一:多态数组,对象数组
数组的定义类型父类类型,里面保存的实际元素魏子类类型
1:应用实例
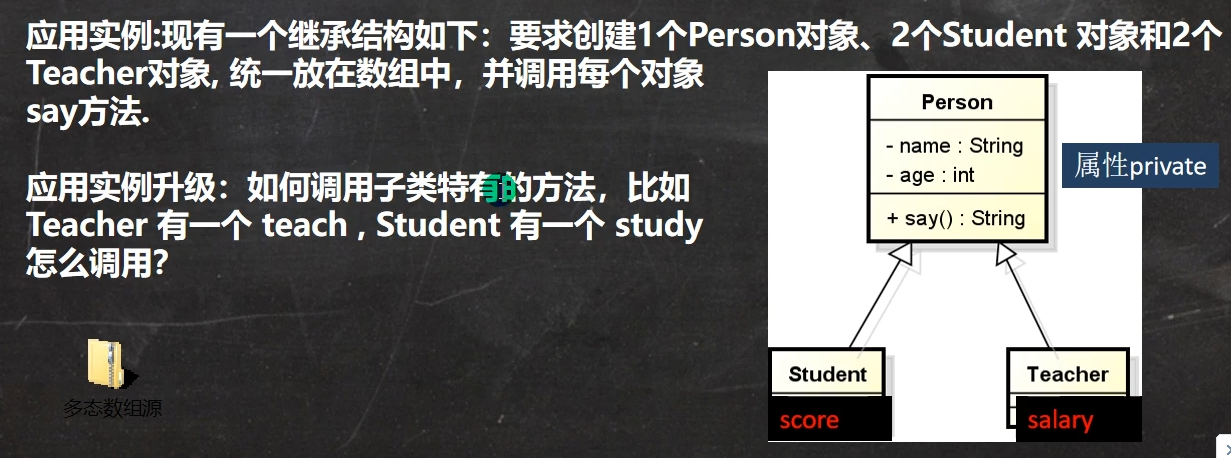
public class arrtest00 {
public static void main(String[] args) {
//对象数组
Person[] person = new Person[5];
//意味着person以及person的子类都可以放进去
person[0] = new Person("sakura",18);
person[1] = new Teacher("Tom",50,25000);
person[2] = new Student("jack",20,100);
for (int i = 0; i < 3; i++) {
System.out.println(person[i].say());
//如何调用子类的特有方法
if (person[i] instanceof Student){
// Student student = (Student) person[i];//向下转型
((Student)person[i]).study();//匿名对象的向下转型
}else if (person[i] instanceof Teacher){
((Teacher)person[i]).teach();
}
}
}
}
package Date0802.Test00;
/**
* 作者:sakura
* 日期:2022年08月03日 11:59
*/
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String say (){
return "name:"+this.name + "\t"+"age:"+ this.age;
}
}
package Date0802.Test00;
/**
* 作者:sakura
* 日期:2022年08月03日 11:59
*/
public class Student extends Person{
private int Score;
public Student(String name, int age, int score) {
super(name, age);
Score = score;
}
public int getScore() {
return Score;
}
public void setScore(int score) {
Score = score;
}
@Override
public String say() {
return "(学生)"+super.say() +"\t"+ "score:"+"\t"+this.Score;
}
public void study(){
System.out.println("学生:"+getName()+"正在学习");
}
}
package Date0802.Test00;
/**
* 作者:sakura
* 日期:2022年08月03日 12:00
*/
public class Teacher extends Person{
private int salary;
public Teacher(String name, int age, int salary) {
super(name, age);
this.salary = salary;
}
public int getSalary() {
return salary;
}
public void setSalary(int salary) {
this.salary = salary;
}
@Override
public String say() {
return "(老师)"+super.say() +"\t"+ "salary:" + this.salary;
}
public void teach(){
System.out.println("老师:"+ getName()+ "正在授课");
}
}
二:多态参数
1:应用举例
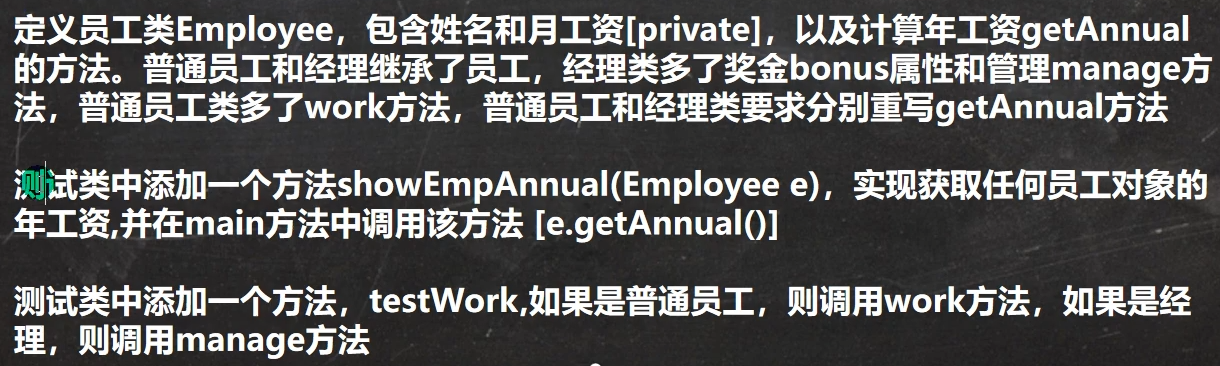
package Date0802.Test01;
/**
* 作者:sakura
* 日期:2022年08月03日 15:02
*/
public class Test01 {
public static void main(String[] args) {
staff tom = new staff("tom", 5000);
Managerment sakura = new Managerment("sakura", 10000, 9999);
Test01 T = new Test01();
T.showEmpAnn(sakura);
T.showEmpAnn(tom);
T.testWork(sakura);
T.testWork(tom);
}
public void showEmpAnn(Employee E){
E.getAnnual();
}
public void testWork(Employee E){
if (E instanceof staff){
((staff)E).work();
}else if(E instanceof Managerment){
((Managerment)E).manager();
}
}
}
package Date0802.Test01;
/**
* 作者:sakura
* 日期:2022年08月03日 14:49
*/
public class Employee {
private String name;
private int salary;
public Employee(String name, int salary) {
this.name = name;
this.salary = salary;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getSalary() {
return salary;
}
public void setSalary(int salary) {
this.salary = salary;
}
public void getAnnual(){
System.out.println(12*getSalary());
}
}
package Date0802.Test01;
/**
* 作者:sakura
* 日期:2022年08月03日 15:00
*/
public class Managerment extends Employee{
private int bonus;
public Managerment(String name, int salary, int bonus) {
super(name, salary);
this.bonus = bonus;
}
public int getBonus() {
return bonus;
}
public void setBonus(int bonus) {
this.bonus = bonus;
}
@Override
public void getAnnual() {
System.out.print("经理的"+getName()+"年工资:");
super.getAnnual();
}
public void manager(){
System.out.println("经理管理员工");
}
}
package Date0802.Test01;
/**
* 作者:sakura
* 日期:2022年08月03日 14:55
*/
public class staff extends Employee{
public staff(String name, int salary) {
super(name, salary);
}
public void work(){
System.out.println("员工工作");
}
@Override
public void getAnnual() {
System.out.print("员工"+getName()+"的年工资:");
super.getAnnual();
}
}