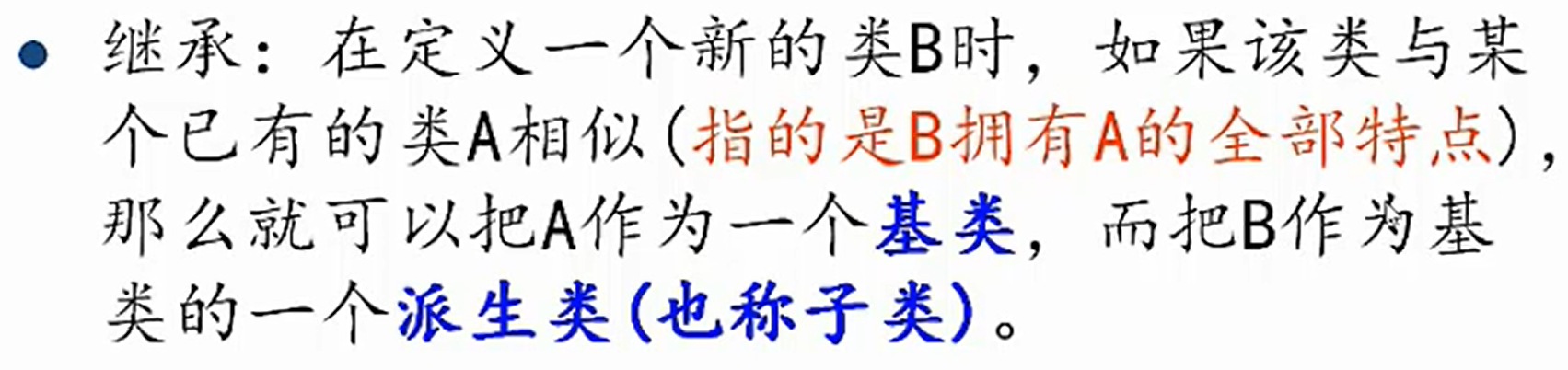
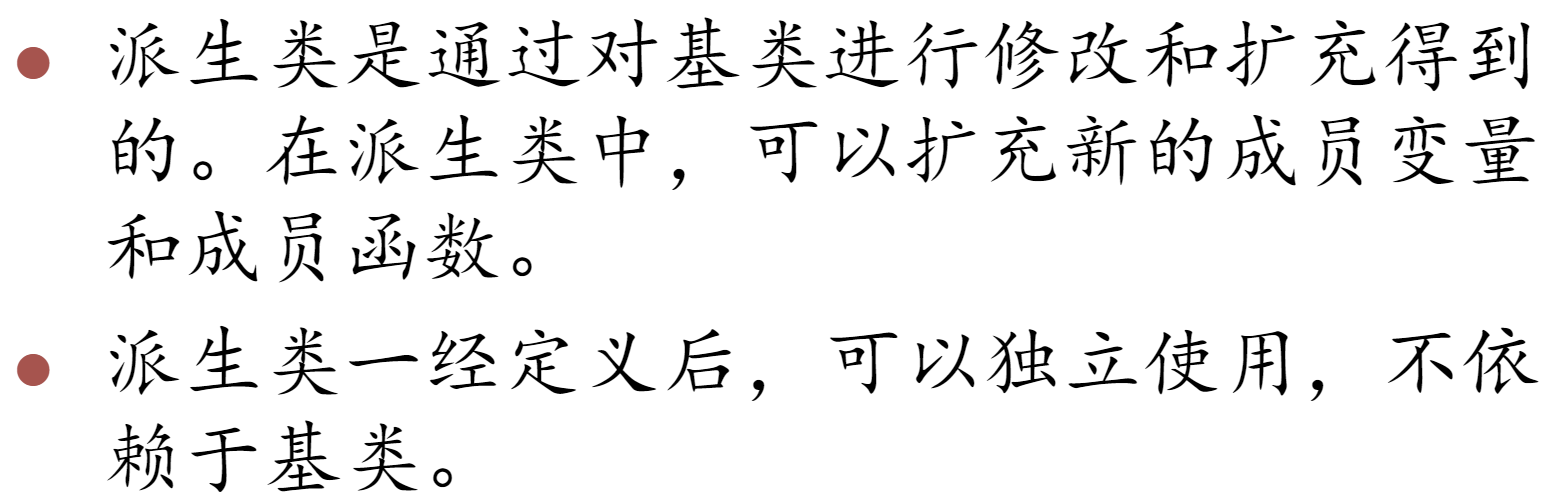
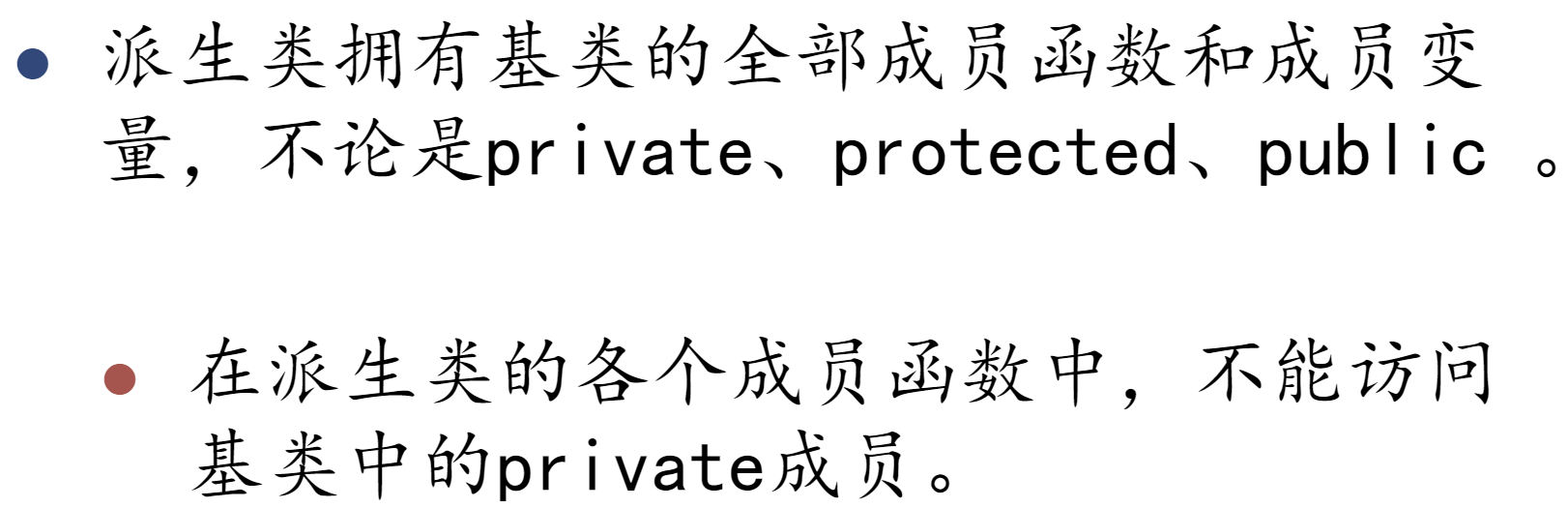
#include <bits/stdc++.h>
using namespace std;
//Dividing Line----------------------------------------------------------------------------------------
class CStudent
{
private:
string name;
string id;
char gender;
int age;
public:
void SetInfo(const string &, const string &, const char &, const int &);
void PrintInfo(void);
};
void CStudent::SetInfo(const string &_name, const string &_id, const char &_gender, const int &_age)
{
name = _name;
id = _id;
gender = _gender;
age = _age;
}
void CStudent::PrintInfo(void)
{
cout << "Name: " << name << endl;
cout << "Id: " << id << endl;
cout << "Gender: " << gender << endl;
cout << "Age: " << age << endl;
}
//Dividing Line--------------------------------------------------------------------------
class CUnderGraduate : public CStudent
{
private:
string major;
public:
void SetInfo(const string &, const string &, const char &, const int &, const string &);
void PrintInfo(void);
};
void CUnderGraduate::SetInfo(const string &_name, const string &_id, const char &_gender, const int &_age, const string &_major)
{
CStudent::SetInfo(_name, _id, _gender, _age);
major = _major;
}
void CUnderGraduate::PrintInfo(void)
{
CStudent::PrintInfo();
cout << "Major: " << major << endl;
}
//Dividing Line-----------------------------------------------------------------------------------------------------
int main(void)
{
CUnderGraduate c;
c.SetInfo("Hermann", "21200319", 'M', 19, "Computer Science");
c.PrintInfo();
return 0;
}