一:String反转

public class Test1 {
public static void main(String[] args) {
String string = "abcdef";
try {
System.out.println(reverse(string, -1, 4));
} catch (Exception e) {
System.out.println(e.getMessage());;
}
}
public static String reverse(String str, int start, int end) {
//还可以添加验证
//1: 先想出正确的
//2: 然后再取反(因为正确的容易想到)
if (!(str != null && start > end && start < str.length() &&
end < str.length() && start != end && start > +0)) {
throw new RuntimeException("参数不正确");
}
//先将String转换为char类型数组
char[] chars = str.toCharArray();
for (int i = start, j = end; i < j; i++, j--) {
char temp = chars[i];
chars[i] = chars[j];
chars[j] = temp;
}
return new String(chars);
}
}
二:注册处理
三:字符串统计

public class Test3 {
public static void main(String[] args) {
String name = "Han shun Ping";
System.out.println(printName(name));
}
public static String printName(String str) {
String[] s = str.split(" ");
String resultString = String.format("%s,%s .%c" ,s[2],s[0],s[0].toUpperCase().charAt(0));
return resultString;
}
}
四:统计字符串

public class Test4 {
public static void main(String[] args) {
String str = "saddfHOHN 1q534215";
countSting(str);
}
public static void countSting(String str){
int NumCount = 0;
int LowerCount = 0;
int UpCount = 0;
int Other = 0;
for (int i = 0; i < str.length(); i++) {
if(str.charAt(i)>='A' && str.charAt(i)<= 'Z'){
UpCount++;
}else if (str.charAt(i)>='0' && str.charAt(i)<= '9'){
NumCount++;
}else if (str.charAt(i)>='a' && str.charAt(i)<= 'z'){
LowerCount++;
}else {
Other++;
};
}
System.out.println("数字有:"+ NumCount);
System.out.println("大写字母有:"+ UpCount);
System.out.println("小写字母有:"+ LowerCount);
System.out.println("其他有:"+ Other);
}
}
五:
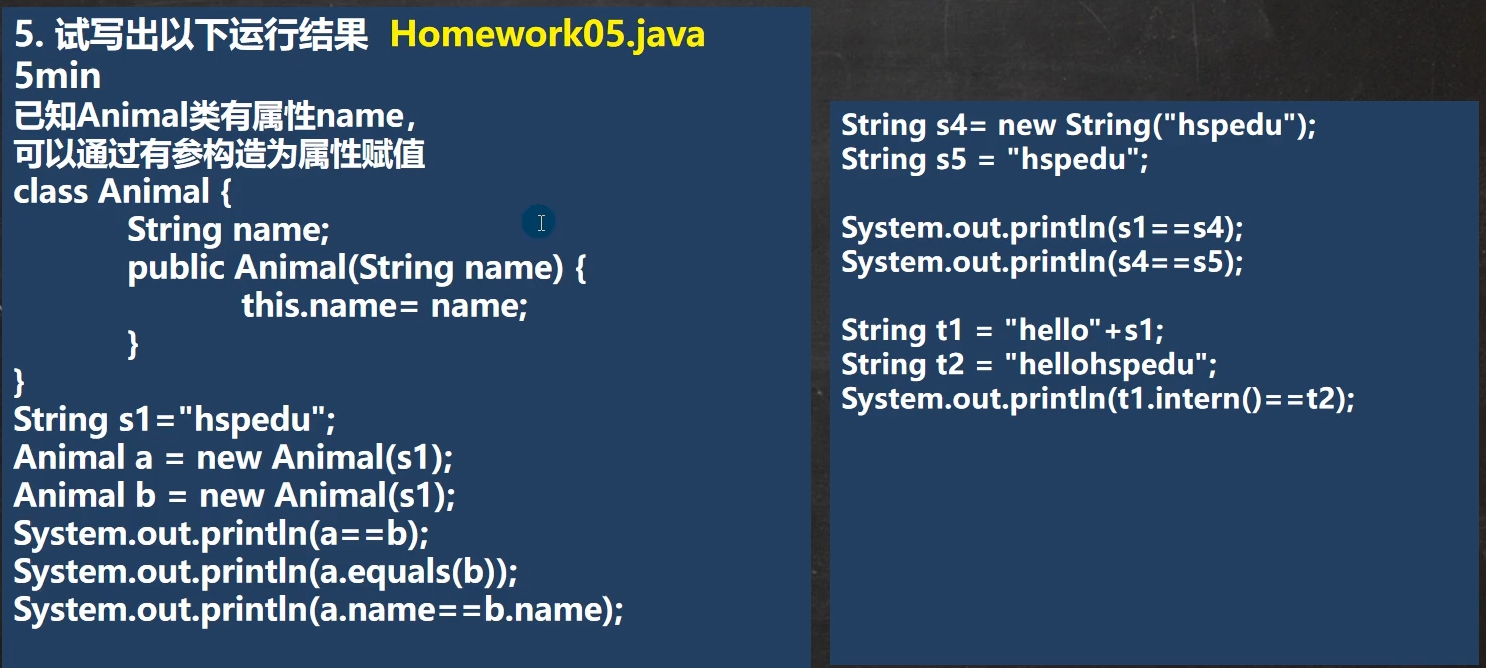
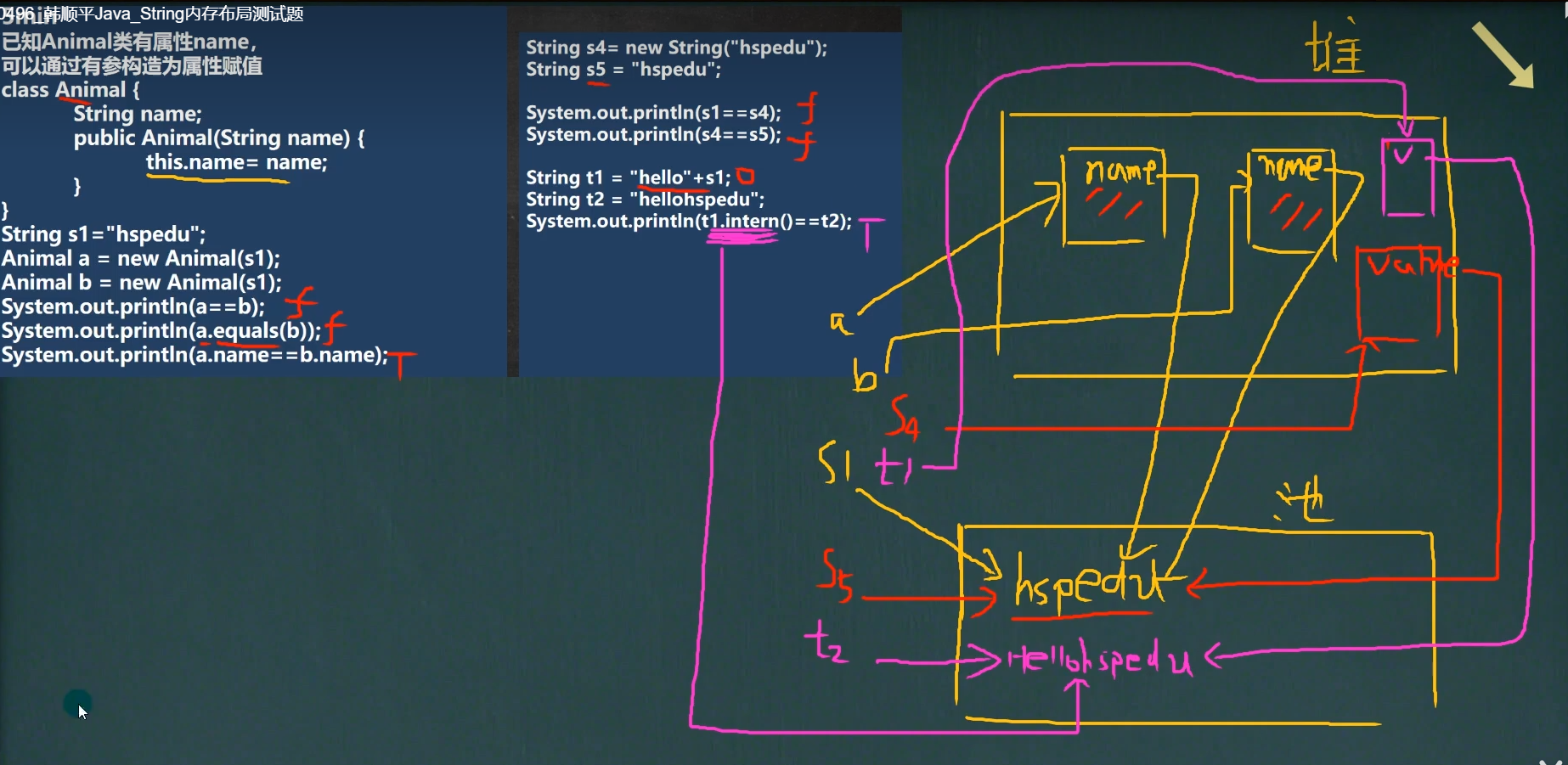