1、Collections
- Collections概述和使用
- 针对集合操作的工具类
Collections类的常用方法
- public static
> void sort(List list):将指定的列表按升序排序 - public static void reverse(List<?> list):反转指定列表中元素的顺序
public static void shuffle(List<?> list):使用默认的随机源随机排列指定的列表
/*
ArrayList存储学生对象,使用Collections对ArrayList进行排序
要求:按照年龄从小到大排序,年龄相同时,按照姓名的字母顺序排序
*/
public class CollectionsDemo {
public static void main(String[] args) {
//创建对象
ArrayList<Student> arr = new ArrayList<>();
Student s1 = new Student("a", 18);
Student s2 = new Student("b", 20);
Student s3 = new Student("c", 19);
Student s4 = new Student("d", 19);
arr.add(s1);
arr.add(s2);
arr.add(s3);
arr.add(s4);
Collections.sort(arr, new Comparator<Student>() {
@Override
public int compare(Student o1, Student o2) {
int num = o1.getAge() - o2.getAge();
int num2 = num == 0 ? o1.getName().compareTo(o2.getName()) : num;
return num2;
}
});
for (Student s : arr) {
System.out.println(s.getName() + ", " + s.getAge());
}
}
}
- public static
模拟斗地主
```java / 通过程序实现斗地主过程中的洗牌,发牌和看牌 / public class PokerDemo { public static void main(String[] args) { //创建牌盒 ArrayList
arrayList = new ArrayList<>(); //创建牌 //牌有四种花色,每种花色都有2-A的牌,大小王 //创建花色数组和数字数组,进行拼接得到需要的牌 String[] colors = {“♥”, “♦”, “♠”, “♣”}; String[] numbers = {“2”, “3”, “4”, “5”, “6”, “7”, “8”, “9”, “10”, “J”, “Q”, “K”, “A”}; for (String color : colors) {
for (String number : numbers) {
arrayList.add(color + number);
}
} arrayList.add(“大王”); arrayList.add(“小王”);
//洗牌 //使用Collections的shuffle方法 Collections.shuffle(arrayList);
//发牌 //创建三个人的牌盒,以及底牌的牌盒并把牌分发给四个牌盒 ArrayList
arrayList1 = new ArrayList<>(); ArrayList arrayList2 = new ArrayList<>(); ArrayList arrayList3 = new ArrayList<>(); ArrayList dpArrayList = new ArrayList<>(); for (int i = 0; i < arrayList.size(); i++) { String poker = arrayList.get(i);
//如果是最后三张牌则放入底牌
if (i >= arrayList.size() - 3) {
dpArrayList.add(poker);
} else if (i % 3 == 0) {
arrayList1.add(poker);
