一、Sentinel
https://github.com/alibaba/Sentinel 中文
Sentinel 是轻量级的流量控制、熔断降级Java库;功能类似于Hystrix
下载地址
怎么玩:
入门文档
服务使用中的各种问题:服务雪崩、服务降级、服务熔断、服务限流
二、安装Sentinel控制台
Sentinel分为两个部分:
- 核心库(Java客户端)不依赖任何框架/库,能够云星宇所有Java运行时环境,同时对Dubbo/Spring Cloud等框架也有较好的支持——后台;
- 控制台(Dashboard)基于Spring Boot开发,打包后可以直接运行,不需要额外的Tomcat等应用容器——前台 8080
安装步骤
下载到本地sentinel-dashboard-1.8.2.jar
运行命令:
前提需要Java8,且8080端口不能被占用;java -jar sentinel-dashboard-1.8.2.jar
访问 localhost:8080,账号密码均为sentinel
三、初始化演示工程
3.1 启动Nacos8848
3.2 新建Module cloudalibaba-sentinel-service8401
3.2.1 pom
以后基本上nacos 跟sentinel一起配置
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>jdk8cloud2021</artifactId>
<groupId>com.atguigu.springcloud</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>cloudalibaba-sentinel-service8401</artifactId>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<dependencies>
<!--SpringCloud ailibaba nacos -->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<!--SpringCloud ailibaba sentinel-datasource-nacos 后续做持久化用到-->
<dependency>
<groupId>com.alibaba.csp</groupId>
<artifactId>sentinel-datasource-nacos</artifactId>
</dependency>
<!--SpringCloud ailibaba sentinel -->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-sentinel</artifactId>
</dependency>
<!--openfeign-->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
<!-- SpringBoot整合Web组件+actuator -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<!--日常通用jar包配置-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>4.6.3</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
</project>
3.2.2 yaml
spring.cloud.sentinel.transport.port 端口配置会在应用对应的机器上启动一个 Http Server,该 Server 会与 Sentinel 控制台做交互。
比如 Sentinel 控制台添加了1个限流规则,会把规则数据push给这个Http Server接收,Http Server再将规则注册到Sentinel中。
spring.cloud.sentinel.transport.port:指定与Sentinel控制台交互的端口,应用本地会启动一个占用该端口的Http Server
server:
port: 8401
spring:
application:
name: cloudalibaba-sentinel-service
cloud:
nacos:
discovery:
server-addr: localhost:8848 #Nacos服务注册中心地址
sentinel:
transport:
#配置Sentinel dashboard地址
dashboard: localhost:8080
#默认8719端口,假如被占用会自动从8719开始依次+1扫描,直至找到未被占用的端口
port: 8719
management:
endpoints:
web:
exposure:
include: '*'
3.2.3 主启动类
package com.atguigu.cloudalibaba;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
@EnableDiscoveryClient
@SpringBootApplication
public class SentinelMainApp8401 {
public static void main(String[] args) {
SpringApplication.run(SentinelMainApp8401.class, args);
}
}
3.2.4 业务类
流量控制controller:FlowLimitController
package com.atguigu.cloudalibaba.controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class FlowLimitController {
@GetMapping("/testA")
public String testA() {
return "------testA";
}
@GetMapping("/testB")
public String testB() {
return "------testB";
}
}
3.3 测试
启动Sentinel8080 java -jar sentinel-dashboard-1.8.2.jar
、启动微服务8401
查看Sentinel控制台,发现什么也没有。
原因:Sentinel采用懒加载机制
执行一下:http://localhost:8401/testA
sentinel8080正在监控微服务8401
四、流控规则
4.1 流控模式
4.1.1 直接(默认)+快速失败(默认)
(1) QPS直接快速失败
QPS:query per second,每秒钟的请求数量,当调用该api的QPS达到阈值时,进行限流。
下面设置表示1秒钟内查询一次就是OK,若QPS>1,就直接-快速失败,报默认错误
测试一下,当/testA的访问超过1次/s是,页面报错。被Sentinel限流,还能继续请求只要QPS<=1。
