3D Computer Game Programming-Note 3
1、简答并用程序验证
- 游戏对象运动的本质是什么?
游戏对象运动的本质是游戏对象的空间属性的变化,通过矩阵变换(平移、旋转、缩放)实现。
- 请用三种方法以上方法,实现物体的抛物线运动。
方法一:直接修改物体的 Transform 的属性 position。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Sports1 : MonoBehaviour {
private float Xspeed = 2.0f;
private float Yspeed = 0;
private float gravity = 9.8f;
// Start is called before the first frame update
void Start() {
}
// Update is called once per frame
void Update() {
this.transform.position += Xspeed * Vector3.left * Time.deltaTime;
this.transform.position += Yspeed * Vector3.down * Time.deltaTime;
Yspeed += gravity * Time.deltaTime;
}
}
方法二:使用 Transform 的方法 Translate。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Sports2 : MonoBehaviour {
private float Xspeed = 2.0f;
private float Yspeed = 0;
private float gravity = 9.8f;
// Start is called before the first frame update
void Start() {
}
// Update is called once per frame
void Update() {
this.transform.Translate(Xspeed * Time.deltaTime, 0, 0);
this.transform.Translate(0, -Yspeed * Time.deltaTime, 0, Space.World);
Yspeed += gravity * Time.deltaTime;
}
}
方法三:使用 Vector3 的方法构造向量。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Sports3 : MonoBehaviour {
private float Xspeed = 2.0f;
private float Yspeed = 0;
private float gravity = 9.8f;
// Start is called before the first frame update
void Start() {
}
// Update is called once per frame
void Update() {
Vector3 myVector = new Vector3(Xspeed * Time.deltaTime, -Yspeed * Time.deltaTime, 0);
this.transform.position += myVector;
Yspeed += gravity * Time.deltaTime;
}
}
写一个程序,实现一个完整的太阳系,其他星球围绕太阳的转速必须不一样,且不在一个法平面上。
在编写脚本前先创建所有天体对应的游戏对象,使八大行星成为太阳的子对象:
编写脚本 SolarSystem.cs,使用 RotateAround;使用Rotate实现天体自转;参数参考真实宇宙大致给出:
