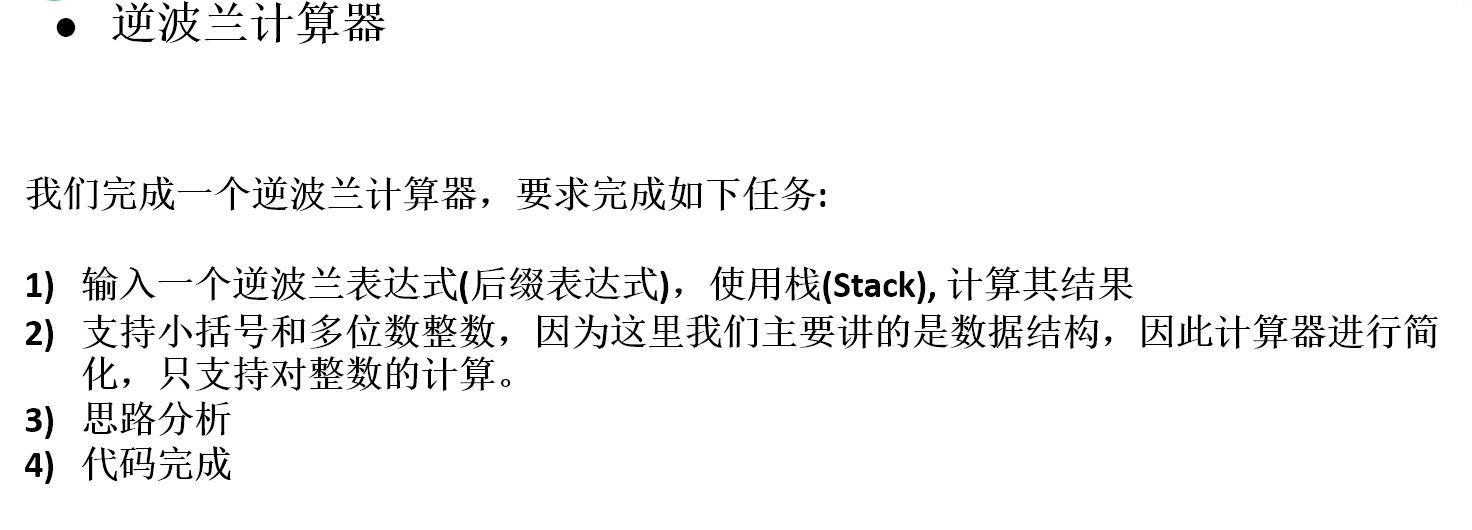
package com.atguigu.stack;
import java.util.ArrayList;
import java.util.List;
import java.util.Stack;
/**
* @author Dxkstart
* @create 2021-10-08-19:49
*/
public class PolandNotation {
public static void main(String[] args) {
//先定义一个逆波兰表达式
//(3+4)*5-6 => 3 4 + 5 * 6 -
String suffixExpression = "3 4 + 5 * 6 -";
//思路
//1.先将 "3 4 + 5 * 6 -" => 放到ArrayList中
//2.将ArrayList传递给一个方法,遍历ArrayList配合栈完成计算
List<String> rpnList = getListString(suffixExpression);
System.out.println("计算结果为:" + calculate(rpnList));
}
//将一个逆波兰表达式,依次将数据和运算符放入到ArrayList中
public static List<String> getListString(String suffixExpression) {
//将sufferExpression 分割
String[] split = suffixExpression.split(" ");
List<String> list = new ArrayList<>();
for (String str : split) {
list.add(str);
}
return list;
}
//完成对逆波兰表达式的运算
/*
1.从左至右扫描,将3和4压入堆栈
2.遇到+运算符,因此弹出4和3(4为栈顶元素,3为次顶元素),计算出3+4的值,得7,再将7入栈
3.将5入栈
4.接下来是×运算符,因此弹出5和7,计算出7*5=35,将35入栈
5.将6入栈
6.最后是-运算符,计算出35-6的值,即29,由此得出最终结果
*/
public static int calculate(List<String> ls) {
int val;
int num2;
int num1;
//创建一个栈,只需要一个就可以
Stack<String> stack = new Stack<>();
//遍历ls
for (String item : ls) {
//这里用正则表达式来判断取出来的是数字还是符号
if (item.matches("\\d+")) { //匹配的是多位数
//入栈
stack.push(item);
} else {
//pop出两个数,并运算,再将结果入栈
num2 = Integer.parseInt(stack.pop());
num1 = Integer.parseInt(stack.pop());
if (item.equals("+")) {
val = num1 + num2;
} else if (item.equals("-")) {
val = num1 - num2;
} else if (item.equals("*")) {
val = num1 * num2;
} else if (item.equals("/")) {
val = num1 / num2;
} else {
throw new RuntimeException("运算符有误!");
}
//把val入栈
stack.push(Integer.toString(val));
}
}
return Integer.parseInt(stack.pop());
}
}