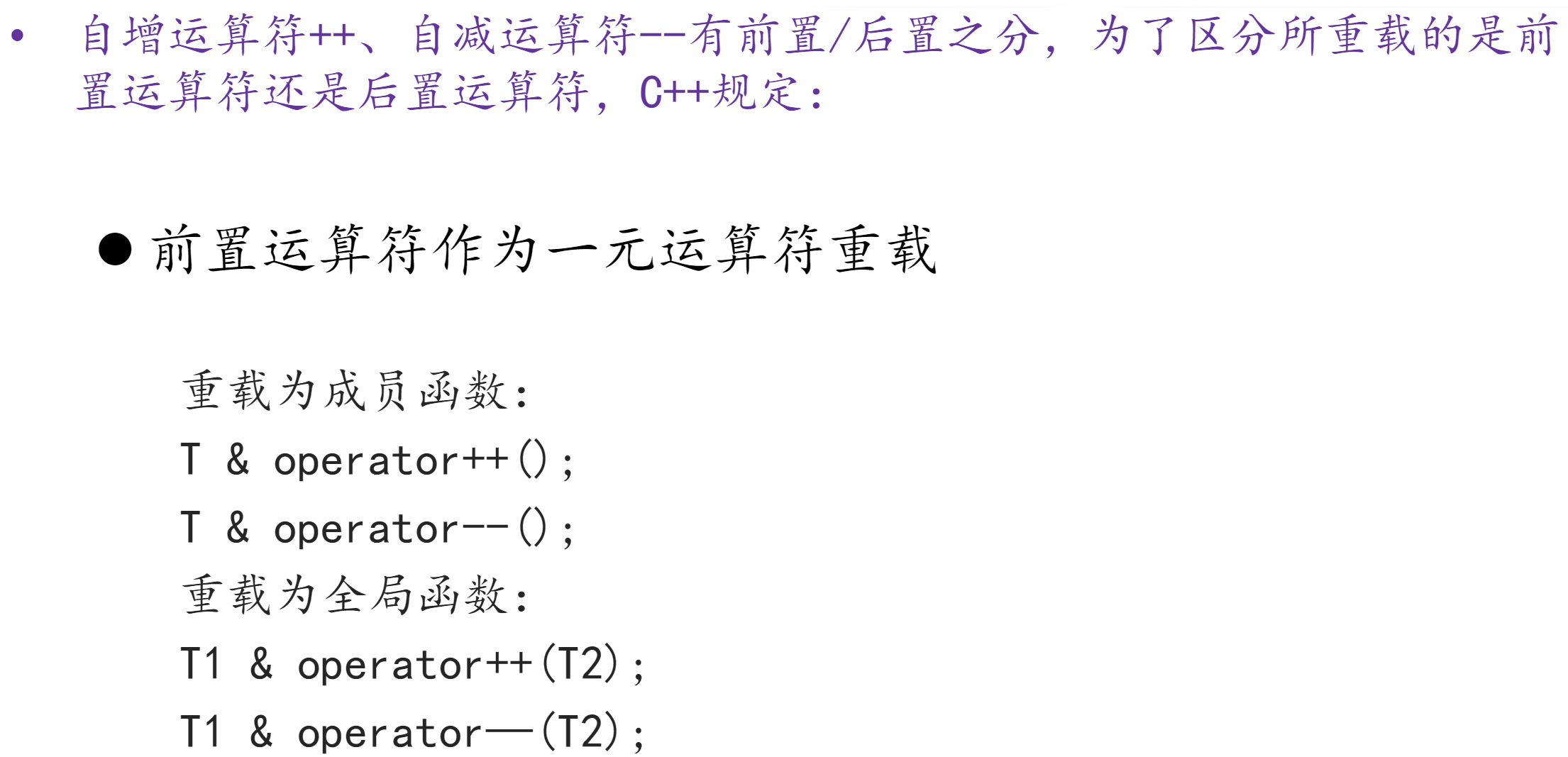
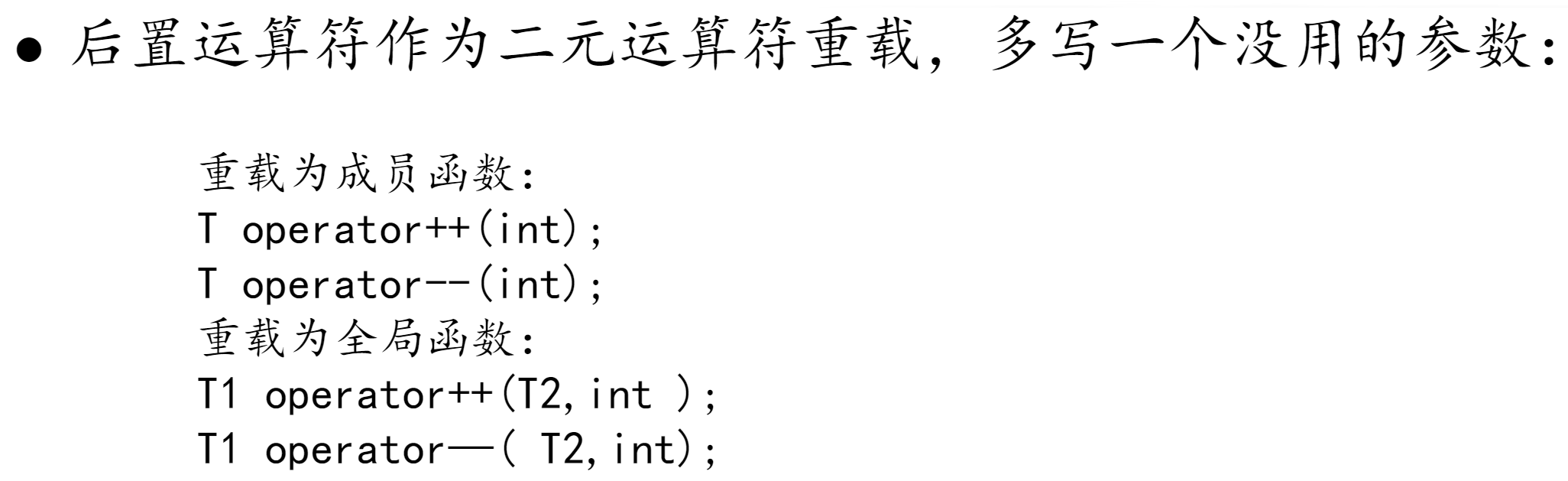
#include <bits/stdc++.h>
using namespace std;
//Dividing Line---------------------------------------------------------------------------------------------
class demo
{
public:
int n;
demo(int i = 0) : n(i){};
demo &operator++(void); //前置
demo operator++(int x); //后置
friend demo &operator--(demo &);
friend demo operator--(demo &, int);
};
demo &demo::operator++(void)
{
++n;
return *this;
}
demo demo::operator++(int x)
{
demo tmp(*this);
n++;
return tmp;
}
demo &operator--(demo &c)
{
--c.n;
return c;
}
demo operator--(demo &c, int useless)
{
demo tmp(c);
c.n--;
return tmp;
}
ostream &operator<<(ostream &os, const demo &c)
{
os << c.n;
return os;
}
//Dividing Line-----------------------------------------------------------------------------------------------
int main(void)
{
demo d(8);
cout << d++ << endl;
cout << d << endl;
cout << ++d << endl;
cout << d << endl;
cout << d-- << endl;
cout << d << endl;
cout << --d << endl;
cout << d << endl;
return 0;
}
/*
8
9
10
10
10
9
8
8
*/