```csharp using System.Collections; using System.Collections.Generic; using UnityEngine;
public class SolarSystem : MonoBehaviour { public Transform Sun; public Transform Mercury; public Transform Venus; public Transform Earth; public Transform Mars; public Transform Jupiter; public Transform Saturn; public Transform Uranus; public Transform Neptune;
// Start is called before the first frame update
void Start() {
//初始化天体的位置
Sun.position = Vector3.zero;
Mercury.position = new Vector3(0.9f, 0, 0);
Venus.position = new Vector3(1.5f, 0, 0);
Earth.position = new Vector3(2.2f, 0, 0);
Mars.position = new Vector3(3.0f, 0, 0);
Jupiter.position = new Vector3(4.0f, 0, 0);
Saturn.position = new Vector3(5.2f, 0, 0);
Uranus.position = new Vector3(6.5f, 0, 0);
Neptune.position = new Vector3(7.8f, 0, 0);
//调整天体的大小
Sun.localScale += new Vector3(0.5f, 0.5f, 0.5f);
Mercury.localScale -= new Vector3(0.5f, 0.5f, 0.5f);
Venus.localScale -= new Vector3(0.4f, 0.4f, 0.4f);
Earth.localScale -= new Vector3(0.4f, 0.4f, 0.4f);
Mars.localScale -= new Vector3(0.5f, 0.5f, 0.5f);
Saturn.localScale -= new Vector3(0.05f, 0.05f, 0.05f);
Uranus.localScale -= new Vector3(0.2f, 0.2f, 0.2f);
Neptune.localScale -= new Vector3(0.2f, 0.2f, 0.2f);
}
// Update is called once per frame
void Update() {
//RotateAround 方法模拟天体绕太阳公转,Rotate 方法模拟天体自转
Mercury.RotateAround(Sun.position, new Vector3(0, 1, 2), 48 * Time.deltaTime);
Mercury.Rotate(Vector3.up * 30 * Time.deltaTime);
Venus.RotateAround(Sun.position, new Vector3(0, 1, -1), 35 * Time.deltaTime);
Venus.Rotate(Vector3.up * 30 * Time.deltaTime);
Earth.RotateAround(Sun.position, Vector3.up, 30 * Time.deltaTime);
Earth.Rotate(Vector3.up * 30 * Time.deltaTime);
