基本属性
const PopupMenuButton({
Key key,
@required this.itemBuilder, // 菜单中的每一项
this.initialValue, // 选中的项
this.onSelected, // 点击的时候触发
this.onCanceled, // 取消时候触发
this.tooltip, // 提示信息
this.elevation,
this.padding = const EdgeInsets.all(8.0),
this.child, // 按钮 【字】
this.icon, //
this.offset = Offset.zero,
this.enabled = true,
this.shape, // 外观
this.color, // 菜单背景色
this.captureInheritedThemes = true,
}) : assert(itemBuilder != null),
assert(offset != null),
assert(enabled != null),
assert(captureInheritedThemes != null),
assert(!(child != null && icon != null),
'You can only pass [child] or [icon], not both.'),
super(key: key);
基本用法
默认基本样式
String _initaiaValue = "索隆";
PopupMenuButton(
itemBuilder: (context){
return <PopupMenuEntry <String>> [
PopupMenuItem<String>(
value: '托尼', // 查找的内容
textStyle: TextStyle(color: Colors.yellow[700]), // 设置字体属性
height: 30.0, // 设置文本高度
child: Text('乔巴'), // 展示的内容
),
PopupMenuItem<String>(
value: '索隆',
child: Text('索隆'),
),
PopupMenuItem<String>(
value: '布鲁克',
child: Text('布鲁克'),
),
PopupMenuItem<String>(
value: '娜美',
child: Text('娜美'),
),
PopupMenuItem<String>(
value: '乌索普',
child: Text('乌索普'),
),
];
},
initialValue : _initaiaValue, // 默认选择项 是按 value来查找的
onSelected : (e){ // 点击的时候触发
setState(() {
_initaiaValue = e;
});
print("this is onSelected and $e");
},
onCanceled : (){ // 取消的时候触发。
print("this is onCanceled!");
},
)
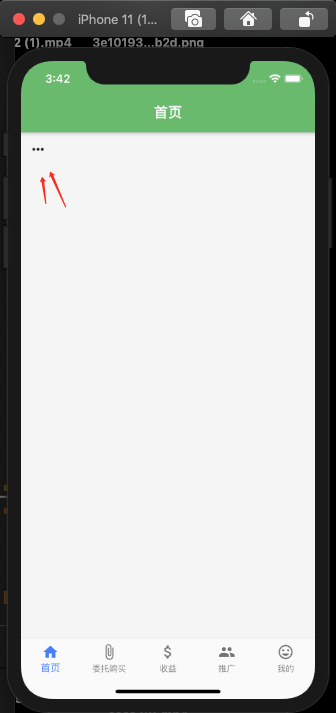
添加提示信息
PopupMenuButton(
itemBuilder: (context){
return <PopupMenuEntry <String>> [
PopupMenuItem<String>(
value: '托尼', // 查找的内容
textStyle: TextStyle(color: Colors.yellow[700]), // 设置字体属性
height: 30.0, // 设置文本高度
child: Text('乔巴'), // 展示的内容
),
PopupMenuItem<String>(
value: '索隆',
child: Text('索隆'),
),
PopupMenuItem<String>(
value: '布鲁克',
child: Text('布鲁克'),
),
PopupMenuItem<String>(
value: '娜美',
child: Text('娜美'),
),
PopupMenuItem<String>(
value: '乌索普',
child: Text('乌索普'),
),
];
},
initialValue : _initaiaValue, // 默认选择项 是按 value来查找的
onSelected : (e){ // 点击的时候触发
setState(() {
_initaiaValue = e;
});
print("this is onSelected and $e");
},
onCanceled : (){ // 取消的时候触发。
print("this is onCanceled!");
},
tooltip : "按着我不动?想??", // 提示信息。
)
更换默认【 … 】菜单图标
PopupMenuButton(
itemBuilder: (context){
return <PopupMenuEntry <String>> [
PopupMenuItem<String>(
value: '托尼', // 查找的内容
textStyle: TextStyle(color: Colors.yellow[700]), // 设置字体属性
height: 30.0, // 设置文本高度
child: Text('乔巴'), // 展示的内容
),
PopupMenuItem<String>(
value: '索隆',
child: Text('索隆'),
),
PopupMenuItem<String>(
value: '布鲁克',
child: Text('布鲁克'),
),
PopupMenuItem<String>(