小结:表示1秒钟内请求次数大于1,就直接快速失败,报默认错误。
直接调用默认的报错信息在技术上是OK的,但是是否应该有自定义的后续处理?应该有类似Hystrix的fallback的兜底方法。
(2) 线程数直接快速失败
当调用该api的线程数达到阈值的时候,进行限流。
与QPS直接快速失败不同的是,QPS情况下限制的是流量,比如银行的人流量只能是1人/s,也就是说每次只能一个人进入银行办理业务;而线程数就好比银行只有一个窗口开放,一群人都可以进入银行,但是每次只能处理一个人的业务。
演示效果:
先修改一下8401的业务类
然后重启8401,测试/testA,最好用两个浏览器访问,效果更明显
4.1.2 关联
当关联的资源达到阈值时,就限流自己。比如当与A关联的资源B达到阈值后,就限流A自己。
支付接口达到阈值,限流下订单的接口。
1. 配置
设置效果:当关联资源/testB的qps阀值超过1时,就限流/testA的Rest访问地址,当关联资源到阈值后限制配置好的资源名
2. 测试
单独访问testB成功。
postman模拟并发密集访问testB
先创建一个集合,名字自己随便取。
然后将创建的访问/testB的请求保存在创建的集合中
设定集合运行参数,20个线程,每次间隔0.3s访问一次(QPS>1),执行:
然后再访问/testA,发现被限流,等postman执行完毕,testA又可以访问了
4.1.3 链路
需要测试链路的话,springcloud 阿里巴巴版本需要2.1.1.RELEASE以上,在父工程的pom中修改,不要直接在子module的pom中修改,版本有对应关系,不然报错。
- Sentinel从1.6.3版本开始,Sentinel Web Filter 默认收敛所有的URL入口的Context,因此链路限流不生效
- 1.7.0版本开始,官方在CommomFilter中引入了
WEB_CONTEXT_UNIFY
这个init parameter,用于控制是否收敛context,将其配置为false
即可根据不同的URL进行链路限流 - Spring Cloud Alibaba 在2.1.1.RELEASE版本后,可以通过配置spring.cloud.sentinel.web-context-unify=false关闭
https://github.com/alibaba/Sentinel/issues/1313
测试
启动8401,给/testA设置链路+快速失败流控规则:
这里入口资源就是簇点链路中,资源名称的上一级。
访问http://localhost:8401/linktestA ,多次刷新出现限流,但是这种情况我个人觉得跟直接快速失败区别不大,只直接是监控/testA资源,而链路是监控/testA的资源入口sentinel_web_servlet_context。
然后我又参看了其他博客,流控模式——链路。增加了FlowLimitService 修改了controller
package com.atguigu.cloudalibaba.service;
import com.alibaba.csp.sentinel.annotation.SentinelResource;
import org.springframework.stereotype.Service;
@Service
public class FlowLimitService {
@SentinelResource("message")
public String message() {
return "success";
}
}
package com.atguigu.cloudalibaba.controller;
import com.atguigu.cloudalibaba.service.FlowLimitService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.concurrent.TimeUnit;
@RestController
public class FlowLimitController {
@Autowired
FlowLimitService flowLimitService;
@GetMapping("/testA")
public String testA() {
//暂停0.8秒
// try {
// TimeUnit.MILLISECONDS.sleep(800);
// } catch (InterruptedException e) {
// e.printStackTrace();
// }
return "------testA";
}
@GetMapping("/testB")
public String testB() {
return "------testB";
}
// 链路测试
@GetMapping("/linktestA")
public String linktestA() {
return flowLimitService.message();
}
@GetMapping("/linktestB")
public String linktestB() {
return flowLimitService.message();
}
}
分别通过/linktetA 和 /linktestB都是message的入口,然后设置/linktestA入口的流量限制,发现不起作用。。。
4.2 流控效果
快速失败在上面的流控模式演示过了,他是默认的流控效果,直接失败,抛出异常。源码:com.alibaba.csp.sentinel.slots.block.flow.controller.DefaultController
4.2.1 warm up 预热
官网 源码:com.alibaba.csp.sentinel.slots.block.flow.controller.WarmUpController
公式:阈值除以coldFactor(默认值为3),经过预热时长后才会达到阈值
测试
狂点请求,可以看通过的QPS逐渐增加,最开始会报错限流,之后就可以抗住10/s的QPS了
应用场景:秒杀系统在开启的瞬间,会有很多流量上来,很有可能把系统打死,预热方式就是把为了保护系统,可慢慢的把流量放进来,慢慢的把阀值增长到设置的阀值。
4.2.2 排队等待
官网 源码:com.alibaba.csp.sentinel.slots.block.flow.controller.RateLimiterController
匀速排队,让请求以均匀的速度通过,阀值类型必须设成QPS,否则无效。
/testA的QPS最大为1,超过的话就排队等待,等待的超时时间为20000ms。
测试
postman:
可以看到刚好满足1s一个请求,说明请求的执行进行了排队。
五、降级规则(熔断规则)
官网
老版本的Sentinel的断路器是没有半开状态的,半开的状态系统自动去检测是否请求有异常,没有异常就关闭断路器恢复使用,有异常则继续打开断路器不可用。具体可以参考Hystrix。(在Hystrix中 快照时间窗口是值 阈值检测时间 ,而休眠时间窗口是指 断路器从开启到半开状态间隔的时间)
新版本的Sentinel加入了半开状态!
