题意:
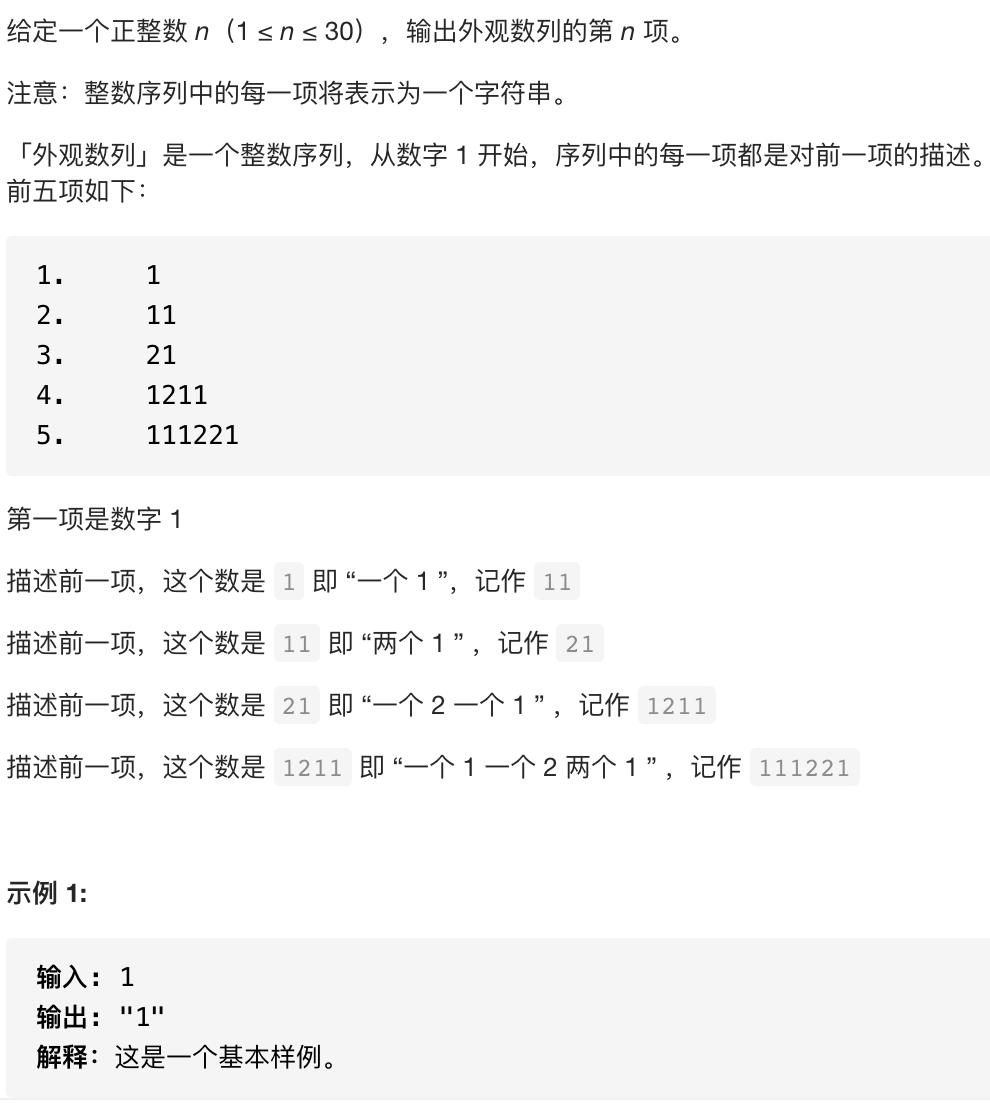
解题思路:
思路:
1. 从2-n的顺序生成字符串,每次找连续相同的数字段合并,比如11=》即 “两个 1”,记作 21;
2. 下一个数是对上一个数的描述,比方说4=1211 里有 “1 个 1 ,1 个 2,2 个 1”,那么 111221就是它的下一个数5的结果。
PHP代码实现:
class Solution {
function countAndSay($n) {
$res = "1";
for ($i = 2; $i <= $n; $i ++) {
$repeatCount = 1;
$str = '';
for ($j = 0; $j < strlen($res); $j++) {
if (isset($res[$j + 1]) && $res[$j] == $res[$j + 1]) {
$repeatCount++;
} else {
$str .= $repeatCount. $res[$j];
$repeatCount = 1;
}
}
$res = $str;
}
return $res;
}
}
GO代码实现:
func countAndSay(n int) string {
str := []string{"1"}
tmp := []string{}
l := 0
count := 1
for i := 2; i <= n; i++ {
l = len(str)
for j := 0; j < l; j++ {
if j + 1 < l && str[j + 1] == str[j] {
count++
continue
} else {
tmp = append(tmp, strconv.Itoa(count))
tmp = append(tmp, str[j])
count = 1
}
}
str = tmp
tmp = []string{}
}
return strings.Join(str, "")
}