一、安装依赖
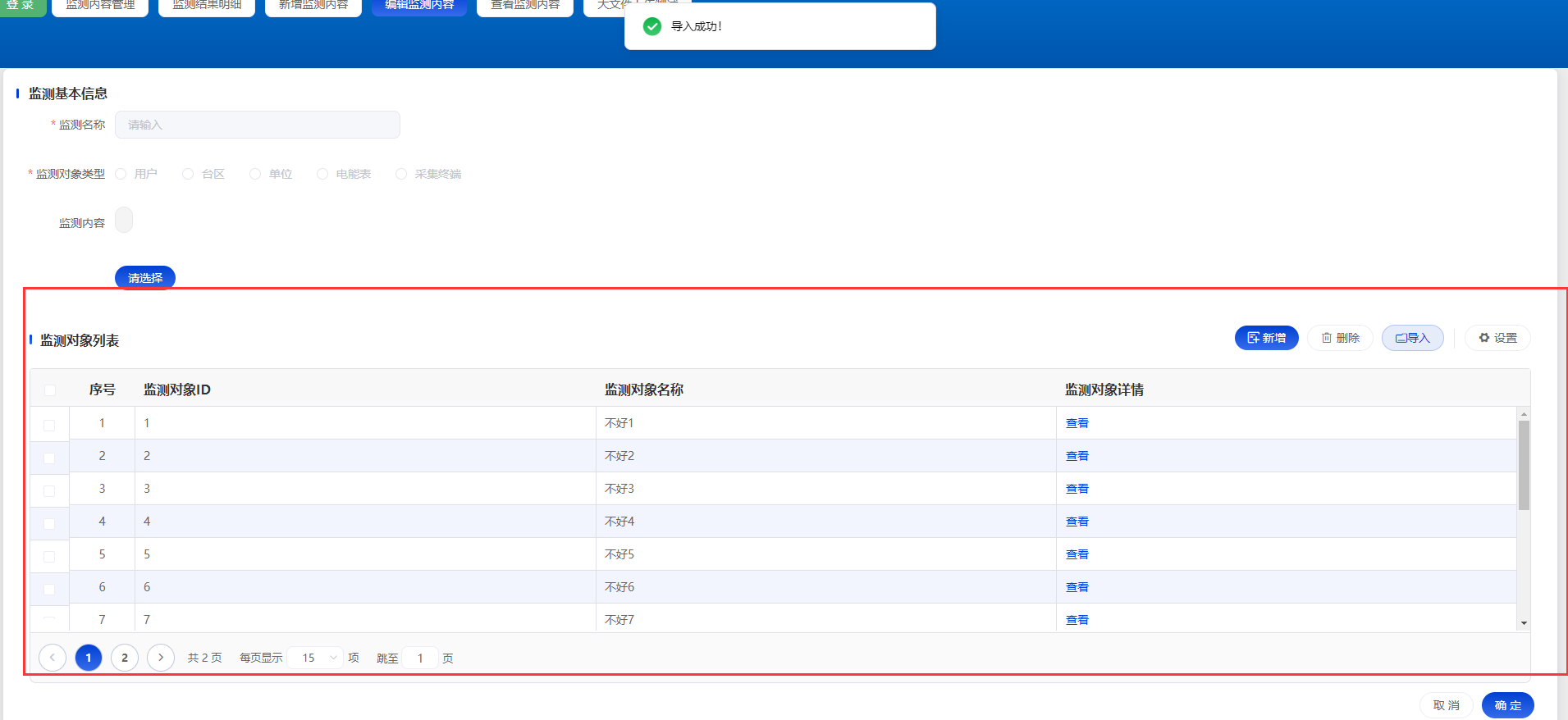
1、安装依赖
npm install -S file-saver xlsx
npm install -D script-loader
npm install xlsx
2、在main.js中引入XLSX
import XLSX from 'xlsx'
Vue.use(XLSX)
3.创建Filechang.js文件
/*
* @description:二进制表格读取
* @author: 王浩
* @Date: 2022-05-30 17:36:20
* @Modified By:
* @version: 1.0.0
*/
/**
* 把文件按照二进制进行读取
* @param {*} file
* @returns
*/
export function readFile(file) {
return new Promise((resolve) => {
let reader = new FileReader();
reader.readAsBinaryString(file);
// onload 读取完成后触发
reader.onload = (ev) => {
resolve(ev.target.result);
};
});
}
// 字段对应表
export let character = {
monitorObjId: {
text: "监测对象ID",
type: String,
},
monitorObjName: {
text: "监测对象名称",
type: String,
},
monitorObjectsId: {
text: "监测对象详情",
type: String,
},
};
4.使用
<!-- 导入文件 -->
<ami-upload
action="/ami/ma01-02-068/monitor/importObjs"
class="upload-demo"
ref="upload"
accept=".xls, .xlsx"
:limit="1"
auto-upload
:show-file-list="false"
:data="{ monitorFieldId }"
:file-list="fileList"
:before-upload="beforeUpload"
:before-remove="beforeRemove"
:on-exceed="handleExceed"
:on-change="handleChange"
:auto-upload="false"
>
<!-- -->
<ami-button class="import" round> <i class="ami-icons-daoru"></i> 导入</ami-button>
</ami-upload>
//----------------------------------导入部分----------------------------
import * as XLSX from "xlsx"; // 引入xlsx
import { readFile, character } from "@/utils/Filechang"; // 引入文件读取
// 采集excel数据
async handleChange(ev) {
// 判断是否为确定文件
if (ev.name === "测试导入文件.xlsx") {
const file = ev.raw;
if (!file) return;
// 读取excel数据
let reader = await readFile(file);
const worker = XLSX.read(reader, { type: "binary" });
const worsheet = worker.Sheets[worker.SheetNames[0]];
// 将返回的数据转换为json对象的数据
reader = XLSX.utils.sheet_to_json(worsheet);
// 将读取出来的数据转换为可以发送给服务器的数据
let data = [];
reader.forEach((item) => {
let obj = {};
for (let key in character) {
// eslint-disable-next-line no-prototype-builtins
if (!character.hasOwnProperty(key)) break;
let v = character[key];
let text = v.text;
let type = v.type;
v = item[text] || "";
type === "string" ? (v = String(v)) : null;
type === "number" ? (v = Number(v)) : null;
obj[key] = v;
}
data.push(obj);
});
if (data.length === 0) {
this.$message.error("请选择正确的excel文件!");
return;
} else {
// 拼接数据
this.objsVOS.list.push(...data);
return this.$message.success("导入成功!");
}
} else {
this.$refs.upload.clearFiles();
this.$message.error("请选择正确的excel文件!");
}
},
// 限制上传个数
handleExceed(files) {
if (files.length >= 1) {
this.$message.warning("只能上传一个文件");
this.$refs.upload.clearFiles();
return false;
} else if (files.length === 0) {
this.$message.warning("请选择文件");
}
},
// 文件上传前限制
beforeUpload(file) {
console.log(file);
const testmsg = file.name.substring(file.name.lastIndexOf(".") + 1);
const extension = testmsg === "xls" || "xlsx";
const isLt2M = file.size / 1024 / 1024 < 10; //这里做文件大小限制
if (!extension) {
this.$message.error("文件上传只能是xls、xlsx 格式");
return false;
}
if (!isLt2M) {
this.$message.error("文件大小不能超过 10MB!");
return false;
}
return extension && isLt2M;
},
// 文件移除
beforeRemove() {
this.$refs.upload.clearFiles();
},