判断Versiondata是否为空,如果为空停止
if (this.Versiondata==undefined||this.Versiondata.length<=0) return;
1.字节转换单位 MB
/**
* 文件大小 字节转换单位
* @param size
* @returns {string|*}
* @name:王浩
* @params:封装在utils里面的方法
*/
export const filterSize = (size) => {
if (!size) return "";
if (size < pow1024(1)) return size + " B";
if (size < pow1024(2)) return (size / pow1024(1)).toFixed(2) + " KB";
if (size < pow1024(3)) return (size / pow1024(2)).toFixed(2) + " MB";
if (size < pow1024(4)) return (size / pow1024(3)).toFixed(2) + " GB";
return (size / pow1024(4)).toFixed(2) + " TB";
};
// 求次幂
function pow1024(num) {
return Math.pow(1024, num);
}
-----------------------------------------------------------------------------------------
/**
* 根据字典的value查找对应的index
*/
export const findArray = (dic, value) => {
for (let i = 0; i < dic.length; i++) {
if (dic[i].value === value) {
return i;
}
}
return -1;
};
/**
* 根据字典的value显示label
*/
export const findByValue = (dic, value) => {
let result;
if (validateNull(dic)) return value;
if (typeof value == "string" || typeof value == "number" || typeof value == "boolean") {
let index = 0;
index = findArray(dic, value);
if (index !== -1) {
result = dic[index].label;
} else {
result = value;
}
} else if (value instanceof Array) {
result = [];
let index = 0;
value.forEach((ele) => {
index = findArray(dic, ele);
if (index !== -1) {
result.push(dic[index].label);
} else {
result.push(value);
}
});
result = result.toString();
}
return result;
};
/**
* 递归寻找子类的父类
*/
export const findParent = (menu, id) => {
for (let i = 0; i < menu.length; i++) {
if (menu[i].children.length != 0) {
for (let j = 0; j < menu[i].children.length; j++) {
if (menu[i].children[j].id == id) {
return menu[i];
} else {
if (menu[i].children[j].children.length != 0) {
return findParent(menu[i].children[j].children, id);
}
}
}
}
}
};
- 在mian.js中使用,设置成全局过滤器 ```javascript import * as filterSize from “@/utils/chang.js”; //字节转换单位MB
Object.keys(filterSize).forEach((key) => { Vue.filter(key, filterSize[key]); });
++ 组件中直接使用{{day | filterSize }} filterSize 为过滤,转成字节
<a name="ALRcP"></a>
### 2.时间过滤器
```javascript
/**
* @name:王浩
* @params:封装在utils里面的方法
* @description: 当前系统日期 yyyy-MM-dd
* @param {String} date 需要转换的时间例如:"2020-1-1"
* @return {String} "2020-01-01"
*/
export const getYearMonthDay = function (date) {
const d = new Date(date);
const yyyy = d.getFullYear();
let MM = d.getMonth() + 1;
MM = MM < 10 ? `0${MM}` : MM;
let dd = d.getDate();
dd = dd < 10 ? `0${dd}` : dd;
return `${yyyy}-${MM}-${dd}`;
};
/**
* @description: 将时间转换为2020/1/1
* @param {String} timestamp 需要转换的时间
* @return {String} t 转换后的时间
*/
export const formatTs = function (timestamp) {
const t = new Date(timestamp).toLocaleDateString();
return t;
};
/**
* @description: 与当前时间的时间差
* @param {String} date 需要转换的时间
* @return {String} 时间差
*/
export const formatTimestamp = function (date) {
const t = new Date(date).toLocaleDateString();
const timestamp = new Date(t);
const currentTime = new Date();
return currentTime - timestamp;
};
/**
* @description: 返回当前月和最后一天
* @param {String} timeSecond 需要转换的时间例如:"2020-1-1"
* @return {Array} ["2020-01-01", "2020-01-31", "2020-01"]
*/
export const getDateRange = function (timeSecond) {
const timeDifference = formatTimestamp(timeSecond);
const time = new Date(timeSecond);
const year = time.getFullYear();
let month = time.getMonth() + 1;
const month2 = month;
let day = timeDifference >= 30 * 24 * 3600 * 1000 ? time.getDate() : new Date(new Date()).getDate();
if (month < 10) {
month = `0${month}`;
}
if (day < 10) {