} else if (i % 3 == 1) {
arrayList2.add(poker);
} else if (i % 3 == 2) {
arrayList3.add(poker);
}
}
System.out.println(“王小恒的牌是:” + arrayList1); System.out.println(“王中恒的牌是:” + arrayList2); System.out.println(“王大恒的牌是:” + arrayList3); System.out.println(“底牌是:” + dpArrayList);
}
}
- **模拟斗地主升级版**
- 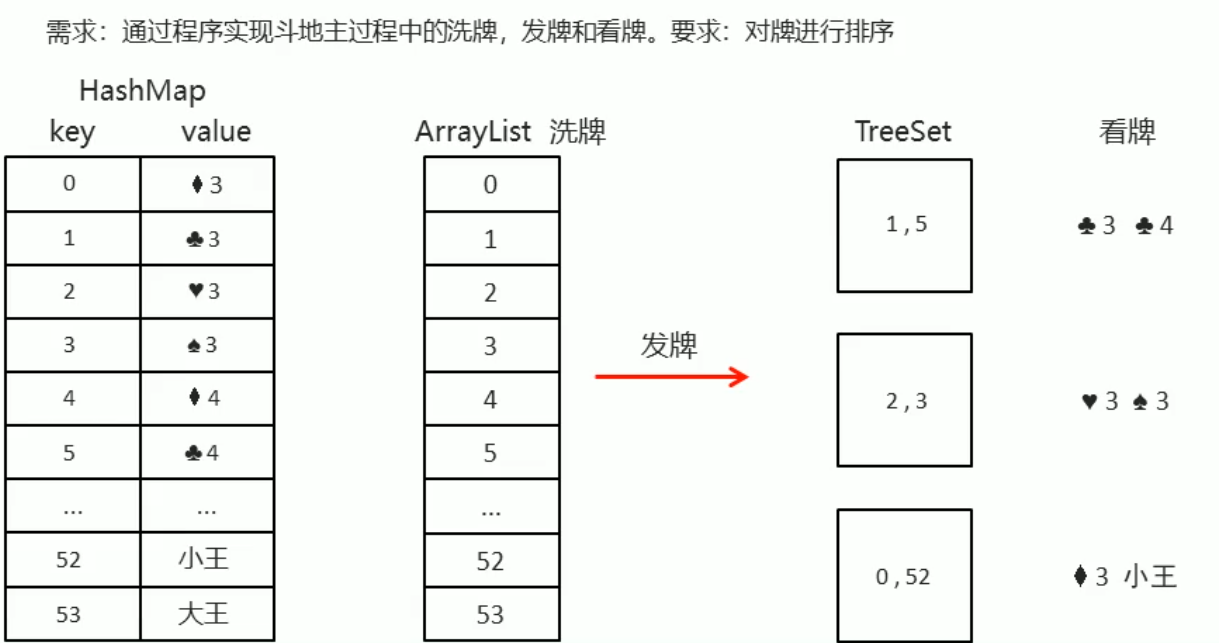
```java
/*
模拟斗地主升级版
通过程序实现斗地主过程中的洗牌,发牌和看牌
对牌进行排序
*/
public class PokerDemoPlus {
public static void main(String[] args) {
//创建索引和牌之间的映射
HashMap<Integer, String> hm = new HashMap<>();
//创建牌
StringBuilder sb = new StringBuilder();
String[] colors = {"♥", "♦", "♠", "♣"};
String[] numbers = {"2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K", "A"};
for (String number : numbers) {
for (String color : colors) {
sb.append(color + number);
sb.append(" ");
}
}
sb.append("大王 ");
sb.append("小王");
String poker = sb.toString();
String[] pokers = poker.split(" "); //得到牌的一个String数组
//新建牌盒,牌盒中存储索引值即map中的键
ArrayList<Integer> arr = new ArrayList<>();
//将索引与牌对应,并把索引存入牌盒
for (int i = 0; i < pokers.length; i++) {
hm.put(i, pokers[i]);
arr.add(i);
}
//洗牌 将索引值打乱
Collections.shuffle(arr);
//发牌,需要创建牌盒
TreeSet<Integer> ts1 = new TreeSet<>();
TreeSet<Integer> ts2 = new TreeSet<>();
TreeSet<Integer> ts3 = new TreeSet<>();
TreeSet<Integer> dpTs = new TreeSet<>();
for (int i = 0; i < arr.size(); i++) {
int pokerIndex = arr.get(i);
//最后三张牌放入底牌盒
if (i >= arr.size() - 3) {
dpTs.add(pokerIndex);
} else if (i % 3 == 0) {
ts1.add(pokerIndex);
} else if (i % 3 == 1) {
ts2.add(pokerIndex);
} else if (i % 3 == 2) {
ts3.add(pokerIndex);
}
}
lookPoker("王小恒", ts1, hm);
lookPoker("王中恒", ts2, hm);
lookPoker("王大恒", ts3, hm);
lookPoker("底牌", dpTs, hm);
System.out.println("");
}
public static void lookPoker(String name, TreeSet<Integer> ts, HashMap<Integer, String> hm) {
System.out.print(name + "的牌是:");
for (int index : ts) {
System.out.print(hm.get(index) + " ");
}
System.out.println();
}
}