5.1 降级策略
Sentinel 熔断降级会在调用链路中某个资源出现不稳定状态时(例如调用超时或异常比例升高),对这个资源的调用进行限制,让请求快速失败,避免影响到其它的资源而导致级联错误。
当资源被降级后,在接下来的降级时间窗口之内,对该资源的调用都自动熔断(默认行为是抛出 DegradeException)。
5.1.1 慢调用比例,RT(平均响应时间,秒级)
老版本:
新版本:
- 慢调用比例 (SLOW_REQUEST_RATIO):选择以慢调用比例作为阈值,需要设置允许的慢调用 RT(即最大的响应时间),请求的响应时间大于该值则统计为慢调用。当单位统计时长(statIntervalMs)内请求数目大于设置的最小请求数目,并且慢调用的比例大于阈值,则接下来的熔断时长内请求会自动被熔断。经过熔断时长后熔断器会进入探测恢复状态(HALF-OPEN 状态),若接下来的一个请求响应时间小于设置的慢调用 RT 则结束熔断,若大于设置的慢调用 RT 则会再次被熔断。(跟豪猪科类似)
实战测试
业务类中加一个rest 接口,以用于测试:
@GetMapping("/testD")
public String testD() {
//暂停1秒
try {
TimeUnit.MILLISECONDS.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
log.info("testD 测试慢调用比例 RT");
return "------tedtD";
}
访问没有问题
编辑熔断规则:
在1000ms的统计时间内,总请求数(超过5次)中有80%的请求最大RT超过了200ms,那么触发熔断机制,熔断2s。
jmeter压测:
永远一秒钟打进来10个线程(大于5个了)调用testD,我们希望200毫秒处理完本次任务,如果超过200毫秒还没处理完,在未来s秒钟的时间内,断路器打开(保险丝跳闸)微服务不可用,保险丝跳闸断电了。
testD被熔断了
从实时监控也可以看到,在09的时候开始熔断。
后续我停止jmeter,没有这么大的访问量了,断路器半开到关闭(保险丝恢复),微服务恢复OK。
5.1.2 异常比例
老版本:
新版本:
- 异常比例 (ERROR_RATIO):当单位统计时长(statIntervalMs)内请求数目大于设置的最小请求数目,并且异常的比例大于阈值,则接下来的熔断时长内请求会自动被熔断。经过熔断时长后熔断器会进入探测恢复状态(HALF-OPEN 状态),若接下来的一个请求成功完成(没有错误)则结束熔断,否则会再次被熔断。异常比率的阈值范围是 [0.0, 1.0],代表 0% - 100%。
实战测试
修改业务类:
编辑熔断规则:
1000ms统计时长内,大于5次的请求中超过80%的请求出现异常,则熔断2s。
jmeter压测:
单独访问一次,必然来一次报错一次(int age = 10/0),调一次错一次;开启jmeter后,直接高并发发送请求,多次调用达到我们的配置条件了。断路器开启(保险丝跳闸),微服务不可用了,不再报错error而是服务降级了。testD被熔断。
停掉jemeter后过2s。报/zero错误,因为业务类中有个10/0。
5.1.3 异常数
老版本:
时间窗口一定要大于等于60秒。
新版本
- 异常数 (ERROR_COUNT):当单位统计时长内的异常数目超过阈值之后会自动进行熔断。经过熔断时长后熔断器会进入探测恢复状态(HALF-OPEN 状态),若接下来的一个请求成功完成(没有错误)则结束熔断,否则会再次被熔断。
实战测试
修改业务类:
编辑熔断规则:
手动测试:
这里我测试有bug,虽然熔断了但是熔断时长不是我配置的5s,大约是一分钟,统计时长也不是1s,好像也是一分钟,同时没达到最小请求数,只达到3次异常就直接熔断了。虽然我用的新版本,但是逻辑好像跟老版本的一样?
六、热点key限流
6.1 基本介绍
官网
何为热点:热点即经常访问的数据,很多时候我们希望统计或者限制某个热点数据中访问频次最高的TopN数据,并对其访问进行限流或者其它操作。比如:
- 商品 ID 为参数,统计一段时间内最常购买的商品 ID 并进行限制
- 用户 ID 为参数,针对一段时间内频繁访问的用户 ID 进行限制
热点参数限制会统计传入参数中的热点参数,并根据配置的限流阈值与模式,对包含热点参数的资源调用进行限制。热点参数限流可以看作是一种特殊的流量控制,仅对包含热点参数的资源调用生效。
Sentinel利用LRU策略统计最近最常访问的热电参数,结合令牌桶算法来进行参数级别的流控。热点参数限流支持集群模式。
6.2 基本使用
兜底防范分为系统默认和客户自定义;两种,根据之前的case,都是使用sentinel系统默认的提示:Blocked by Sentinel (flow limiting)。那我们能不能自定义兜底方法呢?类似hystrix,某个方法出问题了,就找对应的兜底降级方法?
类似于@HystrixCommand, 引入@SentinelResource注解。
热点规则共有资源名、限流模式(只支持QPS模式)、参数索引、单机阈值、统计窗口时长、是否集群6种参数,还有一些高级选项,用到时会详细介绍。这里会用到注解中的value作为资源名,兜底方法会在后面详细介绍@SentinelResource注解详解
注意:
资源名:唯一路径,默认为请求路径。此处必须是 @SentinelResource 注解的 value 属性值,配置@GetMapping 的请求路径无效)
6.2.1 测试方法
还是在8401的controller中,加入热点测试方法。
@GetMapping("/testHotKey")
@SentinelResource(value = "testHotKey", blockHandler = "del_testHotKey") //这里的名称可以随便写,但是一般跟rest地址一样
public String testHotkey(@RequestParam(value = "p1", required = false) String p1,
@RequestParam(value = "p1", required = false) String p2) {
return "------testHotkey";
}
//这里是我们自定义的兜底方法,BlockException不要打成了BlockedException
public String del_testHotKey(String p1, String p2, BlockException e) {
return "这次不用默认的兜底提示Blocked by Sentinel(flow limiting),自定义提示:del_testHotKeyo(╥﹏╥)o...";
}
注解@SentinelResource(value = “testHotKey”, blockHandler = “del_testHotKey”)
分析:
- 其中 value = “testHotKey” 是一个标识(Sentinel资源名),与rest的
/testHotKey
对应,这里value的值可以任意写,但是我们约定与rest地址一致,唯一区别是没有/
