对话框通常作为按钮点击信号的插槽,故对话框代码常写在槽函数中。
1 输入对话框(QInputDialog)
输入各类型数据 |
|
python def showDialog(self): sender = self.sender() if sender == self.bt1: # 单行字符串-输入对话框 text, ok = QInputDialog.getText(self, '输入', '单行字符串') # 返回值1, 返回值2 = QInputDialog.getText(self, 对话栏标题, 对话栏内容') # 返回值1(str):用户输入的内容;返回值2(bool):用户是否点击ok按钮 if ok: self.lb6.setText(text) elif sender == self.bt2: # 整数-输入对话框 text, ok = QInputDialog.getInt(self, '输入', '整数', min = 1) # 返回值1, 返回值2 = QInputDialog.getInt(self, 对话栏标题, 对话栏内容, 最小值) # 返回值1(int):用户输入的内容;返回值2(bool):用户是否点击ok按钮 if ok: self.lb7.setText(str(text)) elif sender == self.bt3: # 列表元素-输入对话框 text, ok = QInputDialog.getItem(self, '输入', '列表元素', ['Male', 'Female']) # 返回值1, 返回值2 = QInputDialog.getItem(self, 对话栏标题, 对话栏内容, 列表) # 返回值1(str):用户输入的内容;返回值2(bool):用户是否点击ok按钮 if ok: self.lb8.setText(text) elif sender == self.bt4: # 小数-输入对话框 text, ok = QInputDialog.getDouble(self, '输入', '双精度小数:', min = 1.0) # 返回值1, 返回值2 = QInputDialog.getDouble(self, 对话栏标题, 对话栏内容, 最小值) # 返回值1(double):用户输入的内容;返回值2(bool):用户是否点击ok按钮 if ok: self.lb9.setText(str(text)) elif sender == self.bt5: # 多行字符串-输入对话框 text, ok = QInputDialog.getMultiLineText(self, '输入', '多行字符串') # 返回值1, 返回值2 = QInputDialog.getText(self, 对话栏标题, 对话栏内容') # 返回值1(str):用户输入的内容;返回值2(bool):用户是否点击ok按钮 if ok: self.tb.setText(text) |
|
dlc - 所有功能 |
|
[List of All Members for QInputDialog |
Qt Widgets 6.3.2](https://doc.qt.io/qt-6/qinputdialog-members.html) |
2 消息对话框(QMessageBox)
2.1 按类型创建对话框
2.1.1 基本对话框(about)
基本对话框 — about |
|
python def about(self): # 创建基本对话栏 QMessageBox.about(self, 'title', 'content') # QMessageBox.about(父组件, 标题, 对话框信息) |
2.1.2
提示对话框(information)
提示对话框 — information |
|
```python def information(self): # 创建提示对话栏 reply = QMessageBox.information(self,’提示’,’提示对话框’, QMessageBox.StandardButton.Ok |
QMessageBox.StandardButton.Close, QMessageBox.StandardButton.Close) # QMessageBox.information(self, 标题, 对话栏信息, 选项按钮, 默认选项按钮) # 多选项按钮,使用 |
间隔 # 判定所选选项按钮 if reply == QMessageBox.StandardButton.Ok: print(‘ok’) else: print(‘close’) ``` |
2.1.3
询问对话框(question)
询问对话框 — question |
|
```python def question(self): reply = QMessageBox.question(self,’询问’,’询问对话框’, QMessageBox.StandardButton.Yes |
QMessageBox.StandardButton.No |
QMessageBox.StandardButton.Cancel, QMessageBox.StandardButton.No) # QMessageBox.question(self, 标题, 对话栏信息, 选项按钮, 默认选项按钮) # 多选项按钮,使用 |
间隔 # 判定所选选项按钮 if reply == QMessageBox.StandardButton.Yes: print(‘yes’) elif reply == QMessageBox.StandardButton.No: print(‘no’) else: print(‘cancel’) ``` |
2.1.4
警告对话框(warning)
警告对话框 — warning |
|