day = `0${day}`;
}
const day31 = [1, 3, 5, 7, 8, 10, 12];
let timeStart = null;
let timeEnd = null;
const yearMonth = `${year}-${month}`;
// 获取当前的时间
if (timeDifference >= 30 * 24 * 3600 * 1000) {
timeStart = `${year}-${month}-${day}`;
for (let i = 0; i < day31.length; i++) {
if (day31[i] === month2) {
timeEnd = `${year}-${month}-${31}`;
break;
} else if (month2 === 2) {
timeEnd = `${year}-${month}-${28}`;
} else {
timeEnd = `${year}-${month}-${30}`;
}
}
} else {
timeStart = `${year}-${month}-${"01"}`;
timeEnd = `${year}-${month}-${day}`;
}
return [timeStart, timeEnd, yearMonth];
};
/**
* @description: 当前系统日期 yyyy-MM-dd
* @param {String} date 需要转换的时间例如:"2020-1-1"
* @return {String} "2020-01-01"
*/
export const getYearMonthDay = function (date) {
const d = new Date(date);
const yyyy = d.getFullYear();
let MM = d.getMonth() + 1;
MM = MM < 10 ? `0${MM}` : MM;
let dd = d.getDate();
dd = dd < 10 ? `0${dd}` : dd;
return `${yyyy}-${MM}-${dd}`;
};
/**
* @description: 格式 年-月
* @param {String} date 需要转换的时间例如:"2020-1-1"
* @return {String} 年月
*/
export const getYearMonth = function (date) {
const d = new Date(date);
const datetime = d.getFullYear() + "-" + (d.getMonth() + 1);
return datetime;
};
/**
* @description: 格式 时间到时分秒
* @param {String} date 需要转换的时间例如:"2020-1-1"
* @return {String} 年月日时分秒
*/
export const format = function (date) {
if (!date) return;
var date2 = new Date(date);
var y = date2.getFullYear();
var m = date2.getMonth() + 1;
m = m < 10 ? "0" + m : m;
var d = date2.getDate();
d = d < 10 ? "0" + d : d;
var h = date2.getHours();
h = h < 10 ? "0" + h : h;
var minute = date2.getMinutes();
minute = minute < 10 ? "0" + minute : minute;
var seconds = date2.getSeconds();
seconds = seconds < 10 ? "0" + seconds : seconds;
return y + "-" + m + "-" + d + " " + h + ":" + minute + ":" + seconds;
};
/**
* @description: 格式 处理时间到时
* @param {String} date 需要转换的时间
* @param {String} h 不足两位补两位
* @return {String} 年月日时
*/
export const todayTime = function (date, h) {
var date2 = new Date(date);
var y = date2.getFullYear();
var m = date2.getMonth() + 1;
m = m < 10 ? "0" + m : m;
var d = date2.getDate();
d = d < 10 ? "0" + d : d;
h = h < 10 ? "0" + h : h;
return y + "-" + m + "-" + d + " " + h + ":" + "00" + ":" + "00";
};
/**
* @description: 格式 处理时间到年月日
* @param {String} date 需要转换的时间
* @return {String} 年月日
*/
export const todayTime1 = function (date) {
var date2 = new Date(date);
var y = date2.getFullYear();
var m = date2.getMonth() + 1;
m = m < 10 ? "0" + m : m;
var d = date2.getDate();
d = d < 10 ? "0" + d : d;
return y + "-" + m + "-" + d + " " + "00" + ":" + "00" + ":" + "00";
};
/**
* @description: 格式 获取是分秒
* @param {String} date 需要转换的时间
* @param {String} type 转换类型
* @return {String} 时分/时分秒
*/
export const formatTime = function (date, type) {
if (!date) return;
var h = date.getHours();
h = h < 10 ? "0" + h : h;
var minute = date.getMinutes();
minute = minute < 10 ? "0" + minute : minute;
var seconds = date.getSeconds();
seconds = seconds < 10 ? "0" + seconds : seconds;
if (type === "HH:mm") {
return h + ":" + minute;
}
return h + ":" + minute + ":" + seconds;
};
/**
* @description: 格式 获取周几
* @param {String} date 需要转换的时间
* @return {String} 星期几
*/
export const formatDay = function (date) {
if (!date) return;
var days = date.getDay();
switch (days) {
case 1:
days = "星期一";
break;
case 2:
days = "星期二";
break;
case 3:
days = "星期三";
break;
case 4:
days = "星期四";
break;
case 5:
days = "星期五";
break;
case 6:
days = "星期六";
break;
case 0:
days = "星期日";
break;
}
return days;
};
/**
* @description: 转换时间戳年月日
