一:Arrays类常见方法应用举例
1:toString( ) 返回数组的字符串形式
public class Test00 {
public static void main(String[] args) {
Integer[] integers = {1,5,7,8};
//遍历数组 ,返回的是字符串形式
System.out.println(Arrays.toString(integers));
}
}
2:sort( ) 排序(自然排序和定制排序)
<1>自然排序
public class Test00 {
public static void main(String[] args) {
Integer[] Arr2 = {5,9,1,4,7};
Arrays.sort(Arr2);
System.out.println("--------排序完成----------");
System.out.println(Arrays.toString(Arr2));
}
}
<2>定制排序
public class Test00 {
public static void main(String[] args) {
Integer[] Arr2 = {5,9,1,4,7};
Arrays.sort(Arr2);
Arrays.sort(Arr2, new Comparator<Integer>() {
@Override
public int compare(Integer o1, Integer o2) {
return o2 - o1;
}
});
System.out.println(Arrays.toString(Arr2));
}
}
<3>模拟排序
public class Test00 {
public static void main(String[] args) {
int[] Arr2 = {5,9,1,4,7};
bubble02(Arr2, new Comparator() {
@Override
public int compare(Object o1, Object o2) {
int n1 = (Integer)o1;
int n2 = (Integer)o2;
return n2 - n1;
}
});
Systen.out.println("-------排序结果---------")
System.out.println(Arrays.toString(Arr2));
}
public static void bubble02(int[] arr, Comparator c) {
int temp = 0;
for (int i = 0; i < arr.length - 1; i++) {
for (int j = 0; j < arr.length - 1 - i; j++) {
//数组排序由 c.compare(arr[j], arr[j + 1])返回的值决定
if (c.compare(arr[j], arr[j + 1] ) > 0) {
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
}
3:binarySearch( ) 通过二分搜索法进行查找
public class Test00 {
public static void main(String[] args) {
Integer[] integers = {1,5,7,8};
//1:要求该数组是有序的. 如果该数组是无序的,不能使用 binarySearch
//2:如果数组中不存在该元素
// 就返回 return -(low + 1); // key not found.
// (它应该在的位置的负数)
System.out.println(Arrays.binarySearch(integers,8)); //3
System.out.println(Arrays.binarySearch(integers,9)); //-5
}
}
4:copyOf 数组元素的复制 数组元素的复制
public class Test00 {
public static void main(String[] args) {
Integer[] Arr1 = {1,5,7,8};
//1. 从 arr 数组中,拷贝 arr.length 个元素到 newArr 数组中
//2. 如果拷贝的长度 > arr.length 就在新数组的后面 增加 null
//3. 如果拷贝长度 < 0 就抛出异常NegativeArraySizeException
//4. 该方法的底层使用的是 System.arraycopy()
Integer[] Arr2 = Arrays.copyOf(Arr1,4);
System.out.println("-----拷贝执行完毕-------");
System.out.println(Arrays.toString(Arr2));
