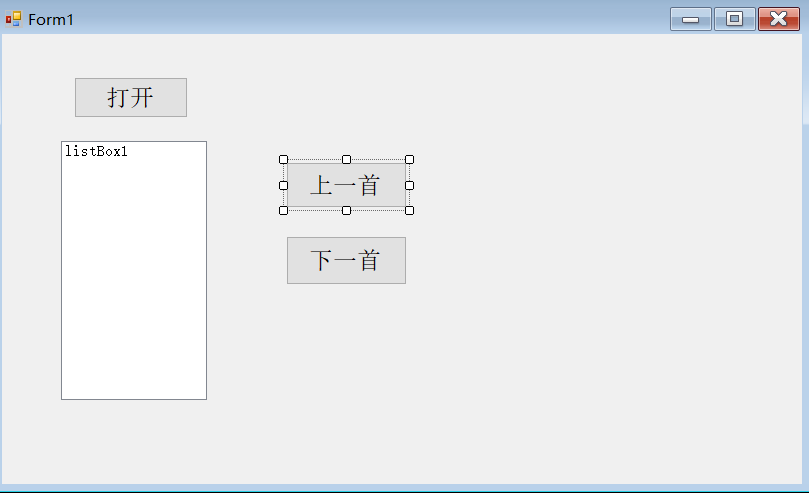
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Media;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace _121_播放音乐上一曲下一曲
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
List<string> listSongs = new List<string>();
//Dictionary<string, string> keyValuePairs = new Dictionary<string, string>();
private void button1_Click(object sender, EventArgs e)
{
OpenFileDialog ofd = new OpenFileDialog();
ofd.Title = "请选择音乐文件";
ofd.InitialDirectory = @"C:\Users\46124\Desktop\.wav音乐";
ofd.Multiselect = true;
ofd.Filter = "媒体文件|*.wav|所有文件|*.*";
ofd.ShowDialog();
//获得我们在文件夹中选择所有文件的全路径
string[] path = ofd.FileNames;
foreach (var item in path)
{
listBox1.Items.Add(Path.GetFileNameWithoutExtension(item));
//将音乐文件存储到泛型集合中
listSongs.Add(item);
//keyValuePairs.Add(Path.GetFileNameWithoutExtension(item), item);
}
}
SoundPlayer sp = new SoundPlayer();
/// <summary>
/// 实现双击播放音乐
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void listBox1_DoubleClick(object sender, EventArgs e)
{
sp.SoundLocation = listSongs[listBox1.SelectedIndex];
//sp.SoundLocation = keyValuePairs[listBox1.Items.ToString()];
sp.Play();
}
/// <summary>
/// 点击下一曲
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button3_Click(object sender, EventArgs e)
{
//获得当前选中歌曲的索引
int index = listBox1.SelectedIndex;
index++;
if (index == listBox1.Items.Count)
{
index = 0;
}
//将改变后的索引重新赋值给我当前项中的索引,实现蓝条移动
listBox1.SelectedIndex = index;
sp.SoundLocation = listSongs[index];
sp.Play();
}
/// <summary>
/// 点击上一曲
/// </summary>
/// <param name="sender"></param>
/// <param name="e"></param>
private void button2_Click(object sender, EventArgs e)
{
//获得当前选中歌曲的索引
int index = listBox1.SelectedIndex;
index--;
if (index < 0)
{
index = listBox1.Items.Count - 1;
}
//将改变后的索引重新赋值给我当前项中的索引,实现蓝条移动
listBox1.SelectedIndex = index;
sp.SoundLocation = listSongs[index];
sp.Play();
}
}
}