运算符重载的需求
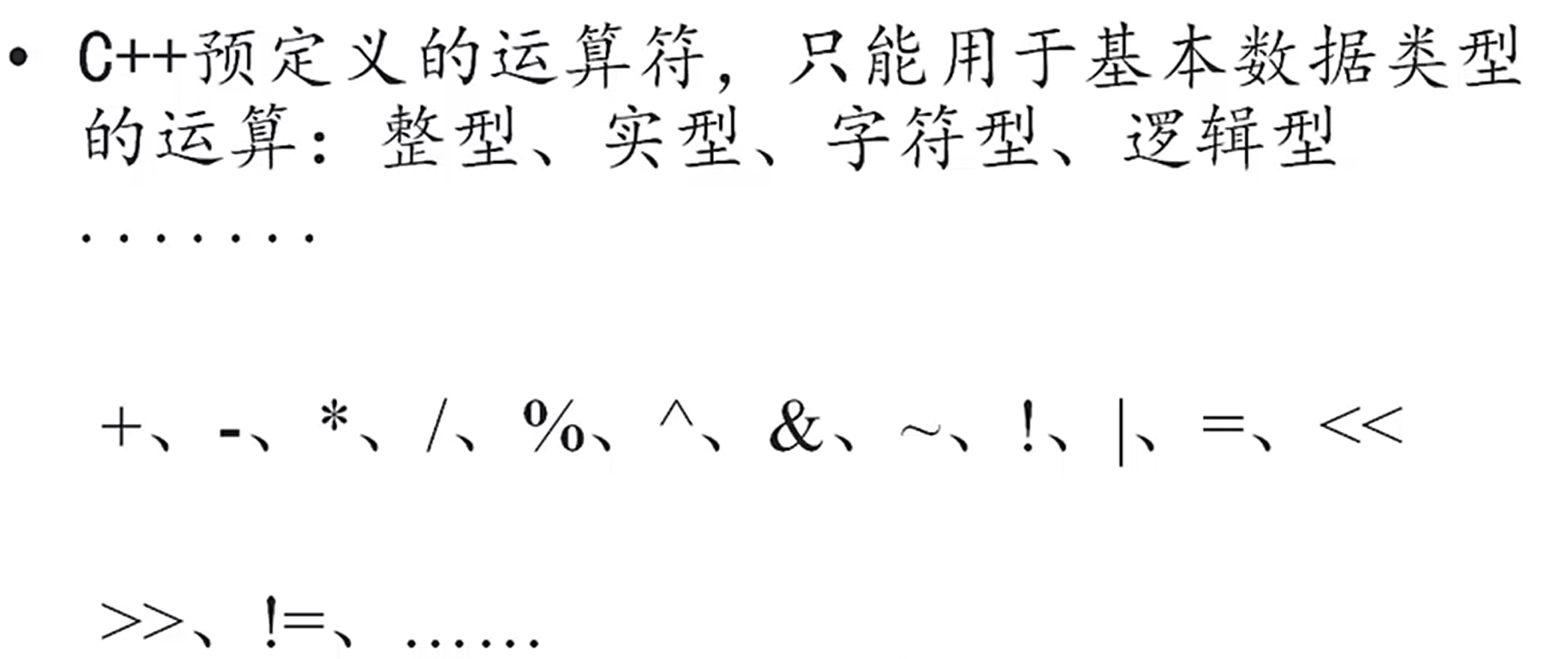
运算符重载的概念
运算符重载的形式
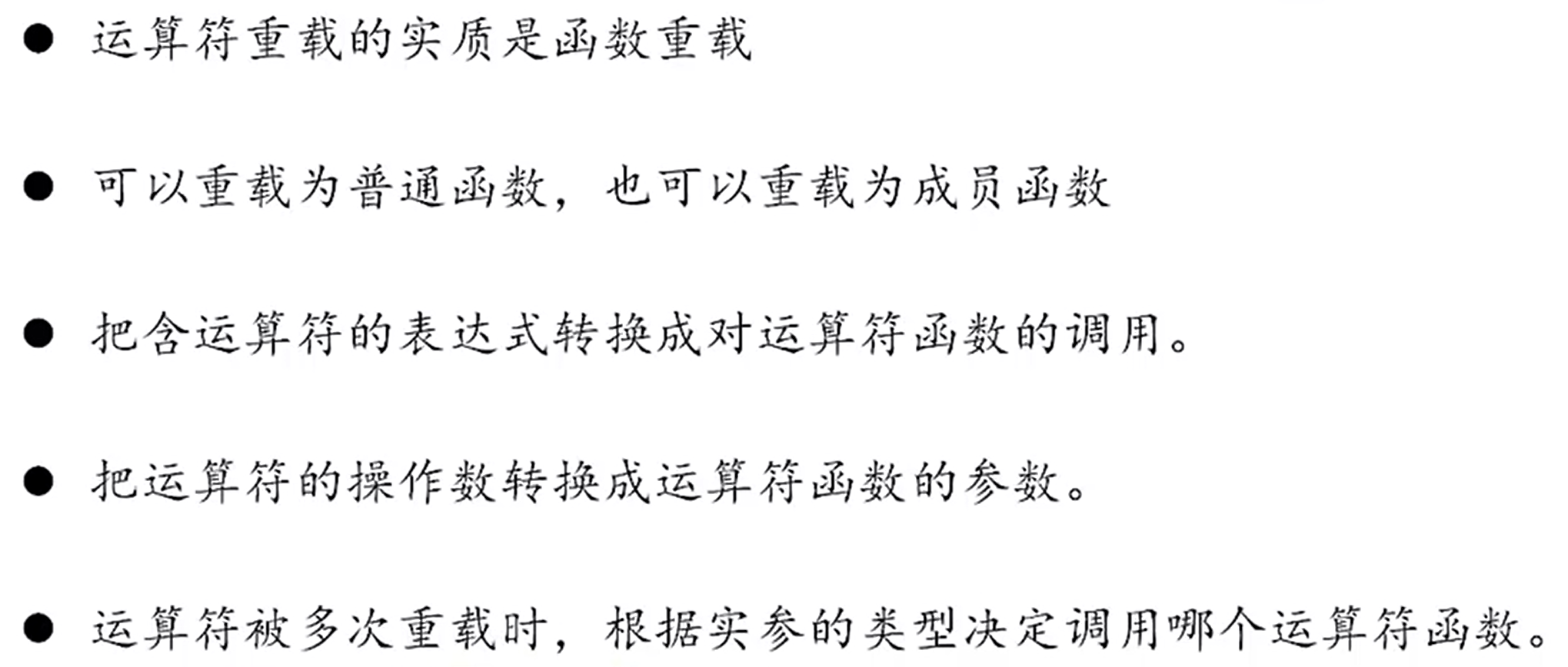
#include <bits/stdc++.h>
using namespace std;
class Complex
{
public:
double real, imag;
Complex(double, double);
Complex operator-(const Complex &);//重载为成员函数时,参数个数=运算符目数-1
};
Complex::Complex(double r, double i)
{
real = r;
imag = i;
}
Complex operator+(const Complex &c1, const Complex &c2)
{
return Complex(c1.real + c2.real, c1.imag + c2.imag);//返回临时对象
}//重载为外部普通函数时,参数个数=运算符目数
Complex Complex::operator-(const Complex &c)
{
return Complex(real - c.real, imag - c.imag);
}
int main(void)
{
Complex c1(4, 4), c2(1, 1);
Complex c3 = c1 + c2;//等价于c3=operator+(c1,c2)
Complex c4 = c1 - c2;//等价于c4=c1.operator-(c2)
cout << c3.real << ends << c3.imag << endl;// 5 5
cout << c4.real << ends << c4.imag << endl;// 3 3
return 0;
}