类型转换构造函数(内置类型->自定义类型)
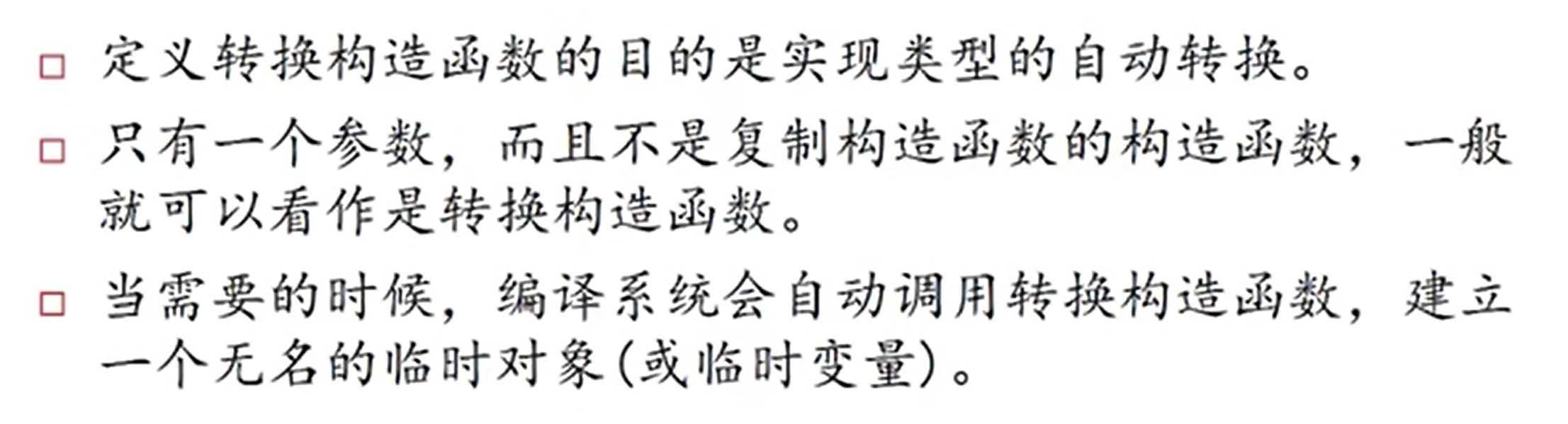
实例
#include<bits/stdc++.h>
using namespace std;
class Complex
{
public:
double real,imag;
Complex(int);
Complex(double,double);
};
Complex::Complex(double r,double i)
{
real=r;
imag=i;
std::cout<<"Constructor Called"<<endl;
}
Complex::Complex(int n)
{
real=n;
imag=0;
std::cout<<"int-type Conversion Constructor Called"<<endl;
}
int main(void)
{
Complex c1(3,4);//普通构造函数
Complex c2=5;//类型转换构造函数
c1=7;//类型转换构造函数 7自动转换成一个临时Complex类对象赋给c1
std::cout<<c1.real<<ends<<c1.imag<<endl;
/*Output
Constructor Called
int-type Conversion Constructor Called
int-type Conversion Constructor Called
7 0 */
return 0;
}
自动类型转换函数(自定义类型->内置/自定义类型)
实例
#include <bits/stdc++.h>
using namespace std;
class A
{
private:
int x;
public:
A(int m): x(m) {};
void int_t(int i) { cout << "int = " << i << endl; };
void double_t(double d) { cout << "double = " << d << endl; }
void A_t(const A& a) { cout << "A = " << a.x << endl; }
};
class Fraction
{
private:
int num;
int den;
public:
Fraction(int x, int y):num(x), den(y) {};
operator int() const {return (int)(num / den);};
operator double() const {return (double)(num * 1.0 / den);};
operator A() const {return A(num);}
};
int main(void)
{
Fraction fra(9,7);
A a(0);
a.double_t(fra);
a.int_t(fra);
a.A_t(fra);
}
/*
double = 1.28571
int = 1
A = 9
*/
析构函数
实例
#include<bits/stdc++.h>
using namespace std;
class CName
{
private:
char *Name;
public:
CName(void);
~CName(void);
};
CName::CName(void)
{
Name=new char[10];
cout<<"Constructor Called"<<endl;
}
CName::~CName(void)
{
delete [] Name;
cout<<"Destructor Called"<<endl;
}
int main(void)
{
CName c;//对象初始化,调用构造函数
//Constructor Called
return 0;//程序结束,对象消亡,调用析构函数
//Destructor Called
}
析构函数和数组
#include<bits/stdc++.h>
using namespace std;
class CTest
{
public:
~CTest(void);
};
CTest::~CTest(void)
{
cout<<"Destructor Called"<<endl;
}
int main(void)
{
CTest arr[2];
//delete运算符导致析构函数的调用
CTest *pTest=new CTest;
delete pTest;
CTest *c=new CTest[3];
delete [] c;
cout<<"End Main"<<endl;
return 0;
}
/*Destructor Called
Destructor Called
Destructor Called
Destructor Called
End Main
Destructor Called
Destructor Called
*/
在对象作为形参和作为函数返回值时被调用
#include<bits/stdc++.h>
using namespace std;
class CTest
{
public:
~CTest(void);
};
CTest::~CTest(void)
{
cout<<"Destructor Called"<<endl;
}
CTest C;
CTest obj(CTest c)
{
return c;//函数执行完毕,对象形参消亡,调用
}
int main(void)
{
C=obj(C);//函数调用返回的临时对象被使用过了,消亡
return 0;//程序结束,全局对象消亡
}
/*
Destructor Called
Destructor Called
Destructor Called
*/