Mars.RotateAround(Sun.position, new Vector3(0, 1, 1), 24 * Time.deltaTime);
Mars.Rotate(Vector3.up * 30 * Time.deltaTime);
Jupiter.RotateAround(Sun.position, new Vector3(0, 5, 1), 13 * Time.deltaTime);
Jupiter.Rotate(Vector3.up * 30 * Time.deltaTime);
Saturn.RotateAround(Sun.position, new Vector3(0, 4, 1), 10 * Time.deltaTime);
Saturn.Rotate(Vector3.up * 30 * Time.deltaTime);
Uranus.RotateAround(Sun.position, new Vector3(0, 6, 1), 7 * Time.deltaTime);
Uranus.Rotate(Vector3.up * 30 * Time.deltaTime);
Neptune.RotateAround(Sun.position, new Vector3(0, 2, 1), 5 * Time.deltaTime);
Neptune.Rotate(Vector3.up * 30 * Time.deltaTime);
}
}
- 将脚本拖放到 Main Camera,并设置相应的公有变量,运行:<br />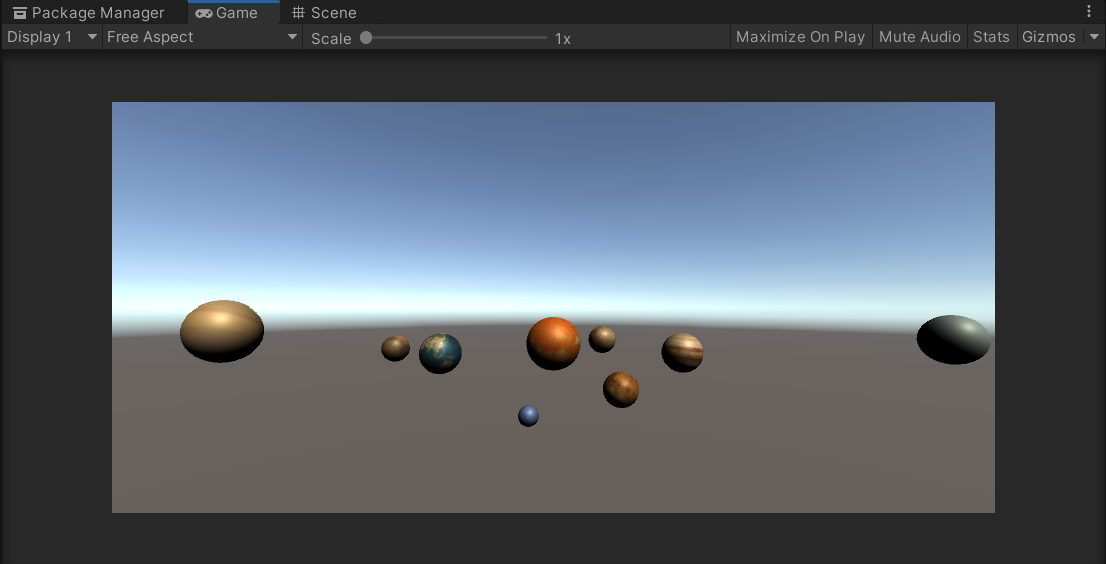
- 为所有天体添加部件 Trail Renderer,可以在运行时显示天体运动轨迹:<br />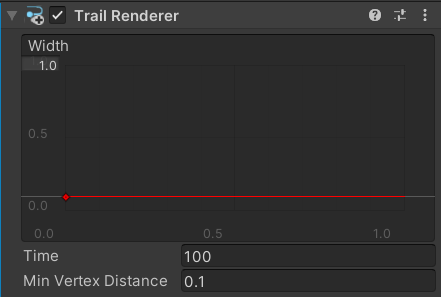
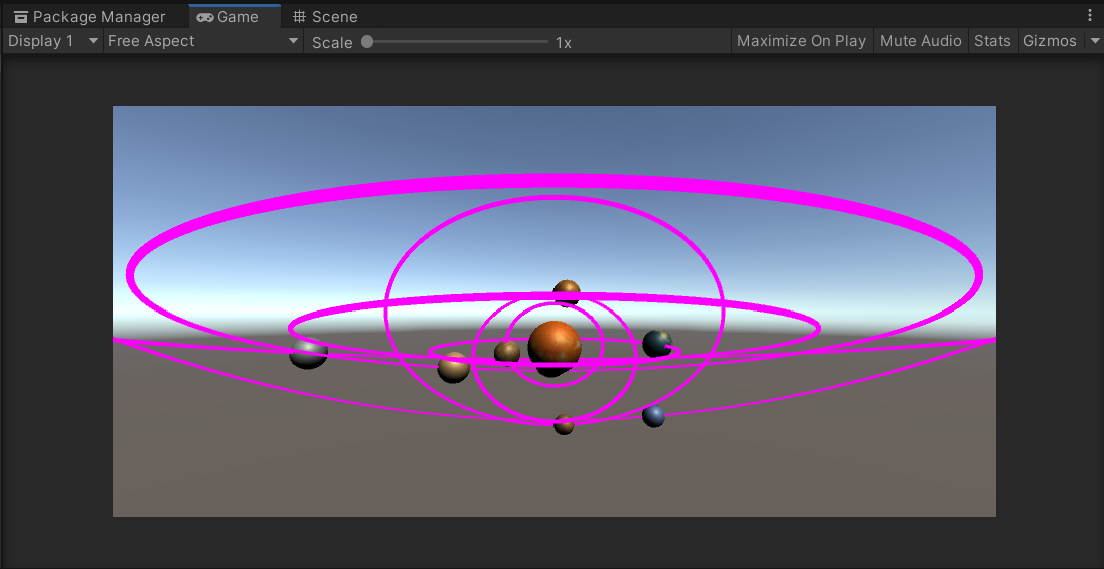
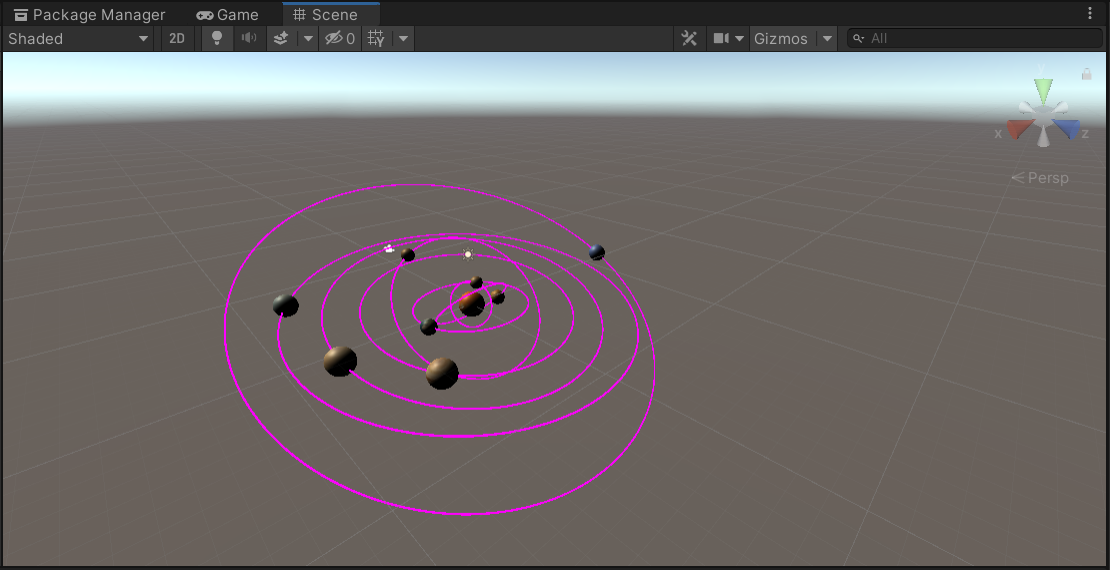
游戏成果动态展示[🔗视频链接](https://www.bilibili.com/video/BV1R54y117nB/)
2、编程实践
- 阅读以下游戏脚本<br />
Priests and Devils
Priests and Devils is a puzzle game in which you will help the Priests and Devils to cross the river within the time limit. There are 3 priests and 3 devils at one side of the river. They all want to get to the other side of this river, but there is only one boat and this boat can only carry two persons each time. And there must be one person steering the boat from one side to the other side. In the flash game, you can click on them to move them and click the go button to move the boat to the other direction. If the priests are out numbered by the devils on either side of the river, they get killed and the game is over. You can try it in many ways. Keep all priests alive! Good luck!