value: '娜美',
child: Text('娜美'),
),
PopupMenuItem<String>(
value: '乌索普',
child: Text('乌索普'),
),
];
},
initialValue : _initaiaValue, // 默认选择项 是按 value来查找的
onSelected : (e){ // 点击的时候触发
setState(() {
_initaiaValue = e;
});
print("this is onSelected and $e");
},
onCanceled : (){ // 取消的时候触发。
print("this is onCanceled!");
},
tooltip : "aaa", // 提示信息。
elevation: 50.0,
icon : Icon(
IconData(
0xe660,
fontFamily : 'wz',
matchTextDirection: true,
),
size: 44.0,
),
)
设置外观
PopupMenuButton(
itemBuilder: (context){
return <PopupMenuEntry <String>> [
PopupMenuItem<String>(
value: '托尼', // 查找的内容
textStyle: TextStyle(color: Colors.yellow[700]), // 设置字体属性
height: 30.0, // 设置文本高度
child: Text('乔巴'), // 展示的内容
),
PopupMenuItem<String>(
value: '索隆',
child: Text('索隆'),
),
PopupMenuItem<String>(
value: '布鲁克',
child: Text('布鲁克'),
),
PopupMenuItem<String>(
value: '娜美',
child: Text('娜美'),
),
PopupMenuItem<String>(
value: '乌索普',
child: Text('乌索普'),
),
];
},
initialValue : _initaiaValue, // 默认选择项 是按 value来查找的
onSelected : (e){ // 点击的时候触发
setState(() {
_initaiaValue = e;
});
print("this is onSelected and $e");
},
onCanceled : (){ // 取消的时候触发。
print("this is onCanceled!");
},
tooltip : "aaa", // 提示信息。
elevation: 50.0,
icon : Icon(
IconData(
0xe660,
fontFamily : 'wz',
matchTextDirection: true,
),
size: 44.0,
),
shape: OutlineInputBorder( // 外观
borderSide: BorderSide(color: Colors.yellow[700]),
borderRadius: BorderRadius.circular(10),
),
color: Colors.green[100], // 列表的背景色
// child: Text(""),
)
设置背景色
PopupMenuButton(
itemBuilder: (context){
return <PopupMenuEntry <String>> [
PopupMenuItem<String>(
value: '托尼', // 查找的内容
textStyle: TextStyle(color: Colors.yellow[700]), // 设置字体属性
height: 30.0, // 设置文本高度
child: Text('乔巴'), // 展示的内容
),
PopupMenuItem<String>(
value: '索隆',
child: Text('索隆'),
),
PopupMenuItem<String>(
value: '布鲁克',
child: Text('布鲁克'),
),
PopupMenuItem<String>(
value: '娜美',
child: Text('娜美'),
),
PopupMenuItem<String>(
value: '乌索普',
child: Text('乌索普'),
),
];
},
initialValue : _initaiaValue, // 默认选择项 是按 value来查找的
onSelected : (e){ // 点击的时候触发
setState(() {
_initaiaValue = e;
});
print("this is onSelected and $e");
},
onCanceled : (){ // 取消的时候触发。
print("this is onCanceled!");
},
tooltip : "aaa", // 提示信息。
elevation: 50.0,
icon : Icon(
IconData(
0xe660,
fontFamily : 'wz',
matchTextDirection: true,
),
size: 44.0,
),
shape: OutlineInputBorder( // 外观
borderSide: BorderSide(color: Colors.yellow[700]),
borderRadius: BorderRadius.circular(10),
),
color: Colors.green[100], // 列表的背景色
// child: Text(""),
)
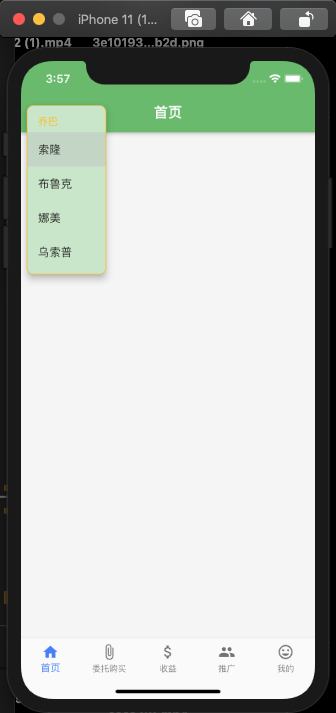