Checkbox
基本属性
const Checkbox({
Key key,
@required this.value, // true 选中,false非选中
this.tristate = false, // 如果为真,复选框的[value]可以为真、假或空。复选框的值为空时显示破折号。当一个三态复选框([tristate]为真)被点击时,如果当前值为假,它的[onChanged]回调将被应用到true,如果值为真,将被应用到null,如果值为空,
@required this.onChanged, // 点击事件
this.activeColor, // 为激活状态下颜色,是矩形区域内的颜色
this.checkColor, // 是选中后“对勾”的颜色,用法如下:
this.focusColor,
this.hoverColor,
this.materialTapTargetSize,
this.visualDensity,
this.focusNode,
this.autofocus = false,
}) : assert(tristate != null),
assert(tristate || value != null),
assert(autofocus != null),
super(key: key);
基本用法
Checkbox(
value: _checkbox,
tristate: true,
activeColor: Colors.yellow[700],
checkColor: Colors.black,
onChanged: (e){
print("$e, $_checkbox");
setState(() {
_checkbox = e;
});
},
),
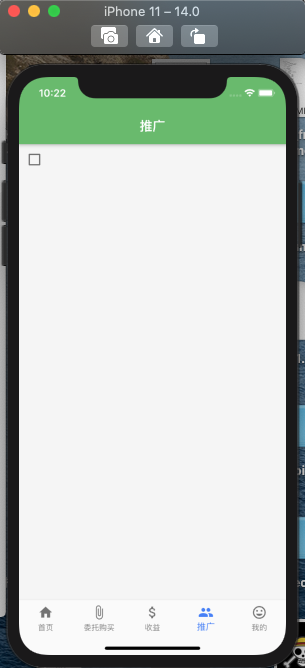
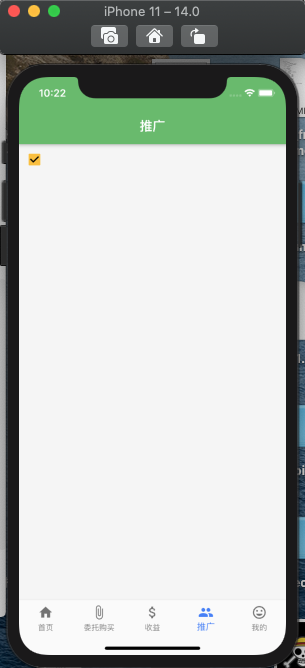
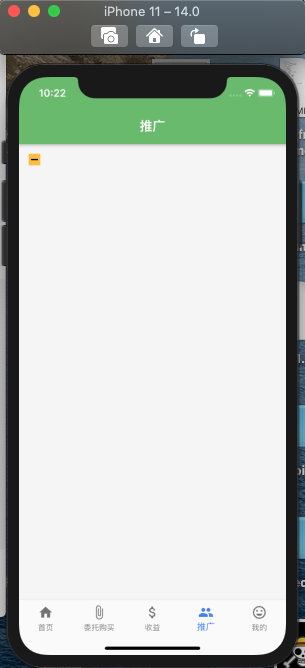
CheckboxList
基本属性
const CheckboxListTile({
Key key,
@required this.value,
@required this.onChanged,
this.activeColor,
this.checkColor,
this.title, // 主标题
this.subtitle, // 副标题
this.isThreeLine = false,
this.dense,
this.secondary, // 将显示在复选框平铺的另一侧的小部件。
this.selected = false,
this.controlAffinity = ListTileControlAffinity.platform,
}) : assert(value != null),
assert(isThreeLine != null),
assert(!isThreeLine || subtitle != null),
assert(selected != null),
assert(controlAffinity != null),
super(key: key);
基本用法
List <Map>_checkbox = [
{
"title" : "国庆倒计时",
"subTitle" : "剩余天数 3天",
"isActive" : false,
"Icon" : 0xe6b2
},
{
"title" : "中秋倒计时",
"subTitle" : "剩余天数 3天",
"isActive" : false,
"Icon" : 0xe6d9
},
{
"title" : "元旦倒计时",
"subTitle" : "剩余天数 90天",
"isActive" : false,
"Icon" : 0xe6ef
},
{
"title" : "除夕倒计时",
"subTitle" : "剩余天数 146",
"isActive" : false,
"Icon" : 0xe6ef
}
];
body: Column(
children : <Widget>[
for(num i = 0 ; i < _checkbox.length ; i ++)
CheckboxListTile(
title: Text( _checkbox[i]['title'] ) ,
subtitle: Text( _checkbox[i]['subTitle'] ),
secondary: Icon(
IconData(
_checkbox[i]['Icon'],
fontFamily : 'wz'
),
textDirection : TextDirection.ltr ,
size: 33,
color: Colors.yellow[700],
),
value: _checkbox[i]['isActive'],
onChanged: (e){
setState(() {
_checkbox[i]['isActive'] = e;
});
},
),
]
),
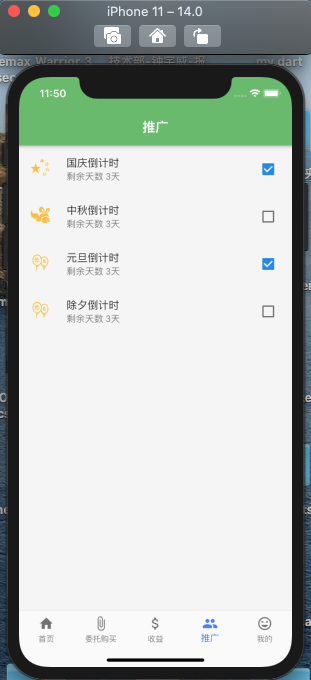