* @param {String} str 需要转换的时间
* @return {String} 时间戳
*/
export const getLocalTimes = (str) => {
if (!str || str === null || str === "") {
return str;
}
var tf = function (i) {
return (i < 10 ? "0" : "") + i;
};
var oDate = new Date(str);
var oYear = oDate.getFullYear() + "-";
var oMonth = (oDate.getMonth() + 1 < 10 ? "0" + (oDate.getMonth() + 1) : oDate.getMonth() + 1) + "-";
var oDay = tf(oDate.getDate()) + " ";
return oYear + oMonth + oDay;
};
/**
* @description 获取当前时间的年月日信息星期几的信息
* @param date
* @return {{month: number, year: number, days: number, day: number}}
*/
export const getNewDate = (date) => {
let year = date.getFullYear();
let month = date.getMonth();
let day = date.getDate();
let days = date.getDay();
return { year, month, day, days };
};
/**
* @description获取一个月份的所有天数
* @param year 年份
* @param month 月份
* @return {[]} 一个月所有天数的数组
*/
export const getMonthList = (year, month) => {
let d = new Date(year, month, 0).getDate(); // 获取某一个月的天数
let list = [];
for (let j = 0; j < d; j++) {
list.push(month + "-" + (j + 1));
}
return list;
};
/**
* 返回每个月的开始时候和结束时间,或者一年的开始时间和结束时间
* @param date 时间对象
* @param status 1:月,2:年
* @param flag 0:开始时间,1:结束时间
* @returns {string} 返回时间字符串格式 xxxx-xx-xx xx:xx:xx
*/
export const getYearOrMonthFirstTime = (date, status, flag) => {
let year = date.getFullYear();
let month = date.getMonth();
if (status === 1) {
if (flag === 0) {
month = month + 1;
return year + "-" + (month < 10 ? `0${month}` : month) + "-01 00:00:00";
} else {
let day = new Date(year, month, 0);
month = month + 1;
return year + "-" + (month < 10 ? `0${month}` : month) + "-" + day.getDate() + " 23:59:59";
}
} else {
if (flag === 0) {
return year + "-" + "01" + "-01 00:00:00";
} else {
return year + "-" + "12" + "-31 23:59:59";
}
}
};
/**
* @description 根据当前时间获取上个月的时间
* @param date 传入的时间
* @return {string} 返回时间字符串格式 xxxx-xx-xx xx:xx:xx
*/
export const getLastMouthDate = (date) => {
let year = date.getFullYear();
let month = date.getMonth();
let dateTime = new Date(year, month - 1, 1);
return format(dateTime);
};
/**
* @description 获取当前月的第一天
* @param date 日期时间
* @return {string} 返回xxxx-xx-xx格式的字符串时间
*/
export const getMouthFirstDay = (date) => {
const d = new Date(date);
const yyyy = d.getFullYear();
let MM = d.getMonth() + 1;
MM = MM < 10 ? `0${MM}` : MM;
return `${yyyy}-${MM}-01`;
};
/**
* Parse the time to string
* @param {(Object|string|number)} time
* @param {string} cFormat
* @returns {string | null}
*/
export function parseTime(time, cFormat) {
if (arguments.length === 0) {
return null;
}
const format = cFormat || "{y}-{m}-{d} {h}:{i}:{s}";
let date;
if (typeof time === "object") {
date = time;
} else {
if (typeof time === "string" && /^[0-9]+$/.test(time)) {
time = parseInt(time);
}
if (typeof time === "number" && time.toString().length === 10) {
time = time * 1000;
}
date = new Date(time);
}
const formatObj = {
y: date.getFullYear(),
m: date.getMonth() + 1,
d: date.getDate(),
h: date.getHours(),
i: date.getMinutes(),
s: date.getSeconds(),
a: date.getDay(),
};
const time_str = format.replace(/{([ymdhisa])+}/g, (result, key) => {
const value = formatObj[key];
// Note: getDay() returns 0 on Sunday
if (key === "a") {
return ["日", "一", "二", "三", "四", "五", "六"][value];
}
return value.toString().padStart(2, "0");
});
return time_str;
}
- 在mian.js中使用,设置成全局过滤器 ```javascript import * as getYearMonthDay from “@/utils/chang.js”; //时间格式化:yyyy-MM-dd
Object.keys(getYearMonthDay).forEach((key) => { Vue.filter(key, getYearMonthDay[key]); });