。 - blockHandler = “del_testHotKey” 则表示如果违背了Sentinel中配置的流控规则,就会调用我们自己的兜底方法del_testHotKey
6.2.2 配置热点key限流规则
绑定testHotKey资源,把testHotKey对应的第一个参数作为热点key进行监控。设定热点限流规则:当该资源的访问QPS超过1次/s的时候,产生限流并执行自定义的del_testHotKey兜底方法。
简而言之:方法testHotKey里面第一个参数只要QPS超过每秒1次,马上降级处理。
6.2.3 测试
1次/s正常显示,迅速点击两次,触发热点限流,执行自定义兜底方法:
- 仅传入参数p2没有任何影响: http://localhost:8401/testHotKey?p2=b
- 现在开两个访问,一个通过jmeter压测http://localhost:8401/testHotKey?p1=a&p2=b,另外再单独使用浏览器访问http://localhost:8401/testHotKey?p2=b,发现只带参数p2访问没有任何影响。
- 不配置blockeHandler(兜底方法)
6.3 参数例外项
上述案例演示了第一个参数p1,当QPS超过1秒1次点击后马上被限流
特例情况:我们期望p1参数当它是某个特殊值时,它的限流值和平时不一样,比如当p1的值等于5时,它的阈值可以达到200。
测试
狂点http://localhost:8401/testHotKey?p1=5&p2=b 没有限流
6.4 其他
手动添加一个异常:
测试直接错误页面。
要注意: Sentinel它只管你有没有触发它的限流规则,也可以说只管这个web交互页面(控制台)里面的东西。 配置类的东西Sentinel可以管,java异常的错误我不管。
@SentinelResource
处理的是Sentinel控制台配置的违规情况,有blockHandler方法配置的兜底处理;
RuntimeException
int age = 10/0,这个是java运行时报出的运行时异常RunTimeException,@SentinelResource不管
总结:
@SentinelResource主管配置出错,运行出错该走异常走异常
七、系统规则(系统自适应限流)
官网
Sentinel 系统自适应限流从整体维度对应用入口流量进行控制,结合应用的 Load、CPU 使用率、总体平均 RT、入口 QPS 和并发线程数等几个维度的监控指标,通过自适应的流控策略,让系统的入口流量和系统的负载达到一个平衡,让系统尽可能跑在最大吞吐量的同时保证系统整体的稳定性。
系统保护规则是应用整体维度的,而不是资源维度的,并且仅对入口流量生效。入口流量指的是进入应用的流量(EntryType.IN),比如 Web 服务或 Dubbo 服务端接收的请求,都属于入口流量。
系统规则支持以下的模式:
- Load 自适应(仅对 Linux/Unix-like 机器生效):系统的 load1 作为启发指标,进行自适应系统保护。当系统 load1 超过设定的启发值,且系统当前的并发线程数超过估算的系统容量时才会触发系统保护(BBR 阶段)。系统容量由系统的 maxQps minRt 估算得出。设定参考值一般是 CPU cores 2.5。
- CPU usage(1.5.0+ 版本):当系统 CPU 使用率超过阈值即触发系统保护(取值范围 0.0-1.0),比较灵敏。
- 平均 RT:当单台机器上所有入口流量的平均 RT 达到阈值即触发系统保护,单位是毫秒。
- 并发线程数:当单台机器上所有入口流量的并发线程数达到阈值即触发系统保护。
- 入口 QPS:当单台机器上所有入口流量的 QPS 达到阈值即触发系统保护。
案例——配置全局QPS
不管是/testA还是/testB 只要QPS > 1 整个系统就不能用。
这个粒度太粗,就相当于一个窗口人很多,整个银行就不接待人了,不太建议使用。八、@SentinelResource 注解详解
8.1 按资源名称限流+后续处理
启动nacos+sentinel8.1.1 修改8401
(1) pom
引入我们自定义的公共api jar包<dependency><!-- 引入自己定义的api通用包,可以使用Payment支付Entity -->
<groupId>com.atguigu.springcloud</groupId>
<artifactId>cloud-api-commons</artifactId>
<version>${project.version}</version>
</dependency>
(2) 业务类
```java package com.atguigu.cloudalibaba.controller;
import com.alibaba.csp.sentinel.annotation.SentinelResource; import com.alibaba.csp.sentinel.slots.block.BlockException; import com.atguigu.springcloud.entities.CommonResult; import com.atguigu.springcloud.entities.Payment; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController;
@RestController public class RateLimitController { @GetMapping(“/byResource”) @SentinelResource(value = “byResource”, blockHandler = “handleException”) public CommonResult byResource() { return new CommonResult(200, “按资源名称限流测试OK”, new Payment(2020L, “serial001”)); }
public CommonResult handleException(BlockException exception) {
return new CommonResult(444, exception.getClass().getCanonicalName() + "\t 服务不可用");
}
}
<a name="LdBS8"></a>
### 8.1.2 配置流控规则——按资源名称添加流控规则
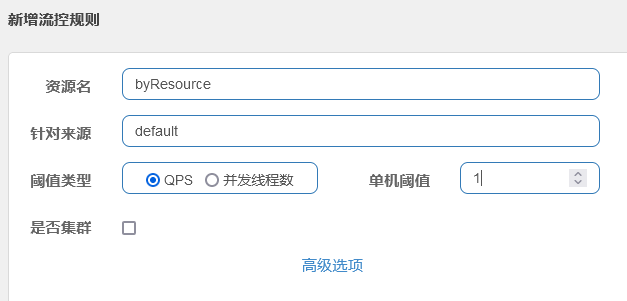
<a name="SX658"></a>
### 8.1.3 测试
自测:<br />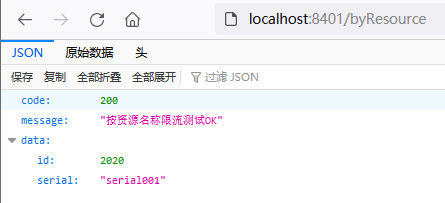<br />触发流控规则:<br />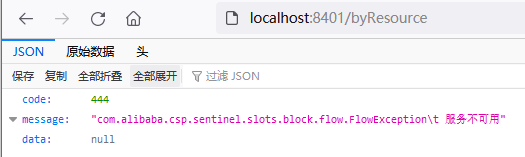
<a name="K0Qon"></a>
### 8.1.4 问题
如果我们重启8401会发现之前配置的一些规则都没有了。难道每次重启服务器都要重新配置一遍规则吗?规则如何进行持久化?