```python def warning(self): reply = QMessageBox.warning(self,’警告’,’警告对话框’, QMessageBox.StandardButton.Save |
QMessageBox.StandardButton.Discard |
QMessageBox.StandardButton.Cancel, QMessageBox.StandardButton.Save) # QMessageBox.question(self, 标题, 对话栏信息, 选项按钮, 默认选项按钮) # 多选项按钮,使用 |
间隔 # 判定所选选项按钮 if reply == QMessageBox.StandardButton.Save: print(‘save’) elif reply == QMessageBox.StandardButton.Discard: print(‘discard’) else: print(‘cancel’) ``` |
2.1.5
错误对话框(critical)
错误对话框 — critical |
|
```python def critical(self): reply = QMessageBox.critical(self,’错误’,’错误对话框’, QMessageBox.Retry |
QMessageBox.Abort |
QMessageBox.Ignore , QMessageBox.Retry) # QMessageBox.critical(self, 标题, 对话栏信息, 选项按钮, 默认选项按钮) # 多选项按钮,使用 |
间隔 # 判定所选选项按钮 if reply == QMessageBox.StandardButton.Retry: print(‘retry’) elif reply == QMessageBox.StandardButton.Abort: print(‘abort’) else: print(‘ignore’) ``` |
2.1.6
QT 对话框(aboutQt)
QT 对话框 — aboutQt |
|
python def aboutqt(self): QMessageBox.aboutQt(self,'标题') |
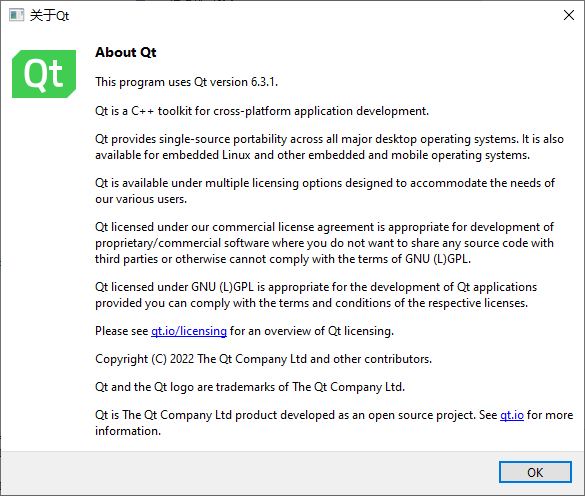
2.1.9 按钮选项(StandardButton)
选项 |
说明 |
对应按钮事件 |
QMessageBox.StandardButton.Ok |
确定 |
AcceptRole |
QMessageBox.StandardButton.Open |
打开 |
AcceptRole |
QMessageBox.StandardButton.Save |
保存 |
AcceptRole |
QMessageBox.StandardButton.Apply |
应用 |
AcceptRole |
QMessageBox.StandardButton.Retry |
重置 |
AcceptRole |
QMessageBox.StandardButton.Ignore |
忽略 |
AcceptRole |
QMessageBox.StandardButton.SaveAll |
全部保存 |
AcceptRole |
QMessageBox.StandardButton.Close |
关闭 |
RejectRole |
QMessageBox.StandardButton.Cancel |
取消 |
RejectRole |
QMessageBox.StandardButton.Reset |
重置 |
RejectRole |
QMessageBox.StandardButton.Abort |
终止 |
RejectRole |
QMessageBox.StandardButton.RestoreDefaults |
恢复默认值 |
RejectRole |
QMessageBox.StandardButton.Discard |
放弃/不保存 |
DestructiveRole |
QMessageBox.StandardButton.Help |
帮助 |
HelpRole |
QMessageBox.StandardButton.Yes |
是 |
YesRole |
QMessageBox.StandardButton.YesToAll |
全部同意 |
YesRole |
QMessageBox.StandardButton.No |
否 |
NoRole |
QMessageBox.StandardButton.NoToAll |
全部拒绝 |
NoRole |
QMessageBox.StandardButton.NoButton |
无 |
无按钮 |
2.2 按属性创建对话框
按属性创建对话框 |
|
```python def msg(self): # 创建一个对话框 # msgbox = QMessageBox() # 设置对话框图标 # msgbox.setIcon(QMessageBox.Icon.Warning) # 设置对话框标题 # msgbox.setWindowTitle(‘标题’) # 设置对话框文本 # msgbox.setText(‘简单说明’) # 创建一个对话框,并设置(图标、标题、说明),等效于2-9行 msgbox = QMessageBox(QMessageBox.Icon.NoIcon, ‘标题’, ‘简单说明’) # 设置对话框图片(QMessageBox.Icon.NoIcon时可用,即与图标矛盾) msgbox.setIconPixmap(QPixmap(“exit.png”)) # 设置对话框信息型文本 msgbox.setInformativeText(‘进一步说明’) # 添加选项按钮 - 方法一(自定义) accept = msgbox.addButton(‘确定’, QMessageBox.ButtonRole.AcceptRole) reject = msgbox.addButton(‘拒绝’, QMessageBox.ButtonRole.RejectRole) cancel = msgbox.addButton(‘取消’, QMessageBox.ButtonRole.DestructiveRole) # 添加选项按钮 - 方法二(预设值) # msgbox.setStandardButtons(QMessageBox.StandardButton.Apply |
