声明语句:
//type/var identifier (= local-variable-initializer 初始化器:是一个表达式)
var x=100;
int[] y={1,2,3);
string name="美容";
迭代(循环)语句:
- while 语句按不同条件执行一个嵌入语句(循环体)零次或多次
- do while 语句按不同条件执行一个嵌入语句一次或多次
- for 计数循环
- foreach语句用于枚举一个集合的元素,并对该集合中的每个元素执行一次相关的嵌入语句
do while
static void Main(string[] args)
{
int score = 0;
bool canContinue= true;
do
{
Console.WriteLine("input first number:");
string str1 = Console.ReadLine();
if (str1.ToLower()=="end")
{
break;
}
int num1 = 0;
try
{
num1 = int.Parse(str1);
}
catch (OverflowException e)
{
Console.WriteLine(e.Message);
continue;
}
catch (Exception)
{
throw; continue;
}
Console.WriteLine("input second number:");
string str2 = Console.ReadLine();
if (str2.ToLower() == "end")
{
break;
}
int num2 = 0;
try
{
num2 = int.Parse(str2);
}
catch (OverflowException e)
{
Console.WriteLine(e.Message);
continue;
}
catch (Exception)
{
throw; continue;
}
int sum = num1 + num2;
if (sum == 100)
{
score++;
Console.WriteLine("{0}+{1}={2}", num1, num2, sum);
}
else
{
Console.WriteLine("{0}+{1}={2}", num1, num2, sum);
canContinue = false;
}
} while (canContinue);
Console.WriteLine("the total score is : {0}",score);
}
for
static void Main(string[] args)
{
for (int i = 0; i < 9; i++)
{
for (int j = 0; j <= i; j++)
{
Console.Write("{0}*{1}={2} ", i + 1, j + 1, (i + 1) * (j + 1));
}
Console.WriteLine();
}
}

foreach
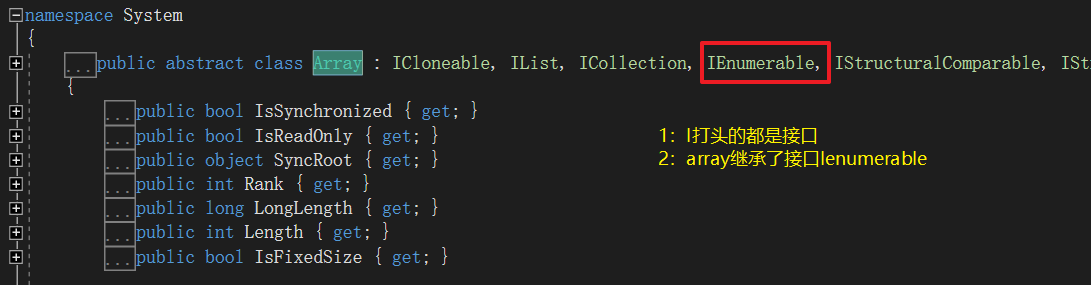
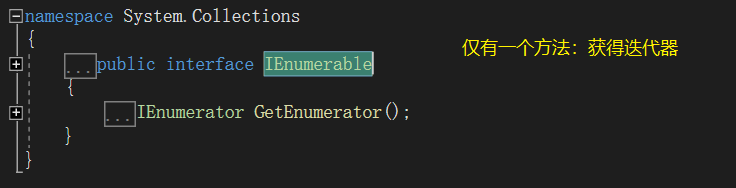
下述为集合遍历的底层原理和迭代器,foreach语句就是对集合遍历的简记法。
int[] arrList = { 1, 2, 3, 4, 5, 6 };
Console.WriteLine(arrList.GetType().FullName);// gettype 返回值为type类型,并且,type具有fullname属性
Console.WriteLine(arrList is Array);//array 继承了Ienumerable接口,故有获得迭代器方法GetEnumerator()
IEnumerator enumerator = arrList.GetEnumerator();
while (enumerator.MoveNext())
{
Console.WriteLine(enumerator.Current);
}
enumerator.Reset();
enumerator.MoveNext();
Console.WriteLine(enumerator.Current);//迭代器三个常用成员:movenext()、reset()、current属性
// foreach简单方法
foreach (var current in arrList)
{
Console.WriteLine(current);
}
//另一个例子
List<int> intList = new List<int>() { 1,2,3,4}; //泛型,等效于ArrayList
IEnumerator enumerator1 = intList.GetEnumerator();
while (enumerator1.MoveNext())
{
Console.WriteLine(enumerator1.Current);
}