<a name="Leb1u"></a>
## 8.2 按照Url地址限流+后续处理
通过访问的URL来限流,会返回Sentinel自带默认的限流处理信息
<a name="jE7ne"></a>
### 8.2.1 修改controller
```java
@GetMapping("/rateLimit/byUrl")
@SentinelResource(value = "byUrl")
public CommonResult byUrl()
{
return new CommonResult(200,"按url限流测试OK",new Payment(2020L,"serial002"));
}
8.2.2 设置流控规则及测试
先自测,没有问题:
按rest URI设置流控规则
触发流控:
这个没有自定义的兜底的方法,返回Sentinel自带的限流处理结果。
8.3 总结以及面临的问题
不管是@GetMapping(rest url)还是 @SentinelResource,只要是唯一的,就可以作为流控规则的资源名称。如果没有自定义自己的兜底方法,那么就使用系统自带的。
问题:
- 依照现有条件,我们自定义的处理方法又和业务代码耦合在一块,不直观。如果都用系统默认的,就没有体现我们自己的业务要求。
- 如果每个业务方法/API接口都添加一个兜底的,那代码膨胀加剧。
- 全局统一的处理方法没有体现。
8.4 客户自定义限流处理逻辑
为了解决代码耦合与膨胀的问题8.4.1 创建CustomerBlockHandler类用于自定义限流处理逻辑
在CustomerBlockHandler类中统一的处理限流提示、服务降级的说明等等。。 ```java package com.atguigu.cloudalibaba.myhandler;
import com.alibaba.csp.sentinel.slots.block.BlockException; import com.atguigu.springcloud.entities.CommonResult; import com.atguigu.springcloud.entities.Payment;
public class CustomerBlockHandler { public static CommonResult handlerException(BlockException e) { return new CommonResult(4444, “按客户自定义, global handlerException——1”); }
public static CommonResult handlerException2(BlockException e) {
return new CommonResult(4444, "按客户自定义, global handlerException----2");
}
}
<a name="tEra4"></a>
### 8.4.2 修改RateLimitController,使用自定义处理逻辑类
```java
package com.atguigu.cloudalibaba.controller;
import com.alibaba.csp.sentinel.annotation.SentinelResource;
import com.alibaba.csp.sentinel.slots.block.BlockException;
import com.atguigu.cloudalibaba.myhandler.CustomerBlockHandler;
import com.atguigu.springcloud.entities.CommonResult;
import com.atguigu.springcloud.entities.Payment;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class RateLimitController {
@GetMapping("/byResource")
@SentinelResource(value = "byResource", blockHandler = "handleException")
public CommonResult byResource() {
return new CommonResult(200, "按资源名称限流测试OK", new Payment(2020L, "serial001"));
}
public CommonResult handleException(BlockException exception) {
return new CommonResult(444, exception.getClass().getCanonicalName() + "\t 服务不可用");
}
@GetMapping("/rateLimit/byUrl")
@SentinelResource(value = "byUrl")
public CommonResult byUrl() {
return new CommonResult(200, "按url限流测试OK", new Payment(2020L, "serial002"));
}
//CustomerBlockHandler自定义类,来处理服务降级、限流提示.....
/**
* 自定义通用的限流处理逻辑,
* blockHandlerClass = CustomerBlockHandler.class
* blockHandler = handleException2
* 上述配置:找CustomerBlockHandler类里的handleException2方法进行兜底处理
*/
//自定义通用的限流处理逻辑
@GetMapping("/rateLimit/customerBlockHandler")
@SentinelResource(value = "customerBlockHandler",
blockHandlerClass = CustomerBlockHandler.class,
blockHandler = "handlerException2")
public CommonResult customerBlockHandler() {
return new CommonResult(200, "按客户自定义", new Payment(2020L, "serial003"));
}
}
8.4.3 测试
启动8401,先测试一次http://localhost:8401/rateLimit/customerBlockHandler
设置流控规则:
触发流控,看是否是我们自定义提示:
自定义提示出来了!
8.4.4 结构说明
8.5 更多属性说明
九、服务熔断
主要内容:
- sentinel分别整合ribbon+openFeign以及设置fallback
-
9.1 Ribbon系列
nacos中整合了Ribbon,所以直接使用nacos就行。启动nacos和Sentinel。
9.1.1 服务提供者9003/9004
新建cloudalibaba-provider-payment9003/9004两个一样的做法
(1) pom
2020版和springcloud和nacos记得引入spring-cloud-starter-loadbalancer依赖
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>jdk8cloud2021</artifactId>
<groupId>com.atguigu.springcloud</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>cloudalibaba-provider-payment9003</artifactId>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<dependencies>
<!--SpringCloud ailibaba nacos -->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<dependency><!-- 引入自己定义的api通用包,可以使用Payment支付Entity -->
<groupId>com.atguigu.springcloud</groupId>
<artifactId>cloud-api-commons</artifactId>
<version>${project.version}</version>
</dependency>
<!-- SpringBoot整合Web组件 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<!--日常通用jar包配置-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
</project>
(2) yml
9004 别忘了改端口号 ```yaml server: port: 9003
spring: application: name: nacos-payment-provider cloud: nacos: discovery: server-addr: localhost:8848 #配置Nacos地址
management: endpoints: web: exposure: include: ‘*’
<a name="WQCxT"></a>
#### (3) 主启动类
```java
package com.atguigu.cloudalibaba;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
@EnableDiscoveryClient
@SpringBootApplication
public class PaymentMain9003 {
public static void main(String[] args) {
SpringApplication.run(PaymentMain9003.class, args);
}
}
(4) 业务类
