BFS问题处理模板
// 计算从起点 start 到终点 target 的最近距离
int BFS(Node start, Node target) {
Queue<Node> q; // 核心数据结构
Set<Node> visited; // 避免走回头路
q.offer(start); // 将起点加入队列
visited.add(start);
int step = 0; // 记录扩散的步数
while (q not empty) {
int sz = q.size();
/* 将当前队列中的所有节点向四周扩散 */
for (int i = 0; i < sz; i++) {
Node cur = q.poll();
/* 划重点:这里判断是否到达终点 */
if (cur is target)
return step;
/* 将 cur 的相邻节点加入队列 */
for (Node x : cur.adj())
if (x not in visited) {
q.offer(x);
visited.add(x);
}
}
/* 划重点:更新步数在这里 */
step++;
}
}
BFS典型案例
LeetCode 102. 二叉树的层序遍历
class Solution {
public List<List<Integer>> levelOrder(TreeNode root) {
return bfs(root);
}
private List<List<Integer>> bfs(TreeNode root) {
List<List<Integer>> result = new ArrayList<>();
if (root == null) {
return result;
}
//BFS类型问题模板, 如果是图等其他结构,还需要用set等保存已经访问过的节点,避免重复访问
//1.初始化Queue,添加头结点
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(root);
//遍历Queue
while (!queue.isEmpty()) {
int size = queue.size();
List<Integer> row = new ArrayList<>();
for (int i=0; i< size; i++) {
TreeNode node = queue.poll();
row.add(node.val);
if (node.left != null) {
queue.offer(node.left);
}
if (node.right != null) {
queue.offer(node.right);
}
}
result.add(row);
}
//其他处理
return result;
}
}
DFS问题处理模板
DFS问题的递归写法:
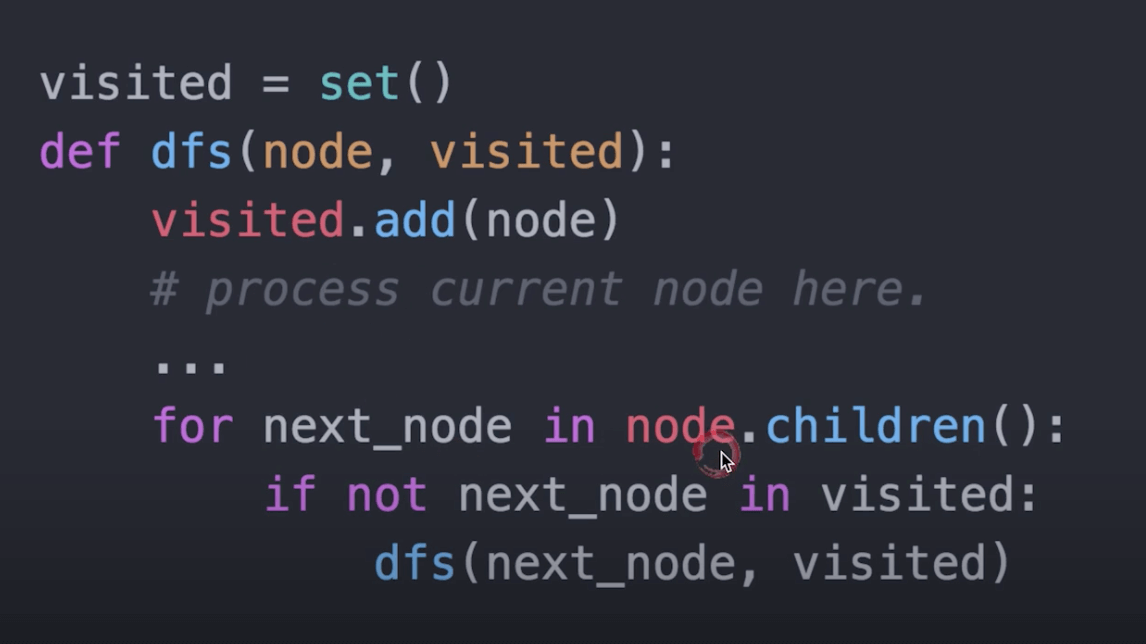
DFS的非递归写法
DFS典型案例
LeetCode 200. 岛屿数量
class Solution {
public int numIslands(char[][] grid) {
//处理边界
if (grid.length == 0) {
return 0;
}
int m = grid.length;
int n = grid[0].length;
int count = 0;
for (int i=0; i<m; i++) {
for (int j=0; j< n; j++) {
if (grid[i][j] == '1') {
count++;
dfs(i, j, m, n, grid);
}
}
}
return count;
}
private void dfs(int i, int j, int m, int n, char[][] grid) {
if (i<0 || i>=m || j<0 || j>=n || grid[i][j] != '1') {
return;
}
grid[i][j] = '0';
dfs(i-1, j, m, n, grid);
dfs(i+1, j, m, n, grid);
dfs(i, j-1, m, n, grid);
dfs(i, j+1, m, n, grid);
}
}
LeetCode 113. 路径总和 ||
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
public List<List<Integer>> pathSum(TreeNode root, int sum) {
List<List<Integer>> result = new ArrayList<>();
if (root == null) {
return result;
}
dfs(root, 0, sum, new ArrayList<>(), result);
return result;
}
private void dfs(TreeNode root, int now, int sum, List<Integer> path, List<List<Integer>> res) {
if (root == null) {
return;
}
//这里把root加入进去
path.add(root.val);
if (root.left == null && root.right == null) {
if (sum == root.val+ now) {
res.add(path);
}
return;
}
if (root.left != null) {
dfs(root.left, now + root.val, sum, new ArrayList<>(path), res);
}
if (root.right != null) {
dfs(root.right, now + root.val, sum, new ArrayList<>(path), res);
}
}
}