题意:
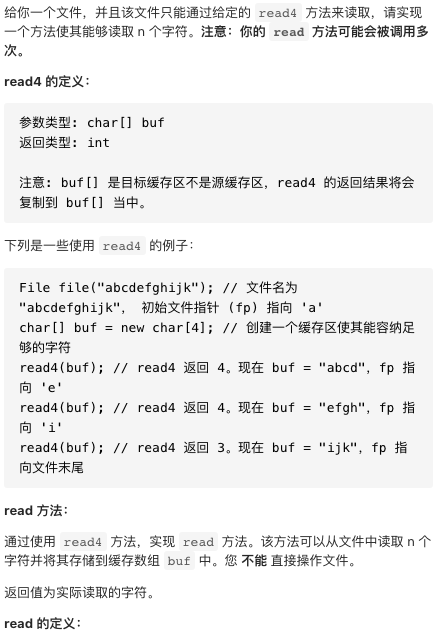
解题思路:
思路:
1. 循环读,循环条件判断长度是否到了n,到了之后继续下一轮读取操作;
PHP代码实现:
/* The read4 API is defined in the parent class Reader4.
public function read4(&$buf){} */
class Solution extends Reader4 {
/**
* @param Char[] &$buf Destination buffer
* @param Integer $n Number of characters to read
* @return Integer The number of actual characters read
*/
public $pointer = 0;
public $len = 0;
public $tmp = [];
function read(&$buf, $n) {
$index = 0;
while ($index < $n) {
if ($this->pointer == 0) {
$this->len = $this->read4($this->tmp);
}
if ($this->len == 0) break;
while ($index < $n && $this->pointer < $this->len) {
$buf[$index++] = $this->tmp[$this->pointer++];
}
// 说明临时字符数组中的内容已经读完,pointer置零以便执行下一次read4操作
if ($this->pointer >= $this->len) $this->pointer = 0;
}
return $index;
}
}
GO代码实现:
/**
* The read4 API is already defined for you.
*
* read4 := func(buf []byte) int
*
* // Below is an example of how the read4 API can be called.
* file := File("abcdefghijk") // File is "abcdefghijk", initially file pointer (fp) points to 'a'
* buf := make([]byte, 4) // Create buffer with enough space to store characters
* read4(buf) // read4 returns 4. Now buf = ['a','b','c','d'], fp points to 'e'
* read4(buf) // read4 returns 4. Now buf = ['e','f','g','h'], fp points to 'i'
* read4(buf) // read4 returns 3. Now buf = ['i','j','k',...], fp points to end of file
*/
var solution = func(read4 func([]byte) int) func([]byte, int) int {
// implement read below.
tmp := make([]byte, 4)
pointer, len := 0, 0
return func(buf []byte, n int) int {
index := 0
for index < n {
if pointer == 0 {
len = read4(tmp)
}
if len == 0 {
break
}
for index < n && pointer < len {
buf[index] = tmp[pointer]
index++
pointer++
}
if pointer >= len {
pointer = 0
}
}
return index
}
}