- [x] 组件中直接使用`{{day | getYearMonthDay }}` **getYearMonthDay 为过滤**
<a name="WZV1T"></a>
### 3.vue中二级联动
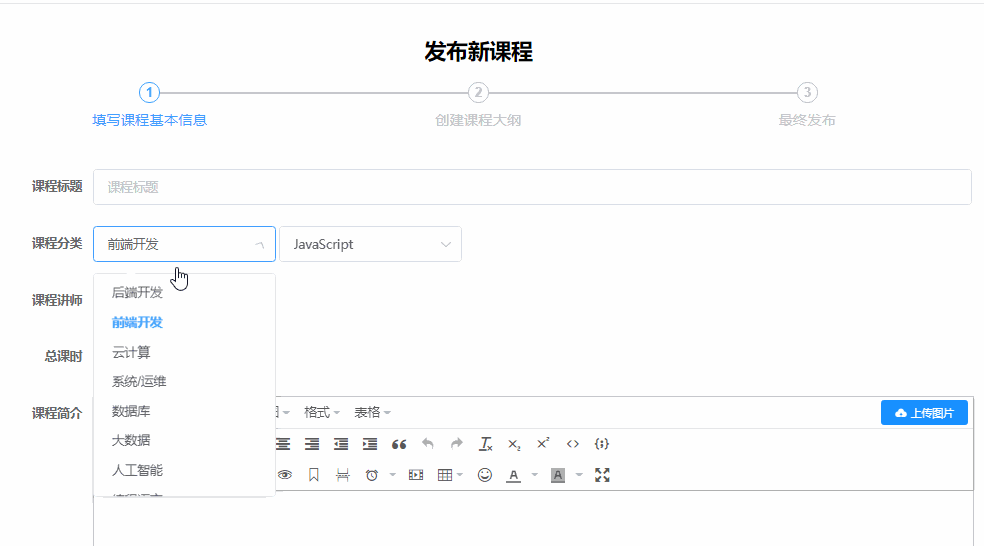
1. 后端返回的数据格式
```javascript
"list": [
{
"id": "1178214681118568449",
"title": "后端开发",
"children": [
{
"id": "1178214681139539969",
"title": "Java"
},
{
"id": "1178585108407984130",
"title": "Python"
},
{
"id": "1454645056483913730",
"title": "C++"
},
{
"id": "1454645056731377666",
"title": "C#"
}
]
},
{
"id": "1178214681181483010",
"title": "前端开发",
"children": [
{
"id": "1178214681210843137",
"title": "JavaScript"
},
{
"id": "1178585108454121473",
"title": "HTML/CSS"
}
]
},
{
"id": "1178214681231814658",
"title": "云计算",
"children": [
{
"id": "1178214681252786178",
"title": "Docker"
},
{
"id": "1178214681294729217",
"title": "Linux"
}
]
},
{
"id": "1178214681324089345",
"title": "系统/运维",
"children": [
{
"id": "1178214681353449473",
"title": "Linux"
},
{
"id": "1178214681382809602",
"title": "Windows"
}
]
},
{
"id": "1178214681399586817",
"title": "数据库",
"children": [
{
"id": "1178214681428946945",
"title": "MySQL"
},
{
"id": "1178214681454112770",
"title": "MongoDB"
}
]
},
{
"id": "1178214681483472898",
"title": "大数据",
"children": [
{
"id": "1178214681504444418",
"title": "Hadoop"
},
{
"id": "1178214681529610242",
"title": "Spark"
}
]
},
{
"id": "1178214681554776066",
"title": "人工智能",
"children": [
{
"id": "1178214681584136193",
"title": "Python"
}
]
},
{
"id": "1178214681613496321",
"title": "编程语言",
"children": [
{
"id": "1178214681626079234",
"title": "Java"
}
]
}
]
- vue页面中
```javascript
export default { data(){ return{ Application: [], //应用分类 business: [], //业务分类 } } }
初始化数据,调用接口 // 应用分类 Applicationls() { queryCategory().then((res) => { this.Application = res.data.data; }); }, // 业务分类—-接口 getRole(val) { for (var i = 0; i < this.Application.length; i++) { // 每个一级分类 var oneSubject = this.Application[i]; // 判断:所有一级分类id和点击分类一级id是否一样 if (val === oneSubject.no) { this.business = oneSubject.childCategoryList; console.log(this.business); // 把二级分类id值清空 this.form.businessCategory = “”; } } },
<a name="iXlze"></a>
### 4.vue中导出文件exc
```javascript
export const exportFile = (result) => {
console.log("result", result);
let contentDisposition = result.headers["content-disposition"];
// 这里后端给的内容中,文件名字可能是驼峰式名称的 fileName ,或者是全部小写的 filename
let filename = decodeURI(contentDisposition.split("fileName=")[1] || contentDisposition.split("filename=")[1]);
// let filename = "1.xlsx";
// 注意这里的 result.data ,如果只传 result 的话,最后下载出来的excel文件,里面显示的是 [object Object]
let blob = new Blob([result.data], { type: result.headers["content-type"] });
// let blob = new Blob([result.data],{type: "application/x-msdownload;charset=GBK"});
// let blob = new Blob([result.data],{type: "application/x-msdownload"});
// let blob = new Blob([result.data]);
// let blob = new Blob([result.data],{type: "application/vnd.ms-excel"});
let url = window.URL.createObjectURL(blob);
if (window.navigator.msSaveBlob) {
//IE
try {
window.navigator.msSaveBlob(blob, filename);
} catch (e) {
console.log(e);
}
} else {
//非IE
let link = document.createElement("a");
link.style.display = "none";
link.href = url;
link.setAttribute("download", filename);
document.body.appendChild(link);
link.click();
}
URL.revokeObjectURL(url); // 释放内存
};
在组件中引用:
_import { exportFile } from "@/utils/exportFile"; //导出文件--封装的方法_
