一、拼接字符串并用逗号分隔
public class StuTest {
public static void main(String[] args) {
Stu stu1 = new Stu(1, "zhangsan");
Stu stu2 = new Stu(2, "lisi");
List<Stu> list = new ArrayList<>();
list.add(stu1);
list.add(stu2);
String result = list.stream().map(Stu::getName).collect(Collectors.joining(","));
System.out.println(result.toString());
}
}
二、在sql中进行判断逻辑
<sql id="sql-count-where">
<choose>
<when test="param.basicIdList == null">
and 1 = 1
</when>
<when test="param.basicIdList != null and param.basicIdList.size > 0">
and aci.basic_id in
<foreach collection="param.basicIdList" index="index" item="item" open="(" close=")" separator=",">
#{item}
</foreach>
</when>
<otherwise>
and 1 = -1
</otherwise>
</choose>
</sql>
三、使用正则切割字符串
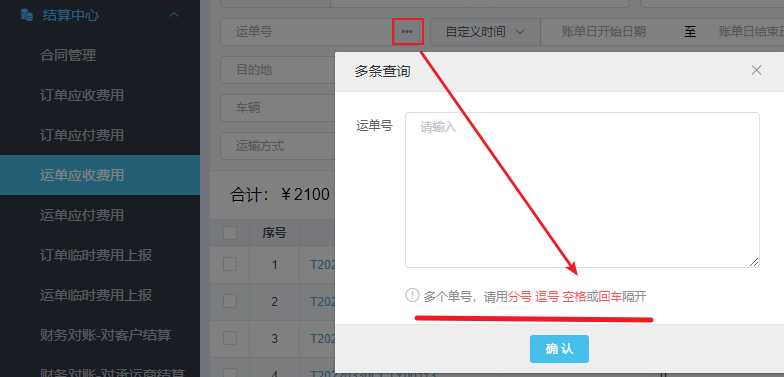
public static final String ORDER_NO_SPLIT_REGEX = ",|,|;|;| |\t|\n|\r";
private List<String> getorderOrWaybillNoList(String orderNoOrWaybillNo) {
if (StringUtils.isBlank(orderNoOrWaybillNo)) {
return Lists.newArrayList();
}
String[] split = orderNoOrWaybillNo.split(ORDER_NO_SPLIT_REGEX);
List<String> orderNoOrWaybillNos = com.google.common.collect.Lists.newArrayList(split);
List<String> orderNoOrWaybillNoList = orderNoOrWaybillNos.stream().filter(o -> StringUtils.isNotBlank(o)).distinct().collect(Collectors.toList());
if (CollectionUtils.isEmpty(orderNoOrWaybillNoList)) {
logger.info("切出的单号集合为空", JSON.toJSONString(split));
}
return orderNoOrWaybillNoList;
}
四、条件语句中的循环模糊查询
<if test="param.businessType == 2 and param.waybillNoList != null and param.waybillNoList.size>0">
and
(
<foreach collection="param.waybillNoList" separator="or" item="waybillNo" index="index">
aci.business_no like concat('%', #{waybillNo},'%')
</foreach>
)
</if>