- 知识点: Dictionary, 委托, 观察者设计模式
- 作用: 降低程序耦合性, 减小程序复杂度
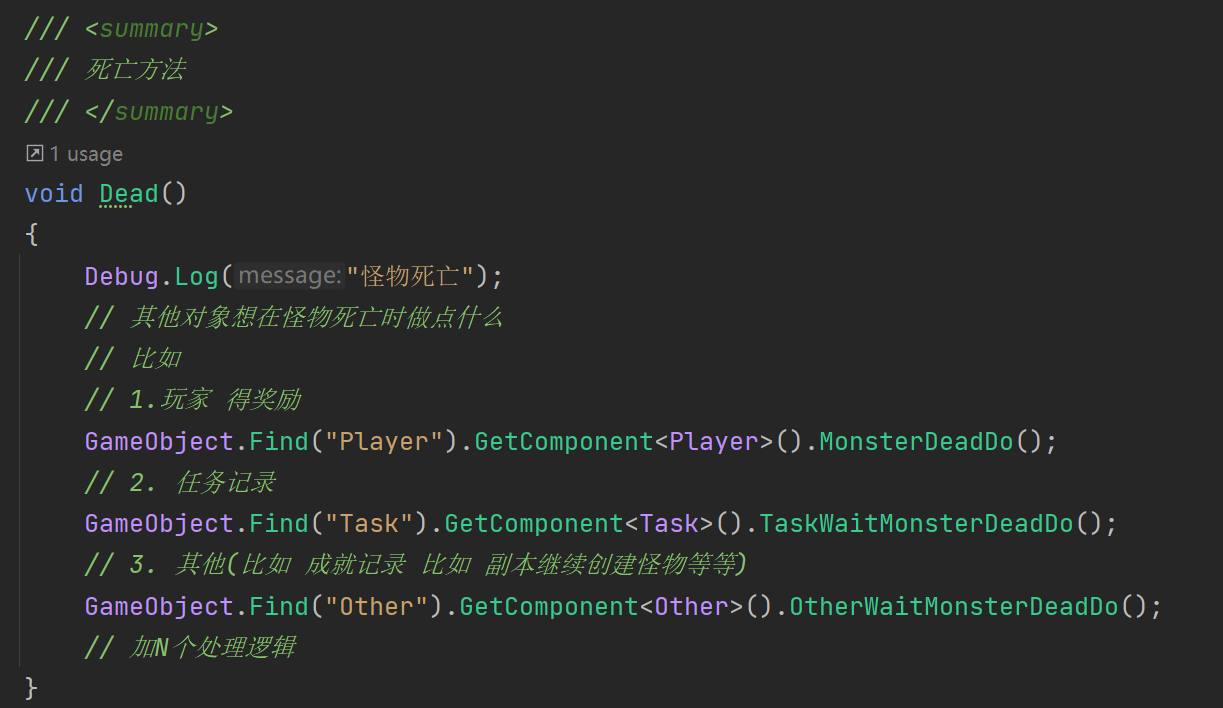
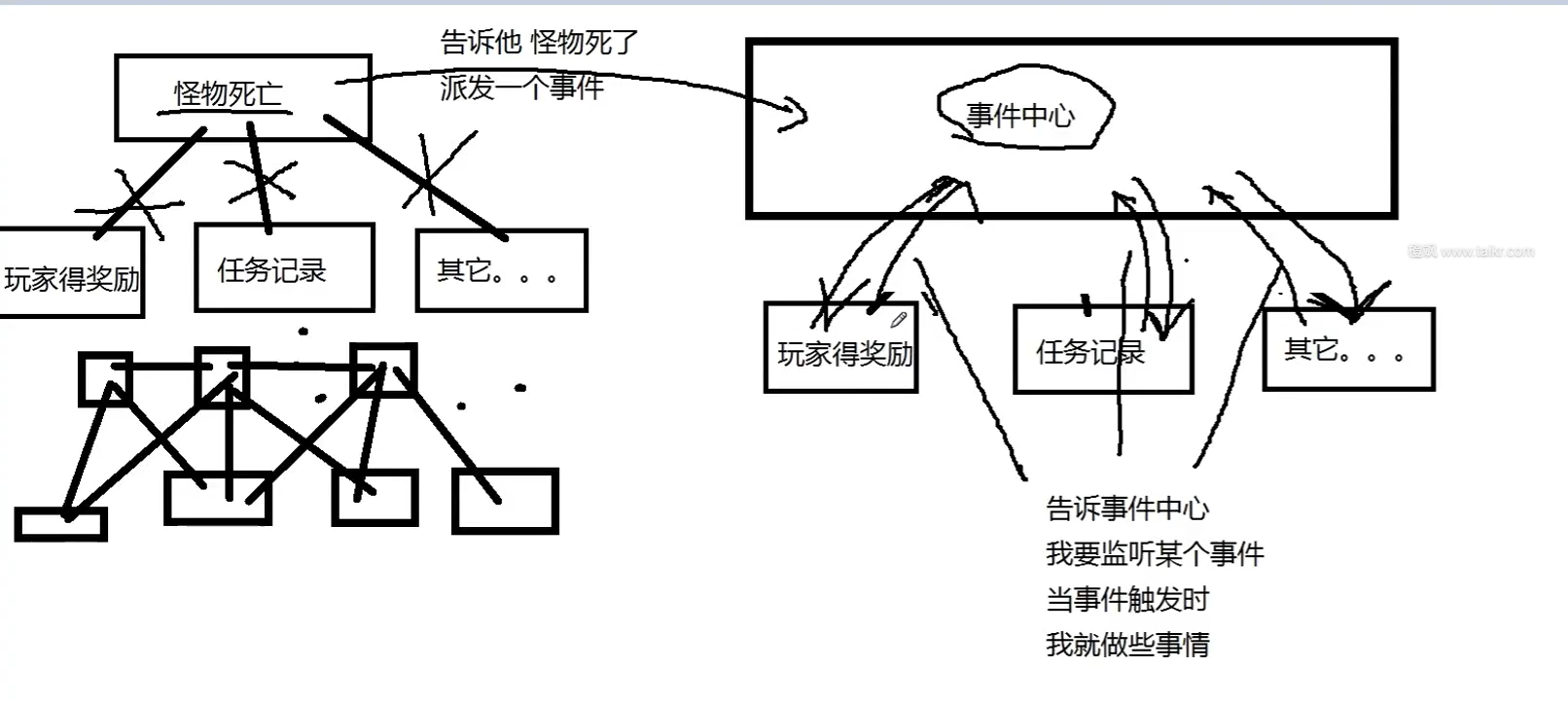
代码
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Events;
/// <summary>
/// 事件中心 单例模式对象
/// 1. Dictionary
/// 2. 委托
/// 3. 观察者设计模式
/// </summary>
public class EventCenter : BaseManager<EventCenter>
{
// key - 事件的名字(比如: 怪物死亡, 玩家死亡, 通关 等等)
// value - 对应的是 监听这个事件 对应的委托函数们
private Dictionary<string, UnityAction<object>> eventDic = new Dictionary<string, UnityAction<object>>();
/// <summary>
/// 添加事件监听
/// </summary>
/// <param name="name">事件的名字</param>
/// <param name="action">准备用来处理事件的委托函数</param>
public void AddEventListener(string name, UnityAction<object> action)
{
// 有没有对应的事件监听
// 有的情况
if (eventDic.ContainsKey(name))
{
eventDic[name] += action;
}
// 没有的情况
else
{
eventDic.Add(name,action);
}
}
/// <summary>
/// 移除对应的事件监听
/// </summary>
/// <param name="name">事件的名字</param>
/// <param name="action">对应之前添加的委托函数</param>
public void RemoveEventListener(string name, UnityAction<object> action)
{
if (eventDic.ContainsKey(name))
eventDic[name] -= action;
}
/// <summary>
/// 事件触发
/// </summary>
/// <param name="name">哪一个名字的事件触发</param>
public void EventTrigger(string name, object info)
{
if (eventDic.ContainsKey(name))
{
// 执行监听的所有函数
eventDic[name].Invoke(info);
}
// 不存在则什么都不用做
}
/// <summary>
/// 清空事件中心
/// 主要用于场景切换时
/// </summary>
public void Clear()
{
eventDic.Clear();
}
}
例子
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Monster : MonoBehaviour
{
public string name = "123123";
void Start()
{
Dead();
}
/// <summary>
/// 死亡方法
/// </summary>
void Dead()
{
Debug.Log("怪物死亡");
