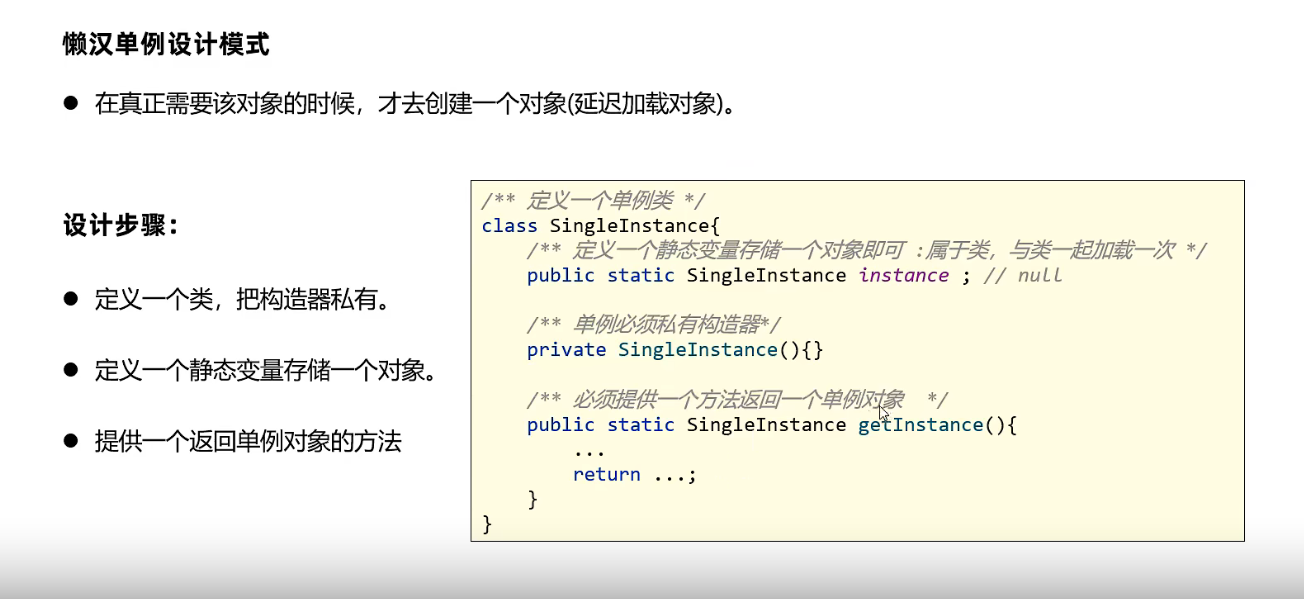
package com.itheima.d4_static_singleinstance;
public class Test2 {
public static void main(String[] args) {
// 目标:掌握懒汉单例的设计。理解其思想
// SingleInstance2 s1 = SingleInstance2.instance;// 默认值为null
// SingleInstance2 s2 = SingleInstance2.instance; // null
// System.out.println(s1);
// System.out.println(s2);
// 这个时候已经产生了对象
System.out.println(SingleInstance2.getInstance());
// 将创建的对象用变量存起来
SingleInstance2 s1 = SingleInstance2.getInstance();
SingleInstance2 s2 = SingleInstance2.getInstance();
System.out.println(s1 == s2); // 为true代表实现了懒汉单例
}
}
package com.itheima.d4_static_singleinstance;
/**
* 懒汉单例
*/
public class SingleInstance2 {
/**
* 2.定义一个静态的成员负责存储一个对象
* 只加载一次,只有一份
* 注意:最好将成员私有化,因为一开始的默认值为null,被别人调用一直为nll
* 防止被别人调用,就将他私有化
*/
// 如果像这种变量类型为:类名 的话,用来存储的都是对象
private static SingleInstance2 instance; // 默认值为:null
/**
* 3. 提供一个方法,对外返回单例对象
*/
public static SingleInstance2 getInstance(){
if (instance == null){
// 第一次来拿对象:此时需要创建对象
instance = new SingleInstance2();
}
// 如果有对象了,就返回对象的地址给他
return instance;
}
/**
* 1. 私有化构造器(要想实现单例模式必须私有化构造器,不能被别人创建对象)
*/
private SingleInstance2(){
}
}