这里图方便,就没有连接数据库。
package com.atguigu.cloudalibaba.controller;
import com.atguigu.springcloud.entities.CommonResult;
import com.atguigu.springcloud.entities.Payment;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
import java.util.HashMap;
@RestController
public class PaymentController {
@Value("${server.port}")
private String serverPort;
public static HashMap<Long, Payment> hashMap = new HashMap<>();
static {
hashMap.put(1L, new Payment(1L, "28a8c1e3bc2742d8848569891fb42181"));
hashMap.put(2L, new Payment(2L, "bba8c1e3bc2742d8848569891ac32182"));
hashMap.put(3L, new Payment(3L, "6ua8c1e3bc2742d8848569891xt92183"));
}
@GetMapping(value = "/paymentSQL/{id}")
public CommonResult<Payment> paymentSQL(@PathVariable("id") Long id) {
Payment payment = hashMap.get(id);
CommonResult<Payment> result = new CommonResult(200, "from mysql,serverPort: " + serverPort, payment);
return result;
}
}
(5) 测试
http://localhost:9003/paymentSQL/1
http://localhost:9004/paymentSQL/1
9.1.2 服务消费者84
新建cloudalibaba-consumer-nacos-order84
(1) pom
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>jdk8cloud2021</artifactId>
<groupId>com.atguigu.springcloud</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>cloudalibaba-consumer-nacos-order84</artifactId>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<dependencies>
<!--SpringCloud ailibaba nacos -->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-nacos-discovery</artifactId>
</dependency>
<!--SpringCloud ailibaba sentinel -->
<dependency>
<groupId>com.alibaba.cloud</groupId>
<artifactId>spring-cloud-starter-alibaba-sentinel</artifactId>
</dependency>
<!-- 引入自己定义的api通用包,可以使用Payment支付Entity -->
<dependency>
<groupId>com.atguigu.springcloud</groupId>
<artifactId>cloud-api-commons</artifactId>
<version>${project.version}</version>
</dependency>
<!-- SpringBoot整合Web组件 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<!--日常通用jar包配置-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
</project>
(2) yml
server:
port: 84
spring:
application:
name: nacos-order-consumer
cloud:
nacos:
discovery:
server-addr: localhost:8848
sentinel:
transport:
#配置Sentinel dashboard地址
dashboard: localhost:8080
#默认8719端口,假如被占用会自动从8719开始依次+1扫描,直至找到未被占用的端口
port: 8719
#消费者将要去访问的微服务名称(注册成功进nacos的微服务提供者)
#方便controller的@value获取
service-url:
nacos-user-service: http://nacos-payment-provider
(3) 主启动类
package com.atguigu.cloudalibaba;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.cloud.client.discovery.EnableDiscoveryClient;
@EnableDiscoveryClient
@SpringBootApplication
public class OrderNacosMain84 {
public static void main(String[] args) {
SpringApplication.run(OrderNacosMain84.class, args);
}
}
(4) 业务类
因为用的Ribbon,需要使用其提供的RestTemplate
package com.atguigu.cloudalibaba.config;
import org.springframework.cloud.client.loadbalancer.LoadBalanced;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.client.RestTemplate;
@Configuration
public class ApplicationContextConfig {
@Bean
@LoadBalanced //不要忘了
public RestTemplate getRestemplate() {
return new RestTemplate();
}
}
package com.atguigu.cloudalibaba.controller;
import com.alibaba.csp.sentinel.annotation.SentinelResource;
import com.atguigu.springcloud.entities.CommonResult;
import com.atguigu.springcloud.entities.Payment;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.client.RestTemplate;
@RestController
public class CircleBreakerController {
@Value("${service-url.nacos-user-service}")
private static String SERVICE_URL;
//public static final String SERVICE_URL = "http://nacos-payment-provider";
@Autowired
RestTemplate restTemplate;
@RequestMapping("/consumer/fallback/{id}")
@SentinelResource(value = "fallback") //没有配置
public CommonResult<Payment> fallback(@PathVariable Long id) {
CommonResult<Payment> result = restTemplate.getForObject(SERVICE_URL + "/paymentSQL/" + id, CommonResult.class, id);
if (id == 4) {
throw new IllegalArgumentException("IllegalArgumentException,非法参数异常....");
} else if (result.getData() == null) {
throw new NullPointerException("NullPointerException,该ID没有对应记录,空指针异常");
}
return result;
}
}
(5) 测试
9.1.3 fallback 和 blockHandler
加深@SentinelResource(value = “xxx”, fallback = “fffff”, blockHandler = “bbbbbb”)注解理解:
fallback管运行异常,blockHandler管配置违规。
fallback对应服务降级,就是服务出错了应该怎么办(需要有个兜底方法);
blockHandler对应服务熔断,就是我现在服务不可用,我应该怎么办,怎么给客户一个户提示(同样需要一个兜底方法)
9.1.4 差异化配置
这里改的业务类代码均是消费者84端的业务类代码,不要搞错了。
降级是服务业务代码出现错误的兜底,熔断是服务不可用。降级是兜底方法,熔断是对服务保护时间窗口期服务不可用。
(1) 没有任何配置
