先前完成的缓存池模块的缺点
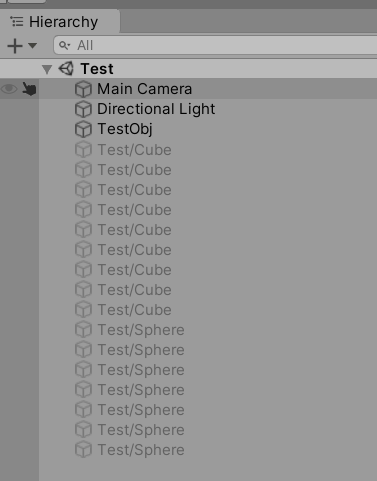
- 很多缓存池对象在hierarchy面板上,不方便管理,可以进行优化
- 切场景时,缓存池里的对象都会被删除,但是仍然在列表中保持连接,应该提供一个清除缓存池的方法
代码
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
/// <summary>
/// 抽屉数据 池子中的一列容器
/// </summary>
public class PoolData
{
// 抽屉中对象挂载的父节点
public GameObject fatherObj;
// 对象的容器
public List<GameObject> poolList;
public PoolData(GameObject obj, GameObject poolObj)
{
// 给我们的抽屉创建一个父对象 并且把他作为我们pool对象的子物体
fatherObj = new GameObject(obj.name);
fatherObj.transform.parent = poolObj.transform;
//poolList = new List<GameObject>() { obj };
//obj.transform.parent = fatherObj.transform;
//obj.SetActive(false);
poolList = new List<GameObject>() {};
PushObj(obj);
}
/// <summary>
/// 往抽屉里面压东西
/// </summary>
/// <param name="obj"></param>
public void PushObj(GameObject obj)
{
// 失活 让其隐藏
obj.SetActive(false);
// 存起来
poolList.Add(obj);
// 设置父对象
obj.transform.parent = fatherObj.transform;
}
/// <summary>
/// 从抽屉里面取东西
/// </summary>
/// <returns></returns>
public GameObject GetObj()
{
GameObject obj = null;
// 取出第一个
obj = poolList[0];
poolList.RemoveAt(0);
// 激活 让其显示
obj.SetActive(true);
// 断开父子关系
obj.transform.parent = null;
