一,通讯录token模块的封装
package com.addicated.wework;
import io.restassured.RestAssured;
public class Wework {
private static String token;
public static String getWeworkToken(){
return RestAssured.given().log().all()
.queryParam("corpid", WeworkConfig.getInstance().corpid)
.queryParam("corpsecret", WeworkConfig.getInstance().secret)
.when().get("https://qyapi.weixin.qq.com/cgi-bin/gettoken")
.then().log().all().statusCode(200)
.extract().path("access_token");
}
public static String getToken(){
if(token==null){
token=getWeworkToken();
}
return token;
}
}
部门模块一览api测试编写
package com.testerhome.hogwarts.wework.contact;
import com.testerhome.hogwarts.wework.Wework;
import io.restassured.response.Response;
import static io.restassured.RestAssured.given;
public class Department {
public Response list(String id){
return given().log().all()
.param("access_token", Wework.getToken())
.param("id", id)
.when().get("https://qyapi.weixin.qq.com/cgi-bin/department/list")
.then().log().all().statusCode(200).extract().response();
}
}
- 之后同前文一样,根据方法生成测试方法 ```java package com.testerhome.hogwarts.wework.contact;
import org.junit.jupiter.api.BeforeEach; import org.junit.jupiter.api.Test;
import static org.hamcrest.CoreMatchers.equalTo; import static org.junit.jupiter.api.Assertions.*;
class DepartmentTest {
@BeforeEach
void setUp() {
}
@Test
void list() {
Department department=new Department();
department.list("").then().statusCode(200).body("department.name[0]", equalTo("定向班第一期"));
department.list("33").then().statusCode(200).body("department.name[0]", equalTo("定向班第一期"));
}
}
- 至此,初步框架成型,后期会进行不断的迭代封装。
- 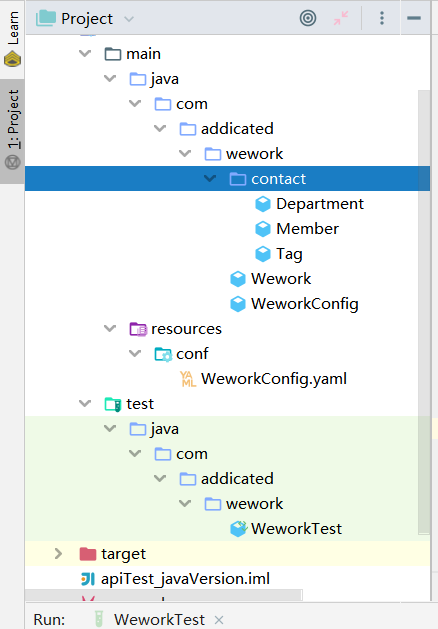
<a name="IpC6B"></a>
# 二,解决痛点
- 前面发送get请求获取部门list这样简单的api还好,不涉及很多参数,但是下面要进行post请求的api测试的话,构建请求体有的时候会让人蛋疼不已,下见例子。
```java
public class Department {
public Response list(String id){
return given().log().all()
.param("access_token", Wework.getToken())
.param("id",id)
.when().get("https://qyapi.weixin.qq.com/cgi-bin/department/list")
.then().log().all().statusCode(200).extract().response();
}
// 创建部门
public Response create(){
given().log().all()
.param("access_token", Wework.getToken())
.body("{\n" +
" \"name\": \"阿迪研发中心\",\n" +
" \"name_en\": \"RDGZ\",\n" +
" \"parentid\": 1,\n" +
" \"order\": 1,\n" +
" \"id\": 2\n" +
"}")
.when().post("https://qyapi.weixin.qq.com/cgi-bin/department/create")
.then().statusCode(200);
}
}
- 如上述代码一样,这样构建请求体,后期根本无法维护,且看着就头大。
- 为了解决这样的痛点,引入模板技术
- 注意点:使用restAssured的时候,如果发送post请求,但是请求地址需要拼接的时候
-
数据封装
使用模板技术
- 使用pojo实体类构建请求体数据
- 当请求提数据参数很多的时候会耗费大量时间去写pojo实体类,效率不高
- 使用jsonPath,yaml之类的对应库进行参数替换
先在resource下建一个data的文件夹,之后建一个json文件保存请求体实例数据
{
"name": "广州研发中心",
"name_en": "RDGZ",
"parentid": 1,
"order": 1,
"id": 2
}
- 之后编写读json的工具类代码,将处理好的请求体数据填入
注意点,有的json类库只可读不可写,本次使用的为下面这个库
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<version>2.4.0</version>
</dependency>
public Response create(String name,String parentid){
String body = JsonPath.parse(this.getClass()
// getResourceAsStream 会吧文件作为字节流读进来
.getResourceAsStream("/data/create.json"))
.set("$.name", name)
.set("parentid", parentid).jsonString();
return given().log().all()
.queryParam("access_token", Wework.getToken())
.body(body)
.when().post("https://qyapi.weixin.qq.com/cgi-bin/department/create")
.then().log().all().statusCode(200).extract().response();
}
三,编写测试方法之后发现新的痛点
对部门进行CRUD(增删查改)的api请求方法编写之后,会发现出现了大量重复臃肿代码,下见图 ```java package com.addicated.wework.contact;
import com.addicated.wework.Wework; import com.jayway.jsonpath.JsonPath; import io.restassured.response.Response;
import static io.restassured.RestAssured.given;
/**
- @description:
- @author: Adi
- @time: 2020/10/4 19:12 **/
public class Department {
public Response list(String id) {
return given().log().all()
.param("access_token", Wework.getToken())
.param("id", id)
.when().get("https://qyapi.weixin.qq.com/cgi-bin/department/list")
.then().log().all().statusCode(200).extract().response();
}
// 创建部门
public Response create(String name, String parentid) {
String body = JsonPath.parse(this.getClass()
.getResourceAsStream("/data/create.json"))
.set("$.name", name)
.set("parentid", parentid).jsonString();
return given().log().all()
.queryParam("access_token", Wework.getToken())
.body(body)
.when().post("https://qyapi.weixin.qq.com/cgi-bin/department/create")
.then().log().all().statusCode(200).extract().response();
}
// 删除部门
public Response delete(String id) {
return given().queryParam("access_token", Wework.getToken())
.queryParam("id", id)
.when().get("https://qyapi.weixin.qq.com/cgi-bin/department/delete")
.then().statusCode(200).extract().response();
}
// 更新部门
public Response update(String name, String id) {
String body = JsonPath.parse(this.getClass()
.getResourceAsStream("/data/update.json"))
.set("$.name", name)
.set("id", id).jsonString();
return given().log().all()
.queryParam("access_token", Wework.getToken())
.body(body)
.when().post("https://qyapi.weixin.qq.com/cgi-bin/department/update")
.then().log().all().statusCode(200).extract().response();
}
}
```
- 观察代码会发现其中有相当多的 given 之类的restAssured请求代码,这些东西需要进行统一的封装达到精简方便后期维护