}
}
5:fill( ) 数组元素的填充
public class Test00 {
public static void main(String[] args) {
Integer[] Arr2 = {1,5,7,8};
///1. 使用 99 去填充 num数组,可以理解成是替换原理的元素
Arrays.fill(Arr2,10);
System.out.println(Arrays.toString(Arr2));
}
}
6:alist( ) 将一组值转换为list
public class Test00 {
public static void main(String[] args) {
Integer[] Arr2 = {1,5,7,8};
//1. asList 方法,会将 (2,3,4,5,6,1)数据转成一个 List 集合
//2. 返回的 asList 编译类型 List(接口)
//3. asList 运行类型 java.util.Arrays#ArrayList,
// 是Arrays 类的静态内部类
List asList = Arrays.asList(2,3,4,5,6,1);
System.out.println("asList=" + asList);
}
}
二:Arrays类课堂练习
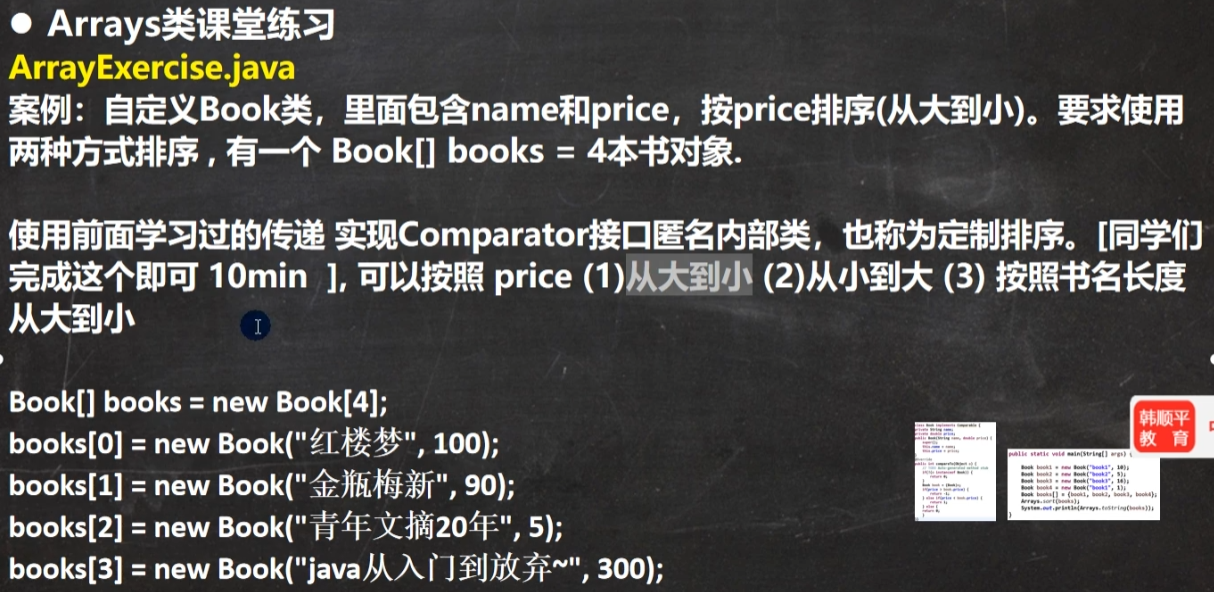
package Date0901.Test01;
import java.util.Comparator;
/**
* 作者:sakura
* 日期:2022年09月02日 00:53
*/
public class Test00 {
public static void main(String[] args) {
Book[] books = new Book[4];
books[0] = new Book("红楼梦", 100);
books[1] = new Book("金瓶梅新", 90);
books[2] = new Book("青年文摘20年", 5);
books[3] = new Book("java从入门到放弃~",300);
Pricesort(books, new Comparator() {
@Override
public int compare(Object o1, Object o2) {
int n1 = (int)o1;
int n2 = (int)o2;
return n2 -n1;
}
});
Namesort(books, new Comparator() {
@Override
public int compare(Object o1, Object o2) {
int n1 = (int)o1;
int n2 = (int)o2;
return n2 -n1;
}
});
System.out.println("--------排序结果--------");
for (int i = 0; i < books.length; i++) {
System.out.println(books[i].toString());;
}
}
public static void Pricesort(Book[] book, Comparator C) {
Book temp ;
for (int i = 0; i < book.length; i++) {
for (int j = 0; j < book.length -i-1 ; j++) {
if ( (C.compare(book[j].getPrice(),book[j+1].getPrice())) >0){
temp = book[j];
book[j] = book[j + 1];
book[j+1] = temp;
}
}
}
}
public static void Namesort(Book[] books,Comparator C){
Book temp;
for (int i = 0; i < books.length; i++) {
for (int j = 0; j < books.length-i-1; j++) {
if ( (C.compare(books[j].getName().length(),books[j+1].getName().length())) >0){
temp = books[j];
books[j] = books[j + 1];
books[j+1] = temp;
}
}
}
}
}
class Book {
private String name;
private int price;
public Book(String name, int price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", price=" + price +
'}';
}
}