QMessageBox.StandardButton.Reset |
QMessageBox.StandardButton.Discard) # 设置默认按钮 msgbox.setDefaultButton(accept) # msgbox.setDefaultButton(QMessageBox.StandardButton.Apply) # 添加细节按钮和细节信息 msgbox.setDetailedText(‘这些内容是你点击完按钮才出现的哦’) # 创建复选框 cb = QCheckBox(‘一个复选框’) # 设置对话框复选框 msgbox.setCheckBox(cb) # 复选框勾选事件信号 cb.stateChanged.connect(self.check) # 显示对话框 reply = msgbox.exec() # 按钮触发事件 if reply == QMessageBox.ButtonRole.AcceptRole: self.check # 实践证明,槽函数名写中文非常容易崩溃 # 复选框触发事件 def check(self): print(‘牛逼’) ``` |
2.2.1 对话框图标(Icon)
图标 |
等效 |
图标 |
QMessageBox.Icon.NoIcon |
基本对话框 |
无图标 |
QMessageBox.Icon.Information |
提示对话框 |
 |
QMessageBox.Icon.Question |
询问对话框 |
 |
QMessageBox.Icon.Warning |
警告对话框 |
 |
QMessageBox.Icon.Critical |
错误对话框 |
 |
2.2.2 按钮事件(ButtonRole)
按钮事件 |
说明 |
QMessageBox.ButtonRole.InvalidRole |
按钮无效 |
QMessageBox.ButtonRole.AcceptRole |
接受/确定 |
QMessageBox.ButtonRole.RejectRole |
拒绝/取消 |
QMessageBox.ButtonRole.DestructiveRole |
放弃 |
QMessageBox.ButtonRole.ActionRole |
更换元素? |
QMessageBox.ButtonRole.HelpRole |
请求帮助 |
QMessageBox.ButtonRole.YesRole |
是 |
QMessageBox.ButtonRole.NoRole |
否 |
QMessageBox.ButtonRole.ApplyRole |
应用 |
QMessageBox.ButtonRole.ResetRole |
默认 |
3 文本类对话框
3.1 颜色对话框(QColorDialog)
颜色对话框 — QColorDialog |
|
python from PyQt6.QtWidgets import (QMainWindow, QWidget, QPushButton, QFrame, QColorDialog, QApplication, QVBoxLayout) from PyQt6.QtGui import QColor import sys class QWindow(QMainWindow): def __init__(self): super().__init__() self.initUI() def initUI(self): # 设置默认颜色 col = QColor(0, 0, 0) self.btn = QPushButton('QColorDialog', self) # 连接颜色对话框 self.btn.clicked.connect(self.showDialog) self.frm = QFrame(self) # 修改框架颜色 self.frm.setStyleSheet("QWidget { background-color: %s }"% col.name()) vbox = QVBoxLayout() vbox.addWidget(self.frm) vbox.addWidget(self.btn) widget = QWidget(self) widget.setLayout(vbox) self.setCentralWidget(widget) self.setGeometry(250, 250, 250, 250) self.show() def showDialog(self): # 打开颜色对话框,并返回选择的颜色 col = QColorDialog.getColor() # 判断已选择颜色有效性 if col.isValid(): # 返回颜色名 self.frm.setStyleSheet("QWidget { background-color: %s }" % col.name()) def main(): app = QApplication(sys.argv) ex = QWindow() sys.exit(app.exec()) if __name__ == '__main__': main() |
3.2 字体对话框(QFontDialog)
字体对话框 — QFontDialog |
|