- 列出游戏中提及的事物(Objects)<br />牧师(Priest)、恶魔(Devil)、船(boat)、河(river)、河岸(sides of river)。
- 用表格列出玩家动作表(规则表)
| 玩家动作(事件) | 条件 | 结果 |
| --- | --- | --- |
| 点击牧师/恶魔 | 牧师/恶魔在岸上且船未满员 | 牧师/恶魔上船 |
| 点击牧师/恶魔 | 牧师/恶魔在船上且船靠岸 | 牧师/恶魔上岸 |
| 点击船 | 船上至少有一个人物 | 船驶向对岸 |
| / | 三个牧师均已过河 | 游戏胜利 |
| / | 有一侧河岸魔鬼数量多于牧师数量 | 游戏失败 |
- 编程要求<br />
请将游戏中对象做成预制 在场景控制器 LoadResources 方法中加载并初始化长方形、正方形、球及其色彩代表游戏中的对象 使用 C# 集合类型有效组织对象 整个游戏仅主摄像机和一个 Empty 对象,其他对象必须代码动态生成整个游戏不许出现 Find 游戏对象,SendMessage 这类突破程序结构的通讯耦合语句 请使用课件架构图编程,不接受非 MVC 结构程序 注意细节,例如:船未靠岸,牧师与魔鬼上下船运动中,均不能接受用户事件
- **编程实现**
【游戏效果图】<br /> 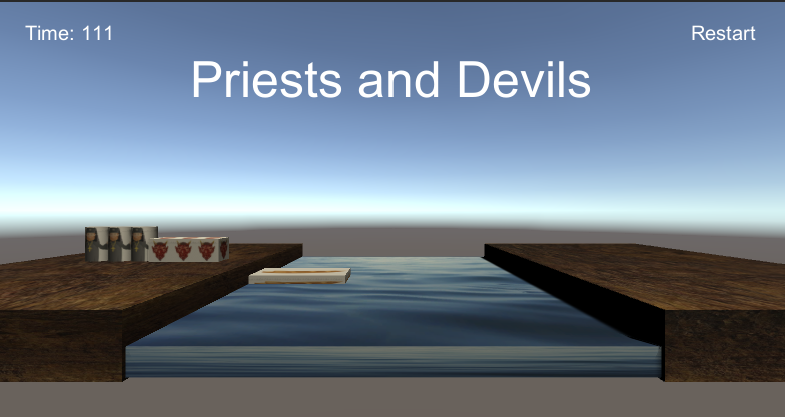
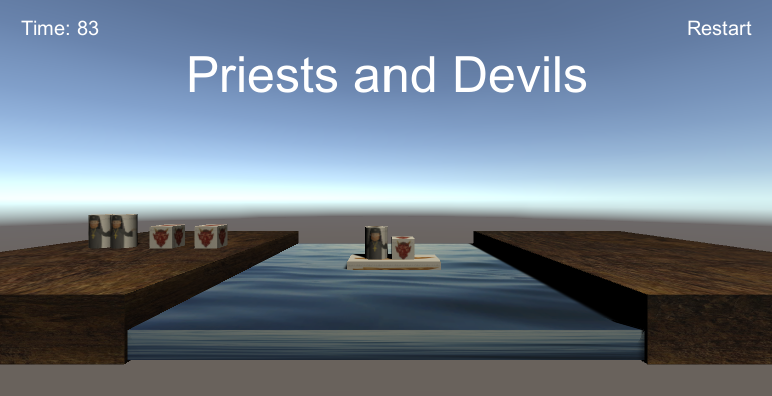
【MVC架构设计】
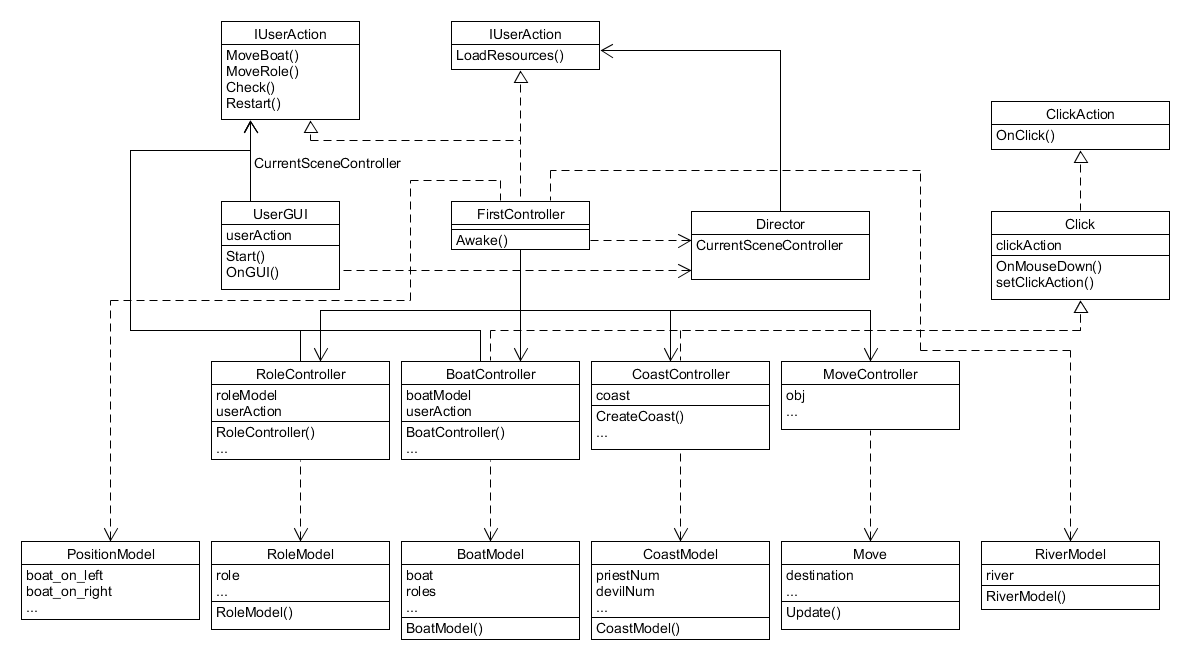
【游戏对象预制】
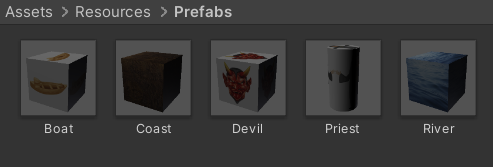
【脚本实现】
以下按照 MVC 架构三个部分介绍,受篇幅影响此处省去代码细节,完整代码参见个人 [🔗github](https://github.com/sherryjw/3D-Computer-Game-Programming/tree/master/Homework3)。
①.模型(Model):主要处理数据对象及关系(包括游戏对象、空间关系等)
**CoastModel.cs**:处理游戏对象——河岸<br />
```csharp
public class CoastModel {
public GameObject obj;
public int priestNum, devilNum;
public CoastModel(string name, Vector3 position) {
}
}
BoatModel.cs:处理游戏对象——船
public class BoatModel {
public GameObject boat;
public RoleModel[] roles;
public int priestNum;
public int devilNum;
public bool OnRight;
public BoatModel(Vector3 position) {
}
}
RiverModel.cs:处理游戏对象——河
public class RiverModel {
private GameObject river;
public RiverModel(Vector3 position) {
}
}
RoleModel.cs:处理游戏对象——角色:牧师、魔鬼
public class RoleModel {
public GameObject role;
public int tag;
public int flag;
public bool OnBoat;
public bool OnRight;
public RoleModel(Vector3 position, int flag, int tag) { // 0 represents Priest, 1 represents Devil
}
}
PositionModel.cs:处理游戏对象的空间位置
public class PositionModel
{
public static Vector3 src_coast = new Vector3(-11, -2, 6);
public static Vector3 des_coast = new Vector3(11, -2, 6);
public static Vector3 river = new Vector3(1, -2.4f, 6);
public static Vector3 boat_on_left = new Vector3(-4, -2, 6);
public static Vector3 boat_on_right = new Vector3(4, -2, 6);