// 文件导出 exportExcels() { this.$confirm("确定导出需求信息管理信息?", "提示", { type: "warning", dangerouslyUseHTMLString: true, }).then(() => { //通过ids集合到demandIdList数组 let ids = this.form.demandIdList; console.log(ids); this.$refs.multipleTable.selection.forEach((e) => { ids.push(parseInt(e.demandId), (this.form.type = "02")); //全选传入type参数02 }); exportExcel(this.tableData).then((res) => { if (res.status === 200) { console.log(res.data); // exportFile(res); //导出文件 const blob = new Blob([res.data]); const a = document.createElement("a"); const url = window.URL.createObjectURL(blob); a.href = url; a.download = "需求信息列表.xlsx"; //文件名称 a.click(); window.URL.revokeObjectURL(url); } else { this.$message({ message: "服务器错误", type: "error", duration: 2000, }); } }); }); },
5.vue中文字超过3行展示文字(隐藏)
如果是弹框中的话不能使用mounted 需要重新创建一个方法在methods当中
// 展开(需要dom加载完执行此方法) Unfold() { this.versionList.forEach((ele, index) => { this.$set(this.versionList, index, Object.assign({}, ele, { status: null })); }); // DOM 加载完执行 this.$nextTick(() => { this.calculateText(); }); window.onresize = () => { this.versionList.forEach((ele, index) => { this.$set(this.versionList, index, Object.assign({}, ele, { status: null })); }); setTimeout(() => { this.calculateText(); }, 0); }; },
其他组件调用它时,需要用 setTimeout 来执行,不要获取报错 undefined 就是取不到这个值
```html <!DOCTYPE html>setTimeout(() => { this.$refs.child.Unfold(); //触发子组件中 Unfold(需要dom加载完执行此方法) }, 0);
展开
收起
<a name="z22sl"></a>
### 6.表格中设置 ` row-key="tableKey" ` 就不会报错
- [ ] 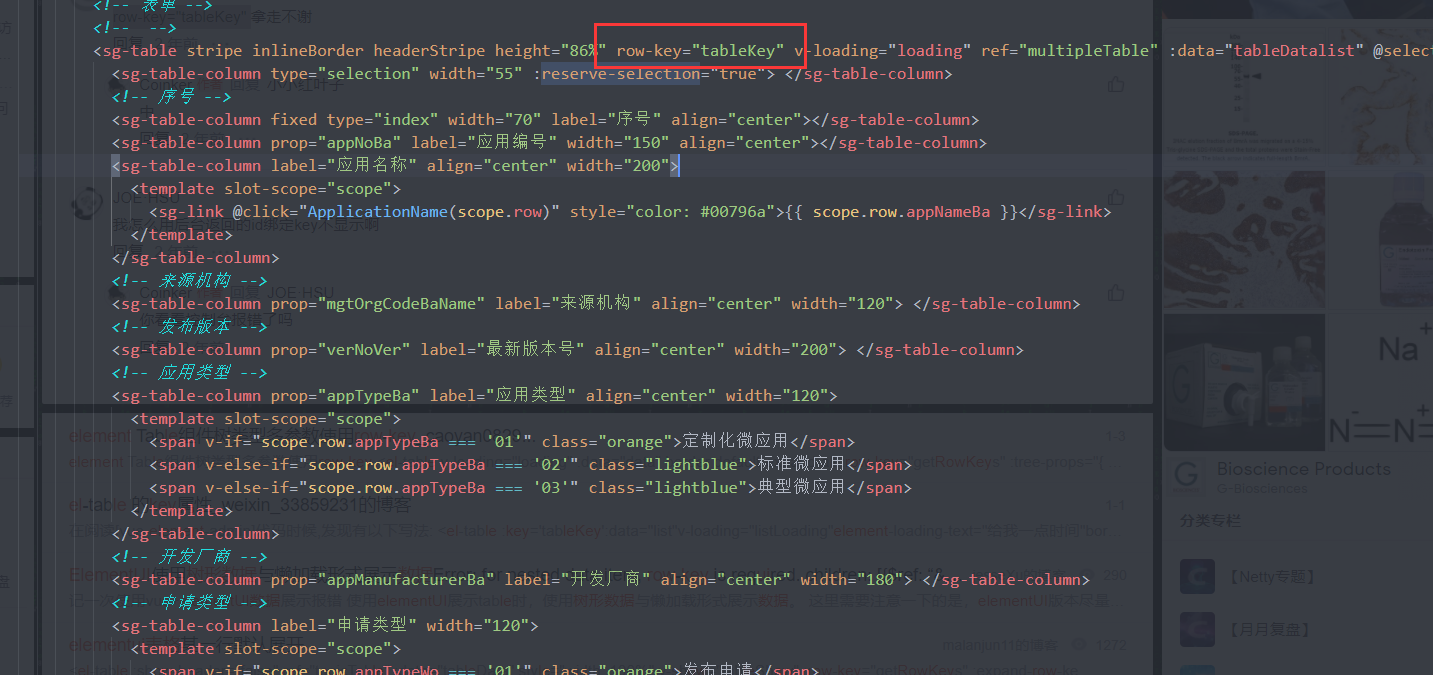
<a name="sYFp0"></a>
### 7.下载附件
```javascript
export const exportFile = (result) => {
console.log("result", result);
let contentDisposition = result.headers["content-disposition"];
// 这里后端给的内容中,文件名字可能是驼峰式名称的 fileName ,或者是全部小写的 filename
let filename = decodeURI(contentDisposition.split("fileName=")[1] || contentDisposition.split("filename=")[1]);
// let filename = "1.xlsx";
// 注意这里的 result.data ,如果只传 result 的话,最后下载出来的excel文件,里面显示的是 [object Object]
let blob = new Blob([result.data], { type: result.headers["content-type"] });
// let blob = new Blob([result.data],{type: "application/x-msdownload;charset=GBK"});
// let blob = new Blob([result.data],{type: "application/x-msdownload"});
// let blob = new Blob([result.data]);
// let blob = new Blob([result.data],{type: "application/vnd.ms-excel"});
let url = window.URL.createObjectURL(blob);
if (window.navigator.msSaveBlob) {
//IE
try {
window.navigator.msSaveBlob(blob, filename);
} catch (e) {
console.log(e);
}
} else {
//非IE
let link = document.createElement("a");
link.style.display = "none";
link.href = url;
link.setAttribute("download", filename);
document.body.appendChild(link);
link.click();
}
URL.revokeObjectURL(url); // 释放内存
};
-------------------------添加时间------------------------------------------------------------------
/**
* @description:导出(下载附件)
* @author:这里添加了dayjs中插件(格式化时间)
* @date:Created in 2021/12/22 16:20
* @modified By:王浩
* @version: 1.0.0
*/
import dayjs from "dayjs"; //时间格式化
export const exportFile = (result) => {
console.log("result", result);
let contentDisposition = result.headers["content-disposition"];
// 这里后端给的内容中,文件名字可能是驼峰式名称的 fileName ,或者是全部小写的 filename
let data = new Date(); //获取当前时间
let Time = dayjs(data).format("YYYY-MM-DD") + "_";
let filename = decodeURI(contentDisposition.split("fileName=")[1] || contentDisposition.split("filename=")[1]);
// 另一种方法
// let filename = window.decodeURI(contentDisposition.substring(contentDisposition.indexOf("=") + 1));
// 注意这里的 result.data ,如果只传 result 的话,最后下载出来的excel文件,里面显示的是 [object Object]
let blob = new Blob([result.data], { type: result.headers["content-type"] });
// let blob = new Blob([result.data],{type: "application/x-msdownload;charset=GBK"});