前面我们的84消费端,@SentinelResource里面只配置了value,fallback和blockHandler都没有配置,该情况下我们测试一下http://localhost:84/consumer/fallback/4
出现了错误页面,error page对客户不友好,所以我们需要有兜底方法。
(2) 只配置fallback
fallback对应服务降级,就是服务可以正常访问,但是业务逻辑出现错误,需要降级兜底。
@RequestMapping("/consumer/fallback/{id}")
//@SentinelResource(value = "fallback") //没有配置
@SentinelResource(value = "fallback", fallback = "handlerFallback") //fallback负责业务异常
public CommonResult<Payment> fallback(@PathVariable Long id) {
CommonResult<Payment> result = restTemplate.getForObject(SERVICE_URL + "/paymentSQL/" + id, CommonResult.class, id);
if (id == 4) {
throw new IllegalArgumentException("IllegalArgumentException,非法参数异常....");
} else if (result.getData() == null) {
throw new NullPointerException("NullPointerException,该ID没有对应记录,空指针异常");
}
return result;
}
public CommonResult handlerFallback(@PathVariable Long id,Throwable e) {
Payment payment = new Payment(id,"null");
return new CommonResult<>(444,"兜底异常handlerFallback,exception内容 "+e.getMessage(),payment);
}
访问http://localhost:84/consumer/fallback/4,可以看到业务异常
(3) 只配置blockHandler
blockHandler对应服务熔断,当前sentinel配置已经违规(RT数过多、异常过多),服务熔断后不可用,需要给客户提示,进行一个熔断的兜底。
@RequestMapping("/consumer/fallback/{id}")
//@SentinelResource(value = "fallback") //没有配置
//@SentinelResource(value = "fallback", fallback = "handlerFallback") //fallback负责业务异常,对应服务降级
@SentinelResource(value = "fallback", blockHandler = "blockHandler") //blockHandler只负责sentinel控制台配置违规,对应服务熔断
public CommonResult<Payment> fallback(@PathVariable Long id) {
CommonResult<Payment> result = restTemplate.getForObject(SERVICE_URL + "/paymentSQL/" + id, CommonResult.class, id);
if (id == 4) {
throw new IllegalArgumentException("IllegalArgumentException,非法参数异常....");
} else if (result.getData() == null) {
throw new NullPointerException("NullPointerException,该ID没有对应记录,空指针异常");
}
return result;
}
//本例是fallback
// public CommonResult handlerFallback(@PathVariable Long id,Throwable e) {
// Payment payment = new Payment(id,"null");
// return new CommonResult<>(444,"兜底异常handlerFallback,exception内容 "+e.getMessage(),payment);
// }
//本例是blockHandler
public CommonResult blockHandler(@PathVariable Long id, BlockException blockException) {
Payment payment = new Payment(id,"null");
return new CommonResult<>(445,"blockHandler-sentinel限流,无此流水: blockException "
+ blockException.getMessage(),payment);
}
配置sentinel
访问:http://localhost:84/consumer/fallback/5
这里还是跟之前一样的bug,很迷。
(4) fallback和blockHandler都配置
同时有降级跟熔断的兜底方法,当降级达到sentinel配置规则后,触发熔断。
@RequestMapping("/consumer/fallback/{id}")
@SentinelResource(value = "fallback", fallback = "handlerFallback", blockHandler = "blockHandler")
public CommonResult<Payment> fallback(@PathVariable Long id) {
CommonResult<Payment> result = restTemplate.getForObject(SERVICE_URL + "/paymentSQL/" + id, CommonResult.class, id);
if (id == 4) {
throw new IllegalArgumentException("IllegalArgumentException,非法参数异常....");
} else if (result.getData() == null) {
throw new NullPointerException("NullPointerException,该ID没有对应记录,空指针异常");
}
return result;
}
//本例是fallback
public CommonResult handlerFallback(@PathVariable Long id,Throwable e) {
Payment payment = new Payment(id,"null");
return new CommonResult<>(444,"兜底异常handlerFallback,exception内容 "+e.getMessage(),payment);
}
//本例是blockHandler
public CommonResult blockHandler(@PathVariable Long id, BlockException blockException) {
Payment payment = new Payment(id,"null");
return new CommonResult<>(445,"blockHandler-sentinel限流,无此流水: blockException "
+ blockException.getMessage(),payment);
}
配置sentinel
删除之前的熔断规则,配置流控:
限流效果:
没有触发限流时,我们触发业务异常http://localhost:84/consumer/fallback/4,会被降级方法fallback兜底:
触发限流时,我们仍然访问可以触发业务异常的连接,此时服务已经被限流(可以理解为服务不可用即熔断),此时触发的是限流(熔断blockHandler):
也就是说,同时配置fallback:处理业务异常(微服务自身异常,服务降级)和blockHandler:处理触发sentinel配置(微服务不可用,服务熔断)时。在没有违反sentinel规则时,出现业务异常(降级)走fallback方法;违反了sentinel规则时,直接微服务不可用(熔断),走blockHandler指定的自定义方法。
(5) 异常忽略属性
可以选择性的配置当某些异常发生时,不触发fallback的兜底方法。
@RequestMapping("/consumer/fallback/{id}")
@SentinelResource(value = "fallback", fallback = "handlerFallback", blockHandler = "blockHandler",
exceptionsToIgnore = {IllegalArgumentException.class})
public CommonResult<Payment> fallback(@PathVariable Long id) {
CommonResult<Payment> result = restTemplate.getForObject(SERVICE_URL + "/paymentSQL/" + id, CommonResult.class, id);
if (id == 4) {
throw new IllegalArgumentException("IllegalArgumentException,非法参数异常....");
} else if (result.getData() == null) {
throw new NullPointerException("NullPointerException,该ID没有对应记录,空指针异常");
