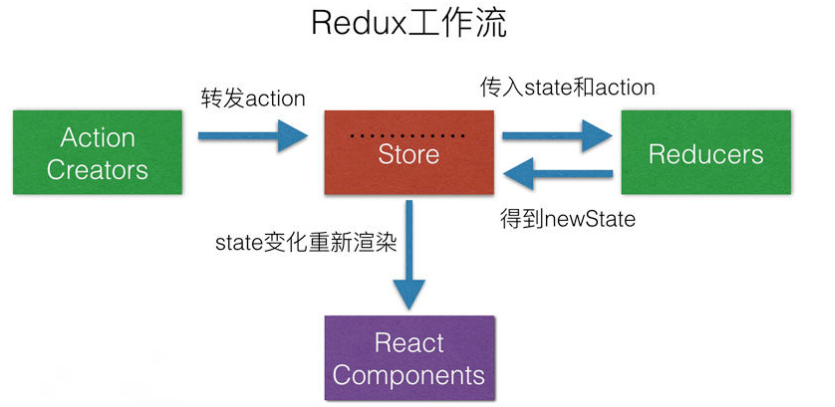
store
--index.js
--reducer.js
1.创建store(容器),管理数据
//1.创建store导入下面的文件 index.js
import {createStore} from 'redux' //1.引入
import reducer from './reducer' //3.导入
//createStore()里面只能接受函数
//3.
let store=createStore(reducer,window.__REDUX_DEVTOOLS_EXTENSION__ && window.__REDUX_DEVTOOLS_EXTENSION__()) //配置redux调式工具
export default store;
2.创建reducer,接收action,重新计算state
//reducer.js
//2.定义默认的状态
const defaultState={
msg:"这是关于redux"
}
export default(state=defaultState,action)=>{
return state
}
3.在组件中导入store,使用
import store from '../store/index'
this.state=store.getState()
4.组件中派发action,通过一个事件
<p>{this.state.msg}</p>
<button onClick={this.handleClick}>改变reducex</button>
handleClick=()=>{
//创建action
const action={
type:"btn_value",
value:"redux很难用"
}
//派发action
store.dispatch(action)
}
5.store自动接收action,之后将action传递给reducer
//reducer可以接收state,但是不能直接修改state
export default(state=defaultState,action)=>{
console.log(action)
switch(action.type){
case "btn_value":
var newState={...state};
newState.msg=action.value;
return newState;
default:
return state
}
}
class Home extends Component {
constructor(props){
...
this.state=store.getState()
store.subscribe(this.handleSotreChange)
}
handleSotreChange=()=>{
this.setState(store.getState())
}
}