// // 其他对象想在怪物死亡时做点什么
// // 比如
// // 1.玩家 得奖励
// GameObject.Find("Player").GetComponent<Player>().MonsterDeadDo();
// // 2. 任务记录
// GameObject.Find("Task").GetComponent<Task>().TaskWaitMonsterDeadDo();
// // 3. 其他(比如 成就记录 比如 副本继续创建怪物等等)
// GameObject.Find("Other").GetComponent<Other>().OtherWaitMonsterDeadDo();
// // 加N个处理逻辑
// 触发事件
EventCenter.GetInstance().EventTrigger("MonsterDead", this);
}
}
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Player : MonoBehaviour
{
private void Awake()
{
EventCenter.GetInstance().AddEventListener("MonsterDead",MonsterDeadDo);
}
/// <summary>
/// 怪物死时要做什么
/// </summary>
/// <param name="info"></param>
public void MonsterDeadDo(object info)
{
Debug.Log("玩家得奖励" + (info as Monster).name);
}
private void OnDestroy()
{
EventCenter.GetInstance().RemoveEventListener("MonsterDead",MonsterDeadDo);
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Task : MonoBehaviour
{
private void Awake()
{
EventCenter.GetInstance().AddEventListener("MonsterDead",TaskWaitMonsterDeadDo);
}
/// <summary>
/// 怪物死时要做什么
/// </summary>
/// <param name="info"></param>
public void TaskWaitMonsterDeadDo(object info)
{
Debug.Log("任务 记录");
}
private void OnDestroy()
{
EventCenter.GetInstance().RemoveEventListener("MonsterDead",TaskWaitMonsterDeadDo);
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Other : MonoBehaviour
{
private void Awake()
{
EventCenter.GetInstance().AddEventListener("MonsterDead",OtherWaitMonsterDeadDo);
}
public void OtherWaitMonsterDeadDo(object info)
{
Debug.Log("其他 各个对象要做的事情");
}
private void OnDestroy()
{
EventCenter.GetInstance().RemoveEventListener("MonsterDead",OtherWaitMonsterDeadDo);
}
}