// let blob = new Blob([result.data],{type: "application/x-msdownload"});
// let blob = new Blob([result.data]);
// let blob = new Blob([result.data],{type: "application/vnd.ms-excel"});
let url = window.URL.createObjectURL(blob);
if (window.navigator.msSaveBlob) {
//IE
try {
window.navigator.msSaveBlob(blob, filename);
} catch (e) {
console.log(e);
}
} else {
//非IE
let link = document.createElement("a");
link.style.display = "none";
link.href = url;
link.setAttribute("download", Time + filename);
document.body.appendChild(link);
link.click();
}
URL.revokeObjectURL(url); // 释放内存
};
- 调用接口进行使用 ```javascript
// import { exportFile } from “@/utils/exportFile”; //导出文件—封装的方法 downLoadFiles() { // 传入附件id this.storeId = this.Checklist.annex; downLoadFile(this.storeId).then((res) => { // exportFile(res); 引用导出的方法 let fileName = “附件”; let url = window.URL.createObjectURL(new Blob([res.data])); let link = document.createElement(“a”); link.style.display = “none”; link.href = url; link.setAttribute(“download”, fileName); document.body.appendChild(link); link.click(); this.$message({ type: “success”, message: “下载文件成功!”, }); }); },
<a name="OGSZa"></a>
### 8.排序
1.在methods中写排序方法
```javascript
// 排序
sortKey(array, key) {
return array.sort(function (a, b) {
var x = a[key];
var y = b[key];
return x > y ? -1 : x < y ? 1 : 0;
});
},
2.在computed 计算属性中计算
//根据时间进行的排序
newdataList() {
return this.sortKey(this.commentlist, "evalTime");
},
9.表单校验
/**
* @description:自定义校验规则
* @author:
* @date:Created in 2021/12/22 16:20
* @modified By:
* @version: 1.0.0
*/
const { validUpper } = require("@/utils/validate");
// 只能输入大写字母与下划线
export const tableCodeValidUpper = (rule, val, callback) => {
if (val != null && val != "") {
const value = val.replace(/^\s*|\s*$/g, "");
if (!validUpper(value)) {
callback(new Error("只能输入大写字母与下划线"));
} else {
callback();
}
} else {
callback();
}
};
// 只能输入中文
export const tableNameValidUpper = (rule, val, callback) => {
if (val != null && val != "") {
const value = val.replace(/^\s*|\s*$/g, "");
if (!/^[\u4E00-\u9FA5]*$/.test(value)) {
callback(new Error("只能输入中文"));
} else {
callback();
}
} else {
callback();
}
};
// 不允许输入特殊字符
export const remarkValidUpper = (rule, val, callback) => {
if (val != null && val != "") {
const value = val.replace(/^\s*|\s*$/g, "");
if (!/^[0-9a-zA-Z\u4E00-\u9FA5]*$/.test(value)) {
callback(new Error("不允许输入特殊字符"));
} else {
callback();
}
} else {
callback();
}
};
// 只能输入数字
export const numberValidUpper = (rule, val, callback) => {
console.log("val====>", val);
if (val != null && val != "") {
if (Number(val)) {
if (!/^\d+$/.test(val)) {
callback(new Error("只能输入数字"));
} else {
callback();
}
} else {
callback();
}
}
};
// 只能输入数字和forever
export const foreverValidUpper = (rule, val, callback) => {
const value = val.replace(/^\s*|\s*$/g, "");
if (val != null && val != "") {
if (value === "forever") {
callback();
} else {
if (!/^\d+$/.test(value)) {
callback(new Error("只能输入forever和数字"));
} else {
callback();
}
}
} else {
callback();
}
};
// 只能输入数字和English
export const englishValidUpper = (rule, val, callback) => {
if (val != null && val != "") {
if (!/^[A-Za-z0-9]+$/.test(val)) {
callback(new Error("只能输入数字和英文"));
} else {
callback();
}
} else {
callback();
}
};
// 只能输入数字,且位数只能在16个
export const numberValidUppers = (rule, val, callback) => {
console.log("val====>", val);
if (val != null && val != "") {
if (Number(val)) {
if (!/^\d{0,16}$/g.test(val)) {
callback(new Error("只能输入数字"));
} else {
callback();
}
} else {
callback();
}
}
};
9.时间过滤
/**
* Created by PanJiaChen on 16/11/18.
