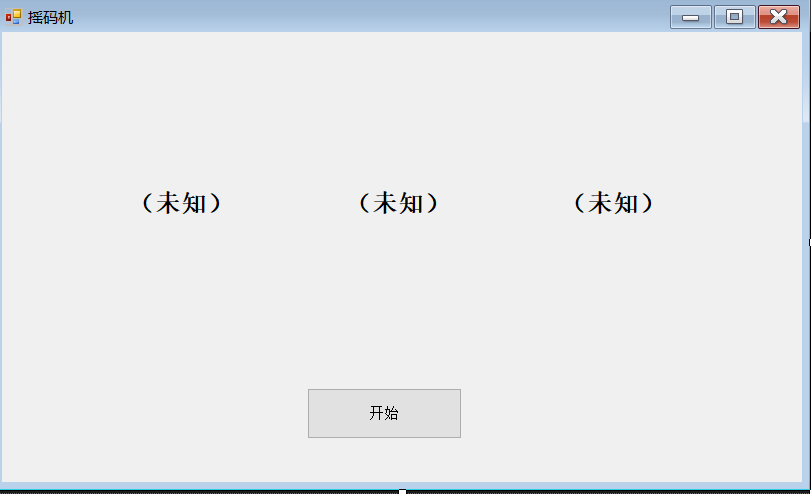
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace _112_摇奖机_多线程
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
Thread th;
bool b = true;
private void button1_Click(object sender, EventArgs e)
{
if (button1.Text == "开始")
{
//Thread th = new Thread(PlayGame);
th = new Thread(PlayGame);
th.IsBackground = true;
b = true;
th.Start();
button1.Text = "停止";
}
else
{
b = false;
button1.Text = "开始";
}
}
private void PlayGame()
{
Random r = new Random();
while (b)
{
label1.Text = r.Next(0, 10).ToString();
label2.Text = r.Next(0, 10).ToString();
label3.Text = r.Next(0, 10).ToString();
}
}
private void Form1_Load(object sender, EventArgs e)
{
//取消不可跨线程访问
Control.CheckForIllegalCrossThreadCalls = false;
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
//当你点击关闭窗口的时候,判断线程是否为null
if (th != null)
{
//th.Abort();//线程被Abort后就不能被Start了
th = null;
}
}
}
}