}
return result;
}
//本例是fallback
public CommonResult handlerFallback(@PathVariable Long id,Throwable e) {
Payment payment = new Payment(id,"null");
return new CommonResult<>(444,"兜底异常handlerFallback,exception内容 "+e.getMessage(),payment);
}
//本例是blockHandler
public CommonResult blockHandler(@PathVariable Long id, BlockException blockException) {
Payment payment = new Payment(id,"null");
return new CommonResult<>(445,"blockHandler-sentinel限流,无此流水: blockException "
+ blockException.getMessage(),payment);
}
测试一下,访问:http://localhost:84/consumer/fallback/4
直接报错误页面,没有了降级兜底方法。
其他异常不受影响:
当然,触发流控之后,仍然通过blockHandler指定的方法进行熔断兜底。
9.2 Feign系列
9.2.1 修改84模块
(1) pom
pom 加入feign的依赖
<!--SpringCloud openfeign -->
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
(2) yml
激活Sentinel对Feign的支持
# 激活Sentinel对Feign的支持
feign:
sentinel:
enabled: true
(3) 业务类
后续84的controller不找restTemplate(Ribbon),不是restTemplate去调用payment微服务中的接口。而是通过调用PaymentFeignService,service再去调用payment微服务中的端口。
Feign需要定义一个业务逻辑(service)接口+ @FeignClient注解以调用服务提供者。
新建PaymentFeignService interface:
package com.atguigu.cloudalibaba.service;
import com.atguigu.springcloud.entities.CommonResult;
import com.atguigu.springcloud.entities.Payment;
import org.springframework.cloud.openfeign.FeignClient;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
// 指明调用失败的兜底方法在PaymentFallbackService
// 使用 fallback 方式是无法获取异常信息的,
// 如果想要获取异常信息,可以使用 fallbackFactory参数
@FeignClient(value = "nacos-payment-provider", fallback = PaymentFallbackService.class)
public interface PaymentFeignService {
//去nacos-payment-provider服务中找相应的接口
// 方法签名一定要和nacos-payment-provider中controller的一致
// 对应9003、9004中的方法
@GetMapping(value = "/paymentSQL/{id}")
public CommonResult<Payment> paymentSQL(@PathVariable("id") Long id);
}
调用失败的兜底方法:
package com.atguigu.cloudalibaba.service;
import com.atguigu.springcloud.entities.CommonResult;
import com.atguigu.springcloud.entities.Payment;
import org.springframework.stereotype.Component;
@Component //不要忘记了
public class PaymentFallbackService implements PaymentFeignService {
//如果nacos-payment-consumer服务中的相应接口出事了,我来兜底
@Override
public CommonResult<Payment> paymentSQL(Long id) {
return new CommonResult<>(444,"服务降级返回,没有该流水信息-------PaymentFallbackService",new Payment(id, "errorSerial......"));
}
}
84端口controller加入openFeign的接口:
//==================OpenFeign
@Resource
private PaymentFeignService paymentFeignService;
@GetMapping(value = "/consumer/paymentSQL/{id}")
public CommonResult<Payment> paymentSQL(@PathVariable("id") Long id) {
if (id == 4) {
throw new RuntimeException("没有该id");
}
return paymentFeignService.paymentSQL(id);
}
(4) 主启动类
加上@EnableFeignClient注解开启OpenFeign
(5) 测试
启动9003、9004、84
访问:http://localhost:84/consumer/paymentSQL/1
9003、9004负载均衡
关闭9003、9004微服务提供者,看到84消费者自动执行降级兜底方法。
如果yaml没有配置Sentinel对Feign的支持,就不会执行降级方法,而是直接报错误页面。
9.3 熔断框架比较
十、持久化规则
前面我们微服务新增的限流规则后,微服务关闭后就会丢失,当时配置都限流规则都是临时的。 将限流配置规则持久化进Nacos保存,只要刷新8401某个rest地址,sentinel控制台的流控规则 就能看到。只要nacos里面的配置不删除,针对8401上的sentinel上的流控规则就持续存在。 (也可以持久化到文件,redis,数据库等)
案例——修改8401已完成持久化设置
(1) pom
导入持久化所需依赖
<!--SpringCloud ailibaba sentinel-datasource-nacos 持久化-->
<dependency>
<groupId>com.alibaba.csp</groupId>
<artifactId>sentinel-datasource-nacos</artifactId>
</dependency>
(2) yaml
添加nacos数据源配置
server:
port: 8401
spring:
application:
name: cloudalibaba-sentinel-service
cloud:
nacos:
discovery:
server-addr: localhost:8848 #Nacos服务注册中心地址
sentinel:
transport:
#配置Sentinel dashboard地址
dashboard: localhost:8080
#默认8719端口,假如被占用会自动从8719开始依次+1扫描,直至找到未被占用的端口
port: 8719
# 关闭默认收敛所有URL的入口context,不然链路限流不生效
# Spring Cloud Alibaba 需要2.1.1.RELEASE以上版本
web-context-unify: false
# filter:
# enabled: false # 关闭自动收敛
#持久化配置
datasource:
ds1:
nacos:
server-addr: localhost:8848
dataId: cloudalibaba-sentinel-service
groupId: DEFAULT_GROUP
data-type: json
rule-type: flow
management:
endpoints:
web:
exposure:
include: '*'
(3) 添加nacos业务规则配置
我们将sentinel的流控配置保存在nacos中,因为nacos的配置持久化在了数据库中。
[
{
"resource": "/rateLimit/byUrl",
"limitApp": "default",
"grade": 1,
"count": 1,
"strategy": 0,
"controlBehavior": 0,
"clusterMode": false
}
]
resource:资源名称;
limitApp:来源应用;
grade:阈值类型,0表示线程数,1表示QPS;
count:单机阈值;
strategy:流控模式,0表示直接,1表示关联,2表示链路;
controlBehavior:流控效果,0表示快速失败,1表示Warm Up,2表示排队等待;
clusterMode:是否集群。
注意:这里如果要配nacos的命名空间(public、dev、test)的话,应该是配namespace的id,不是名称
(4) 测试
启动8401,访问8401任意接口,刷新Sentinel。可以看到Sentinel中加载了通过nacos持久化的规则配置文件。
关掉8401后发现流控规则没有了。
再次启动8401查看sentinel,访问几次8401后流控规则又出现了。
查看数据库,发现规则持久化到数据库中了。