public static Vector3[] roles = new Vector3[]{new Vector3(-0.2f, 0.8f, 0), new Vector3(-0.1f, 0.8f, 0), new Vector3(0, 0.8f, 0),
new Vector3(0.1f, 0.8f,0), new Vector3(0.2f, 0.8f, 0), new Vector3(0.3f, 0.8f, 0)};
public static Vector3[] roles_on_boat = new Vector3[] { new Vector3(-0.1f, 1.2f, 0), new Vector3(0.2f, 1.2f, 0) };
}
②.控制器(Controller):接受用户事件,控制模型的变化
- 一个场景一个主控制器
- 至少实现与玩家交互的接口
- 实现或管理运动
Director.cs:获取当前游戏的场景,控制场景运行、切换、入栈与出栈
public class Director : System.Object {
private static Director _instance;
public ISceneController CurrentSenceController { get; set; }
public static Director GetInstance() {
}
}
CoastController.cs:处理与河岸相关的事件,包括牧师/魔鬼上岸、离岸等
public class CoastController {
private CoastModel coast;
public void CreateCoast(string name, Vector3 position) {
}
public Vector3 AddRole(RoleModel roleModel) {
}
public CoastModel GetCoastModel() {
}
public void RemoveRole(RoleModel roleModel) {
}
}
BoatController.cs:处理与船相关的事件,包括牧师/恶魔上船、下船等
public class BoatController : ClickAction {
BoatModel boatModel;
IUserAction userAction;
public BoatController() {
}
public void CreateBoat(Vector3 position) {
}
public Vector3 AddRole(RoleModel roleModel) {
}
public BoatModel GetBoatModel() {
}
public void RemoveRole(RoleModel roleModel) {
}
public void OnClick() {
}
}
RoleController.cs:处理与游戏角色——牧师和魔鬼相关的事件,包括点击角色等
public class RoleController : ClickAction {
RoleModel roleModel;
IUserAction userAction;
public RoleController() {
}
public void CreateRole(Vector3 position, int flag, int tag) {
}
public RoleModel GetRoleModel() {
}
public void OnClick() {
}
}
MoveController.cs:处理与移动相关的事件,包括船离岸、靠岸等
public class MoveController {
private GameObject obj;
public void SetMove(Vector3 destination, GameObject obj) {
}
public bool GetIsMoving() {
}
}
FirstController.cs:管理本次场景所有的游戏对象,协调游戏对象之间的通讯等
public class FirstController : MonoBehaviour, ISceneController, IUserAction {
private CoastController DesCoastController;
private CoastController SrcCoastController;
private BoatController boatController;
private RoleModelController[] roleModelControllers;