return obj;
}
}
/// <summary>
/// 缓存池模块
/// </summary>
public class PoolMgr : BaseManager<PoolMgr> // 单例模式
{
public Dictionary<string,PoolData> poolDic = new Dictionary<string, PoolData>();
// 作为根节点
private GameObject poolObj;
/// <summary>
/// 往外拿东西
/// </summary>
/// <param name="name">规定预设体的路径作为抽屉的名字</param>
/// <returns></returns>
public GameObject GetObj(string name)
{
GameObject obj = null;
// 有抽屉且抽屉里有东西
if (poolDic.ContainsKey(name) && poolDic[name].poolList.Count > 0)
{
obj = poolDic[name].GetObj();
}
else
{
// 抽屉里没有,则实例化一个新的返回出去
// 规定预设体的路径作为抽屉的名字
obj = GameObject.Instantiate(Resources.Load<GameObject>(name));
obj.name = name; // 让对象的名字和外面传进来的名字一样,即资源路径名,也是我们规定的抽屉名,方便存进池子时获取名字
}
return obj;
}
/// <summary>
/// 还暂时不用的对象
/// </summary>
public void PushObj(string name, GameObject obj)
{
if (poolObj == null)
poolObj = new GameObject("Pool");
// 里面有抽屉
if (poolDic.ContainsKey(name))
{
poolDic[name].PushObj(obj);
}
// 里面没有抽屉
else
{
poolDic.Add(name, new PoolData(obj,poolObj));
}
}
/// <summary>
/// 情况缓存池的方法,主要用于场景切换时
/// </summary>
public void Clear()
{
poolDic.Clear();
poolObj = null; // 切场景时父对象也会被移除
}
}
优化效果
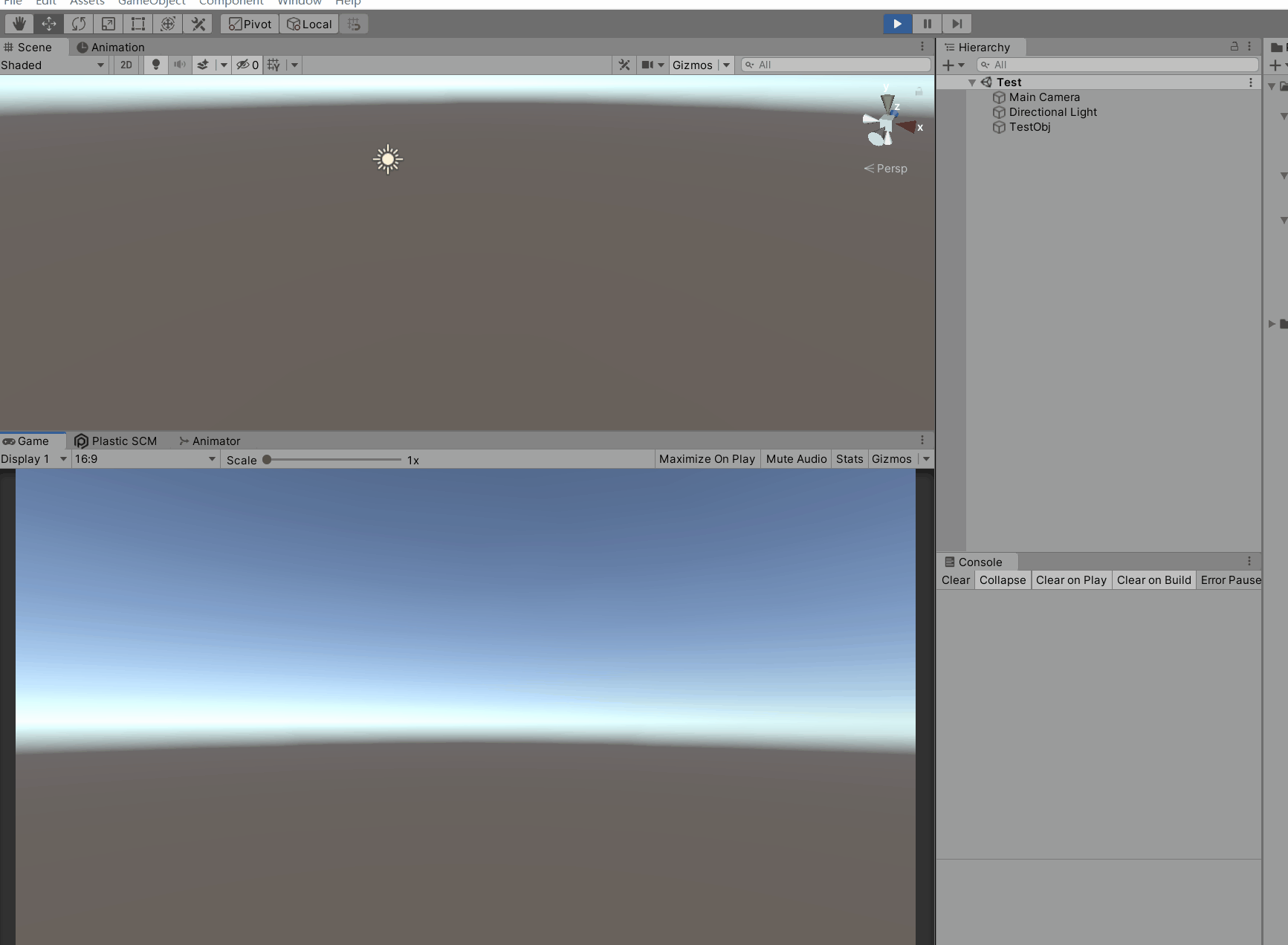