一:
package Date0805.Test03;
/**
* 作者:sakura
* 日期:2022年08月05日 14:24
*/
public class test1 {
public static void main(String[] args) {
Person[] person = new Person[3];
person[0] = new Person("kunkun", 90, "唱跳rap");
person[1] = new Person("sakura",20,"架构师");
person[2] = new Person("LF",18,"保安");
for (int i = 0; i < person.length-1; i++) {
for (int j = 0; j < person.length-1-i; j++) {
if (person[j].getAge()>person[j+1].getAge()){
/*int temp = 0;
temp = person[j].getAge();
person[j].setAge(person[j+1].getAge());
person[j+1].setAge(temp);*/
Person temp = new Person();
temp = person[j];
person[j] =person[j+1];
person[j+1] = temp;
}
}
}
for (int i = 0; i < person.length; i++) {
System.out.println(person[i].toString());
}
}
}
class Person{
private String name;
private int age;
private String job;
public Person() {
}
public Person(String name, int age, String job) {
this.name = name;
this.age = age;
this.job = job;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getJob() {
return job;
}
public void setJob(String job) {
this.job = job;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
", job='" + job + '\'' +
'}';
}
}
二:
三:
四:
五:
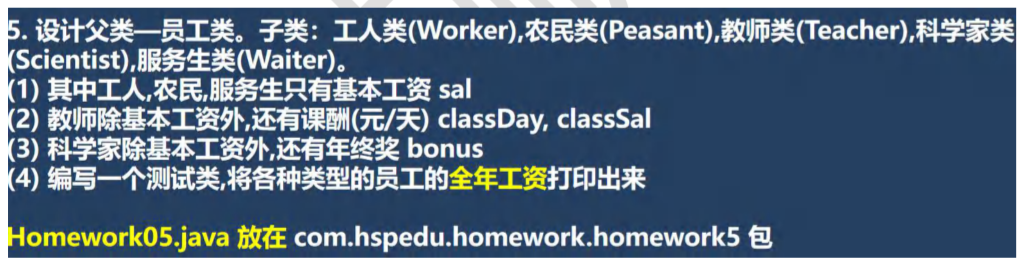
/**
* 作者:sakura
* 日期:2022年08月05日 21:45
*/
public class Test05 {
public static void main(String[] args) {
Employee employee = new Teacher("sakura",5000,30,200);
System.out.println(employee.getYearSal());
Employee employee1 = new Scientist("sakura",18000,80000);
System.out.println(employee1.getYearSal());
}
}
public class Employee {
private String name;
private double salary;
public Employee(String name, double salary) {
this.name = name;
this.salary = salary;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
public double getYearSal(){
return 12* getSalary();
}
}
public class Teacher extends Employee{
private double classDay;
private double classSal;
public Teacher(String name, double salary, double classDay, double classSal) {
super(name, salary);
this.classDay = classDay;
this.classSal = classSal;
}
public double getClassDay() {
return classDay;
}
public void setClassDay(double classDay) {
this.classDay = classDay;
}
public double getClassSal() {
return classSal;
}
public void setClassSal(double classSal) {
this.classSal = classSal;
}
@Override
public double getYearSal() {
System.out.println("老师"+getName()+"的年工资为:");
return super.getYearSal()+(getClassSal()*getClassDay());
}
}
public class Scientist extends Employee{
private double bonus;
public Scientist(String name, double salary, double bonus) {
super(name, salary);
this.bonus = bonus;
}
public double getBonus() {
return bonus;
}
public void setBonus(double bonus) {
this.bonus = bonus;
}
@Override
public double getYearSal() {
return super.getYearSal()+getBonus();
}
}
六:可以调用那些属性和方法
class Father extends Grand{/父类
String id="001";
private double score;
public void f1(){
//super可以访问哪些成员(属性和方法)?
super.name;
super.g10
//this可以访问哪些成员?
this.id;
this.score;
this.f1();
this.name;
this.g1():
}
}
class Son extends Father{//子类
String name="BB";
pubic void show(){
//uper可以访问哪些成员(属性和方法)?
super.id;
super.f1();
super.name;
super.g1():
//this可以访问哪些成员?
this.name;
this.g10;
this.show();
this.id;
this.f10;
}
七:写出程序结果
class Test{//父类
String name = "Rose";
Test()
System.out.println("Test");
}
Test(String name){
this.name = name;
}
}
class Demo extends Test{//子类
String name="Jack";Demo0 {
super();
System.out.println("Demo");
}
Demo(String s){
super(s);
}
public void testo{
System.out.println(super.name);System.out.println(this.name);
}
public static void main(Stringl] args)
new Demo0.testO;//匿名对象new Demo("john").testo;//匿名
}
八:
九:

十:

@Override
public boolean equals(Object o) {
if (this == o){
return true;
}
if (o instanceof Doctor){
Doctor doctor = (Doctor)o;
if (this.getName()==doctor.getName()&&
this.getAge()==doctor.getAge()&&
this.getGender()==doctor.getGender()&&
this.getJob()==doctor.getJob()&&this.getSal()==doctor.getSal()){
return true;
}
}else {
return false;
}
return false;
}
十一:
十二:
十三:

public class Person {
private String Name;
private String Gender;
private int age;
public Person(String name, String gender, int age) {
Name = name;
Gender = gender;
this.age = age;
}
public void play(){
System.out.println("");
}
public String getName() {
return Name;
}
public void setName(String name) {
Name = name;
}
public String getGender() {
return Gender;
}
public void setGender(String gender) {
Gender = gender;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public void printinfo(){
System.out.println();
}
}
public class Student extends Person{
private int stu_id;
public Student(String name, String gender, int age, int stu_id) {
super(name, gender, age);
this.stu_id = stu_id;
}
public void study(){
System.out.println("好好学习");
}
@Override
public void play() {
System.out.println("玩篮球");
}
public int getStu_id() {
return stu_id;
}
public void setStu_id(int stu_id) {
this.stu_id = stu_id;
}
@Override
public void printinfo() {
System.out.println("老师的信息:");
System.out.println("姓名:" + getName() + "\n" + "年龄:" + getAge() + "\n"+ "学号:" + getStu_id());
study();
play();
System.out.println("-----------------------------------------------------------");
}
}
public class Teacher extends Person{
private int work_age;
public Teacher(String name, String gender, int age, int work_age) {
super(name, gender, age);
this.work_age = work_age;
}
public void teach(){
System.out.println("教书育人");
}
@Override
public void play() {
System.out.println("玩象棋");
}
public int getWork_age() {
return work_age;
}
public void setWork_age(int work_age) {
this.work_age = work_age;
}
@Override
public void printinfo() {
System.out.println("老师的信息:");
System.out.println("姓名:" + getName() + "\n" + "年龄:" + getAge() +"\n"+ "工龄:" + getWork_age());
teach();
play();
System.out.println("-----------------------------------");
}
}
public class Test13 {
public static void main(String[] args) {
Person[] person= new Person[4];
person[0] = new Student("tom","男",8,1001);
person[1] = new Student("jack","男",78,365);
person[2] = new Teacher("sa","男",34,56);
person[3] = new Teacher("sak","男",45,54);
for (int i = 0; i < person.length; i++) {
for (int j = 0; j < person.length-1 -i; j++) {
if (person[j].getAge() < person[j+1].getAge()){
Person temp = null;
temp = person[j];
person[j] = person[j+1];
person[j+1] = temp;
}
}
}
for (int i = 0; i < person.length; i++) {
person[i].printinfo();
}
}
}