1:spring5中文文档
2:Spring框架的新功能
Spring 5新功能和增强功能
(1):整个spring5框架代码基于java1.8,运行时兼容jdk1.9,许多不建议使用的类和方法,在代码库中进行删除;
(2):spring5框架自带了通用的日志封装
spring5已经移出log4ConfigListenter,官网建议使用log4j2,spring5已经整合了log4j2,如果要使用log4j则需要将spring版本降低到4
3:spring5整合log4j2
(1):引入jar包
(2):创建log4j2.xml
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="INFO">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{yyyy年MM月dd日 HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</Console>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Console"/>
</Root>
</Loggers>
</Configuration>
(3):自定义日志内容输出
4:Nullable注解和函数式注册对象
1:@Nullable 注解
(1):spring5框架核心容器支持@Nullable 注解可以使用在方法上,属性上, 参数上,使用该注解之后的返回值可以为空
(2):注解使用在方法上,方法返回值可以为空
(3):注解使用在方法参数中,改方法的参数可以为空
(4):注解使用在属性上,属性可以为空;
2:函数式风格
(1):spring5核心容器支持函数式风格GenericApplicationContext
(2):函数式创建对象
测试:因为现在注册的不是跟之前的方式一样是类名的小写,所以找不到对象
解决方法1:通过类的全路径进行获取创建对象
解决方法2:通过设置 registerBean中参数进行自定义对象名称,改名称可以为空也可以自定义
5:整合JUnit5单元测试框架
1:整合junit4测试
(1):引入spring相关测试依赖jar
(2):创建测试类,使用注解完成测试
- [@RunWith作用](https://blog.csdn.net/weixin_43671497/article/details/90543225#RunWith_2)
- 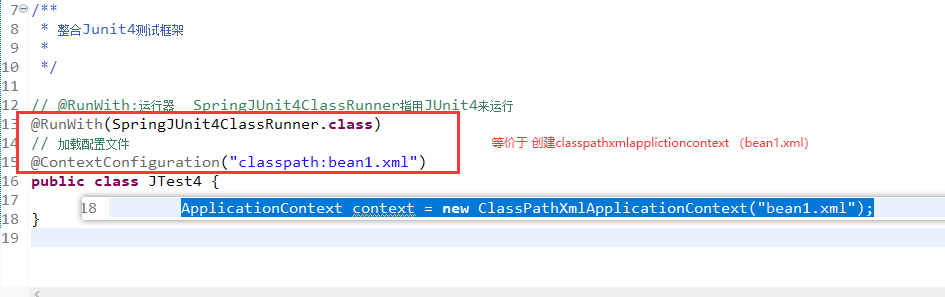
- 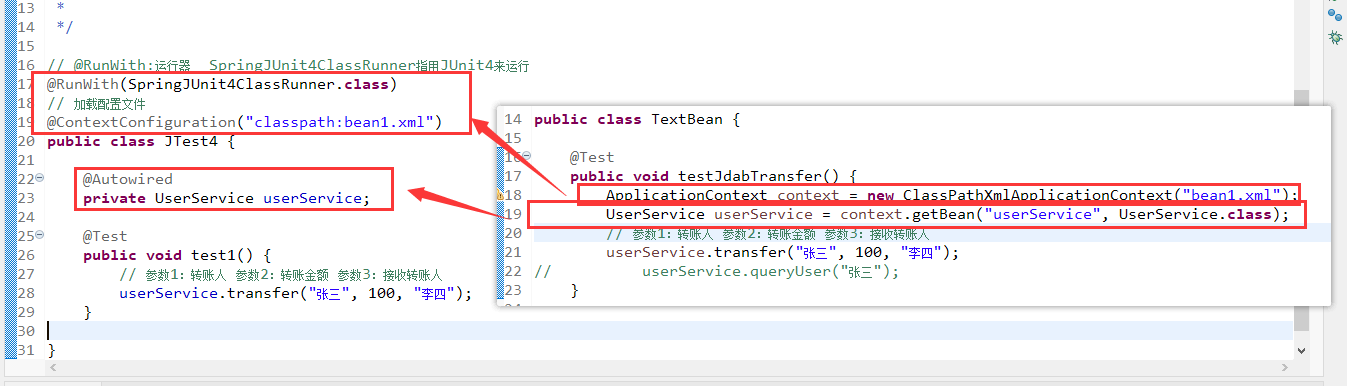
package com.junjay.spring5.test;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import com.junjay.spring5.service.UserService;
/**
* 整合Junit4测试框架
*
*/
// @RunWith:运行器 SpringJUnit4ClassRunner指用JUnit4来运行
@RunWith(SpringJUnit4ClassRunner.class)
// 加载配置文件
@ContextConfiguration("classpath:bean1.xml")
public class JTest4 {
@Autowired
private UserService userService;
@Test
public void test1() {
// 参数1:转账人 参数2:转账金额 参数3:接收转账人
userService.transfer("张三", 100, "李四");
}
}
2:整合junit5框架测试
1:引入junit5 jar
测试:
package com.junjay.spring5.test;
import org.junit.jupiter.api.Test;
import org.junit.jupiter.api.extension.ExtendWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit.jupiter.SpringExtension;
import com.junjay.spring5.service.UserService;
/**
* 整合Junit5测试框架
*
*/
@ExtendWith(SpringExtension.class)
@ContextConfiguration("classpath:bean1.xml")
public class JTest5 {
@Autowired
private UserService userService;
@Test
public void test1() {
// 参数1:转账人 参数2:转账金额 参数3:接收转账人
userService.transfer("张三", 100, "李四");
}
}
3:junit4和junit5对比
4:junit4和junit5复合
@SpringJUnitConfig(locations = “classpath:bean1.xml”) 一个复合注解做到指定版本和加载配置文件
package com.junjay.spring5.test;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.test.context.junit.jupiter.SpringJUnitConfig;
import com.junjay.spring5.service.UserService;
/**
* 复合Junit5和Junit4 测试框架
*
*/
@SpringJUnitConfig(locations = "classpath:bean1.xml")
public class JTest {
@Autowired
private UserService userService;
@Test
public void test1() {
// 参数1:转账人 参数2:转账金额 参数3:接收转账人
userService.transfer("张三", 100, "李四");
}
}
git@github.com:My-Jun/spring5_demo6.git