private MoveController moveController;
private RiverModel river;
private bool isRuning;
private float time;
void Awake() {
}
public void LoadResources() {
}
public void MoveBoat() {
}
public void MoveRole(RoleModel roleModel) {
}
public void Restart() {
}
public void Check() {
}
void Update() {
}
}
ISceneController.cs:提供实现资源加载的接口
public interface ISceneController {
void LoadResources();
}
IUserAction.cs:提供用户交互事件的接口
public interface IUserAction {
void MoveBoat();
void MoveRole(RoleModel roleModel);
void Check();
void Restart();
}
ClickAction.cs:提供点击事件的接口
public interface ClickAction {
void OnClick();
}
Move.cs:处理每一帧的场景变化
public class Move : MonoBehaviour {
public bool isMoving = false;
public float speed = 8;
public Vector3 destination;
void Update() {
}
}
③.界面(View):显示模型,将人机交互事件交给控制器处理
- 处理接收 Input 事件
- 渲染 GUI ,接收事件
Click.cs:接收点击事件并交给控制器处理
public class Click : MonoBehaviour {
ClickAction clickAction;
void OnMouseDown() {
}
public void setClickAction(ClickAction clickAction) {
}
}
UserGUI.cs:渲染 GUI,并接收事件
public class UserGUI : MonoBehaviour {
private IUserAction userAction;
public int time;
public string result;
void Start() {
}
void OnGUI() {
}
}
【游戏成果动态展示】
3、思考题
- 使用向量与变换,实现并扩展 Tranform 提供的方法,如 Rotate、RotateAround 等。
实现如下形式的 Rotate:
public void Rotate(Vector3 axis, float angle, Transform t);
可以使用函数public static Quaternion AngleAxis(float angle, Vector3 axis);
来实现,该函数创建一个绕轴 axis 成 角度 angle 的旋转。为了实现 Rotate 效果的呈现,还需要与 t 点乘:
public void Rotate(float angle, Vector3 axis, Transform t) {
var a = Quaternion.AngleAxis(angle, axis);
t.rotation *= a;
}
实现如下形式的 RotateAround:
public void RotateAround(Vector3 point, Vector3 axis, float angle, Transform t);
同样使用函数public static Quaternion AngleAxis(float angle, Vector3 axis);
来实现,由于是围绕一个中心点旋转,需要计算物体的位移矢量,对其进行旋转后再计算得到新的位置:
public void RotateAround(Vector3 point, Vector3 axis, float angle, Transform t) {
var a = Quaternion.AngleAxis(angle, axis);
var distance = t.position - point;
distance *= a;
t.position = distance + point;
t.rotation *= a;
}