二叉搜索树 | ||
---|---|---|
前缀树 | ||
二叉树
- 翻转等价二叉树
二叉搜索树
×95.96.不同的二叉搜索树-
(1)动态规划 叛徒
```python class Solution: def numTrees(self, n: int) -> int: store = [1,1] #f(0),f(1) if n <= 1:
for m in range(2,n+1):return store[n]
return store[n]s = m-1
count = 0
for i in range(m):
count += store[i]*store[s-i]
store.append(count)
<a name="dCVrA"></a>
### (2)递归
<br />这道理搞清代码的逻辑关系最重要<br />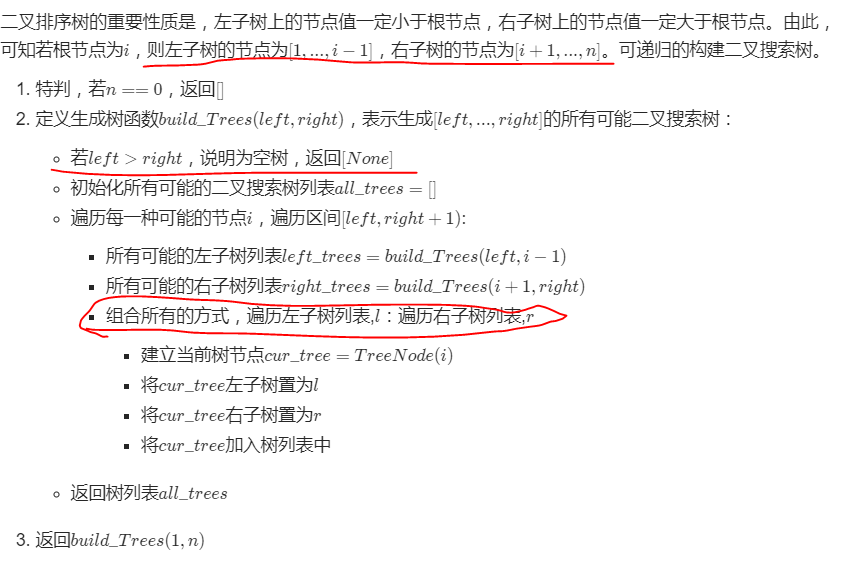
```python
class Solution:
def generateTrees(self, n: int) -> List[TreeNode]:
if(n==0):
return []
def build_Trees(left,right):
all_trees=[]
if(left>right):
return [None]
for i in range(left,right+1):
left_trees=build_Trees(left,i-1)
right_trees=build_Trees(i+1,right)
for l in left_trees:
for r in right_trees:
cur_tree=TreeNode(i)
cur_tree.left=l
cur_tree.right=r
all_trees.append(cur_tree)
return all_trees
res=build_Trees(1,n)
return res
√98. 验证二叉搜索树
可以利用中序遍历结果来进行验证 - 783二叉搜索树节点最小距离
class Solution:
def isValidBST(self, root: TreeNode) -> bool:
self.vals = []
self.inOrder(root)
for i in range(len(self.vals)-1):
if self.vals[i+1]<=self.vals[i]:
return False
return True
def inOrder(self, root):
if not root:
return
self.inOrder(root.left)
self.vals.append(root.val) # 中序遍历 得到的有序序列
self.inOrder(root.right)
×99. 恢复二叉搜索树(困难)
class Solution:
def recoverTree(self, root: TreeNode) -> None:
self.firstNode = None # 双指针
self.secondNode = None
self.preNode = TreeNode(float("-inf")) # 负无穷
def in_order(root):
if not root: # 终止条件
return
in_order(root.left)
# 中序遍历
if self.firstNode == None and self.preNode.val >= root.val:
self.firstNode = self.preNode
if self.firstNode and self.preNode.val >= root.val:
self.secondNode = root
self.preNode = root
in_order(root.right)
in_order(root)
self.firstNode.val, self.secondNode.val = self.secondNode.val, self.firstNode.val
109. 有序链表转换二叉搜索树
173. 二叉搜索树迭代器
235. 二叉搜索树的最近公共祖先
√783. 二叉搜索树节点最小距离
给你一个二叉搜索树的根节点 root
,返回 树中任意两不同节点值之间的最小差值 。
「二叉搜索树(BST)的中序遍历是有序的」
class Solution(object):
def minDiffInBST(self, root):
self.vals = []
self.inOrder(root)
return min([self.vals[i + 1] - self.vals[i] for i in range(len(self.vals) - 1)])
def inOrder(self, root):
if not root:
return
self.inOrder(root.left)
self.vals.append(root.val) # 中序遍历 得到的有序序列
self.inOrder(root.right)
前缀树
×208. 实现 Trie (前缀树)
class Trie:
def __init__(self):
self.d = {}
def insert(self, word: str) -> None:
t = self.d
for c in word:
if c not in t:
t[c] = {}
t = t[c] #字典迭代
t['end'] = True #标记单词终点
def search(self, word: str) -> bool:
t = self.d # 浅复制,b=a,接着对b所有的操作都会在a上也操作一次,如果不想这样,想让b,a没有关联就得import copy.deepcopy
for c in word:
if c not in t: # 如果字典里没有这个字母,那一定搜索不到
return False
t = t[c] # 如果搜索到这个字母,接着后面搜
return 'end' in t
def startsWith(self, prefix: str) -> bool:
t = self.d
print(t)
for c in prefix:
if c not in t:
return False
t = t[c]
return True
# Your Trie object will be instantiated and called as such:
# obj = Trie()
# obj.insert(word)
# param_2 = obj.search(word)
# param_3 = obj.startsWith(prefix)