*/
/**
* Parse the time to string
* @param {(Object|string|number)} time
* @param {string} cFormat
* @returns {string | null}
*/
export function parseTime (time, cFormat) {
if (arguments.length === 0 || !time) {
return null
}
const format = cFormat || '{y}-{m}-{d} {h}:{i}:{s}'
let date
if (typeof time === 'object') {
date = time
} else {
if ((typeof time === 'string')) {
if ((/^[0-9]+$/.test(time))) {
// support "1548221490638"
time = parseInt(time)
} else {
// support safari
// https://stackoverflow.com/questions/4310953/invalid-date-in-safari
time = time.replace(new RegExp(/-/gm), '/')
}
}
if ((typeof time === 'number') && (time.toString().length === 10)) {
time = time * 1000
}
date = new Date(time)
}
const formatObj = {
y: date.getFullYear(),
m: date.getMonth() + 1,
d: date.getDate(),
h: date.getHours(),
i: date.getMinutes(),
s: date.getSeconds(),
a: date.getDay()
}
const time_str = format.replace(/{([ymdhisa])+}/g, (result, key) => {
const value = formatObj[key]
// Note: getDay() returns 0 on Sunday
if (key === 'a') { return ['日', '一', '二', '三', '四', '五', '六'][value ] }
return value.toString().padStart(2, '0')
})
return time_str
}
/**
* @param {number} time
* @param {string} option
* @returns {string}
*/
export function formatTime (time, option) {
if (('' + time).length === 10) {
time = parseInt(time) * 1000
} else {
time = +time
}
const d = new Date(time)
const now = Date.now()
const diff = (now - d) / 1000
if (diff < 30) {
return '刚刚'
} else if (diff < 3600) {
// less 1 hour
return Math.ceil(diff / 60) + '分钟前'
} else if (diff < 3600 * 24) {
return Math.ceil(diff / 3600) + '小时前'
} else if (diff < 3600 * 24 * 2) {
return '1天前'
}
if (option) {
return parseTime(time, option)
} else {
return (
d.getMonth() +
1 +
'月' +
d.getDate() +
'日' +
d.getHours() +
'时' +
d.getMinutes() +
'分'
)
}
}
/**
* @param {string} url
* @returns {Object}
*/
export function param2Obj (url) {
const search = decodeURIComponent(url.split('?')[1]).replace(/\+/g, ' ')
if (!search) {
return {}
}
const obj = {}
const searchArr = search.split('&')
searchArr.forEach(v => {
const index = v.indexOf('=')
if (index !== -1) {
const name = v.substring(0, index)
const val = v.substring(index + 1, v.length)
obj[name] = val
}
})
return obj
}
// 查找pid是指定参数的子元素
export function translateData (list, pid) {
const treeData = []
// 自己设计算法做转换
list.forEach(item => {
if (item.pid === pid) {
// 查找该元素的子元素,如果找到子元素后,以children属性方式添加到对象里面
const childList = translateData(list, item.id)
if (childList && childList.length > 0) {
// 查找了子元素
item.children = childList
}
treeData.push(item)
}
})
return treeData
}
10.vue中全局使用图片
<img :src="require(`@/assets/image/${matchFileSuffixType(Appendix.fileType)}.png`)" alt="" />
//第二种
<img :src="require('@/assets/image/pgy1.jpg')" />
11.vue中将后端返回的二进制图片流转换为base64展示在页面中
有时从后端请求获取到的图片为二进制的流文件,需要展示在页面中,可以将二进制转换为base64即可。这里是在vue项目用的axios请求。
deviceDoDownload().then(d => {
console.log(d);
if (d.data) {//如果返回的有值,则使用返回的底图
// 先将二进制的流转为blob,注意后面type一定要设置为图片格式
let blob = new Blob([d.data], { type: "image/jpg" });
var Fr = new FileReader();
Fr.readAsDataURL(blob);
Fr.onload = (event) => {
//这个就是转换为的base64图片地址
this.staticMap = event.target.result;
};
}
})
当然这样写最后转的base64是有问题的,在页面中显示不出来,还需要在请求接口中添加responseType表明数据类型
export const deviceDoDownload = () => {
return axios({
url: '/aaaaaa',
method: 'post',
responseType: "blob" // 表明返回服务器返回的数据类型
})
}
然后在看后端接口返回的数据
12.配置eslint后运行项目有如下警告(换行格式问题)
执行以下命令(可以自动修复这些问题):
npm run lint --fix 修复
11.base64前台展示页面
<img :src=" 'data:image/png;base64,' + base64的地址 ">
例如: <img :src="'data:image/png;base64,' + item.icon" @click="toDetail(item.appId)" />
将后台给返回的base64直接拼接的img的:src上
前面加固定的前缀 data:image/png;base64,
<head>
<meta charset="utf-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width,initial-scale=1.0" />
<link rel="icon" href="<%= BASE_URL %>favicon.ico" />
<!-- <link rel="stylesheet" href="http://39.99.202.33:8081/cdn/sg-ui/0.0.10-beta/theme-chalk/index.css" /> -->
<!-- 7879注释,8081的解开 -->
<link rel="stylesheet" href="http://192.168.15.54:7879/cdn/sg-ui/0.0.12-beta/theme-chalk/index.css" />
<link rel="stylesheet" href="//at.alicdn.com/t/font_3020864_gpou3s6r4mc.css" />
<title>
<%= htmlWebpackPlugin.options.title %>
</title>
</head>
<body>
<noscript>
<strong>We're sorry but <%= htmlWebpackPlugin.options.title %> doesn't work properly without JavaScript enabled.
