对象
创建 构造函数 this
var obj = {} //对象字面量
var obj = new Object()
Array() Number()
//自定义构造函数
function Person() {}
var p1 = new Person()
console.log(p1) //Person
function Car() {
this.name = 'BMW'
this.height = 1400
this.weight = 1000
this.health = 100
this.run = function() {
this.health--
}
}
var c1 = new Car()
var c2 = new Car()
console.log(c1) //Car {name: 'BMW', height: 1400, weight: 1000}
c2.name = 'Merz'
console.log(c2) //Car {name: 'BMW', height: 1400, weight: 1000}
function Student(name, age, sex) {
//var this = {} StudentAO:{this:{}}
this.name = name
this.age = age
this.sex = sex
// return this
}
var s1 = new Student('xm', 18, '男')
console.log(s1) //Student {name: 'xm', age: 18, sex: '男'}
- .在函数体最前面隐式的加上 var this = {}
- .执行 this.xxx = xxx;
- 隐式的返回 return this
- new Student(),才发生上面3步
如果返回原始值,对于new无效,引用值会覆盖 return this
function Student(name, age, sex) {
this.name = name
this.age = age
this.sex = sex
return 123 //如果返回原始值,对于new无效,引用值会覆盖 return this
}
var s1 = new Student('xm', 18, '男')
console.log(s1) //Student {name: 'xm', age: 18, sex: '男'}
默认return this,有时会形成闭包
function Person() {
var a = 0
this.name = 'xiaoming'
this.age = 18
this.say = function() {
a++
console.log(a)
}
}
var p1 = new Person()
p1.say() //1
p1.say() //2
var p2 = new Person()
p2.say() //1
//PersonAO {
a: 0,
this: {
name: 'xiaoming',
age: 18,
say: function() {
a++
console.log(a)
}
}
}
//所以say里面可以访问a
//对象的方法不能直接访问对象属性,估计是因为对象没有AO环境
属性
- 对象的所有键名都是字符串,所以加不加引号都可以
- 对象的每一个键名又称为“属性”(property),它的“键值”可以是任何数据类型
- 不同的变量名指向同一个对象,那么它们都是这个对象的引用
var o1 = {}; //对象字面量
var o2 = o1;
o1.a = 1;
o2.a // 1
o2.b = 2;
o1.b // 2
var p = {
health: 100,
smoke: function() {
this.health-- //直接访问health会报错
},
drink: function() {
this.health++
},
}
p.smoke()
console.log(p.health) //99
p.drink()
console.log(p.health)//100
属性的操作
属性的增加
直接点出来就行
p.newaa = ‘aa’
属性的读取
- 读取对象的属性,有两种方法,一种是使用点运算符,还有一种是使用方括号运算符 ```javascript var obj = { p: ‘Hello World’ };
obj.p // “Hello World” obj[‘p’] // “Hello World”
obj.o //undefined 没有定义的属性直接访问会报错
- 如果使用方括号运算符,键名必须放在引号里面,否则会被当作变量处理
- 方括号运算符内部还可以使用表达式 `obj['hello' + ' world']` `obj[3 + 3]`
- 数字键可以不加引号,因为会自动转成字符串
```javascript
var obj = {
0.7: 'Hello World'
};
obj['0.7'] // "Hello World"
obj[0.7] // "Hello World"
- 数值键名不能使用点运算符 ```javascript var obj = { 123: ‘hello world’ };
obj.123 // 报错 obj[123] // “hello world”
<a name="RZwRf"></a>
### 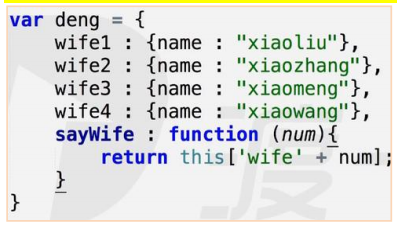
<a name="Hp6fz"></a>
###
<a name="a08f772d"></a>
### 属性名的查看
```javascript
var obj = {
key1: 1,
key2: 2
};
Object.keys(obj);
// ['key1', 'key2']
属性的删除
var obj = { p: 1 };
Object.keys(obj) // ["p"]
delete obj.p // true
obj.p // undefined
Object.keys(obj) // []
属性是否存在
hasOwnProperty 拿自己的属性,但不拿系统原型上的属性
in 不管自己还是父亲的,都属于
var obj = { p: 1 };
'p' in obj // true
'toString' in obj // true
var obj = {};
if ('toString' in obj) {
console.log(obj.hasOwnProperty('toString')) // false
}
var obj = {
lastName: 'kun',
}
obj['__proto__'] = {
name: 'deng',
}
Object.prototype.abc = 123
console.log('lastName' in obj)
console.log('toString' in obj) //true
console.log('name' in obj) //true
console.log('abc' in obj) //true
console.log(obj.hasOwnProperty('lastName')) //true
console.log(obj.hasOwnProperty('toString')) //false
console.log(obj.hasOwnProperty('name')) //false
console.log(obj.hasOwnProperty('abc')) //false
属性的遍历
var person = { name: '老张' }
for (var key in person) {
if (person.hasOwnProperty(key)) {
console.log(typeof key)
console.log(key + ' : ' + person[key])
//person.key 是不行的 person.key-------->person['key']
}
}