python from PyQt6.QtWidgets import (QMainWindow, QWidget, QHBoxLayout, QVBoxLayout, QPushButton, QSizePolicy, QLabel, QFontDialog, QApplication) import sys class QWindow(QMainWindow): def __init__(self): super().__init__() self.initUI() def initUI(self): self.lbl = QLabel('There is some message for testing the function to open fontdialog and change the font.', self) btn = QPushButton('QFontDialog', self) btn.setSizePolicy(QSizePolicy.Policy.Fixed, QSizePolicy.Policy.Fixed) # 连接字体对话框 btn.clicked.connect(self.showDialog) btn.resize(100,50) hbox = QHBoxLayout() hbox.addStretch(1) hbox.addWidget(btn) vbox = QVBoxLayout() vbox.addWidget(self.lbl) vbox.addLayout(hbox) widget = QWidget() widget.setLayout(vbox) self.setCentralWidget(widget) self.show() def showDialog(self): # 打开字体对话框,并返回所选字体对象和点击信息 font, ok = QFontDialog.getFont() # 判断是否点击了确定(True,False) if ok: # 字体赋给标签 self.lbl.setFont(font) def main(): app = QApplication(sys.argv) ex = QWindow() sys.exit(app.exec()) if __name__ == '__main__': main() |
4 文件对话框(QFileDialog)
4.1 单导入(getOpenFileName)
单文件导入 — getOpenFileName |
|
python from PyQt6.QtWidgets import (QMainWindow, QWidget, QVBoxLayout, QHBoxLayout, QTextEdit, QPushButton, QSizePolicy,QFileDialog, QApplication) from PyQt6.QtGui import QIcon, QAction from pathlib import Path import sys class QWindow(QMainWindow): def __init__(self): super().__init__() self.initUI() def initUI(self): self.textEdit = QTextEdit() btn = QPushButton('单文件导入', self) btn.setSizePolicy(QSizePolicy.Policy.Fixed, QSizePolicy.Policy.Fixed) # 连接文件对话框 btn.clicked.connect(self.showDialog) btn.resize(100,50) hbox = QHBoxLayout() hbox.addStretch(1) hbox.addWidget(btn) vbox = QVBoxLayout() vbox.addWidget(self.textEdit) vbox.addLayout(hbox) widget = QWidget() widget.setLayout(vbox) self.setCentralWidget(widget) self.setGeometry(300, 300, 550, 450) self.show() def showDialog(self): # 标记用户主目录 path_default = str(Path.home()) # Path.home()返回用户主目录 # 创建文件对话框 fname = QFileDialog.getOpenFileName(self, '单文件导入', path_default, ('Catch txt (*.*)')) # QFileDialog.getOpenFileName(self, 标题, 对话框工作目录, [过滤器:指定扩展名]) # 判断是否点选文件 if fname[0]: # print(fname) # 返回:('C:/Users/WP02118660/Documents/BOM_Macro/01_FT/G08 ture fahrer/DS113339001.187', 'Catch txt (*.*)') # 打开所选文件 # f = open(fname[0], 'r') # with f: # 56+57等同58 with open(fname[0], 'r') as f: # 读取信息并记录在多行文本框中 self.textEdit.setText(f.read()) def main(): app = QApplication(sys.argv) ex = QWindow() sys.exit(app.exec()) if __name__ == '__main__': main() |
4.2 多导入(getOpenFileNames)
多文件导入 — getOpenFileNames |
|