Please
enable it to continue.</strong>
</noscript>
<div id="app"></div>
<!-- built files will be auto injected -->
<!-- <script src="http://39.99.202.33:8081/cdn/vue/2.6.11/vue.js" charset="utf-8"></script>
<script src="http://39.99.202.33:8081/cdn/vuex/3.4.0/vuex.js" charset="utf-8"></script>
<script src="http://39.99.202.33:8081/cdn/vue-router/3.2.0/vue-router.js" charset="utf-8"></script>
<script src="http://39.99.202.33:8081/cdn/axios/0.21.1/axios.js" charset="utf-8"></script>
<script src="http://39.99.202.33:8081/cdn/sg-ui/0.0.12-beta/index.js" charset="utf-8"></script> -->
<script src="http://192.168.15.54:7879/cdn/vue/2.6.11/vue.min.js" charset="utf-8"></script>
<script src="http://192.168.15.54:7879/cdn/vuex/3.4.0/vuex.min.js" charset="utf-8"></script>
<script src="http://192.168.15.54:7879/cdn/vue-router/3.2.0/vue-router.min.js" charset="utf-8"></script>
<script src="http://192.168.15.54:7879/cdn/axios/0.21.1/axios.min.js" charset="utf-8"></script>
<script src="http://192.168.15.54:7879/cdn/sg-ui/0.0.12-beta/index.js" charset="utf-8"></script>
</body>
12.element合并单元格(根据id)
<template>
<div>
<sg-table :data="tableData6" :span-method="objectSpanMethod" border style="width: 100%; margin-top: 20px">
<sg-table-column prop="id" label="ID" width="180"> </sg-table-column>
<sg-table-column prop="name" label="姓名"> </sg-table-column>
<sg-table-column prop="amount1" label="数值 1(元)"> </sg-table-column>
<sg-table-column prop="amount2" label="数值 2(元)"> </sg-table-column>
<sg-table-column prop="amount3" label="数值 3(元)"> </sg-table-column>
</sg-table>
</div>
</template>
<script>
export default {
data() {
return {
spanArr: [],
tableData6: [
{
id: "1",
name: "王小虎",
amount1: "234",
amount2: "3.2",
amount3: 10,
},
{
id: "1",
name: "王小虎",
amount1: "165",
amount2: "4.43",
amount3: 12,
},
{
id: "2",
name: "王小虎",
amount1: "324",
amount2: "1.9",
amount3: 9,
},
{
id: "2",
name: "王小虎",
amount1: "621",
amount2: "2.2",
amount3: 17,
},
{
id: "2",
name: "王小虎",
amount1: "539",
amount2: "4.1",
amount3: 15,
},
{
id: "2",
name: "王小虎",
amount1: "539",
amount2: "4.1",
amount3: 15,
},
{
id: "3",
name: "王小虎",
amount1: "539",
amount2: "4.1",
amount3: 15,
},
],
};
},
mounted: function () {
// 组件创建完后获取数据,
// 此时 data 已经被 observed 了
this.getSpanArr();
},
methods: {
getSpanArr() {
for (var i = 0; i < this.tableData6.length; i++) {
if (i === 0) {
this.spanArr.push(1);
this.pos = 0;
} else {
// 判断当前元素与上一个元素是否相同
if (this.tableData6[i].id === this.tableData6[i - 1].id) {
this.spanArr[this.pos] += 1;
this.spanArr.push(0);
} else {
this.spanArr.push(1);
this.pos = i;
}
}
}
},
objectSpanMethod({ rowIndex, columnIndex }) {
if (columnIndex === 0) {
const _row = this.spanArr[rowIndex];
const _col = _row > 0 ? 1 : 0;
return {
rowspan: _row,
colspan: _col,
};
}
},
},
};
</script>