题意:
图示:
迭代图示(1):
递归图示(2):
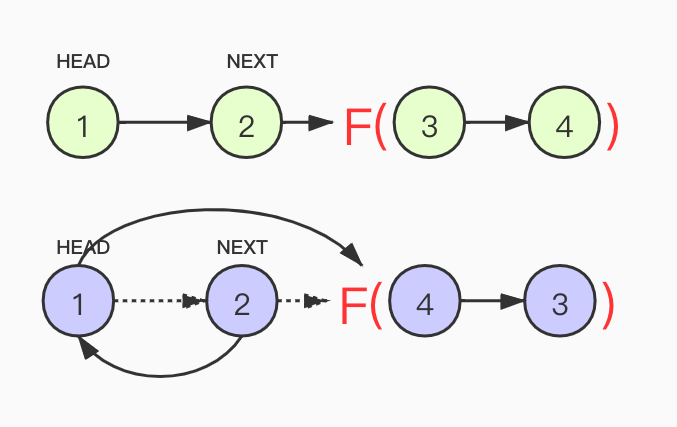
解题思路:
思路:
1. 添加虚拟头结点 dummy;
2. 定义cur指向dummy;
3. 初始化 $node1 = $cur->next; $node2 = $node1->next;
4. 修改cur,node1,node2三者指向关系,如上图所示
5. 移动cur结点到下一个结点,重复上面步骤,直到条件不满足为止
6. 最后返回dummy->next
PHP代码实现:
/**
* Definition for a singly-linked list.
* class ListNode {
* public $val = 0;
* public $next = null;
* function __construct($val) { $this->val = $val; }
* }
*/
class Solution {
/**
* @param ListNode $head
* @return ListNode
*/
function swapPairs($head) {
$dummy = new ListNode(0);
$dummy->next = $head;
$cur = $dummy;
while ($cur->next && $cur->next->next) {
$node1 = $cur->next;
$node2 = $node1->next;
$next = $node2->next;
$node2->next = $node1;
$node1->next = $next;
$cur->next = $node2;
$cur = $node1;
}
return $dummy->next;
}
function swapPairsRecursive($head) {
if ($head == null || $head->next == null) return $head;
$next = $head->next;
$head->next = $this->swapPairsRecursive($next->next);
$next->next = $head;
return $next;
}
}
GO代码实现:
/**
* Definition for singly-linked list.
* type ListNode struct {
* Val int
* Next *ListNode
* }
*/
func swapPairs(head *ListNode) *ListNode {
if head == nil || head.Next == nil {
return head
}
var dummy = &ListNode{}
dummy.Next = head
var cur = dummy
for cur.Next != nil && cur.Next.Next != nil {
node1 := cur.Next
node2 := node1.Next
next := node2.Next
node2.Next = node1
node1.Next = next
cur.Next = node2
cur = node1
}
return dummy.Next
}