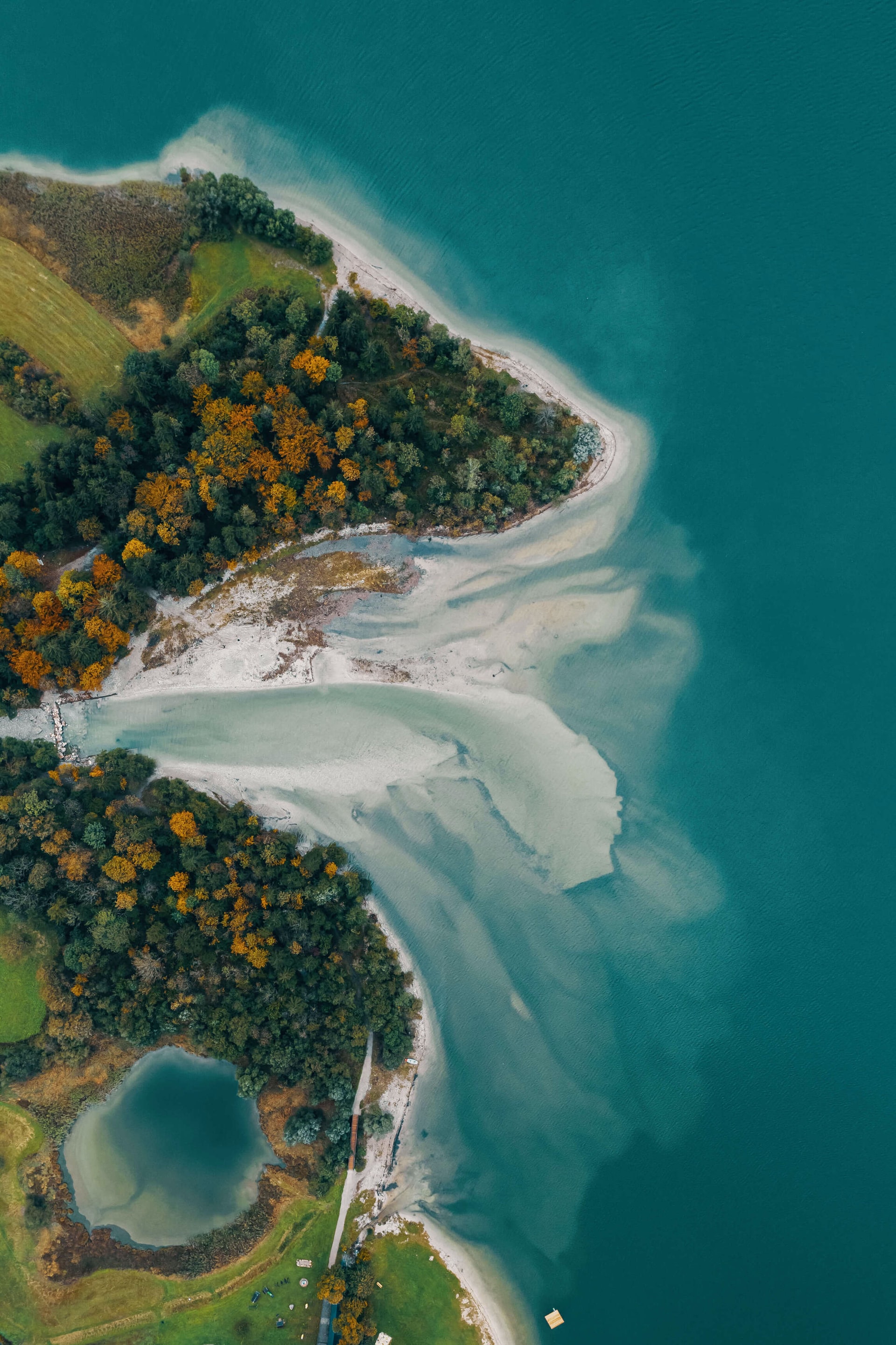
二叉树
代码
/**
* Definition for a binary tree node.
* function TreeNode(val, left, right) {
* this.val = (val===undefined ? 0 : val)
* this.left = (left===undefined ? null : left)
* this.right = (right===undefined ? null : right)
* }
*/
/**
* @param {TreeNode} root
* @return {number[]}
*/
const inOrder = (root, res) => {
if (root === null) {
return null;
}
inOrder(root.left, res);
res.push(root.val);
inOrder(root.right, res);
}
var inorderTraversal = function (root) {
const res = []
inOrder(root, res);
return res;
};