python def showDialog(self): # 标记用户主目录 path_default = str(Path.home()) # 创建文件对话框 fnames = QFileDialog.getOpenFileNames(self, '多文件导入', path_default, ('Catch txt (*.*)')) # QFileDialog.getOpenFileNames(self, 标题, 对话框工作目录, [过滤器:指定扩展名]) # 判断是否点选文件 if fnames[0]: # 循环所有文件并导入 for fname in fnames[0]: with open(fname, 'r') as f: # 读取信息并记录在多行文本框中 self.textEdit.append(f.read()) |
4.3 文件保存(getSaveFileName)
文件保存 — getSaveFileName |
|
python def showDialog(self): # 标记用户主目录 path_default = str(Path.home()) # 创建文件对话框 fname = QFileDialog.getSaveFileName(self, '另存为', path_default, ('txt (*.*)')) # QFileDialog.getSaveFileName(self, 标题, 对话框工作目录, [过滤器:指定扩展名]) # 判断是否选择路径 if fname[0]: with open(fname[0], 'w') as f: # 多行文本框数据导入文本 f.write(self.textEdit.toPlainText()) |
5 打印类对话框
5.1 页面设置(QPageSetupDialog)
页面设置 — QPageSetupDialog |
|
python # 创建打印机对象 self.printer = QPrinter() def pagesettings(self): # 创建页面设置对话框 printsetdialog = QPageSetupDialog(self.printer,self) # 运行对话框 printsetdialog.exec() |
5.2 打印对话框(QPrintDialog)
打印对话框 — QPrintDialog |
|
python def printdialog(self): # 创建打印对话框 printdialog = QPrintDialog(self.printer,self) # 用户点击确定后执行 if QDialog.DialogCode.Accepted == printdialog.exec(): # 打印多行文本框数据 self.tx.print(self.printer) |
6 进度条对话框
进度条对话框 |
|
python from PyQt6.QtWidgets import (QApplication, QMainWindow, QWidget, QVBoxLayout, QPushButton, QTextEdit, QMessageBox, QProgressDialog) from PyQt6.QtCore import Qt import sys class QWindow(QMainWindow): def __init__(self): super().__init__() self.initUI() def initUI(self): self.te = QTextEdit('进度对话框',self) self.bt1 = QPushButton('开始',self) self.bt1.clicked.connect(self.showDialog) vbox = QVBoxLayout() vbox.addWidget(self.te) vbox.addWidget(self.bt1) widget = QWidget() widget.setLayout(vbox) self.setCentralWidget(widget) self.resize(300,150) self.show() def showDialog(self): # 创建进度条对话框 progress = QProgressDialog(self) # 设置进度条对话框标题 progress.setWindowTitle('提示') # 设置进度条对话框说明 progress.setLabelText('运行中,请稍候') # 设置进度条对话框按钮 progress.setCancelButtonText("取消") # 设置进度条对话框出现之前必须经过的时间 progress.setMinimumDuration(5) # 进度条对话框模态 progress.setWindowModality(Qt.WindowModality.WindowModal) # Qt.WindowModality.NonModal 不是模态 # Qt.WindowModality.WindowModal 单窗口是模态,阻止父各级窗口输入 # Qt.WindowModality.ApplicationModal 应用程序是模态,组织一切窗口输入 # 设置进度条对话框范围 progress.setRange(0,100000) for i in range(100000): # 设置进度条对话框当前进度 progress.setValue(i) # 判断是否点击取消按钮 if progress.wasCanceled(): QMessageBox.warning(self,'提示','已取消',) break else: progress.setValue(100000) QMessageBox.information(self,'提示','运行成功') if __name__ == '__main__': app = QApplication(sys.argv) ex = QWindow() sys.exit(app.exec()) |