break;
case 5:
System.out.println("今天的活动是拳击");
break;
case 6:
System.out.println("今天的活动是爬山");
break;
case 7:
System.out.println("今天的活动是好好吃一顿");
break;
default:
System.out.println("请输入正确的星期数(1-7)");
}
}
}
3. 逢7过 for 和 取余
```java //1-100的数值,依次加1,当数值个位为7,十位为7,或者7的倍数时都要输出过,其余输出数值
public class Array{
public static void main(String[] args){
for (int i=1;i<101;i++){
if (i%7 == 0 || i%10 ==7 || i/10%10 == 7){
System.out.println(“过”);
}else {
System.out.println(i);
}
}
}
}
<a name="MDW7u"></a>
### 4. 不死神兔 动态数组 和 赋值
```java
//有一对兔子,出生后的第3个月起每个月都生一对,小兔子到第三个月往后每月再生一对,假如兔子都不死,到第十二个月兔子有多少对
/*
分析:
第一个月:1
第二个月:1
第三个月:2
第四个月:3
第五个月:5
```
规律:第三个月开始,当月数据是上两个月数据之和
*/
public class Array { public static void main(String[] args) { int[] arr = new int[20]; arr[0] = 1; arr[1] = 1; System.out.println(“第1个月:1”); System.out.println(“第2个月:1”); for (int i = 2; i < 12; i++) { arr[i] = arr[i - 1] + arr[i - 2]; System.out.println(“第” + (i + 1) + “个月:” + arr[i]); } } }
<a name="zRKDQ"></a>
### 5. 百钱百鸡 穷举思维 循环的嵌套
```java
//有100文钱,其中公鸡5文钱1只,母鸡3文钱1只,鸡雏1文钱3只,要想买100只鸡且刚好花了100文,问每种鸡各多少只
/*
分析:公鸡x,母鸡y,鸡雏z
x+y+z = 100只
5*x+3*x+z/3 = 100文
且
0<=x<=20
0<=y<=33
0<=z<=100
```
规律:第三个月开始,当月数据是上两个月数据之和
*/
public class Array { public static void main(String[] args) { for (int x = 0; x < 21; x++) { for (int y = 0; y < 34; y++) { int z = (100 - x - y); if (z % 3 == 0 && x 5 + y 3 + z / 3 == 100) { System.out.println(“公鸡” + x + “只,母鸡” + y + “只,鸡雏” + z + “只”); } } } } }
<a name="IfOyy"></a>
### 6. 数组元素求和 判断语句和取余
```java
//有一个数组{68,27,95,88,171,996,51,210} 需要的元素个位十位都不能是7,且只能是偶数,求和
/*
分析:
定义一个变量
两层判断:第一层、判断7;第二层、判断偶数,并将符合条件的元素值加到变量里面
*/
public class Array {
public static void main(String[] args) {
int sum = 0;
int[] arr = {68, 27, 95, 88, 171, 996, 51, 210};
for (int a = 0; a < arr.length; a++) {
if (arr[a] % 10 == 7 || arr[a] / 10 % 10 == 7 || arr[a] % 2 != 0) {
} else if (arr[a] % 2 == 0) {
sum += arr[a];
System.out.println(arr[a]);
}
}
System.out.println("最后的结果是:" + sum);
}
}
7.数组内容相同 方法调用静态数组
//设计一个方法,用于比较两个数组的内容是否相同
/*
分析:
1. 定义两个数组,静态初始化
2. 定义一个方法,用于比较两个数组的内容是否相同
返回值类型:boolean
参数:int[] arr1,int[] arr2
3. 比较数组长度,比较数组每个元素的值
*/
public class Array{
public static void main(String[] args){
int[] arr1 = {68,28,95};
int[] arr2 = {68,27,95};
System.out.println(boolArray(arr1,arr2));
}
public static boolean boolArray(int[] arr1,int[] arr2){
if (arr1.length == arr2.length){
for (int l=0;l<arr1.length;l++){
if (arr1[l] != arr2[l]){
return false;
}
}
return true;
}else{
return false;
}
}
}
8. 数组的元素值查找 break跳出循环
//已知一个数组arr={19,28,37,46,50};键盘录入一个数据,查找该数据在数组种的索引,不存在返回-1,存在返回索引值
/*
分析:
1. 定义数组静态初始化,定义索引,定义键盘录入数据
2. for循环判断录入数据和元素值是否相同 有相同返回索引 没想同返回-1
*/
import java.util.Scanner;
public class Array {
public static void main(String[] args) {
int[] arr = {19, 28, 37, 46, 50};
int index = -1;
Scanner sc = new Scanner(System.in);
System.out.print("请输入一个数值:");
int keyword = sc.nextInt();
for (int a = 0; a < arr.length; a++) {
if (arr[a] == keyword) {
index = a;
break;
}
}
System.out.println(index);
}
}
9. 数组元素的反转 数组的重定义
//已知一个数组arr={19,28,37,46,50};用方法程序实现数组元素值的反转
/*
分析:
1. 循环交换数组内的数值,注意开始和结束索引比较
2. 拿新的数组值去遍历输出
*/
import java.util.Scanner;
public class Array{
public static void main(String[] args){
int[] arr = {19,28,37,46,50};
fanzhuan(arr);
bianli(arr);
}
public static void fanzhuan(int[] arr){
for (int start=0,end=arr.length-1;start<=end;start++,end--){
int tmp = arr[start];
arr[start] = arr[end];
arr[end] = tmp;
}
}
public static void bianli(int[] arr){
System.out.print("[");
for (int a=0;a<arr.length;a++){
if (a == arr.length-1){
System.out.print(arr[a]);
}else {
System.out.print(arr[a]+", ");
}
}
System.out.println("]");
}
}
10. 评委打分
import java.util.Scanner;
public class Array {
public static void main(String[] args) {
int[] arr = new int[6];
for (int a = 0; a < 6; a++) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入第" + (a + 1) + "个评委的打分");
int keyword = sc.nextInt();
arr[a] = keyword;
}
int max = getMax(arr);
int small = getSmall(arr);
int sum = getSum(arr);
int average = (sum - small - max) / arr.length;
System.out.println("平均分是:" + average);
}
public static int getMax(int[] arr) {
int max = arr[0];
for (int a = 1; a < arr.length; a++) {
if (max < arr[a]) {
max = arr[a];
}
}
return max;
}
public static int getSmall(int[] arr) {
int small = arr[0];
for (int a = 1; a < arr.length; a++) {
if (small > arr[a]) {
small = arr[a];
}
}
return small;
}
public static int getSum(int[] arr) {
int sum = 0;
for (int a = 0; a < arr.length; a++) {
sum += arr[a];
}
return sum;
}
}